/*
Judy Li
Section A
judyli@andrew.cmu.edu
Project-07
*/
var nPoints = 100;
var EPITROCHOID = 0; // Cartesian Parametric Form [x=f(t), y=g(t)]
var EPITROCHOIDTWO = 1; // Cartesian Parametric Form [x=f(t), y=g(t)]
var titles = ["1. Epitrochoid", "2. Epitrochoid_Two"];
var curveMode = EPITROCHOID;
function setup() {
createCanvas(480, 480);
}
function draw() {
background("pink");
// draw the frame
fill(0);
noStroke();
text(titles[curveMode], 20, 40);
stroke(0);
noFill();
rect(0, 0, width-1, height-1);
// draw the curve
push();
translate(width / 2, height / 2);
switch (curveMode) {
case EPITROCHOID:
drawEpitrochoidCurve();
break;
case EPITROCHOIDTWO:
drawEpitrochoidTwo();
break;
}
pop();
}
//--------------------------------------------------
function drawEpitrochoidCurve() {
// Circle Orthotomic:
// http://mathworld.wolfram.com/Epicycloid.html
var x;
var y;
var a = 40.0;
var b = 20;
var ph = mouseX / 10.0;
fill(255, 200, 200);
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = ((a + b) * (cos(ph * t))) - (b* cos(((a + b) / b) * (ph * t)));
y = ((a + b) * (sin(ph * t))) - (b* sin(((a + b) / b) * (ph * t)));
vertex(x, y);
}
endShape(CLOSE);
}
//--------------------------------------------------
function drawEpitrochoidTwo() {
// Circle Orthotomic:
// http://mathworld.wolfram.com/Epicycloid.html
var x;
var y;
var a = 100.0;
var b = 20;
var ph = mouseX / 20.0;
fill(255, 200, 200);
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = ((a + b) * (cos(ph * t))) - (b* cos(((a + b) / b) * (ph * t)));
y = ((a + b) * (sin(ph * t))) - (b* sin(((a + b) / b) * (ph * t)));
vertex(x, y);
}
endShape(CLOSE);
}
//--------------------------------------------------
function mousePressed() {
curveMode = 1 - curveMode;
}
This project was a little bit challenging at first because I wasn’t sure which curve to choose and introduce it to the given code example. In the end, I choose to go with the epitrochoid curve because it resembles a shape I like and it’s almost like a flower/star. I like this curve because it seems like it’s being weaved into a different shape as you move your mouse from left to right. For this project, I included the two curves I liked the most, the asymmetrical shaped one, which looks like asymmetric reproduction and the other one with 5 outer petals/vertices.
Epitrochoid Curve: Link to curve
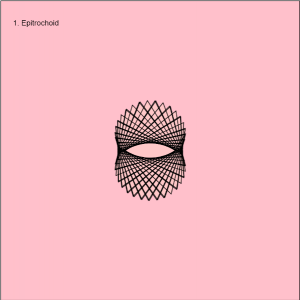
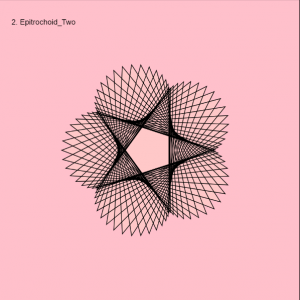