//Lan wei
//Section D
//lanw@andrew.cmu.edu
//Project-11
var img;
var repeatTime = 1; // time repeated before the painting is finished
var numEachTime = 10; // number of turtles in each repeat time
var randomYArrays = []; //nested arrays including Y in each repeat time
var randomY = []; //arrays of Y in each repeat time
var bri; //brightness of the pixel
function preload(){
img = loadImage("https://i.imgur.com/9e7ATQI.jpg?1.jpg");
}
function setup() {
createCanvas(450, 450);
img.loadPixels();
background(0);
for (var i = 0; i < repeatTime; i ++){
for (var j = 0; j < numEachTime; j ++){ //add "numEachTime" values in randomY
randomY.push(random(0, height));
}
randomYArrays.push(randomY);
randomY = [];
}
frameRate(1);
}
function draw() {
for (var i = 0; i < repeatTime; i ++){
randomY = randomYArrays[i];
for (var n = 0; n < numEachTime; n ++){
drawTurtle(-100, randomY[n]);
}
}
}
function drawTurtle(tx, ty){
var ttl = makeTurtle(tx, ty);
ttl.penDown();
//step 1
bri = brightness(img.get(ttl.x, ttl.y)); //brightness of the pixels
ttl.setColor(map(bri, 0, 100, 255, 70));
ttl.setWeight(map(bri, 0, 100, 1, 2)); //change light weight according to brightness
ttl.forward(random(20, 50));
//step 2
for(var i = 0; i < 15; i ++){
var angle = 0;
while (angle < 30){
var angleSingleTime = random(0, 20);
ttl.left(angleSingleTime);
bri = brightness(img.get(ttl.x, ttl.y)); //brightness of the pixels
ttl.setColor(map(bri, 0, 100, 255, 70));
ttl.setWeight(map(bri, 0, 100, 1, 2)); //change light weight according to brightness
ttl.forward(random(1, 3));
angle += angleSingleTime;
}
//step 3
while (angle < 90){
var angleSingleTime = random(0, 20);
ttl.right(angleSingleTime);
bri = brightness(img.get(ttl.x, ttl.y)); //brightness of the pixels
ttl.setColor(map(bri, 0, 100, 255, 70));
ttl.setWeight(map(bri, 0, 100, 1, 2)); //change light weight according to brightness
ttl.forward(random(1, 3));
angle += angleSingleTime;
}
//step 4
while (angle < 120){
var angleSingleTime = random(0, 20);
ttl.left(angleSingleTime);
bri = brightness(img.get(ttl.x, ttl.y)); //brightness of the pixels
ttl.setColor(map(bri, 0, 100, 255, 70));
ttl.setWeight(map(bri, 0, 100, 1, 2)); //change light weight according to brightness
ttl.forward(random(1, 3));
angle += angleSingleTime;
}
//step 5
bri = brightness(img.get(ttl.x, ttl.y)); //brightness of the pixels
ttl.setColor(map(bri, 0, 100, 255, 70));
ttl.setWeight(map(bri, 0, 100, 1, 2)); //change light weight according to brightness
ttl.forward(random(20, 50));
}
}
/////////////////////////////////////////////////////////
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
I planned to draw abstract ‘Sherlock’ with turtles. The process didn’t go smoothly. I tested different moving modes of the turtles and finally decided to use the one with some randomness but generally moving from the left of the canvas to the right. There are some aspects of the project that don’t reach my expectation (for example the nested loop might have some problems), but the result is good and the effect of old movie is kind of surprising.
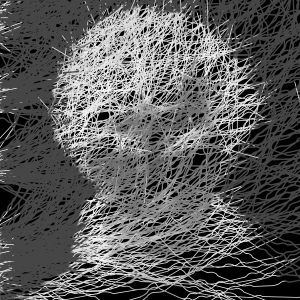
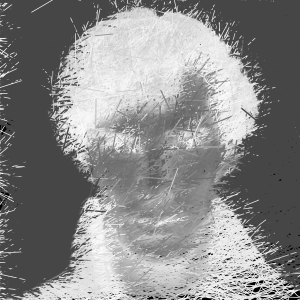