sketch
For this project I show time by having multiple objects to rotate around the Earth, with the sun/moon representing the hour, clouds representing the minute and satellite representing the seconds. As seen in the sketch below the numerical values are represented in a semicircle around the Earth with the left side representing 9am/pm and the right side representing 9pm/am. For the minutes and seconds the left side represents 15/45 and the right side represents 45/15. In addition the sky is darkest at 3am and lightest at 3pm with a gradient between those times. I wanted to have a clock that was abstract but could still be used to read time if you understood how it worked.
At first I thought the rotation around the Earth would be fairly simple and be used simply by translating and rotating, but I realized that wouldn’t work how I wanted it to using the hour(), minutes(), and seconds() commands so I had to figure it out more mathematically. I couldn’t get each object to move from the right to the left once it finished its rotation from the left side to the right side so I had two of each object (only one is visible on the screen at any given time).
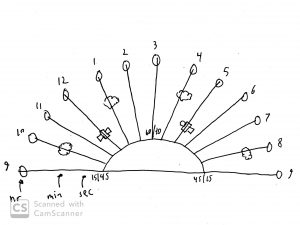
//Taisei Manheim
//Section C
//tmanheim@andrew.cmu.edu
//Assignment-06
var prevSec;
var millisRolloverTime;
function setup() {
createCanvas(480, 480);
millisRolloverTime = 0;
}
function draw() {
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
//Reckon the current millisecond,
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
//sky color lightest at 3pm and darkest 3am with color changing between
var skyColor = 22 * abs(15 - H);
background(135 - skyColor, 206 - skyColor, 235 - skyColor);
//Earth
noStroke();
fill(0,0,255);
ellipse(width / 2, height, width / 4, width / 4);
//North America
fill(127,255,0);
beginShape();
vertex(width / 2 + 6, height - 51.5);
vertex(width / 2 + 5, height - 52);
vertex(width / 2 + 5, height - 52.7);
vertex(width / 2 + 6, height - 54.5);
vertex(width / 2 + 8, height - 56.3);
vertex(width / 2 + 10, height - 57.5);
vertex(width / 2 + 12, height - 58.5);
vertex(width / 2 + 7, height - 59.5);
vertex(width / 2, height - width / 8);
vertex(width / 2 - 7, height - 59.5);
vertex(width / 2 - 12, height - 58.5);
vertex(width / 2 - 17, height - 57.5);
vertex(width / 2 - 22, height - 55.5);
vertex(width / 2 - 27, height - 53);
vertex(width / 2 - 32, height - 50.5);
vertex(width / 2 - 37, height - 47);
vertex(width / 2 - 42, height - 42.5);
vertex(width / 2 - 42 + 8, height - 42 + 4);
vertex(width / 2 - 42 + 12, height - 42 + 9);
vertex(width / 2 - 42 + 25, height - 42 + 35);
vertex(width / 2 - 42 + 27, height - 42 + 38);
vertex(width / 2 - 42 + 30, height - 42 + 40);
vertex(width / 2 - 42 + 35, height);
vertex(width / 2 + 5, height);
vertex(width / 2 + 2, height - 2);
vertex(width / 2 + 1, height - 3);
vertex(width / 2 - 0.5, height - 5);
vertex(width / 2 - 2, height - 8);
vertex(width / 2 - 4, height - 12);
vertex(width / 2 - 4, height - 14);
vertex(width / 2 - 3, height - 15);
vertex(width / 2 - 1, height - 16.5);
vertex(width / 2 + 1, height - 17.3);
vertex(width / 2 + 3, height - 18);
vertex(width / 2 + 5, height - 18.5);
vertex(width / 2 + 7, height - 18.5);
vertex(width / 2 + 9, height - 18.2);
vertex(width / 2 + 11, height - 17.7);
vertex(width / 2 + 13, height - 17.2);
vertex(width / 2 + 15, height - 16.5);
vertex(width / 2 + 17, height - 15.7);
vertex(width / 2 + 19, height - 15.4);
vertex(width / 2 + 21, height - 15.4);
vertex(width / 2 + 21.5, height - 15.7);
vertex(width / 2 + 21.7, height - 16.3);
vertex(width / 2 + 21.7, height - 17);
vertex(width / 2 + 21.4, height - 17.5);
vertex(width / 2 + 21, height - 18.5);
vertex(width / 2 + 19.5, height - 19.8);
vertex(width / 2 + 18.5, height - 20.5);
vertex(width / 2 + 18, height - 21.5);
vertex(width / 2 + 18, height - 23);
vertex(width / 2 + 19.5, height - 28);
vertex(width / 2 + 22.5, height - 33);
vertex(width / 2 + 25.5, height - 36);
vertex(width / 2 + 28.5, height - 40);
vertex(width / 2 + 29.5, height - 42);
vertex(width / 2 + 29.5, height - 44);
vertex(width / 2 + 28.5, height - 45);
vertex(width / 2 + 27, height - 46);
endShape();
//text
fill(255);
textSize(24);
text(H + ":" + M + ":" + S, width / 2 - 30, 100);
