/*Eunice Choe
Section E
ejchoe@andrew.cmu.edu
Final Project*/
// options variable
var option = 1;
// scene 1
var Px = [];
var Py = [];
var Pdx = [];
var Pdy = [];
var newP = [];
// scene 2
var r = 220;
var g = 247;
var b = 255;
var cloud = [];
var landscape = 0.002;
var spot = [];
var flowers = [];
// scene 3
var img;
// spring constants (blinds pulling bar)
var springHeight = 32;
var left;
var right;
var maxHeight = 100;
var minHeight = 200;
var over = false;
var move = false;
// spring constants (main blinds)
var mass = 0.8;
var sConstant = 0.2;
var damping = 0.92;
var rest = 40;
// spring movement variables
var ps = rest; // position
var vs = 0.0; // velocity
var as = 0; // acceleration
var f = 0; // force
//scene 4
var img4;
var input;
var analyzer;
function preload() {
img = loadImage("https://i.imgur.com/IdD3GJq.png");
img4 = loadImage("https://i.imgur.com/sR307j6.png?2");
}
function setup(){
createCanvas(480, 300);
// initializing floating particles
for (i = 0; i < 100; i++) {
Px[i] = random(480);
Py[i] = random(300);
Pdx[i] = random(-5, 5);
Pdy[i] = random(-5, 5);
}
frameRate(10);
push();
angleMode(DEGREES);
// initial collection of flowers and clouds
for (var i = 0; i < 10; i++) {
var cloudX = random(width);
var rx = random(width);
cloud[i] = makeCloud(cloudX);
flowers[i] = makeFlowers(rx);
}
// initializing lefts and rights for spring
left = width/2 - 150;
right = width/2 + 150;
// initializing audio input
input = new p5.AudioIn();
input.start();
}
function draw() {
background(220, 247, 255);
noStroke();
// scene 1
if (option == 1){
scene1();
}
// scene 2
else if (option == 2) {
scene2();
}
// scene 3
else if (option == 3) {
scene3();
}
// scene 4
else if (option == 4) {
scene4();
}
}
function scene1() {
translate(width / 2, height / 2);
// flower petals
for (var i = 0; i < 20; i ++) {
fill(255, 224, 122, 90);
ellipse(0, 30, 100, 500);
rotate(PI * 8);
}
// flower center
for (var i = 0; i < 10; i ++) {
fill(84, 46, 13, 80);
ellipse(0, 0, 100, 200);
rotate(PI * 8);
}
// floating particles
for (i = 0; i < 500; i++) {
fill(255, 90);
ellipse(Px[i], Py[i], 10, 10);
Px[i] += Pdx[i];
Py[i] += Pdy[i];
}
}
function scene2(){
background(r, g, b);
if (mouseX > 0 & mouseX < width) {
r = 229 - mouseX / 20;
g = 247 - mouseX / 50;
b = 224 + mouseX / 20;
}
// grass in the back; randomizes when page refreshed
beginShape();
fill(139, 189, 125);
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = x * landscape;
var y = map(noise(t), 0, 1, 0, height / 2);
vertex(x, y + 100);
}
vertex(width, height);
endShape();
updateFlowers();
// rain when mouse is pressed
if (mouseIsPressed) {
frameRate(90);
fill(255);
spot.x = random(width);
spot.y = random(height);
ellipse(spot.x, spot.y, 5, 70);
}
// clouds
for (var i = 0; i < cloud.length; i++) {
cloud[i].draw();
cloud[i].move();
}
}
function scene3() {
image(img, 0, 0);
fill(156, 38, 27);
rect(0, 0, 100, height);
rect(380, 0, 100, height);
rect(100, 0, 280, 40);
rect(100, 260, 280, 40);
fill(242, 235, 202);
rect(90, 270, 300, 10);
fill(201, 196, 168);
quad(100, 260, 380, 260, 390, 270, 90, 270);
stroke(74, 89, 79);
noFill();
strokeWeight(10);
// sky gets darker when mouse is in window
if ((mouseX > 100) & (mouseX < 380) &&
(mouseY > 40) && (mouseY < 260)) {
fill(43, 29, 133, 50);
}
rect(100, 40, 280, 220);
updateSpring();
drawSpring();
}
function scene4() {
image(img4, 0, 0);
push();
var volume = input.getLevel();
// if the volume of the sound goes above threshold, then a firefly will appear
