“BAD SIGNALS” and “FUZZY BLOB” were both created in the beginning of 2018. Both projects are created by Avseoul. While both projects use webgl, “BAD SIGNALS” uses the webcam as a part of their visuals, and “FUZZY BLOB” uses the microphone to make audio. I noticed the use of vibrant colors throughout both projects. I admire how the sound transitions are shown visually through change of colors, because they are bold and noticeable. For “BAD SIGNALS,” I noticed how the glitches are responsive to sound. I admire how the glitches are not subtle, but rather exaggerative to show change. “FUZZY BLOB” allows the user to interact with the webgl not only with realtime audio, but also with mouse movement (can make affects and indents on the ‘fuzzy blob’). Similar to “FUZZY BLOB,” “BAD SIGNALS” utilizes realtime audio to make glitches on the visuals, which is from the web camera. I admire both projects, because they get the user to interact with the visuals and audio. Also, these projects depend on the user’s interaction (ie the sounds made by the user/their environment, their mouse movement).
Author: sophiaki@andrew.cmu.edu
Sophia Kim – Project 12 – Proposal – Sec C
Mimi Jiao and I plan on collaborating for this final project. We want to further explore interactive sound implementation and WEBGL. We started off exploring currently existing work and discovered code that integrates sound and visuals together. They utilized the frequency and amplitude of imported songs to alter the imported image. We started off looking at how static imported images are altered based off of sound with this video and we branched off static images by looking at more dynamic and generative shapes through WEBGL. We found this interactive particle to be really interesting and cool and we definitely want to play around with geometries. Since our current skillsets are not developed enough to create something as complicated and fleshed out as this particle equalizer, we want to stay confined to shapes like ellipses, boxes, and custom shapes generated by basic math functions like sin, cos, and tan. From this, we want to play around with the idea of interacting with multiple human senses to create an experience. The audience is able to have a more heightened experience because of the mix of visuals and audio. The use of sound can make visuals easier to comprehend. In a way, the visuals will almost be a method of data visualization of the structure of the song.
Sophia Kim – Project 11 – Sec C
// Sophia S Kim
// Section C 1:30
// sophiaki@andrew.cmu.edu
// Project-11-Turtle Freestyle
var strOpt = 1;
var turtle1;
var turtle2;
function setup() {
createCanvas(480, 400);
}
function draw() {
background(175);
if (strOpt === 1) {
orangeLines();
}
if (strOpt === 2) {
redLines();
}
if (strOpt === 3) {
greenLines();
}
if (strOpt === 4) {
purpleLines();
}
}
//function for line 1 - longest line
function line1(x, y, z){
turtle1 = makeTurtle(x, y);
turtle1.setWeight(10);
turtle1.setColor(z);
turtle1.right(30);
turtle1.penDown();
for (i = 0; i < 6; i++) {
turtle1.forward(15);
turtle1.right(50);
turtle1.forward(15);
turtle1.left(50);
}
turtle1.penUp();
}
//function for line 2
function line2(a, b, n) {
turtle2 = makeTurtle(a, b);
turtle2.setWeight(10);
turtle2.setColor(n);
turtle2.left(30);
turtle2.penDown();
for (i = 0; i < 3; i++) {
turtle2.forward(15);
turtle2.left(50);
turtle2.forward(15);
turtle2.right(50);
