var x1;
var x2;
var y1;
var y2;
var x1ss;
var y1ss;
var x3;
var x4;
var y3;
var y4;
var y2ss;
var x5;
var y5;
function setup() {
createCanvas(300, 400);
background (0);
x1 = 0;
y1 = 0;
x1ss = 10;
y1ss = 50;
x2 = x1 + x1ss;
y2 = y1 + y1ss;
x3 = 0;
y3 = 400;
x1ss = 10;
y1ss = 50;
x4 = x1 + x1ss;
y4 = y1 + y1ss;
}
function draw() {
noFill();
stroke(255);
strokeWeight(1);
line(x1, y1, x2, y2);
x1ss = x1ss * 1.35;
y1ss = y1ss * 1.3;
x2 = x1 + x1ss;
y2 = y1 + y1ss;
y2ss = -y1ss;
stroke("red");
strokeWeight(1);
line(x3, y3, x4, y4);
x4 = x3 + x1ss;
y4 = y3 + y2ss;
stroke("pink");
strokeWeight(1);
line(x2 - x1, y2 - y1, y2- y1, x2 - x1);
stroke("grey")
line(300 - x2, 400 - x2, x4 / 3, y4 / 3);
}
Category: SectionE
Jina Lee – Project 04
For this project, I played around with curves. I wanted to give a disco/chaotic vibe. When move your mouse around the canvas, the lines move making it seem more busy. I enjoyed this project because there were so many ways I could make my strings move. In the future, I want to add more curves to make it seem like a camera lens opening and closing with the string curve. This assignment helped me better understand how loops work and how I can manipulate curves with mouseX and mouseY.
//Jina Lee
//Section E
//jinal2@andrew.cmu.edu
//Project-04
function setup() {
createCanvas(300, 400);
}
function draw() {
//background is random
red = random(255);
green = random(255);
blue = random(255);
background(red, green, blue, 40);
//lines that just go up and down
//as the mouse goes right the lines appear
for (var i = 0; i <= mouseX; i += 50) {
stroke(255);
strokeWeight(1);
line(i, 0, i, height + i);
}
//top left corner and bottom right
//it curves down
for (var a = 0; a < mouseX; a += 20) {
stroke(255);
strokeWeight(1);
line(a, 0, width, a);
}
//top right and left bottom
for (var b = 0; b < mouseY; b += 5) {
stroke(255);
strokeWeight(1);
line(width + b, b, width - b, height);
}
//left top and bottom right
for (var c = 0; c < mouseX; c += 20) {
stroke(255);
strokeWeight(1);
line(width - c, height, 0, height - c);
}
//left bottom and top right
for (var d = 0; d < mouseY; d += 5) {
stroke(255);
strokeWeight(1);
line(0, height - d, d, 0);
}
}
Charmaine Qiu – Project 04 – String Art
/* Charmaine Qiu
charmaiq@andrew.cmu.edu
Section E
Project 04 String Art*/
var spacing = 10;
var gcolor = 34;
var bcolor = 140;
function setup() {
createCanvas(400, 300);
}
function draw() {
//set background color and initial stroke color of center piece
background(0, 13, 255);
stroke(255, gcolor, bcolor);
//the center piece that follows the mouse
for(var i = 0; i < 30; i++){
//changes color when moving between left and right side of canvas
if(mouseX > width / 3){
stroke(150, gcolor, bcolor);
}
if(mouseX > 2*width / 3){
stroke(50, gcolor, bcolor);
}
//the center piece drawn with a spacing variable that sets distance between two lines
line(mouseX, i * spacing, width, height / 2);
line(0, height / 2, mouseX, i * spacing);
}
//line art that surrounds the opposite corners of canvas
for(var i = 0; i < width; i += 10){
//yellow lines
stroke(218, 245, 66);
line(i, 0, width, i / 2);
line(0, i, i /2, width);
//orange lines
stroke(255, 162, 0);
line(width, height - i, width - i, 0);
line(0, i / 2, i, height);
}
}
It was challenging to figure out how to efficiently create the lines on canvas. I tried to incorporate an interactive aspect towards my project, and it was fun to explore with. I now have a better understanding of how digital line art is created and the thought process behind it.