//remapping hour, second, minute values to use with cos and sin for circular motion
var sec = map(second(), 0, 60, 0, TWO_PI) - HALF_PI;
var min = map(minute() + map(second(), 0, 60, 0, 1), 0, 60, 0, TWO_PI) - HALF_PI;
var hr = map(hour() + map(minute(), 0, 60, 0, 1), 0, 24, 0, TWO_PI * 2) - HALF_PI;
//seconds
//sattelite 1
//wing 1
fill(150);
quad(width / 2 + cos(sec) * width / 2 * 0.75, height + sin(sec) * width / 2 * 0.75,
width / 2 + cos(sec) * width / 2 * 0.75, (height + sin(sec) * width / 2 * 0.75) - 30,
(width / 2 + cos(sec) * width / 2 * 0.75) - 10, (height + sin(sec) * width / 2 * 0.75) - 30,
(width / 2 + cos(sec) * width / 2 * 0.75) - 10, height + sin(sec) * width / 2 * 0.75);
//wing 2
quad(width / 2 + cos(sec) * width / 2 * 0.75, height + sin(sec) * width / 2 * 0.75,
width / 2 + cos(sec) * width / 2 * 0.75, (height + sin(sec) * width / 2 * 0.75) + 20,
(width / 2 + cos(sec) * width / 2 * 0.75) - 10, (height + sin(sec) * width / 2 * 0.75) + 20,
(width / 2 + cos(sec) * width / 2 * 0.75) - 10, height + sin(sec) * width / 2 * 0.75);
fill(255);
//body
quad(width / 2 + cos(sec) * width / 2 * 0.75, height + sin(sec) * width / 2 * 0.75,
width / 2 + cos(sec) * width / 2 * 0.75, (height + sin(sec) * width / 2 * 0.75) - 10,
(width / 2 + cos(sec) * width / 2 * 0.75) - 20, (height + sin(sec) * width / 2 * 0.75) - 10,
(width / 2 + cos(sec) * width / 2 * 0.75) - 20, height + sin(sec) * width / 2 * 0.75);
//sattelite 2
//wing 1
fill(150);
quad(width / 2 - cos(sec) * width / 2 * 0.75, height - sin(sec) * width / 2 * 0.75,
width / 2 - cos(sec) * width / 2 * 0.75, (height - sin(sec) * width / 2 * 0.75) - 30,
(width / 2 - cos(sec) * width / 2 * 0.75) - 10, (height - sin(sec) * width / 2 * 0.75) - 30,
(width / 2 - cos(sec) * width / 2 * 0.75) - 10, height - sin(sec) * width / 2 * 0.75);
//wing 2
quad(width / 2 - cos(sec) * width / 2 * 0.75, height - sin(sec) * width / 2 * 0.75,
width / 2 - cos(sec) * width / 2 * 0.75, (height - sin(sec) * width / 2 * 0.75) + 20,
(width / 2 - cos(sec) * width / 2 * 0.75) - 10, (height - sin(sec) * width / 2 * 0.75) + 20,
(width / 2 - cos(sec) * width / 2 * 0.75) - 10, height - sin(sec) * width / 2 * 0.75);
fill(255);
//body
quad(width / 2 - cos(sec) * width / 2 * 0.75, height - sin(sec) * width / 2 * 0.75,
width / 2 - cos(sec) * width / 2 * 0.75, (height - sin(sec) * width / 2 * 0.75) - 10,
(width / 2 - cos(sec) * width / 2 * 0.75) - 20, (height - sin(sec) * width / 2 * 0.75) - 10,
(width / 2 - cos(sec) * width / 2 * 0.75) - 20, height - sin(sec) * width / 2 * 0.75);
//minutes
//cloud1
fill(255);
ellipse(width / 2 + cos(min) * width / 2 * 0.5, height + sin(min) * width / 2 * 0.5, 15, 15);
ellipse((width / 2 + cos(min) * width / 2 * 0.5) + 10, height + sin(min) * width / 2 * 0.5, 15, 15);
ellipse((width / 2 + cos(min) * width / 2 * 0.5) - 10, height + sin(min) * width / 2 * 0.5, 15, 15);
ellipse((width / 2 + cos(min) * width / 2 * 0.5) + 5, (height + sin(min) * width / 2 * 0.5) + 7, 15, 15);
ellipse((width / 2 + cos(min) * width / 2 * 0.5) - 5, (height + sin(min) * width / 2 * 0.5) + 7, 15, 15);
ellipse((width / 2 + cos(min) * width / 2 * 0.5) + 5, (height + sin(min) * width / 2 * 0.5) - 7, 15, 15);
ellipse((width / 2 + cos(min) * width / 2 * 0.5) - 5, (height + sin(min) * width / 2 * 0.5) - 7, 15, 15);
///cloud2
ellipse(width / 2 - cos(min) * width / 2 * 0.5, height - sin(min) * width / 2 * 0.5, 15, 15);
ellipse((width / 2 - cos(min) * width / 2 * 0.5) + 10, height - sin(min) * width / 2 * 0.5, 15, 15);
ellipse((width / 2 - cos(min) * width / 2 * 0.5) - 10, height - sin(min) * width / 2 * 0.5, 15, 15);
ellipse((width / 2 - cos(min) * width / 2 * 0.5) + 5, (height - sin(min) * width / 2 * 0.5) + 7, 15, 15);
ellipse((width / 2 - cos(min) * width / 2 * 0.5) - 5, (height - sin(min) * width / 2 * 0.5) + 7, 15, 15);
ellipse((width / 2 - cos(min) * width / 2 * 0.5) + 5, (height - sin(min) * width / 2 * 0.5) - 7, 15, 15);
ellipse((width / 2 - cos(min) * width / 2 * 0.5) - 5, (height - sin(min) * width / 2 * 0.5) - 7, 15, 15);
//hours
//moon & sun 1
fill(255,255,0);
ellipse(width / 2 + cos(hr) * width / 2, height + sin(hr) * width / 2, 50, 50);
//moon & sun 2
fill(255,255,0);
ellipse(width / 2 - cos(hr) * width / 2, height - sin(hr) * width / 2, 50, 50);
}