// fireflies are at random positions and their sizes reflect the volume
var threshold = 0.05;
if (volume > threshold) {
push();
frameRate(10);
noStroke();
fill(199, 255, 57);
ellipse(random(width), random(170, height), volume * 50, volume * 50);
pop();
}
fill(161, 156, 125);
rect(40, 120, 60, 145);
pop();
}
function updateFlowers() {
// Update the flowers positions; random when page refreshed
for (var i = 0; i < flowers.length; i++){
flowers[i].display();
}
}
function flowersDisplay() {
var floorHeight = 10;
var bHeight = this.nFloors * floorHeight * 2;
noStroke();
push();
translate(this.x, height);
fill(204, 187, 145);
rect(0, -bHeight, this.breadth, bHeight);
noStroke();
translate(0, -bHeight);
for (var i = 0; i < 20; i ++) {
fill(255, 224, 122, 90);
ellipse(0, 30, 20, 50);
rotate(PI * 8);
}
fill(84, 46, 13, 90);
ellipse(0, 0, 30, 30);
pop();
}
function makeFlowers(birthLocationX) {
var fl = {x: birthLocationX,
breadth: 5,
nFloors: round(random(2,8)),
display: flowersDisplay}
return fl;
}
function cloudDraw() {
push();
translate(this.xPos, this.yOffset);
stroke(255, 255, 255, 70);
strokeWeight(this.cHeight);
line(0, 0, this.cSize, 0);
pop();
}
function cloudMove() {
this.xPos += this.speed;
if(this.xPos < 0 - this.cSize - 30) {
this.cHeight = random(10, 50);
this.cSize = random(30, 150);
this.xPos = width + this.cSize + random(-25, 25);
}
}
function makeCloud() {
var cloud = {xPos: random(width), //, width*4
speed: random(-3, -1),
cSize: random(30, 150),
cHeight: random(20, 60),
yOffset: random(50, height),
draw: cloudDraw,
move: cloudMove};
return cloud;
}
function drawSpring() {
// draw main cream blinds
noStroke();
fill(255, 251, 243);
rect(100, ps + springHeight, 280, - height);
var baseWidth = 20;
push();
rectMode(CORNERS);
// if mouse is over gray bar, turn white
if (over || move) {
fill(255);
} else {
fill(204);
}
rect(left, ps, right, ps + springHeight);
pop();
}
function updateSpring() {
// update the spring position
if (!move) {
f = -sConstant * ( ps - rest );
as = f / mass; // acceleration
vs = damping * (vs + as); // velocity
ps = ps + vs; // updated position
}
if (abs(vs) < 0.1) {
vs = 0.0;
}
// see if mouse is over bottom bar
if (mouseX > left & mouseX < right && mouseY > ps && mouseY < ps + springHeight) {
over = true;
} else {
over = false;
}
// constrain position of bottom bar
if (move) {
ps = mouseY - springHeight/2;
ps = constrain(ps, minHeight, maxHeight);
}
}
function mousePressed() {
if (over) {
move = true;
}
}
function mouseReleased() {
move = false;
}
// move through 4 slides
function keyPressed() {
option++;
if (option > 4) option = 1;
}
My final project is based off of a book I enjoyed from childhood called Zoom, by Istvan Banyai. I tried to replicate a small glimpse of the premise of the book, which is to keep zooming out as each page is turned. My project starts off with a zoomed in sunflower and it eventually zooms out to a house. In my project, I wanted to make the zoom outs seem less static, so I incorporated movement and interactions in each scene. Some interactions include clicking for a spring effect, pressing for rain, moving the mouse around for color changes, and making sounds for objects to appear.
Instructions:
Press any key to move on to the next scene.
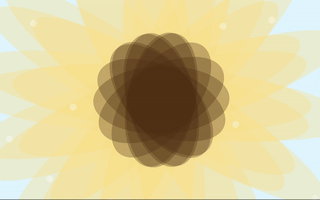
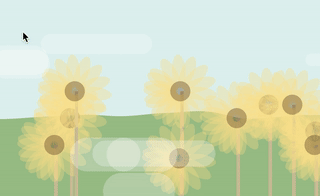
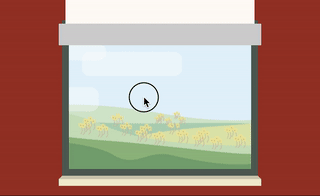
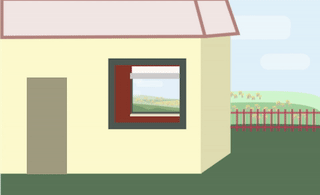