hi! welcome to my final project. please allow webcam access and sit in the center of your webcam for full effect. my project is a commentary on the artist John Baldessari. I have included a zip file below of my work as I am aware it may not run on WP. i will explain more and include photos of his work below:
sketch
var floatingText = 0
var cd = 80var myCamera;
function setup() {
createCanvas(640, 480);
myCamera = createCapture(VIDEO);
myCamera.size(640, 480);
myCamera.hide(); }
function draw() {
image(myCamera, 0, 0);
myCamera.loadPixels();
noStroke();
fill('yellow');
rect(0, 400, 640, 200); textSize(40);
textStyle(BOLD);
fill('black');
textFont('Courier New');
text('press keys a or b for a surprise', floatingText, 450);
floatingText = floatingText + 1
if (floatingText > 640) {
floatingText = -750
}
noStroke();
if (keyIsPressed) {
if ((key == 'a') || (key == 'A')) { fill('blue')
ellipseMode(CENTER);
ellipse(100, 100, cd, cd);
ellipse(200, 200, cd, cd);
ellipse(100, 200, cd, cd);
ellipse(200, 300, cd, cd);
ellipse(500, 100, cd, cd);
ellipse(600, 200, cd, cd);
ellipse(600, 300, cd, cd);
ellipse(450, 300, cd, cd);
ellipse(0, 0, cd, cd);
ellipse(0, 200, cd, cd);
ellipse(0, 300, cd, cd);
ellipse(450, 0, cd, cd);
ellipse(600, 0, cd, cd);
ellipse(260, 0, cd, cd);
ellipse(390, 0, cd, cd);
ellipse(80, 0, cd, cd);
ellipse(525, 260, cd, cd);
ellipse(200, 60, cd, cd);
ellipse(0, 150, cd, cd);
fill('yellow');
ellipse(0, 100, cd, cd);
ellipse(250, 70, cd, cd);
ellipse(200, 350, cd, cd);
ellipse(570, 290, cd, cd);
ellipse(480, 310, cd, cd);
ellipse(300, 0, cd, cd);
ellipse(640, 220, cd, cd);
ellipse(250, 300, cd, cd);
ellipse(0, 250, cd, cd);
ellipse(640, 0, cd, cd);
ellipse(210, 0, cd, cd);
ellipse(150, 210, cd, cd);
ellipse(470, 200, cd, cd);
ellipse(140, 120, cd, cd);
ellipse(600, 100, cd, cd);
ellipse(570, 30, cd, cd);
ellipse(120, 70, cd, cd);
ellipse(100, 350, cd, cd);
fill('red');
ellipse(0, 200, cd, cd);
ellipse(550, 330, cd, cd);
ellipse(550, 170, cd, cd);
ellipse(50, 170, cd, cd);
ellipse(175, 190, cd, cd);
ellipse(100, 300, cd, cd);
ellipse(640, 100, cd, cd);
ellipse(150, 0, cd, cd);
ellipse(510, 0, cd, cd);
ellipse(640, 290, cd, cd);
ellipse(450, 0, cd, cd);
ellipse(30, 77, cd, cd);
ellipse(460, 240, cd, cd);
ellipse(540, 50, cd, cd);
ellipse(160, 80, cd, cd);
ellipse(0, 350, cd, cd);
ellipse(450, 95, cd, cd);
ellipse(170, 280, cd, cd);
}
if ((key == 'b') || (key == 'B')) { fill('orange');
ellipse(100, 100, cd, cd);
ellipse(200, 200, cd, cd);
ellipse(100, 200, cd, cd);
ellipse(200, 300, cd, cd);
ellipse(500, 100, cd, cd);
ellipse(600, 200, cd, cd);
ellipse(600, 300, cd, cd);
ellipse(450, 300, cd, cd);
ellipse(0, 0, cd, cd);
ellipse(0, 200, cd, cd);
ellipse(0, 300, cd, cd);
ellipse(450, 0, cd, cd);
ellipse(600, 0, cd, cd);
ellipse(260, 0, cd, cd);
ellipse(390, 0, cd, cd);
ellipse(80, 0, cd, cd);
ellipse(525, 260, cd, cd);
ellipse(200, 60, cd, cd);
ellipse(0, 150, cd, cd);
fill('purple');
ellipse(0, 100, cd, cd);
ellipse(250, 70, cd, cd);
ellipse(200, 350, cd, cd);
ellipse(570, 290, cd, cd);
ellipse(480, 310, cd, cd);
ellipse(300, 0, cd, cd);
ellipse(640, 220, cd, cd);
ellipse(250, 300, cd, cd);
ellipse(0, 250, cd, cd);
ellipse(640, 0, cd, cd);
ellipse(210, 0, cd, cd);
ellipse(150, 210, cd, cd);
ellipse(470, 200, cd, cd);
ellipse(140, 120, cd, cd);
ellipse(600, 100, cd, cd);
ellipse(570, 30, cd, cd);
ellipse(120, 70, cd, cd);
ellipse(100, 350, cd, cd);
fill('green');
ellipse(0, 200, cd, cd);
ellipse(550, 330, cd, cd);
ellipse(550, 170, cd, cd);
ellipse(50, 170, cd, cd);
ellipse(175, 190, cd, cd);
ellipse(100, 300, cd, cd);
ellipse(640, 100, cd, cd);
ellipse(150, 0, cd, cd);
ellipse(510, 0, cd, cd);
ellipse(640, 290, cd, cd);
ellipse(450, 0, cd, cd);
ellipse(30, 77, cd, cd);
ellipse(460, 240, cd, cd);
ellipse(540, 50, cd, cd);
ellipse(160, 80, cd, cd);
ellipse(0, 350, cd, cd);
ellipse(450, 95, cd, cd);
ellipse(170, 280, cd, cd);
}
}
}
below are some screen grabs of my working project, if for some reason the webcam isn’t working you can reference these! final 104 <- here is a zip file of my project as I am aware that it is not working on the WP.
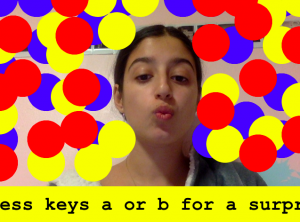
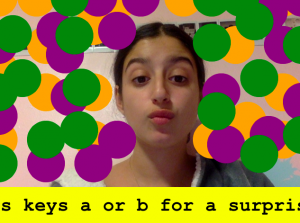
John Baldessari is an artist who is most famous for his works where he took colored sticker dots and put them over the faces of people in photographs. This act is meant to be about erasure and obliteration. For my project I decided to flip that on its head and make it about erasing the background and focusing on the individuality. The duality of color (primary colors and their complement) are meant to emphasize individuality in humanity as wellBelow is a John Baldessari image to reference:
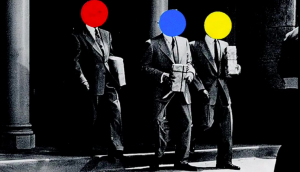