YouieCho-Project-04-String-Art
/*Youie Cho
Section E
minyounc@andrew.cmu.edu
Project-04-String Art*/
var a = 7
var b = 1
function setup() {
createCanvas(400, 300);
angleMode();
}
function draw() {
background(255, 179, 0);
//pink sequence
for (var i = 1; i < 1000; i++) {
stroke(255, 69, 128);
line(mouseX, i * a* mouseY / 100, i * a, height / 4);
}
//black sequence and rotation
for (var i = 1; i <= 1000; i++) {
stroke(0);
rotate(90);
line(mouseX, i * mouseY / 50, i * b, height / 2);
}
//yellow sequence and rotation
for (var i = 1; i <= 1000; i++) {
stroke(255, 215, 69);
rotate(180);
line(mouseX, i * mouseY / 25, i * a, height / 1.333333);
}
//purple sequence and rotation
for (var i = 1; i <= 1000; i++) {
stroke(174, 82, 255);
rotate(270);
line(mouseX, i * mouseY / 10, i * b, height);
}
}
I tried to play around with changing ratios to generate different sequences on different parts of the canvas, and rotated them to create complexity. I tried complex lines because interesting shapes and patterns can be generated through the movement of the mouse. It was challenging to understand the mechanism of loop, but I enjoyed how dynamic it could be.
Charmaine Qiu-LookingOutwards 04
The Prelude in ACGT is a project created by Pierry Jaquillard where he used his own DNA to generate music by utilizing the chromosomes 1 to 22 and XY. Believing that DNA is the core structure of nature, Pierry wanted to experiment with his own personal code.
The project was created through JavaScript, and he generated midi signals that was passed into Ableton Live, a music sequencer, to play the sounds. The project was displayed on 4 ipads and a phone, where the phone is used to change tempo and arrangement. I found this project really interesting since it celebrated the nature of human beings and creating art through biology could become a direction of art in the future.
William Su – LookingOutwards – 04
Musical Fire Table: https://www.youtube.com/watch?v=2awbKQ2DLRE
Description from the video: The pressure variations due to the sound waves affect the flow rate of flammable gas from the holes in the Pyro Board and therefore affect the height and colour of flames. This is interesting for visualizing standing wave patterns and simply awesome to watch when put to music.
While the people that made this didn’t really intend or utilize this as “art”, It is a performance that is really interesting to look at despite how simple it is to get the fire table to work. While i’m not sure if there is any computational stuff behind it (most of it involves simply physics and running standing waves through the gas pipes), I can see this being scaled up, like in a stadium or stage performance with the pyrotechnic stuff that is commonly found there.
Margot Gersing – Project 04 – String Art
// Margot Gersing - Project 04 String Art - Section E - mgersing@andrew.cmu.edu
function setup() {
createCanvas(300,400);
}
function draw(){
background(0);
// tight lines less wide
for (i = 0; i < 200; i += 5) {
stroke(i, 208, 247); //blue and purple
line(100, 450 + i, i, 10 - i); //'curve 1'
line(100, 450 + i, mouseX * 10, 10 - i); //'curve 1' with interaction
}
// tight lines
for (i = 0; i < 600; i += 5) {
stroke(i, 235, 225, i); //green to peach
line(0, i - 100, i, 400); //'curve 2'
line(0, i - 100, mouseX, mouseY); //'curve 2' with interaction
}
// wider lines
for (i = 0; i < 600; i += 15) {
stroke(230, 202, i); //purple to ochre
line(0, 100 - i, 400, 200 + i); //'curve 3'
line(0, mouseY / 5, 400, 200 + i); //'curve 3' with interaction
// curve 4
stroke(252, 186, i, i); //yellow to magenta
line(400, 200 - i, i - 400, i); //'curve 4'
line(400, mouseY - i, i - 400, i); //'curve 4' with interaction
}
}
I enjoyed making this project. I decided that I wanted to incorporate my ‘i’ variable into more than just the creation of the lines. I experimented with replacing rgb values with ‘i’ and it created really interesting color shifts. I also decided to make a copy of each of the curves I made and make one of them interactive with mouseX and mouseY.
