wpf-portraits.js
//Patrick Fisher, Section B, wpf@andrew.cmu.edu Assignment -09-project
let img;
let drawState = 0; //variable to change the type of drawing that is happeing
let x2 = 5; //startin x variable for the bouncing drawing
let y2 = 5; //startin y variable for the bouncing drawing
let r2 = 5; //startin radius variable for the bouncing drawing
let dx2; //delta for x for bouncing drawing
let dy2; //delta for y for bouncing drawing
var ly1; //first end of the line for the line drawing
var ly2; //second end of the line for line drawing
var lx; //mid x point of the line for line drawing
function preload() {
img = loadImage('https://i.imgur.com/HFzVcQF.jpeg');
}
function setup() {
createCanvas(480, 480);
img.resize(480,480); //fits image to canvas
imageMode(CENTER);
noStroke();
background(255);
img.loadPixels();
dx2 = floor(random(1,10)); //sets delta x
dy2 = floor(random(1,10)); //sets delta y
while(dy2 == dx2){ //makes sure the deltas are not the same
dy2 = floor(random(1,10));
}
ly1 = random(0,height); //sets the random line locations
ly2 = random(0,height);
lx = random(50,width-50);
}
function draw() {
if(drawState == 0){ //line drawings
push();
strokeWeight(4);
let pix = img.get(lx,(ly1+ly2)/2);
stroke(pix,128);
line(lx-50,ly1,lx+50,ly2);
ly1 = random(0,height);
ly2 = random(0,height);
lx = random(50,width-50);
pop();
}
if(drawState == 1){ //bouncing drawing
let pix = img.get(x2,y2);
fill(pix,128);
circle(x2,y2,2*r2);
x2 += dx2;
y2 += dy2;
if(x2 >= width){
dx2 = -dx2;
}
if(y2 >= height){
dy2 = -dy2;
}
if(x2 <= 0){
dx2 = -dx2;
}
if(y2 <= 0){
dy2 = -dy2;
}
}
if(drawState == 2){ // square loop drawing
var xy3 = mouseX/10;
