//Alana Wu
//ID: alanawu
//Project 11
var planets = [];
var stars = [];
var dots = [];
function setup()
{
createCanvas(400, 400);
for (var i = 0; i < 2; i++) //creates initial planets
{
var pX = random(width);
var pC = [random(50, 255), random(50, 255), random(50, 255)];
var pSize = random (20, 120);
var faceNum = random (1);
var o = random (0,1);
planets[i] = makePlanet(pX, pC, pSize, faceNum, o);
}
for (var i = 0; i < 5; i++) //creates initial dot clusters
{
var aX = random(50, width*2-50);
var aCol = [random(0, 255), random(0, 255), random(0, 255), 75];
var aSize = random (10, 150);
var locationX = [];
var locationY = [];
for (var j = 0; j < 200; j++)
{
var bX = randomGaussian(0, 30);
locationX.push(bX);
var bY = randomGaussian(0, 30);
locationY.push(bY);
}
dots[i] = makeDots (aX, aCol, aSize, locationX, locationY);
}
frameRate(10);
}
function draw()
{
background (0);
updateAndDisplayDots();
removeDots();
addDots();
updateAndDisplayStars();
removeStars();
addStars();
updateAndDisplayPlanets();
removePlanets();
addPlanets();
astronaut();
if (keyIsPressed) //massive sun appears when key is pressed
{
sun();
}
}
function updateAndDisplayPlanets () //moves and draws planets
{
for (var i = 0; i < planets.length; i++)
{
planets[i].move();
planets[i].display();
}
}
function updateAndDisplayStars () //moves and draws stars
{
for (var i = 0; i < stars.length; i++)
{
stars[i].move();
stars[i].display();
}
}
function updateAndDisplayDots () //moves and draws dots
{
for (var i = 0; i < dots.length; i++)
{
dots[i].move();
dots[i].display();
}
}
function removePlanets() //removes planets above the canvas from array
{
var planetsKeep = [];
for (var i = 0; i < planets.length; i++)
{
if (planets[i].y + planets[i].size/2 > 0)
{
planetsKeep.push(planets[i]);
}
}
planets = planetsKeep; //remember surviving planets
}
function removeStars() //removes stars above the canvas from array
{
var starsKeep = [];
for (var i = 0; i < stars.length; i++)
{
starsKeep.push(stars[i]);
}
stars = starsKeep;
}
function removeDots() //removes dots above the canvas from array
{
var dotsKeep = [];
for (var i = 0; i < dots.length; i++)
{
dotsKeep.push(dots[i]);
}
dots = dotsKeep;
}
function addPlanets() //add new planets from bottom
{
var chance = .05;
if (random(1) < chance)
{
planets.push(makePlanet(random(width), [random(50, 255),
random (50, 255), random (50, 255)], random (20, 120), random (1)));
}
}
