//Sihan Dong
//sihand@andrew.cmu.edu
//Section B
//Project Week 09: pixel portrait
var sAngle = 5;
var sRadius = 1;
var sFrameCount = 0;
var ellipseW = 5;
var portraitW;
var portraitH;
function preload() {
var myImageURL = "http://i.imgur.com/GJ7CafK.jpg";
portrait = loadImage(myImageURL);
}
function setup() {
portraitW = portrait.width;
portraitH = portrait.height;
//canvas size is identical to that of the portrait
createCanvas(portraitW, portraitH);
background(240);
portrait.loadPixels();
}
function drawSpiral() {
//spiral starts at the middle of the canvas
translate(width/2, height/2);
var centerX = 0;
var centerY = 0;
var x = cos(radians(sAngle)) * sRadius;
var y = sin(radians(sAngle)) * sRadius;
//capture the color of the pixels
noStroke();
var theColorAtLocationXY = portrait.get(width/2 - x, height/2 + y);
fill(theColorAtLocationXY);
ellipseMode(CENTER);
ellipse(centerX - x, centerY + y, ellipseW, ellipseW);
//pixel dimensions
sAngle = sAngle + 3; //the angle between rays of pixels
sRadius = sRadius + 0.05; //the density of the spiral
sFrameCount = sFrameCount + 1; //count of number of pixels
ellipseW = ellipseW + .0003; //the increase in pixel size
//when there are more than a certain number of pixels, start over
if (sFrameCount > 10000) {
sAngle = 5;
sFrameCount = 0;
sRadius = 1;
ellipseW = 5;
background(240);
}
print(frameCount);
}
function draw() {
drawSpiral();
}
I experimented with a few images on pixel sizes, pixel distance, and spiral “density”. Overall, portraits with a focus in the middle work best for two reasons 1) when the distance between pixels is small, more details are “smudged” towards the periphery; 2) when the distance is larger, the pixels are further apart towards the periphery, therefore depicting fewer details. Below are some screenshots.
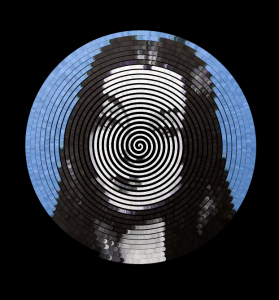
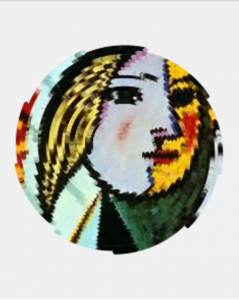