var rcnum = height/(xy3+1); //variable for the width of the square based on how many squares there are
background(255);
for(var col = 0; col <=xy3; col++){
for(var row = 0; row <= xy3; row++){
let pix = img.get((row*(rcnum))+(rcnum)/2,(col*(rcnum))+(rcnum)/2);
fill(pix,128);
square(row*(rcnum),col*(rcnum),(rcnum));
}
}
}
}
function mousePressed (){ //changes the drawing with a press of the mouse
if(drawState == 0) {
background(255);
drawState = 1;
}
else if(drawState == 1){
background(255);
drawState = 2;
}
else if(drawState == 2){
background(255);
drawState = 0;
}
}
This project was a lot of fun. I had fun coming up with different ideas of how the photo could be revealed and while not simple, the coding process was rewardingly not strenous. I did have a lot of difficulty with imgur and p5.js trusting my image at first, including a few people commenting mean things, which was annoying.
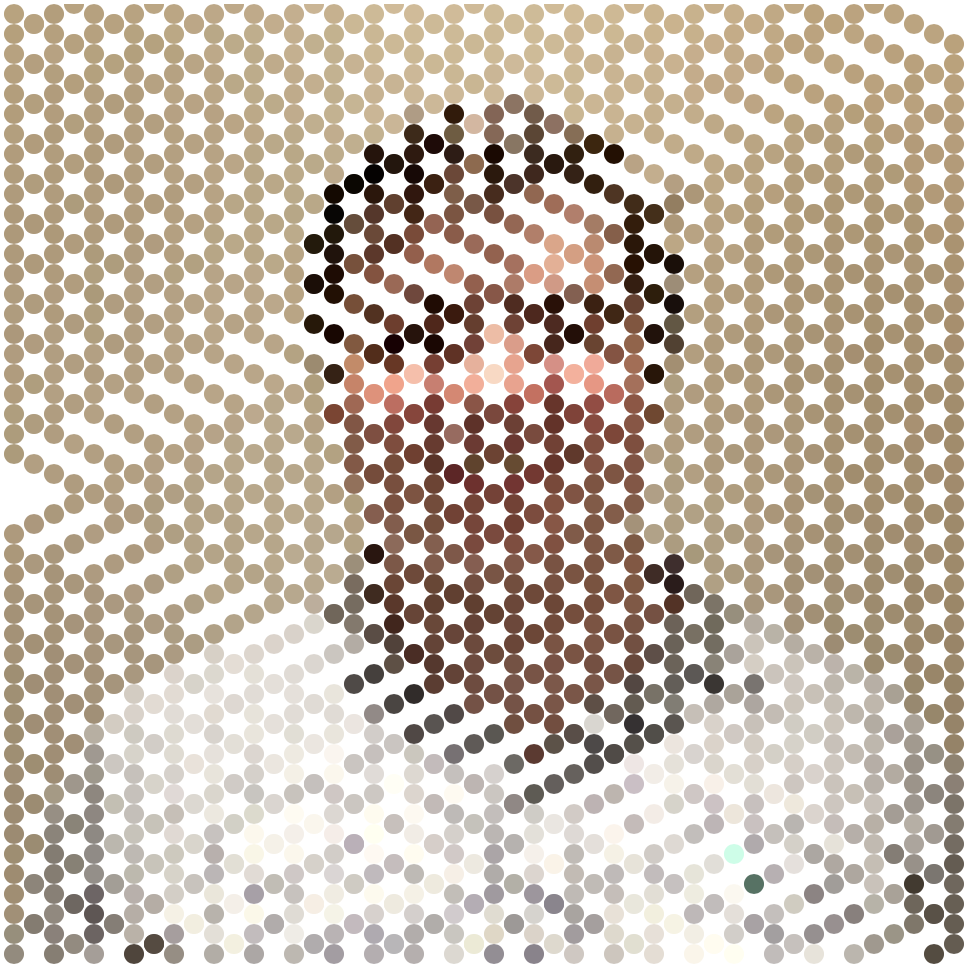
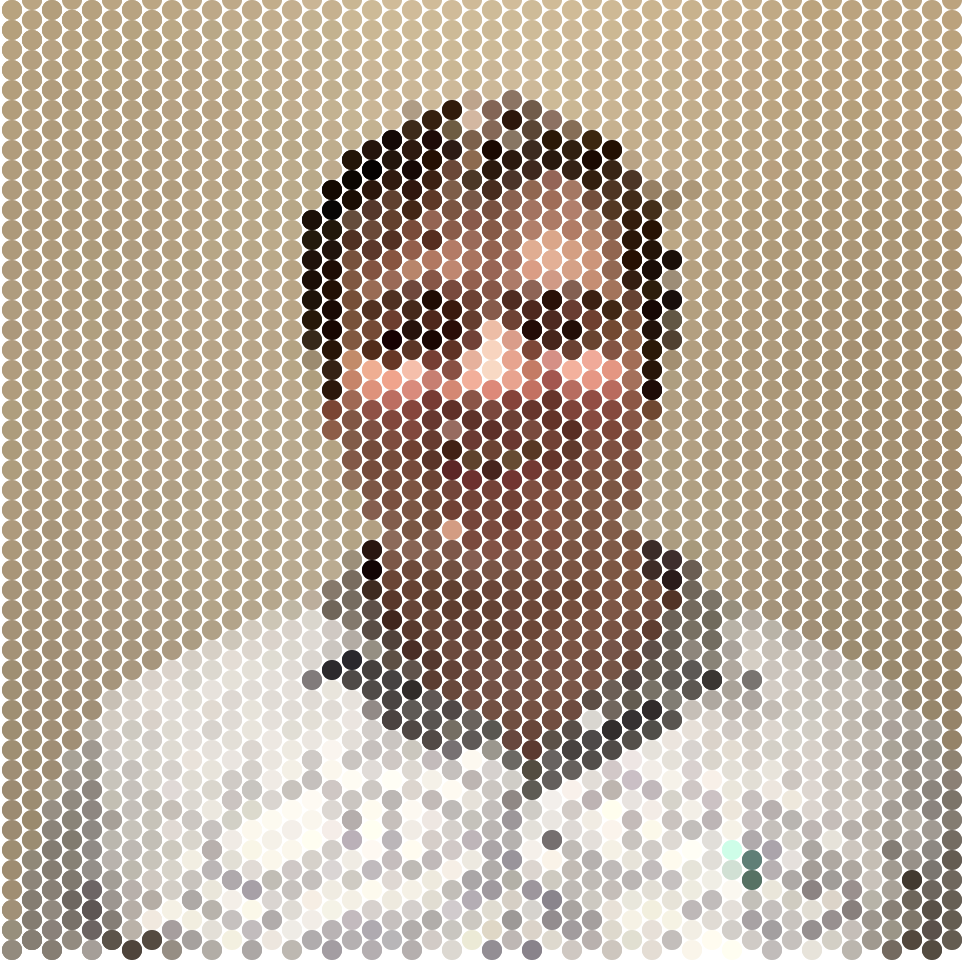
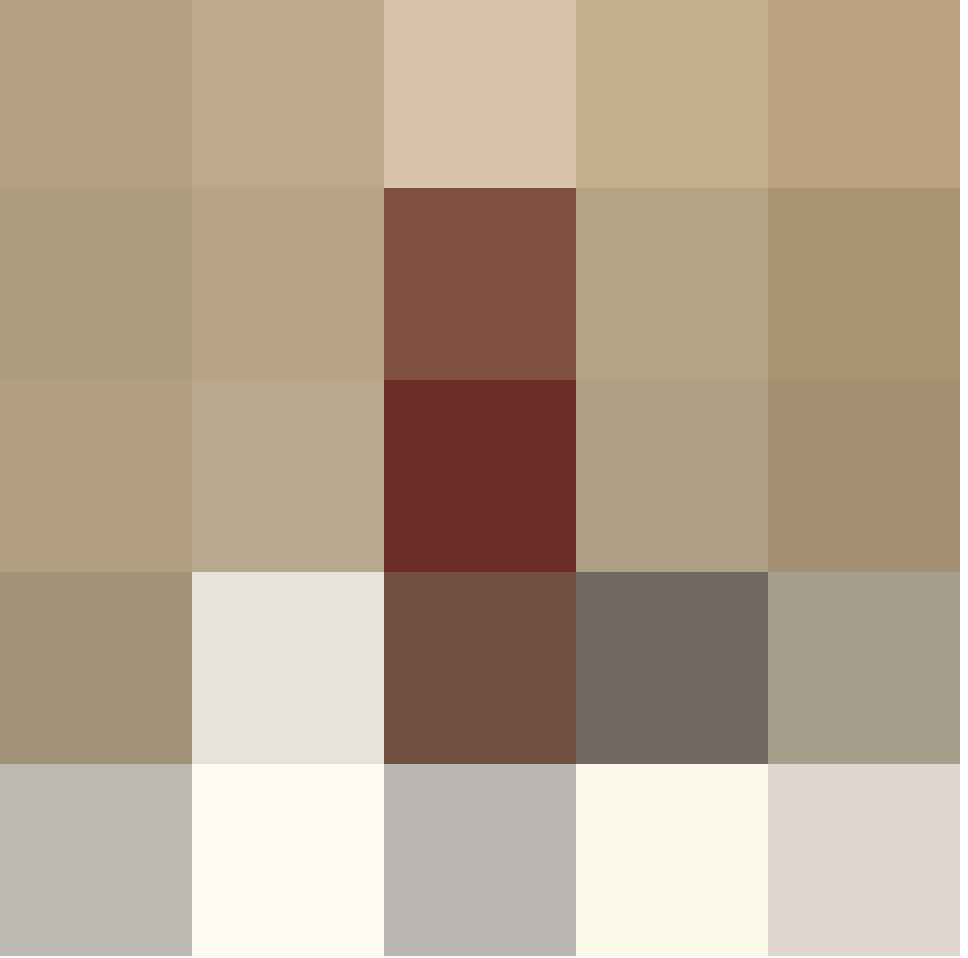
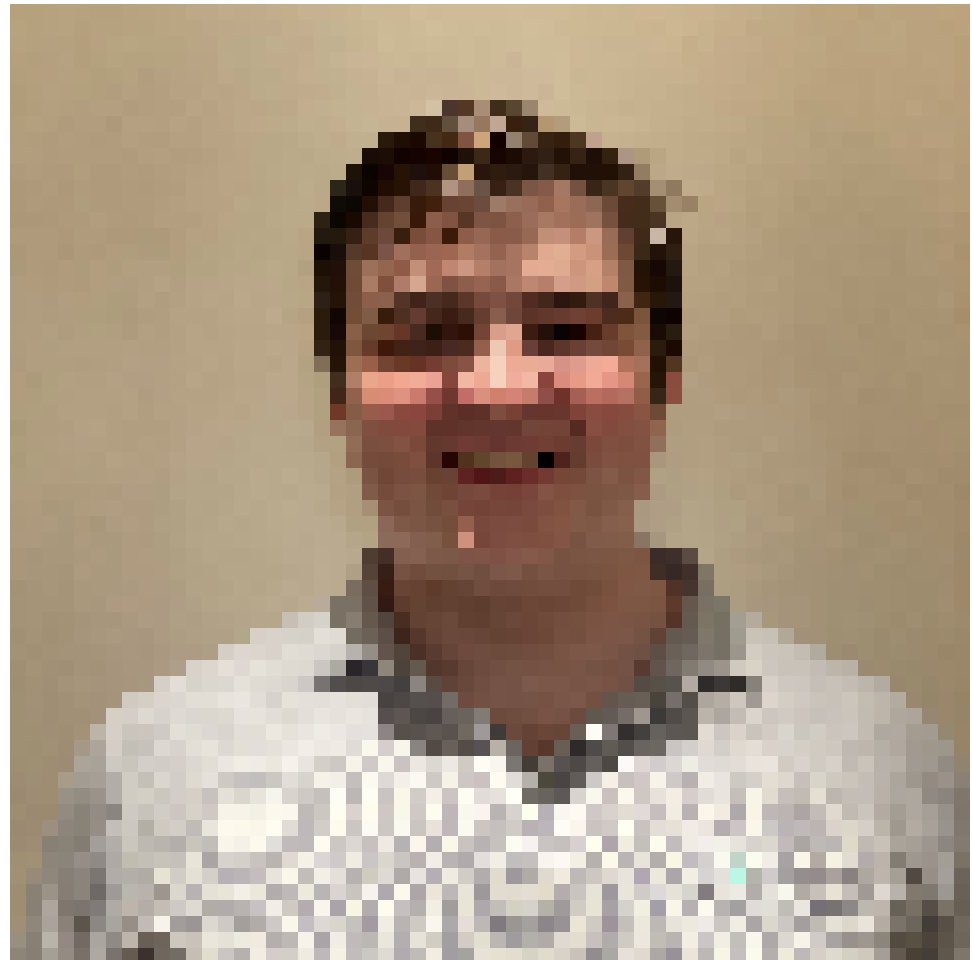
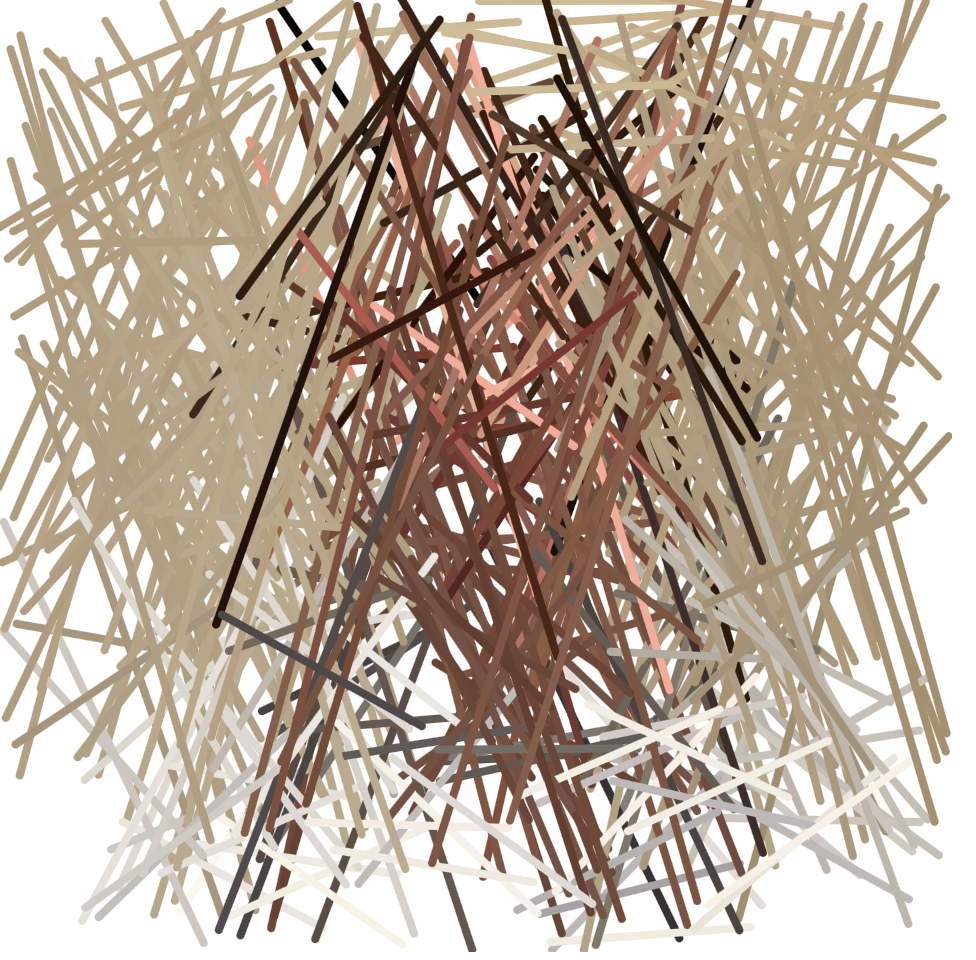
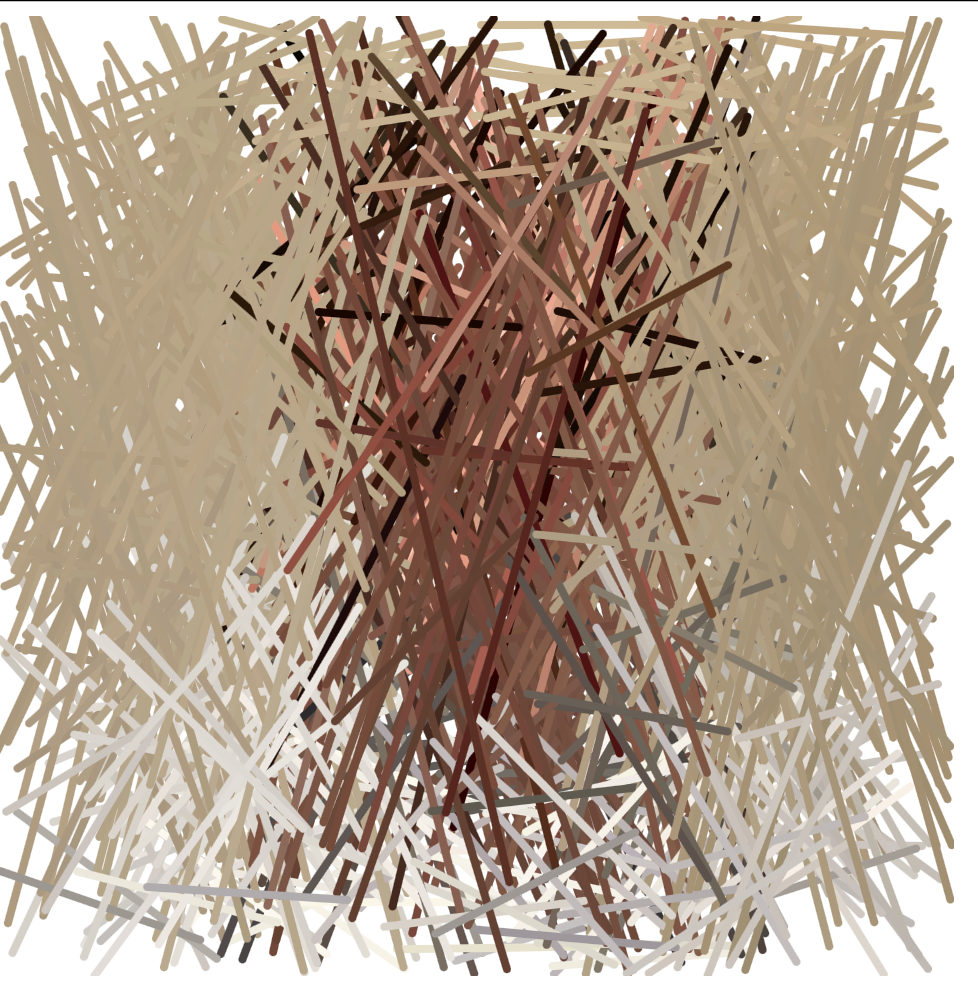