//Sarah Yae
//smyae@andrew.cmu.edu
//Section B
//Project 11
var baseface;
//Load Base Face Image
function preload() {
var basefaceload = "https://i.imgur.com/FXyP6ss.gif";
baseface = loadImage(basefaceload);
}
//Set up loaded Base Face
function setup () {
createCanvas(280,400);
background(0);
image(baseface, 0, 0);
text("drag the mouse to make my brows thicker!", 10, 10);
}
function draw() {
var distmousex = map(mouseX, 0, width, 0, 5);
var distmousey = map(mouseY, 0, height, 0, 5);
//Right brow
var browr = makeTurtle((width / 2) + 40 + distmousex, height/3 + distmousey);
browr.setColor(color(210, 180, 140));
browr.setWeight(4);
browr.face(0);
browr.forward(50);
//Left brow
var browl = makeTurtle((width / 2) + distmousex, height/3 + distmousey);
browl.setColor(color(210, 180, 140));
browl.setWeight(4);
browl.face(180);
browl.forward(50);
//Right Eye
var eyer = makeTurtle((width / 2) + 60, (height/3) + 15);
eyer.setColor(50);
eyer.setWeight(1);
for (var i = 0; i < 100; i++) {
eyer.right(360/100);
eyer.forward(0.7);
}
//Left Eye
var eyel = makeTurtle((width / 2) - 20, (height/3) + 15);
eyel.setColor(50);
eyel.setWeight(1);
for (var i = 0; i < 100; i++) {
eyel.right(360/100);
eyel.forward(0.7);
}
//Mouth
stroke("Salmon");
strokeWeight(6);
arc((width / 2) + 20, (height/2) + 15, 80, 40, 0, PI);
//Right Iris
var irisr = makeTurtle((width / 2) + 60, (height/3) + 23);
irisr.setColor(color(30, 17, 0));
irisr.setWeight(7);
for (var i = 0; i < 100; i++) {
irisr.right(360/100);
irisr.forward(0.2);
}
//Left Iris
var irisl = makeTurtle((width / 2) - 20, (height/3) + 23);
irisl.setColor(color(30, 17, 0));
irisl.setWeight(7);
for (var i = 0; i < 100; i++) {
irisl.right(360/100);
irisl.forward(0.2);
}
}
//Turtle Graphics
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
I wanted to originally create a makeup simulation, but as I was working on the project, I found that to be difficult, as turtle graphics work more as a pen, rather than a painting function. I feel like my final product turned out to be way different than my first ideas.
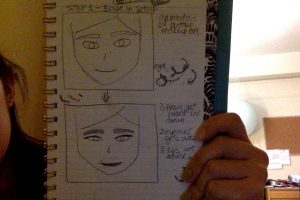
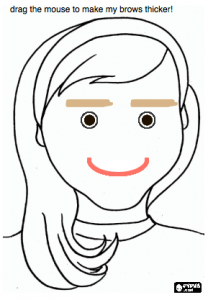