move your mouse to have the farmer tend to his animals. hover over animals for them to make noise.
Category: SectionA
Project 09 – Portrait
//Brandon Yi
//btyi@andrew.cmu.edu
//Section A
//Initialization
var img;
var counter = 0;
//preload image
function preload() {
img = loadImage('https://i.imgur.com/ox0pEHV.jpg');
}
function setup() {
img.resize(img.width/5, img.height/5); //Resizing image
createCanvas(img.width, img.height); //Canvas size based on image size
imageMode(CENTER);
rectMode(CENTER);
textAlign(CENTER);
noStroke();
background(255);
img.loadPixels();
}
//drawing shapes based on mouse position to imitate loaded image
function draw() {
if (counter == 0) {
settingNumber();
var pointcolor = img.get(mouseX, mouseY); //point color based on mouse position and image
fill(pointcolor);
rect(mouseX, mouseY, 15, 15); //draw rectangle in mouse position
}
else if (counter == 1) {
settingNumber();
var pointcolor = img.get(mouseX, mouseY);
fill(pointcolor);
ellipse(mouseX, mouseY, 15, 15); //draw circle in mouse position
}
else if (counter == 2) {
settingNumber();
var pointcolor = img.get(mouseX, mouseY);
fill(pointcolor);
rect(mouseX, mouseY, 30, 30);
}
else if (counter == 3) {
settingNumber();
var pointcolor = img.get(mouseX, mouseY);
fill(pointcolor);
ellipse(mouseX, mouseY, 30, 30);
}
else {
settingNumber();
var pointcolor = img.get(mouseX, mouseY);
fill(pointcolor);
rect(mouseX, mouseY, 50, 50);
}
}
//Changing setting number and resetting canvas
function mousePressed() {
counter++;
counter = counter % 5;
print(counter);
background(255);
}
//Setting number in label
function settingNumber() {
textSize(30);
fill(0);
rect(350, 590, 150, 50)
fill(255);
text("Setting " + (counter+1), 350, 600);
}
Project 09
I started up by pixelating my photo, then I went into the idea of creating a mirror-like, dynamic, and interactive image. Therefore, I created 1500 moving hexagons to show my image. I am satisfied with the beauty and abstractness.
var img;
var hexagon = []
function preload(){
img = loadImage("https://i.imgur.com/VGvokSI.png");
}
//making a hexagon
function makeHexagon(cx, cy, cdx, cdy) {
var h = {x: cx, y: cy, dx: cdx, dy: cdy,
drawFunction: drawHexagon
}
return h;
}
function drawHexagon(){
push()
translate(this.x,this.y)
//hexagon color is the color of the pixel it is at
this.c = img.get(10*this.x,10*this.y)
fill(this.c)
//draws the hexagon from 6 triangles
noStroke()
triangle(0,0,-s,0,-s/2,-s*sqrt(3)/2)
triangle(0,0,-s/2,-s*sqrt(3)/2,s/2,-s*sqrt(3)/2)
triangle(0,0,s,0,s/2,-s*sqrt(3)/2)
triangle(0,0,-s,0,-s/2,s*sqrt(3)/2)
triangle(0,0,-s/2,s*sqrt(3)/2,s/2,s*sqrt(3)/2)
triangle(0,0,s,0,s/2,s*sqrt(3)/2)
//hexagons would bounce backs if touches the edges
if (this.x >= width || this.x <= 0){
this.dx = -this.dx
}
if (this.y >= height || this.y <= 0){
this.dy = -this.dy;
}
this.x += this.dx;
this.y += this.dy;
pop()
}
function setup() {
createCanvas(480,480);
img.resize(4800,4800)
//make 1500 hexagons explode outwards from my left eye, pushed into array
for (var i = 0; i < 1500; i++) {
hexagon[i] = makeHexagon(random(255,260),random(230,250),random(-4,4),random(-4,4))
}
text("p5.js vers 0.9.0 test.", 10, 15);
}
var s = 14
function draw() {
background(200)
image(img,0,0,480,480)
//pixelated because having a clear image of my huge face on wordpress is horrifying
for(var x = 0; x < width; x += 10){
for(var y = 0; y < height; y += 10){
var c = img.get(10*x,10*y);
fill(color(c));
noStroke();
rect(x,y,10,10);
}
}
//draw 1500 hexagons
for (var i = 0; i < 1500; i++) {
hexagon[i].drawFunction()
}
}
LO9
Link of the :https://www.novajiang.com/projects/cacophony/
This week I looked at Nova Jiang’s interactive sculpture: Cacophony, created in 2020. Cacophony is a crystal sculpture which gives physical form to the sound of an orchestra tuning. It is generated from a recording of the Sacramento Philharmonic tuning before a performance of Stravinsky’s The Firebird. The media involved are steel, aluminum, Swarovski crystals, and lexan. It is a huge sculpture, having the dimensions of 35’ x 5’ x 5’. It presents cacophony of the opera tuning, but is also harmonic because of the crystal texture and the beautiful look. The shape of the audio is also beautiful. The artist studied in Skowhegan School of Painting and Sculpture in 2009, completed master degree in University of California, Los Angeles, Department of Design Media Arts in 2009, completed bachelor degree of fine arts in University of Auckland, Elam School of Fine Arts in 2007. She mostly do interactive sculptures and paintings. She now lives in LA.
