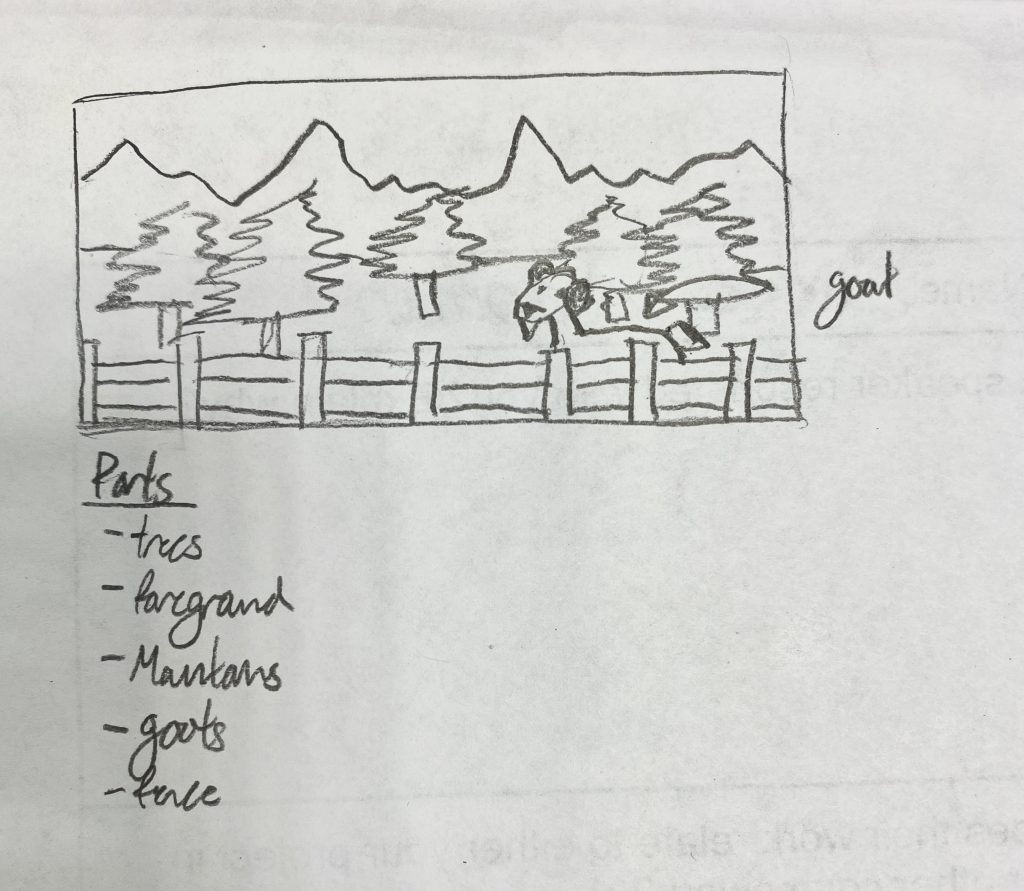
I’ve been missing my grandparents recently, so I knew I wanted to do something that reminds me of them. That means mountains. My grandfather is Austrian and he and my grandmother live in Colorado currently, so I wanted to make the scene feel like I was riding in the car in some mountainous area, complete with rolling hills, pine trees, a nice wooden fence, and a couple goats. I used a noise function for the mountains and hills at different amplitudes. The hill noise function gave me a little trouble when I started implementing my trees, but by lowering the amplitude more and limiting the range for the random tree generation, I was able to mitigate most of the floating trees. Originally, I was thinking about making the goat change color as well, but I couldn’t successfully implement it, so I left it out. Overall, I’m happy with how my landscape came out. I think that if I had more time, there are certainly more tweaks I would make, but with the time that was available to me this week I’m happy with what I made.