}
turtle2.penUp();
}
//function for line 3
function line3(j, k, f) {
turtle3 = makeTurtle(j, k);
turtle3.setWeight(10);
turtle3.setColor(f);
turtle3.right(50);
turtle3.penDown();
for (i = 0; i < 4; i++) {
turtle3.forward(15);
turtle3.right(50);
turtle3.forward(15);
turtle3.left(50);
}
turtle3.penUp();
}
//function for line 4
function line4(l, m, p) {
turtle4 = makeTurtle(l, m);
turtle4.setWeight(10);
turtle4.setColor(p);
turtle4.right(-50);
turtle4.penDown();
for (i = 0; i < 4; i++) {
turtle4.forward(15);
turtle4.right(50);
turtle4.forward(15);
turtle4.left(50);
}
turtle4.penUp();
}
//function for line 5
function line5(o, h, q) {
turtle5 = makeTurtle(o, h);
turtle5.setWeight(10);
turtle5.setColor(q);
turtle5.right(-80);
turtle5.penDown();
for (i = 0; i < 5; i++) {
turtle5.forward(15);
turtle5.right(50);
turtle5.forward(15);
turtle5.left(50);
}
turtle5.penUp();
}
//draws white lines, pink shapes, and green block in background
function orangeLines() {
//makes the green block of color in background
fill("GREEN");
noStroke();
beginShape();
vertex(0, 150);
vertex(480,75);
vertex(480, 400);
vertex(0, 400);
endShape(CLOSE);
//line color orange
line1(350, 30, "ORANGE");
line2(50, 330, "ORANGE");
line3(370, 270, "ORANGE");
line4(50, 100, "ORANGE");
line5(190, 190, "ORANGE");
}
//draws pink lines, white shapes, and blue block in background
function redLines() {
//makes the blue block in background
fill("BLUE");
noStroke();
beginShape();
vertex(0, 140);
vertex(480, 200);
vertex(480, 400);
vertex(0, 400);
endShape(CLOSE);
//line color red
line1(20, 240, "RED");
line2(250, 90, "RED");
line3(100, 90, "RED");
line4(340, 360, "RED");
line5(300, 270, "RED");
}
//draws green lines, yellow shapes, and pink block in background
function greenLines() {
//makes pink block in background
fill(255, 125, 125);
noStroke();
beginShape();
vertex(0, 150);
vertex(420, 0);
vertex(480, 0);
vertex(480, 400);
vertex(0, 400);
endShape(CLOSE);
//draw green line
line1(60, 250, "GREEN");
line2(370, 350, "GREEN");
line3(390, 50, "GREEN");
line4(50, 90, "GREEN");
line5(220, 250, "GREEN");
}
//draws orange lines, blue shapes, and yellow block in background
function purpleLines() {
//makes orange block in background
fill("GOLDENROD");
noStroke();
beginShape();
vertex(0, 0);
vertex(100, 0);
vertex(480, 300);
vertex(480, 480);
vertex(0, 480);
endShape(CLOSE);
//draws purple line
line1(350, 50, "PURPLE");
line2(120, 90, "PURPLE");
line3(90, 190, "PURPLE");
line4(350, 380, "PURPLE");
line5(220, 200, "PURPLE");
}
function mousePressed() {
strOpt ++; // mouse is pressed every time changes the lines & line colors
if (strOpt > 4) {
strOpt = 1;
}
}
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
Starting this project, I really wanted crinkle-cut fries. Also, in one of the studio classes I am taking for design, we talked about color theory, so I decided to combine both the “crinkle-cut fries” idea and color theory into this project. It was definitely really fun to experiment with turtles.
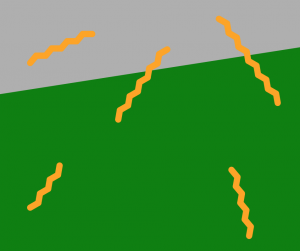
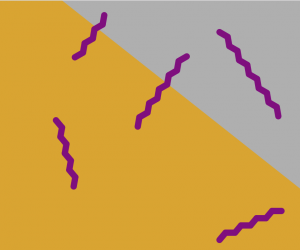
Sophia Kim – Looking Outwards 11 – Sec C
I am taking the option of using my Looking Outwards 04, focusing on the sound design of the project. “Apparatum” was created by two different teams. The “Symphony – electronic music” was composed by Boguslaw Schaeffer, and the produced analogue sounds were created by the panGenerator team.
