I started off by playing with different shapes and ways to depict the image. Here are some of the variations I tried.
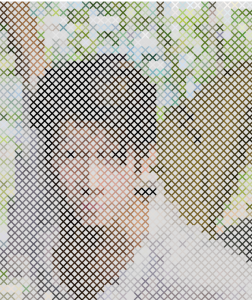
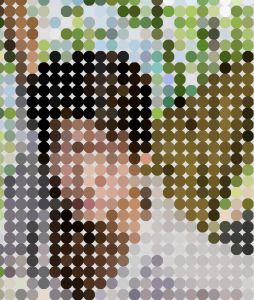
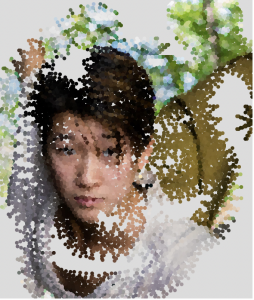
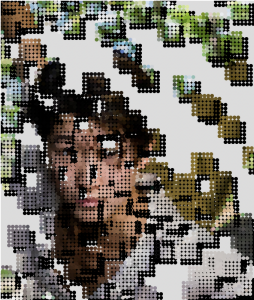
The variation I chose drew the image with randomly bouncing particles. When pressing a key, the color of the particles would be rainbow. When pressing the mouse, larger, black particles moved around the page to act as “erasers”.
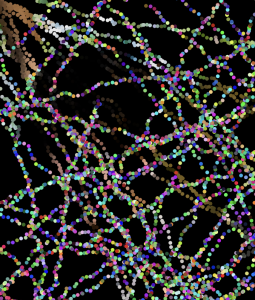
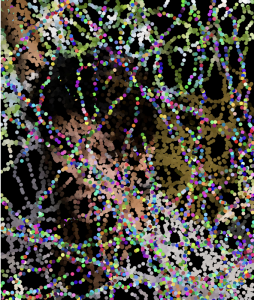
//Alana Wu
//ID: alanawu
//Project 09
var img;
var balls = [];
var ballNum = 15;
function preload()
{
img = loadImage ("https://i.imgur.com/2U02mf4.jpg");
}
function makeBall (ax, ay, adx, ady)
{
var a = {
x: ax,
y: ay,
dx: adx,
dy: ady,
dirX: 1,
dirY: -1,
stepFunction: moveBall,
drawFunction: drawBall
}
return a;
}
function moveBall ()
{
//bounce off of walls
if (this.x >= 338 || this.x <= 0)
{
this.dirX *= -1;
}
if (this.y >= 400 || this.y <=0)
{
this.dirY *= -1;
}
this.x += this.dx*this.dirX + random(-3, 3);
this.y += this.dy*this.dirY + random(-3, 3);
}
function drawBall ()
{
//color of ball = pixel from image in that location
var col = img.get(this.x, this.y);
fill (col);
//when key is pressed, taste the rainbow :)
if (keyIsPressed)
{
fill (random(255), random(255), random(255));
}
//draws balls
circle (this.x, this.y, 5, 5);
//when mouse is pressed, black jittery particles that act as erasers
if (mouseIsPressed)
{
fill (0);
circle (this.x + random(40), this.y + random(40), 20);
}
}
function setup()
{
createCanvas(338, 400);
background(0);
noStroke();
//fits image to canvas size
img.resize(width, height);
//makes objects for each ball
for (var i = 0; i < ballNum; i++)
{
a = makeBall (0,0, random(5), random(5));
balls.push(a);
}
}
function draw()
{
for (var i = 0; i < balls.length; i++)
{
balls[i].drawFunction();
balls[i].stepFunction();
}
}
//other shapes and ideas I played with, but didn't use
function ripple2 (size)
{
for (var x = 0; x < size; x+=5)
{
for (var y = 0; y < size; y+=5)
{
fill (0);
circle (mouseX + x + 8, mouseY + y + 8, 5);
circle(mouseX - x + 8, mouseY - y + 8, 5);
}
}
for (var x = 0; x < size; x+=5)
{
for (var y = 0; y < size; y+=5)
{
var col = img.get(mouseX + x,mouseY +y);
fill(col);
circle (mouseX + x, mouseY + y, 5);
var col2 = img.get(mouseX - x, mouseY - y);
fill (col2);
circle(mouseX - x, mouseY - y, 5);
}
}
}
function shape1 (x, y, dx, dy) //diagonal lines
{
for (var y = 0; y < height; y += dy)
{
for (var x = 0; x < width; x += dx)
{
var col = img.get(x, y);
strokeWeight (mouseX/100);
stroke (col);
line (x, y, x + dx, y + dy);
}
}
}
function shape2 (x, y, size) //circles, animated if w/ random
{
for (var y = 0; y < height; y += size)
{
for (var x = 0; x < width; x += size)
{
var col = img.get(x, y);
fill(col);
circle (x, y, size);
}
}
}
function shape3 (x, y, w, h) //moving ellipse in a ripple effect
{
for (var y = 0; y < height; y += h)
{
for (var x = 0; x < width; x += 15)
{
var col = img.get(x, y);
fill(col);
ellipse (x, y, random(35), h);
}
}
}
function shape4 (x, y, size) //triangles that slowly get bigger along the diagonal
{
for (var y = 0; y < height; y += size/3)
{
for (var x = 0; x < width; x += size)
{
var col = img.get(x, y);
fill(col);
triangle (x, y, x + size, y + size, x - size, y + size);
}
size += size/10;
}
}
function ripple ()
{
var x = 0;
var y = 0;
var r = 5;
push();
for (var j = 0; j < count; j++)
{
for (var i = 0; i < count; i++)
{
x = r*cos(radians(i*200));
y = r*sin(radians(i*200));
var col = img.get(mouseX + x, mouseY + y);
fill (col);
circle (mouseX + x, mouseY + y, 5, 5);
}
r += 5;
}
pop();
}
function drawIt () //uncovers image w/ mouse location
{
var col = img.get(mouseX, mouseY);
fill (col);
circle (mouseX, mouseY, 15, 15);
}