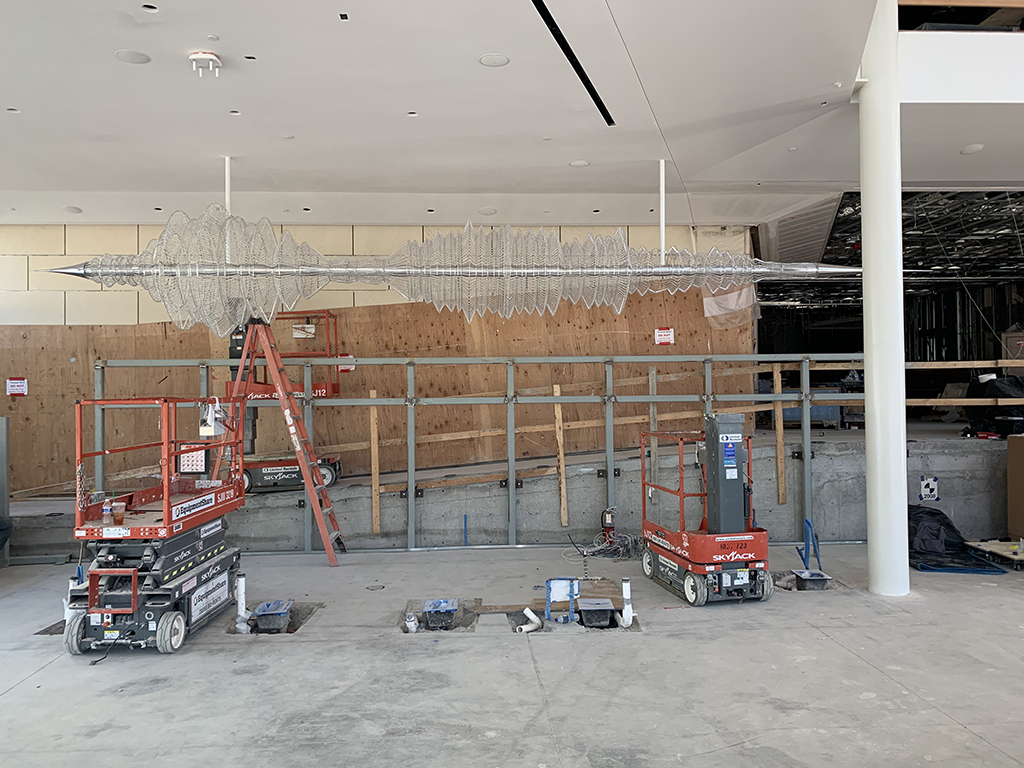
Looking Outwards – 09
I decided to look into Angela Washko and her work in feminist and alternative video games.
“The Game: The Game” is a fresh take on dating sims, as the player goes through the experience of seduction as a femme-presenting individual.
Along with the traditional dating sim mechanics, this game also provides commentary on politics, tactics and practices of the male pick-up strategies.
It creates empathy or a sense of understanding among people in these specific and hyper-gendered social situations.
I’ve always thought of video games as a platform of expression, but I hadn’t considered political or social commentary to be a particularly prominent aspect.
However, Washko and her work has brought this element into the forefront of my attention, and I will continue to look for more meaningful avenues that video games may promote in the future.
More generally, Washko’s work takes on a specific angle in interdisciplinary art, combining aspects of performance, mainstream media, digital art, cinematography and video games.