function addStars() //adds new stars from bottom
{
var chance = .05;
if (random(1) < chance)
{
stars.push(makeStars(random (width)));
}
}
function addDots() //adds new dots from bottom
{
var chance = .5;
if (random(1) < chance)
{
var aX = random(0, width*2-50);
var aCol = [random(0, 255), random(0, 255), random(0, 255), 75];
var aSize = random (10, 150);
var locationX = [];
var locationY = [];
for (var j = 0; j < 200; j++)
{
var bX = randomGaussian(0, 30);
locationX.push(bX);
var bY = randomGaussian(0, 30);
locationY.push(bY);
}
var a = makeDots (aX, aCol, aSize, locationX, locationY);
dots.push(a);
}
}
function makePlanet (planX, c, size, f, o) //makes planet object
{
var planet =
{ x: planX,
y: height,
size: size,
color: c,
speed: -3,
faceNum: f,
move: planetMove,
display: planetDisplay,
orbit: o
}
return planet;
}
function makeStars(sX) //makes star object
{
var star =
{x: sX,
y: height,
speed: -3,
move: starMove,
display: starDisplay
}
return star;
}
function makeDots (aX, col, size, locationX, locationY) //makes dots object
{
var dot =
{x: aX,
y: height*2,
locX: locationX,
locY: locationY,
size: size,
color: col,
speed: -3,
move: dotMove,
display: dotDisplay
}
return dot;
}
function planetMove () //moves planets upwards
{
this.y += this.speed;
}
function starMove () //moves stars upwards
{
this.y += this.speed;
}
function dotMove () //moves dots upwards
{
this.y += this.speed;
}
function planetDisplay () //draws planet
{
fill (this.color);
noStroke();
circle (this.x, this.y, this.size); //colored circle
stroke(0);
strokeWeight(this.size/20);
if (this.faceNum < .33) //dead face, surprised when mouse is pressed
{
line (this.x - this.size/6, this.y - this.size/12, this.x - this.size/6, this.y - this.size/6);
line (this.x + this.size/6, this.y - this.size/12, this.x + this.size/6, this.y - this.size/6);
push();
strokeWeight(this.size/14);
stroke(200);
noFill();
if (this.orbit > .5) //2 possible orbital rings around planet
{
arc (this.x, this.y + this.size/10, this.size*1.4, this.size/3, TWO_PI*.973, PI*1.07); //wide orbital ring
}
else
{
arc (this.x, this.y + this.size/10, this.size*1.1, this.size/6, TWO_PI*.995, PI*1.01); //narrow orbital ring
}
pop();
if (mouseIsPressed) //surprised mouth when mouse is pressed
{
noFill();
ellipse(this.x, this.y, this.size/8, this.size/10);
}
else
{
line (this.x - this.size/12, this.y, this.x + this.size/12, this.y);
}
}
else if (this.faceNum >= .33 & this.faceNum < .66) //eyes on left, smile, frown if mouse is pressed
