Who lives in a pineapple under the sea
/*Elena Deng
Section E
edeng1@andrew.cmu.edu
Project-10
}
*/
var Pineapples = [];
var col;
var frameB = 1;
var bubbles= [];
function setup() {
createCanvas(480, 480);
// create an initial collection of Pineapples
for (var i = 0; i < 10; i++){
var rx = random(width);
Pineapples[i] = makePineapple(rx);
}
for (var i = 0; i < 20; i++) {
var newBub = makeBubbles(random(width));
bubbles.push(newBub);
}
frameRate(38);
}
function draw() {
background(243,233,126);
displayHorizon();
waveOne();
waveTwo();
waveFour();
waveThree();
updateAndDisplayPineapples();
removePineapplesThatHaveSlippedOutOfView();
addNewPineapplesWithSomeRandomProbability();
for (var i = 0; i < bubbles.length; i++) {
bubbles[i].draw();
bubbles[i].move();
//adds the new bubbles
if ((frameB % 5 == 0) & (random(1) < .2)) {
newBub = makeBubbles(width);
bubbles.push(newBub);
}
}
}
function waveOne(){
var waterSpeed = 0.0002;
var waterDetail = 0.004;
stroke(100,200,254);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * waterDetail/4) + (millis() * waterSpeed*2);
var y = map(noise(t), 0, 1, 0, 100);
line(x, y, x, height);
}
endShape();
}
function waveTwo(){
var waterSpeed = 0.0002;
var waterDetail = 0.004;
stroke(20,100,254,70);
push();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * waterDetail) + (millis() * waterSpeed*2);
var y = map(noise(t), 0, 1, 100, 140);
line(x, y, x, height);
}
endShape();
pop();
}
function waveFour(){
var waterSpeed = 0.0002;
var waterDetail = 0.004;
stroke(20,100,254,70);
push();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * waterDetail/6) + (millis() * waterSpeed*2);
var y = map(noise(t), 0, 1, 100, 300);
line(x, y, x, height+400);
}
endShape();
pop();
}
function waveThree(){
var waterSpeed = 0.0002;
var waterDetail = 0.004;
stroke(198,186,146);
push();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * waterDetail/6) + (millis() * waterSpeed/6);
var y = map(noise(t), 0, 1, 200, 300);
line(x, y+160, x, height+150);
}
endShape();
pop();
}
function updateAndDisplayPineapples(){
// Update the Pineapple's positions, and display them.
for (var i = 0; i < Pineapples.length; i++){
Pineapples[i].move();
Pineapples[i].display();
}
}
function makeBubbles(origin) {
var bubble = {
x: origin,
y: random(height),
velX: random(-2, 1.5),
velY: random(-3, 1),
sizeF: random(20),
draw: drawBubble,
move: moveBubble
}
return bubble;
}
function removePineapplesThatHaveSlippedOutOfView(){
// If a Pineapple has dropped off the left edge,
// remove it from the array. This is quite Cutcky, but
// we've seen something like this before with particles.
// The easy part is scanning the array to find Pineapples
// to remove. The Cutcky part is if we remove them
// immediately, we'll alter the array, and our plan to
// step through each item in the array might not work.
// Our solution is to just copy all the Pineapples
// we want to keep into a new array.
var PineapplesToKeep = [];
for (var i = 0; i < Pineapples.length; i++){
if (Pineapples[i].x + Pineapples[i].breadth > 0) {
PineapplesToKeep.push(Pineapples[i]);
}
}
Pineapples = PineapplesToKeep; // remember the surviving Pineapples
}
function addNewPineapplesWithSomeRandomProbability() {
// With a very tiny probability, add a new Pineapple to the end.
var newPineappleLikelihood = 0.01;
if (random(0,1) < newPineappleLikelihood) {
Pineapples.push(makePineapple(width));
}
}
//draws the bubbles that appear at beginning
function drawBubble() {
noFill();
strokeWeight(2);
stroke(255, 255, 255, 80);
ellipse(this.x, this.y, this.sizeF * 1.5, this.sizeF * 1.5);
}
function moveBubble() {
this.x -= 1.5;
this.x += this.velX;
this.y += this.velY;
}
// method to update position of Pineapple every frame
function PineappleMove() {
this.x += this.speed;
}
// draw the Pineapple decorations door and leaves
function PineappleDisplay() {
var CutHeight = 20;
var bHeight = this.nCuts * 20;
// var bWidth = this.nCuts * 30;
push();
noStroke();
//draws leaves of Pineapple
fill(155,182,59);
translate(this.x, height - 40);
rect(10,-bHeight-20,this.breadth/2,bHeight,30);
rect(30,-bHeight-20,this.breadth/2,bHeight,30);
fill(122,167,59);
rect(40,-bHeight-30,this.breadth/4,bHeight);
fill(122,167,59);
rect(20,-bHeight-30,this.breadth/2,bHeight,30);
fill(122,167,59);
rect(40,-bHeight-50,this.breadth/4,bHeight,20);
fill(88,150,59);
rect(10,-bHeight-40,this.breadth/4,bHeight);
fill(88,150,59);
rect(15,-bHeight-50,this.breadth/4,bHeight,10);
fill(61,150,59);
rect(30,-bHeight-60,this.breadth/4,bHeight);
//draws the actual pineapple
fill(255,196,74);
stroke(230,170,50);
strokeWeight(2);
rect(0,-bHeight, this.breadth+15,bHeight,40);
//draw the decorations of the pineapple/grooves
noStroke();
fill(230,170,50);
ellipse(18,-20,10,10);
ellipse(33,-20,10,10);
ellipse(48,-20,10,10);
ellipse(33,-40,10,10);
ellipse(18,-40,10,10);
ellipse(48,-40,10,10);
ellipse(33,-60,10,10);
ellipse(18,-60,10,10);
ellipse(48,-60,10,10);
//draws the door of the pineapple
fill(0)
rect(20,-bHeight/2, 20,40,20)
pop();
}
function makePineapple(LocationX) {
var pin = {x: LocationX,
breadth: 50,
speed: -1.0,
nCuts: round(random(4,7)),
move: PineappleMove,
display: PineappleDisplay}
return pin;
}
function displayHorizon(){
function setup() {
}
}
I had a lot of fun with this project! I really loved Spongebob as a child and I was really excited to have a reason to do this subject because of the wavelike forms the the terrain tool given to us creates. If I had more time I would make the pineapple details look more like pineapples and maybe include a random sponge bob around!
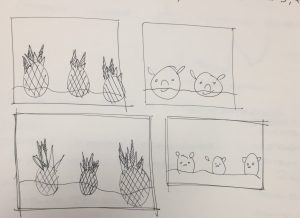