Compared to regular music, which is prerecorded and planned, sound art uses various beats that gives texture to art, specifically installations, conceptual art, and exhibits. Like I said in Looking Outwards 04, I really liked how they fused communication, product, and sound design all together. With the sound design, the aesthetic design of the entire project is very well done. The designers of this project used various linear tape samplers as primary mediums. Also, to obtain noise and basic tones, spinning discs with graphic patterns were used, which are installed in the radio.
I really admire how different sound designs can be fused together by the user. Also, I admire how user customization is a big part of this project. The sound patterns the user picks are recorded, played out loud, and then printed on a receipt to document the patterns.
Sophia Kim – Project 10 Landscape – Sec C
// Sophia S Kim
// Section C 1:30
// sophiaki@andrew.cmu.edu
// Project-09-Portrait
var fish = [];
function setup() {
createCanvas(480, 480);
frameRate(7);
for(var l = 0; l < 10; l++) {
var fx = random(width);
fish[l] = makeFish(fx);
}
frameRate(6);
}
function draw() {
background(212, 211, 234);
sun(); //sun in the sky
mountainBackground(); //mountain
hillInFrontofMountain(); //small hill in front of mountain
waterRiver(); //river at the bottom
updateFish();
removeFish();
randomFish();
fishDisplay();
//randomizes fish in the "river"
}
// displays fish
function updateFish(){
for (var i = 0; i < fish.length; i++){
fish[i].move();
fish[i].display();
}
}
// fish disappear when hitting the edge
function removeFish(){
var fishKeep = [];
for (var q = 0; q < fish.length; q++){
if (fish[q].x + fish[q].breadth > 0) {
fishKeep.push(fish[q]);
}
}
fish = fishKeep;
}
// randomized fish to be added to the end
function randomFish() {
var updatedNewFish = 0.009;
if (random(0, 1) < updatedNewFish) {
fish.push(makeFish(width));
}
}
// update position of fish
function fishMove() {
this.x += this.speed;
}
// drawing the fish
function fishDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
push();
noStroke();
translate(this.x, height - 140);
//fish body
fill(255, 161, 210);
ellipse(3, bHeight+20, 35, 25);
//fish tail
fill(255, 161, 210);
triangle(10, bHeight+20, 30, bHeight, 30, bHeight+40);
pop();
}
function makeFish(birthLocationX) {
var fhm = {x: birthLocationX,
breadth: 5,
speed: -3.0,
nFloors: round(random(2,4)),
move: fishMove,
display: fishDisplay}
return fhm;
}
function waterRiver() {
//makes the "river" appear
fill(8, 42, 102);
rect(-1, 350, 480, 130);
}
function hillInFrontofMountain() {
//makes the hill in front of the mountain move
var terrainSpeed3 = 0.0001;
var terrainDetail3 = 0.002;
beginShape();
stroke(44, 87, 181);
for (var x3 = 0; x3 < width; x3++) {
var p = (x3 * terrainDetail3) + (millis() * terrainSpeed3);
var z = map(noise(p), 0, 1, 300, 200);
line(x3, z, x3, height);
}
endShape();
}
function mountainBackground() {
//makes mountains in the back
var terrainSpeed2 = 0.0005;
var terrainDetail2 = 0.01;
stroke(99, 129, 214);
beginShape();
for (var x2 = 0; x2 < width; x2++) {
var t = (x2 * terrainDetail2) + (millis() * terrainSpeed2);
var y2 = map(noise(t), 0, 1, 200, 10);
line(x2, y2, x2, height);
}
endShape();
}
function sun() {
fill(234, 119, 101);
noStroke();
ellipse(417, 60, 80, 80);
fill(255, 146, 128);
noStroke();
ellipse(420, 60, 75, 75);
}
As it gets colder in Pittsburgh, I started to miss the warm weather and my trip to Taiwan. For my landscape, I definitely wanted to make a view of mountains. This photo came to mind.