She’s a Associate Professor of Art at CMU and primarily works with the MFA program.
https://angelawashko.com/section/437138-The-Game-The-Game.html
project-09
function preload() {
var myImage="https://i.imgur.com/0PVv59G.jpeg";
loadedImage=loadImage(myImage);
}
function setup() {
createCanvas(400,400);
background(0);
loadedImage.resize(400,400);
loadedImage.loadPixels();
frameRate(100000000000000000000000000000000000000000000000000000000000);
}
function draw() {
var x=random(width);
var y=random(height);
var pixelx=constrain(floor(x),0,width);
var pixely=constrain(floor(y),0,height);
var pixelcolor=loadedImage.get(pixelx,pixely);
noStroke();
fill(pixelcolor);
square(x,y,random(0,5));
}
LO-09
I chose to look at Camille Utterback, who focuses on interactive artworks and permanent public installations, I focused on the project Precarious
Precarious was created for the National Portrait Gallery exhibition Black Out: Silhouettes Then and Now, which opened in May, 2018. Precarious is an interactive installation that extends the historical practice of tracing human silhouettes with a mechanical apparatus on a backlit screen, by instead “tracing” gallery visitors with contemporary digital tools. Utterback uses a ceiling mounted depth camera to record peoples’ silhouettes as seen from above. Her software then continually interprets and redraws this data, rendering the silhouettes not with the stark fixedness of paper cut outs, but as tremulous outlines of bodies moving through time. The painterly aesthetic of Precarious builds on the algorithmically generated visual language which Utterback has refined over many years via her custom coded interactive drawing systems.
In the Precarious system, silhouettes are never fixed. As Utterback’s software draws peoples’ outlines, each line is translated into a series of points. The points are programmed to exert an animating force on the other points, which generates ongoing momentum in the continually redrawn forms.
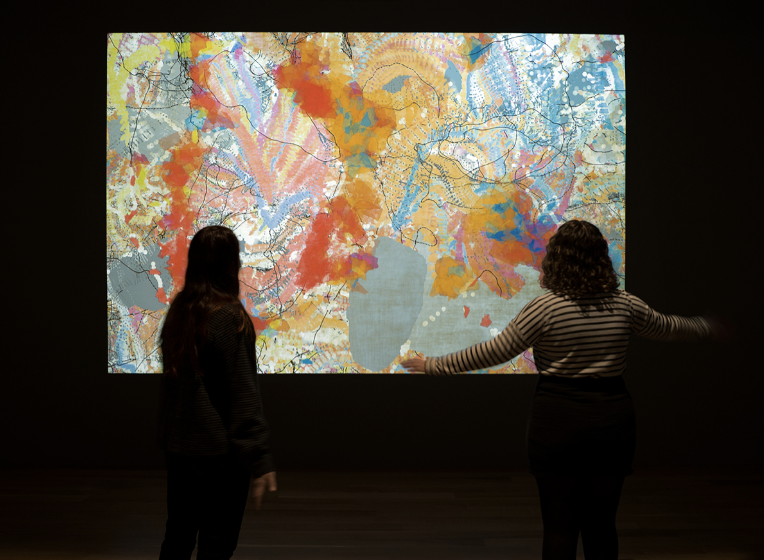
Looking Outwards – Week 9
Out of all the women artists in the list that was provided, the one the caught my attention the most was Milica Zec and her work in VR filmmaking. I watched some of her work and was hypnotized by the beautiful shots and imagery that she created in her work. I was fascinated by her work because I had never thought that VR could extend its way into the film industry. I have a VR setup at home, and I feel like the future of video games and media is flowing in the direction of Virtual reality, so to see Zec’s interesting work with VR film makes me excited to see where the future of VR will take us.
Looking Outwards 09
A particular work that I find interesting is “US Oil Fix” by Brooke Singer. Made in 2006, Singer was asked to contribute to the book “The Atlas of Radical Cartography” which was a collection of “10 maps and 10 essays about social issues from globalization to garbage”. Her contribution “is a look at the work through the lens of US oil consumption. The mission for this generative art piece is what I admire so much about it. It touches on the idea that art can create change within our world. Using art also enables a larger audience to understand and take action against a complicated idea like Oil Consumption. Brooke Singer’s artistic sensibilities manifest in this piece as they are trying to persuade a larger audience on an issue. With this mission, it forces the artists to portray their art a very specific way making them use their artistic sensibilities. In conclusion, I really enjoy looking at this piece and the message that it has to offer. The creator Brooke Singer is an Associate Professor of New Media at Purchase College at State University of New York. She works with technoscience as an artist, educator, nonspecialist and collaborator.