{
fill (0);
circle (this.x - this.size/3, this.y - this.size/12, this.size/8);
circle (this.x - this.size/6, this.y - this.size/12, this.size/8);
strokeWeight (this.size/20);
noFill();
if (mouseIsPressed) //frowns when mouse is pressed
{
arc (this.x - this.size/4, this.y + this.size/12, this.size/20, this.size/30, PI, 0 , OPEN);
}
else
{
arc (this.x - this.size/4, this.y + this.size/12, this.size/20, this.size/30, 0, PI, OPEN);
}
fill (255);
noStroke();
circle (this.x - this.size/3 - this.size/20, this.y - this.size/12, this.size/18);
circle (this.x - this.size/6 - this.size/20, this.y - this.size/12, this.size/18);
}
else //eyes on right, smile, frowns if mouse is pressed
{
fill (0);
circle (this.x + this.size/3, this.y - this.size/12, this.size/8);
circle (this.x + this.size/6, this.y - this.size/12, this.size/8);
noFill();
if (mouseIsPressed) //frowns when mouse is pressed
{
arc (this.x + this.size/4, this.y + this.size/12, this.size/20, this.size/30, PI, 0, OPEN);
}
else
{
arc (this.x + this.size/4, this.y + this.size/12, this.size/20, this.size/30, 0, PI, OPEN);
}
fill (255);
noStroke();
circle (this.x + this.size/3 - this.size/20, this.y - this.size/12, this.size/18);
circle (this.x + this.size/6 - this.size/20, this.y - this.size/12, this.size/18);
}
}
function starDisplay() //draws stars
{
fill (255, 255, 200);
noStroke();
beginShape();
vertex (this.x + 10, this.y);
vertex (this.x + 3, this.y - 1);
vertex (this.x, this.y - 8);
vertex (this.x - 3, this.y - 1);
vertex (this.x - 10, this.y);
vertex (this.x - 4, this.y + 3);
vertex (this.x - 7, this.y + 10); //bottom left triangle
vertex (this.x, this.y + 5); //middle inner vertex
vertex (this.x + 7, this.y + 10);//bottom right triangle
vertex (this.x + 4, this.y + 3);
vertex (this.x + 10, this.y);
endShape();
}
function dotDisplay() //draws colored dots
//dotDisplay is called EVERY frame, so the randomGaussians change every frame
//get program to push of randomGaussian locations into an array of locaitons when a set of dots is created
//then call those same locations for every frame for that set of dots
{
push();
scale(.5);
stroke(this.color);
for (var i = 0; i < this.locX.length; i++)
{
push();
translate (this.x, this.y);
point(this.locX[i], this.locY[i]);
pop();
}
pop();
}
function astronaut () //draws astronaut hanging from moon balloon
{
//moon balloon
push();
translate (0, -30);