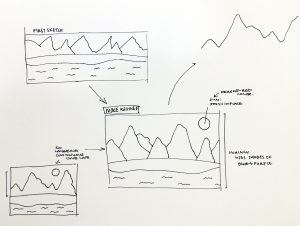
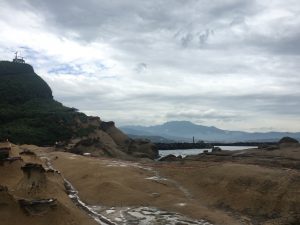
Among all the projects, this project was the hardest. I had a really hard time with making the fishes and making them move. I had a really hard time making the mountains long and keeping them consistent. Overall, I don’t really like the outcome of the final project. If I had to make changes, I would definitely add more details to the mountain.
Sophia Kim – Looking Outwards 10 – Sec C
Lauren Lee McCarthy is an artist based in Los Angeles and Brooklyn. Majority of her works focus on issues of how surveillance, automation, and network culture affect our social relationships. She is the creator of p5.js! In 2011, McCarthy received her MFA from UCLA Design Media Arts. Now, she is an assistant professor at UCLA Design Media Arts. Along with her works being exhibited internationally, McCarthy is a Sundance Institute Fellow
Among all McCarthy’s projects, I really loved her project “24h HOST,” which was collaborated with Casper Schipper. It was created in November of 2017. I approached this project mainly, because I was intrigued by the use of space and colors in this project. Reading into “24h HOST,” I admired how it used algorithms and emphasized the future role of humans in AI driven world. This project is a small, 24 hour party. Unlike most parties, this 24 hour party is driven by a software that automates the event. Every 5 minutes, one guest departs and a new guest arrives. Throughout the 24 hours, the software system all continue to bring in an endless cycle of guests, which will tire the human host.
Above is the link to the project site, but it uses a non-English language; therefore, I relied on using the information from her website (below) and different articles.
Sophia Kim – Project 09 – Sec C
// Sophia S Kim
// Section C 1:30
// sophiaki@andrew.cmu.edu
// Project-09-Portrait
var friendImage;
//function that loads the image
function preload() {
var myImageUrl = "https://i.imgur.com/kEQsen9.jpg";
friendImage = loadImage(myImageUrl);
}
function setup() {
createCanvas(310, 480);
background(0);
imageMode(CENTER);
friendImage.loadPixels();
//loads the image using pixels
frameRate(5000);
}
function draw() {
var positionX = random(width);
var positionY = random(height);
//positions the pixels randomly (x & y values random)
var constrainX = constrain(floor(positionX), 0, width);
var constrainY = constrain(floor(positionY), 0, height);
//constrains the randomly assigned positions within the canvas
var colorGet = friendImage.get(constrainX, constrainY);
//retrieves color from image pixels to a random position
noStroke();
fill(colorGet);
ellipse(positionX - 5, positionY, 2, 2);
//left eye for smiley face
ellipse(positionX + 5, positionY, 2, 2);
//right eye for smiley face
noFill();
stroke(colorGet);
strokeWeight(2);
arc(positionX, positionY + 5, 7.5, 3, 0, radians(180));
//the smile part (arc) of the smiley face
}
function mousePressed() {
textSize(18);
fill(168, 217, 255);
textStyle(BOLD);
noStroke();
text("get me water!", mouseX, mouseY);
}
I chose to do a portrait of Jaclyn that I took last semester at the tennis courts. I knew that I didn’t want to just use simple shapes for the pixels. Instead, I chose to use smiley faces, because Jaclyn is really funny, goofy, and happy. I am really glad I have a friend like her 🙂
Because her tongue is sticked out in the photo, I immediately thought of dehydration and water.
