//Shannon Ha
//Section D
//sha2@andrew.cmu.edu
//Project 09 - Variable Face
//pre load my underlying image
var underlyingImage;
var frameSpeed = 15;
function preload() {
var myImageURL = "https://i.imgur.com/AAXi1mS.jpg";
underlyingImage = loadImage(myImageURL);
}
function setup() {
createCanvas(344, 480);
background(0);
//load the pixels of the image so they can be referenced later
underlyingImage.loadPixels();
framespeed = map(mouseX, 10, width, 2, 150);
frameRate(frameSpeed);
}
function draw() {
//randomly select a pixel on the canvas
var randomX = random(width); //x-coordinate
var randomY = random(height); //y-coordinate
var ix = constrain(floor(randomX), 0, width-1);
var iy = constrain(floor(randomY), 0, height-1);
//loads the color from the base image so the rectangles coordinate with the colors of the base image
var theColorAtLocationXY = underlyingImage.get(ix, iy);
//creates rectangles at different sizes.
noFill();
stroke(theColorAtLocationXY);
strokeWeight(random(1,3));
rect(randomX, randomY, random(5,20), random(5,20));
//creates circles according to position of mouse.
var theColorAtTheMouse = underlyingImage.get(mouseX, mouseY);
strokeWeight(1);
stroke(theColorAtTheMouse);
ellipse(pmouseX, pmouseY, 15, 15);
}
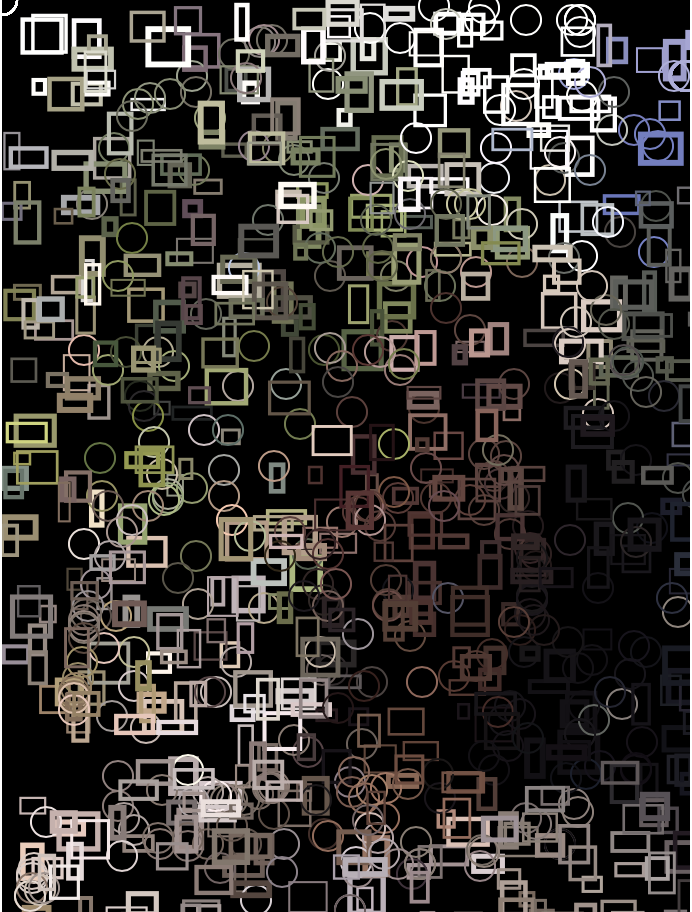
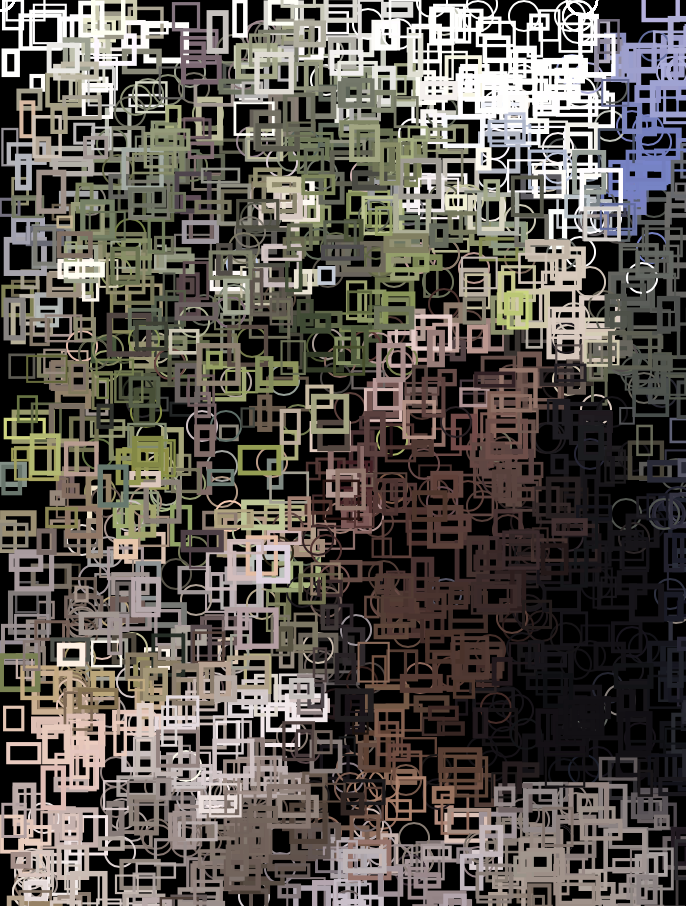
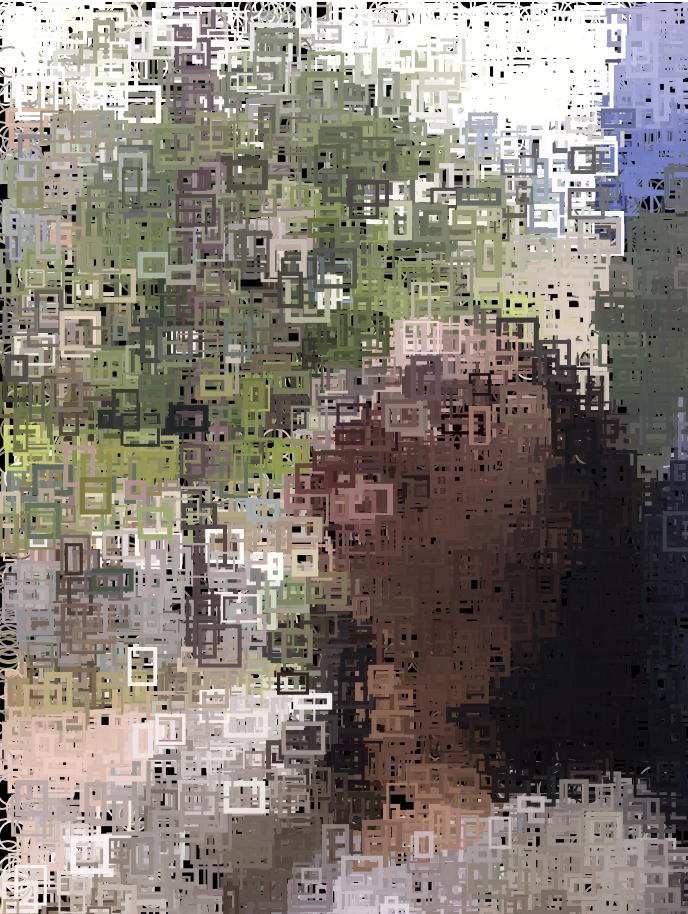
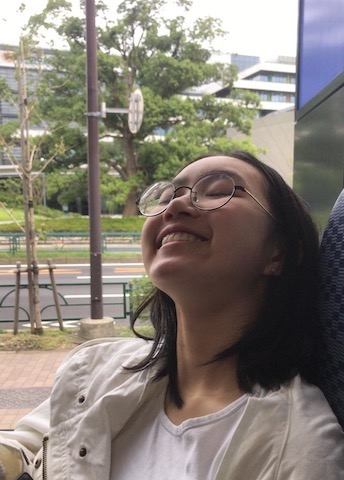
For this project, I chose a portrait of my sister to recreate and explored how to randomize the dimensions of of a rectangle to create variable portrait. I tested different shapes both filled and unfilled to see which has a better effect for the end result and I realized that the filled shapes some times makes the face look too blurred out so I used stroke instead to retain some texture in the image and to distinguish facial features better. It was a fun project to do because it was interesting to see how different shapes produce different textures and effects on the image.