Assignment-08-A: Mixies
//Brandon Yi
//btyi@andrew.cmu.edu
//Section A
// loading all links
var bodyLinks = [
"http://i.imgur.com/5YM2aPE.jpg",
"http://i.imgur.com/oKtGXfd.jpg",
"http://i.imgur.com/Kvg75bG.jpg",
"http://i.imgur.com/0FGzErn.jpg",
"http://i.imgur.com/MJmlPt5.jpg",
"http://i.imgur.com/VvX0k8e.jpg",
"http://i.imgur.com/rLIOBoG.jpg",
"http://i.imgur.com/q03Gko3.jpg",
"http://i.imgur.com/BWpN5SP.jpg",
"http://i.imgur.com/ft10TV3.jpg",
"http://i.imgur.com/CGCZliN.jpg",
"http://i.imgur.com/qrlc4dK.jpg"]
var feetLinks = [
"http://i.imgur.com/oNSO0T6.jpg",
"http://i.imgur.com/OWGETX7.jpg",
"http://i.imgur.com/Zp29aVg.jpg",
"http://i.imgur.com/AXLWZRR.jpg",
"http://i.imgur.com/wgZq717.jpg",
"http://i.imgur.com/sGVMEMw.jpg",
"http://i.imgur.com/hfbrynH.jpg",
"http://i.imgur.com/OOASUMM.jpg",
"http://i.imgur.com/aqtIXi0.jpg",
"http://i.imgur.com/Eu6ruPo.jpg",
"http://i.imgur.com/mTSipwg.jpg",
"http://i.imgur.com/1GzC4Zz.jpg"]
var headLinks = [
"http://i.imgur.com/gBCZVuM.jpg",
"http://i.imgur.com/YLOXAdH.jpg",
"http://i.imgur.com/my3TqY7.jpg",
"http://i.imgur.com/lvtIB9s.jpg",
"http://i.imgur.com/gvDBfhO.jpg",
"http://i.imgur.com/JEuJ2ER.jpg",
"http://i.imgur.com/SbBOG1V.jpg",
"http://i.imgur.com/cuJ5Ao1.jpg",
"http://i.imgur.com/dqHjjig.jpg",
"http://i.imgur.com/mcFUcHf.jpg",
"http://i.imgur.com/0XKU9Dx.jpg",
"http://i.imgur.com/sD1ArAR.jpg"]
//initializing arrays in problem
var headImages = [];
var bodyImages = [];
var feetImages = [];
var head = [];
var body = [];
var feet = [];
//loading links into images array
function preload() {
for (var i = 0; i <= 11; i++) {
img = loadImage(headLinks[i]);
headImages.push(img);
img = loadImage(bodyLinks[i]);
bodyImages.push(img);
img = loadImage(feetLinks[i]);
feetImages.push(img);
}
}
function GenerateRandoms() {
//loop for different "people"
for (var i = 0; i <= 2; i++) {
//generate random head image
r = int(random(0,11));
head.push(headImages[r]);
//check for repeats
while (head[i] == head[i-1] || head[i] == head[i-2]) {
head.pop();
head.push(headImages[int(random(0,11))]);
}
//generate random body image
r = int(random(0,11));
body.push(bodyImages[r]);
//check for repeats
while (body[i] == body[i-1] || body[i] == body[i-2]) {
body.pop();
body.push(bodyImages[int(random(0,11))]);
}
//generate random feet image
r = int(random(0,11));
feet.push(feetImages[r]);
//check for repeats
while (feet[i] == feet[i-1] || feet[i] == feet[i-2]) {
feet.pop();
feet.push(feetImages[int(random(0,11))]);
}
}
}
//setup canvas
function setup() {
createCanvas(600, 450);
background(220);
GenerateRandoms();
}
function mousePressed() {
//clearing different arrays based on different mouseY positions
//generating new images for particular position
if (mouseY<(height/3)) {
head = [];
GenerateRandoms();
}
else if (mouseY>=(height/3) & mouseY <=(2*height/3)) {
body = [];
GenerateRandoms();
}
else {
feet = [];
GenerateRandoms();
}
}
//display new images in positions based on i
function draw() {
for (var i = 0; i <= 2; i++) {
image(head[i],i*200,0,200,150);
image(body[i],i*200,150,200,150);
image(feet[i],i*200,300,200,150);
}
}