William Su – Project 04 – String Art
I just made a rainbowy web of strings that changes colors depending on mouseX and mouseY. Credit to the person that utilized rotate and translate to move stuff around, it made things a lot simpler to think about.
// William Su
// Section E
// wsu1@andrew.cmu.edu
// Project 04
function setup () {
createCanvas(400, 300);
background(0);
strokeWeight(0.5);
}
function draw () {
for (var x = 0; x <= 50; x += 2) {
var1 = x
var2 = x * 10
b = constrain(var2, 0, (3 * width) / 5);
//bottomleft
stroke(mouseX, 0, 50);
line(0, var2 + height / 2, var2, height);
//topleft
push();
translate(300, 0);
rotate(radians(90));
stroke(mouseY, 0, mouseX);
line(0, var2 + height / 2, var2, height);
pop();
//topright
push();
translate(400, 300);
rotate(radians(180));
stroke(mouseY, mouseX, 50);
line(0, var2 + height / 2, var2, height);
pop();
//bottom right
push();
translate(100,300);
rotate(radians(270));
stroke(mouseX, mouseY, 50);
line(0, var2 + height / 2, var2, height);
pop();
//2ndCurveTopRight
stroke(50,mouseX, mouseY);
line(width * 1.2, var2, var2 /2, 0);
//2ndCurveBottomLeft
push();
translate(400, 300);
rotate(radians(180));
stroke(50,mouseY, mouseX);
line(width * 1.2, var2, var2 /2, 0);
pop();
};
}
YouieCho-LookingOutwards-04
Weather Thingy is a sounds controller that takes real time climate-related events data to control and modify the stings of musical instruments. It is made up of a weather station on a tripod microphone, and a custom built controller that is connected to the weather station. I think this is very inspiring for me because I had never thought of connecting weather data with sound production. This has sensors that observe the climate and assign various parameters received to audio effects. The creator’s artistic sensibilities, in my opinion, especially come in the very clear and minimal that makes use of clear color-coded buttons, as well as customized parts of the project as a whole. It is also notable that there are many real-time interactions going on.
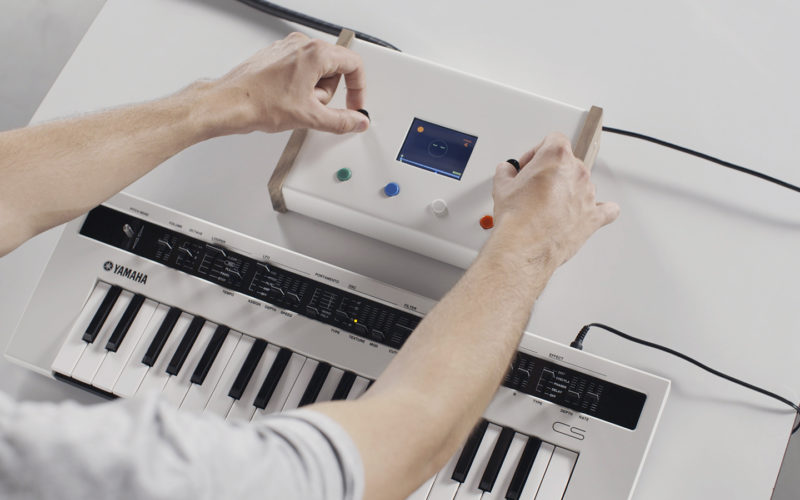
Gretchen Kupferschmid-String Art-Project 04
with this project, I wanted to play around with color and the way layering string art and color can create an abstract piece of art.
function setup() {
createCanvas(400,300);
}
function draw (){
background(235, 192, 52);
for (i= 0; i<200; i+=5){
stroke(244, 192, 252);
line(i-100, 0, 400, i)
stroke(244, 192, 252);
line(0, i, i, 200)
stroke(244, 192, 252);
line(i, 200, 0, 600-i)
line(i, 250, 0, 300-i)
}
for(i=0; i<400; i+=10){
stroke(255);
line(400, 300-i , i, 300);
line(400, 200-i , i, 300);
line(400, 100-i , i, 300);
line(400, 50-i , i, 300);
stroke(209, 61, 90);
line(100-i, 150, mouseX, mouseX);
line(300-i,0, i-290, 150);
}
}