//Elise Chapman
//ejchapma
//ejchapma@andrew.cmu.edu
//Section D
var goats = [];
var hill = [];
var mountain = [];
var fence = [];
var trees = [];
var noiseParam = 1; //for the mountains
var noiseStep = 0.05; //for the mountains
var noiseParam2 = 5; //for the hills
var noiseStep2 = 0.005; //for the hills
function preload() {
treeImg = loadImage("https://i.imgur.com/eI7dRxU.png");
fenceImg = loadImage("https://i.imgur.com/8f0PfZu.png");
goatImg = loadImage("https://i.imgur.com/TX6vImS.png");
}
//the mountains
function mountains() {
//sets up the mountains
strokeWeight(3);
stroke(100); //dark gray
fill(100); //dark gray
//creates the mountains
translate(0,0.5);
beginShape();
vertex(0,height);
for (var i=0; i<(width/5)+1; i+=1) {
vertex(i*5,mountain[i]);
}
vertex(width,height);
endShape();
mountain.shift();
var n=noise(noiseParam);
var value=map(n,0,1,0,height);
mountain.push(value);
noiseParam+=noiseStep;
}
//the hills
function hills() {
//sets up the hills
strokeWeight(3);
stroke(27,81,45); //dark green
fill(27,81,45); //dark green
//creates the hills
translate(0,10);
beginShape();
vertex(0,height);
for (var i=0; i<(width/5)+1; i+=1) {
vertex(i*5,hill[i]);
}
vertex(width,height);
endShape();
hill.shift();
var n=noise(noiseParam2);
var value=map(n,0,1,0,height);
hill.push(value);
noiseParam2+=noiseStep2;
}
//the goats
function makeGoat(gx) {
var g = {x: gx,
goatSpeed: -3.0,
goatSizeMod: random(0,51),
move: goatMove,
display: goatDisplay}
return g;
}
function goatDisplay() {
image(goatImg, this.x+200,90-this.goatSizeMod, 100+this.goatSizeMod,100+this.goatSizeMod);
}
function goatMove() {
this.x += this.goatSpeed;
}
function updateGoats() {
//update the goat positions and display them
for (var i=0; i<goats.length; i+=1) {
goats[i].move();
goats[i].display();
}
}
function removeGoats() {
//remove goat when no longer on screen
var goatOnScreen = [];
for (var i=0; i<goats.length; i+=1) {
if (goats[i].x + 400 > 0) {
goatOnScreen.push(goats[i]);
}
}
goats = goatOnScreen;
}
function addNewGoats() {
var newGoatChance = 0.004;
if (random(0,1) < newGoatChance) {
goats.push(makeGoat(width));
}
}
//the trees
function makeTree(tx) {
var t = {x: tx,
y: 90-random(0,50),
treeSpeed: -5.0,
treeSizeMod: random(0,51),
move: treeMove,
display: treeDisplay}
return t;
}
function treeDisplay() {
image(treeImg, this.x+200, this.y, 50+this.treeSizeMod,75+this.treeSizeMod);
}
function treeMove() {
this.x += this.treeSpeed;
}
function updateTrees() {
//update the tree positions and display them
for (var i=0; i<trees.length; i+=1) {
trees[i].move();
trees[i].display();
}
}
function removeTrees() {
//remove tree when no longer on screen
var treesOnScreen = [];
for (var i=0; i<trees.length; i+=1) {
if (trees[i].x + 400 > 0) {
treesOnScreen.push(trees[i]);
}
}
trees = treesOnScreen;
}
function addNewTrees() {
var newTreeChance = 0.1;
if (random(0,1) < newTreeChance) {
trees.push(makeTree(width));
}
}
function setup() {
createCanvas(480,200);
frameRate(25);
//for the mountains
for (var i=0; i<(width/5)+1; i+=1) {
var n=noise(noiseParam);
var value=map(n,0,1,0,height);
mountain[i]=value;
noiseParam+=noiseStep;
}
//for the mountains
for (var i=0; i<(width/5)+1; i+=1) {
var n=noise(noiseParam2);
var value=map(n,0,1,0,height);
hill[i]=value;
noiseParam2+=noiseStep2;
}
//inital collection of trees
for (var i=0; i<10; i+=1) {
var rx = random(width);
trees[i]=makeTree(rx);
}
}
function draw() {
background(123, 196, 227); //sky blue
//sun
noStroke();
fill(255, 255, 227); //very light yellow
ellipse(40,30,25);
mountains();
hills();
//trees
updateTrees();
removeTrees();
addNewTrees();
//goats
updateGoats();
removeGoats();
addNewGoats();
//fence
var fenceX=0;
for (var i=0; i<5; i+=1) {
image(fenceImg, fenceX+(i*200),115, 200,100);
}
}