For this week’s looking outwards, I looked at Andrej Bauer’s work. I find it tough to interpret randomly generated art because I could not quickly identify the artist’s central message. However, I admire the visuals in Bauer’s artworks; there was a combination of attractive colors and shapes. Also, although computer algorithms randomly generate the artworks, Bauer’s pieces always have a focal point and repetitive graphic elements. This is because the algorithm that Bauer employs is pseudo-random. The artist uses a random number generator to create mathematical formulas, which determine the colors and composition. We can still consider the algorithm as random since we cannot accurately predict the outcome. Bauer declared two main variables in his algorithm to create the visuals: the first one determines the composition, and the second one decides the colors that appear. By reassigning the values in those variables, the artist can create random pictures every time. However, the same values would produce results with similar compositions or colors. Overall, I think Bauer successfully achieves randomness in his artworks with the use of randomly generated numbers and mathematical computation.
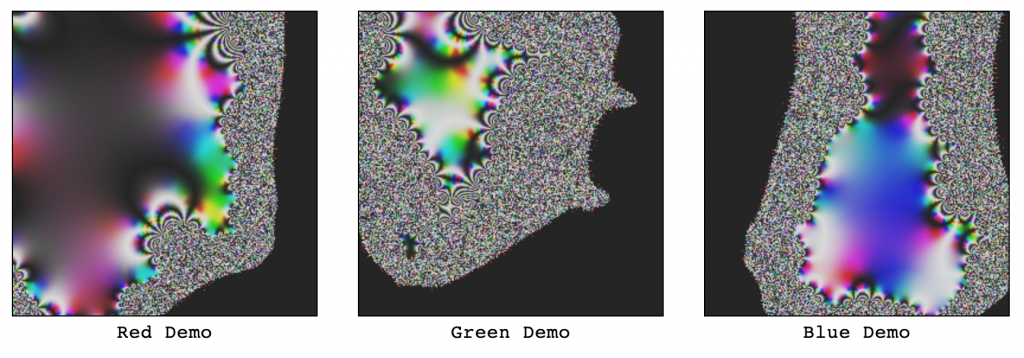
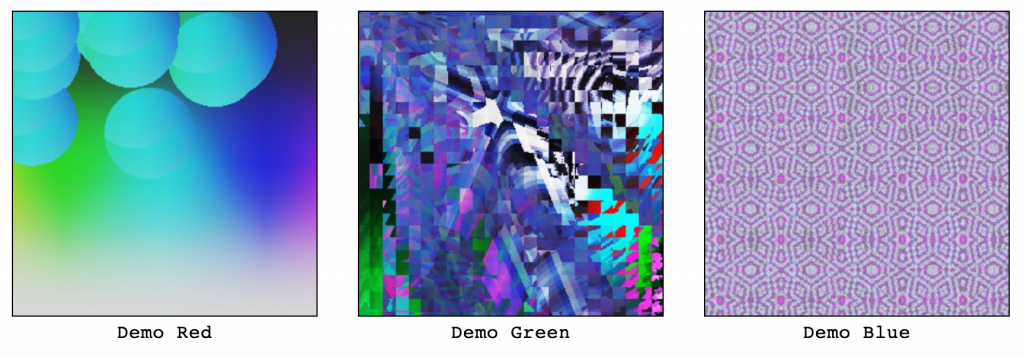