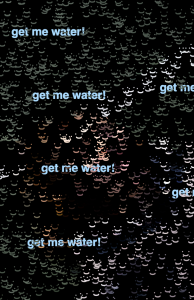
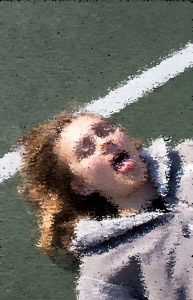
Sophia Kim – Looking Outwards 09 – Sec C
Looking at Julie Choi’s Looking Outwards-03, I was instantly drawn to the vibrant, accent colors within the clear, gel-like material. I like to study projects that fuse science with art/design, because with visual representations, the common person is able to appreciate something that is very complex like the human brain. I really appreciate how these pieces were visualized not on a sheet of paper, but rather printed in layers to create 3D forms. I especially like the 3D printed brain, because using various colors, it represents the different elements of the brain like neurons. Also, I believe it addresses the interaction between the right side of the brain, creativity, and the left side of the brain, logic/thought.
https://www.fastcompany.com/90174994/the-gorgeous-future-of-3d-printing
Sophia Kim Looking Outwards – 08 – Sec C
Stefanie Posavec is a communications designer who moved from Denver, Colorado to London, UK in 2004. Posavec’s works focus on non-traditional representations of data. Posavec received her bachelors degree in graphic design at Colorado State University and master degree in communications design at Central Saint Martins (CSM) College of Art and Design in London. Breaking the strict barriers of data and design, Posavec describes her work to fuse and fall in between the lines of “dataviz” and communications design.
Posavec does not actually code her work, but does prefer to work with data in a hand-crafted way. I admire how she loves to work with data and personalizes the information by visualizing it. I appreciate how she fuses her skills in communications design and her passion for data to create tactical information and interactive design. As a communications designer, I see her style and goals to communicate social/word issues well to the common people. During her EyeO talk in 2018, I liked how she made her presentation flow well, mainly because of the visuals she used to assist her talk. Also, the way she talks during the presentation flowed very well, and I admire how used no filler words.
Among all her works, I really liked the “Air Transformed: Better with Data Society Commission” project, because she was able to communicate the issues of air pollution in London using data, design, and 3D printing. Also, I really like how she was able to approach this issue, which people do not take more seriously, by making her products wearable.
Sophia Kim-Project 07 Sec C
// Sophia S Kim
// Section C 1:30
// sophiaki@andrew.cmu.edu
// Project-07-Composition with Curves
var nPoints = 200;
function setup() {
createCanvas(480, 480);
}
function draw() {
background(0);
//middle green diamond
push();
translate(width/2, height/2);
noFill();
strokeWeight(2);
stroke(35, 255, 0);
drawAstroid();
pop();
//right blue diamond
push();
translate((width/2) + 10, height/2);
noFill()
stroke(35, 0, 255);
drawAstroid();
pop();
//left red diamond
push();
translate((width/2) - 10, height/2);
noFill()
stroke(255, 0, 0);
drawAstroid();
pop();
}
//atroid move based on mouseX and mouseY
// Asteroid - http://mathworld.wolfram.com/Astroid.html
function drawAstroid() {
var x; //xvalue for astroid curve
var y; //yvalue for astroid curve
var a; //for mouse X movement
var b; //constrains mouseY values 0 to 300
a = mouseX;
b = constrain(mouseY, 0, 300);
beginShape();
for(var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
//for degrees based on i
x = 3 * a * (cos(t)) + b * (cos(3*t));
y = 3 * a * (sin(t)) - b * (sin(3*t));
vertex(x,y);
}
endShape();
}
For the “Composition with Curves,” I decided to do an “Astroid” curve, which is a 4-cusped hypocycloid. At first, I was really confused which equation I use for the parametric equations, because the website offered 3 equations for each variable (x, y). I tried all three for each variable to see which one showed the diamond-like shaped curves. Within that process, I got static-like lines, which looked beautiful but was not what I wanted in my final product. After many trials and the right equations, I was able to find the diamond-like curves and create 3 different copies with different colors using push() and pop(). I really liked the way my code turned out, because the changes of the curves reminded me of the Louis Vuitton branding.