fill (255, 200, 50);
noStroke();
circle (width/2, height/2, 100);
triangle (width/2, height/2 + 30, width/2 - width/43, height/2 + 58, width/2 + width/43, height/2 + 58);
fill (150, 75, 0, 100);
stroke(0);
strokeWeight(3);
arc (width/2 - width/14, height/2 + height/20, 10, 10, PI/6, PI*1.8, OPEN);
arc (width/2, height/2, 20, 20, 0, PI*1.5, OPEN);
arc (width/2 + width/20, height/2 - height/17, 25, 25, PI/2, TWO_PI, OPEN);
arc (width/2 - width/15, height/2 - height/18, 15, 15, PI*2.7, PI*1.7, OPEN);
arc (width/2 - width/30, height/2 + height/12, 12, 12, TWO_PI, PI*7/8, OPEN);
arc (width/2 + width/16, height/2 + height/18, 22, 22, PI*1.7, PI, OPEN);
stroke(255);
line (width/2, height/2 + 58, width/2, height/2 + 82);
push();
translate (width*.59, height*.68);
fill(255);
push(); //balloon arm
rotate (radians(-40));
ellipse (-30, 5, 15, 50);
rotate(radians(48));
ellipse (24, 30, 15, 25); //non balloon arm
pop();
ellipse(-5, 35, 45, 55); //body
ellipse (-14, 60, 15, 35); //left leg
ellipse (6, 60, 15, 35); //right leg
push(); //helmet
rotate (radians(25));
fill (255);
ellipse (-25, 0, 10, 20);
ellipse (25, 0, 10, 20);
ellipse (0, 0, 50, 45);
fill (0, 0, 100); //navy part
ellipse (0, 0, 50, 35);
noStroke();
fill (255, 255, 255);
ellipse (14, -2, 8, 12); //large white bubble
rotate (radians(-10));
ellipse (-17, 3, 3, 5); //smaller white bubble
strokeWeight(8); //belt
stroke(220);
line (-13, 45, 24, 39);
strokeWeight(2);
stroke(0);
fill(220);
rotate(radians(-10));
rect (-11, 37, 20, 10);
pop();
pop();
pop();
}
function sun () //massive sun appears when key is pressed
{
noStroke();
fill (255, 255, 0);
circle (width/2, height/2, 300);
strokeWeight(10);
stroke(255, 255, 0);
push();
translate (width/2, height/2);
for (var i = 0; i < 36; i ++)
{
line (0, -170, 0, -230);
rotate (radians (30));
}
pop();
}
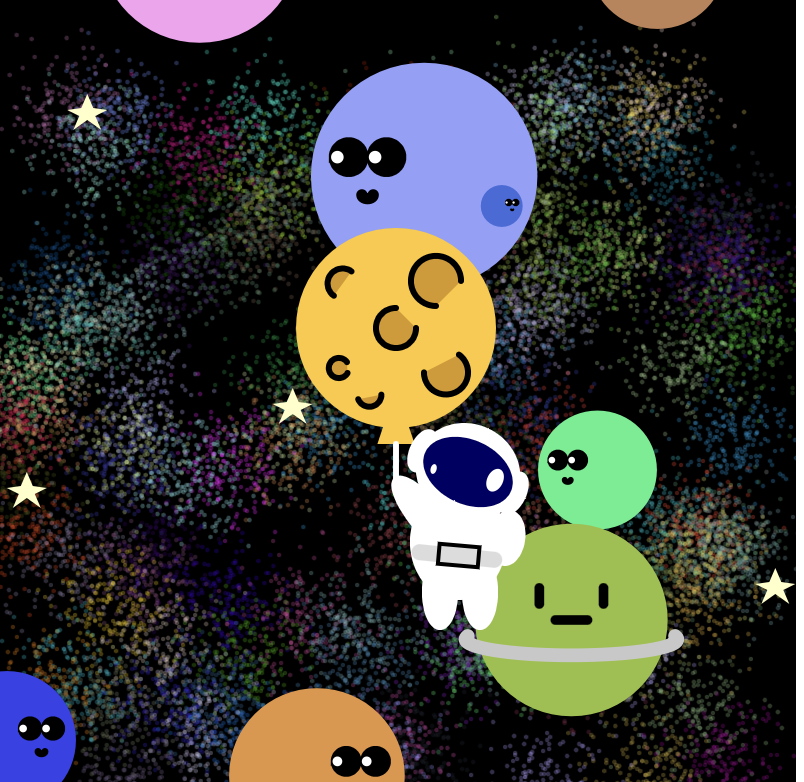
For this project, I decided to depict an astronaut floating through space. The elements I have passing by are various types of planets, individual stars, and faraway colorful clusters of stars. This project prompt let me play around with lots of fun colors and shapes, while also helping me learn how objects work. I started with the planets object, and ended with the colorful clusters of dots. I also added features where the facial expressions of the planets change when you press the mouse and a sun appears if you press a key.
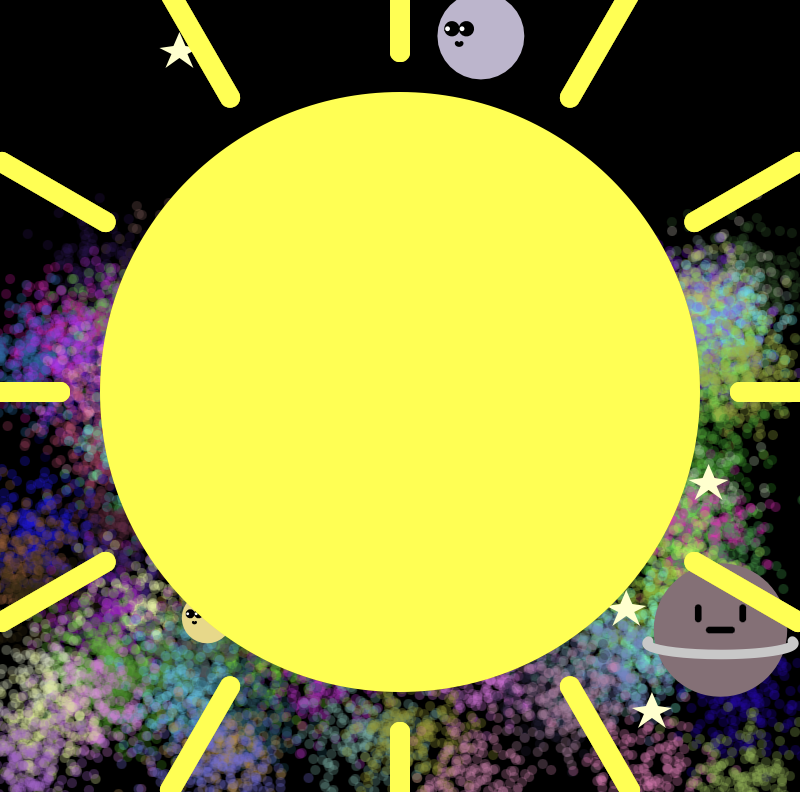