function setup() {
createCanvas(600, 300);
background(200, 150, 100);
noStroke(0);
var one = 50;
var two = 2 * one;
var three = 3 * one;
var four = 4 * one;
fill(250);
ellipse(200 - (one / 2), 50 + (one / 2), one, one);
fill(0);
rect(200, 50 + one, one, three);
fill(100);
rect( 200, 50, three, one);
fill(250);
ellipse(200 + two, 50 + (2.5 * one), two, three);
fill(200);
rect( 200 + three, 50, two, two);
fill(50);
rect( 200 + three, 50 + two, two, two);
fill(250);
triangle( 200 + four + one, 50, 200 + four + one, 50 + four, 200 + two + four, 50 + two);
}
Category: SectionE
Project – 02 – Variable Face
function setup () {
createCanvas(600, 600);
headW = random(250, 450);
headH = random(300, 450);
noseH = random(60, 150);
noseW = random(50, 110);
eyeLX = 300 - (headW / 6);
eyeRX = 300 + (headW / 6);
eyeY = 300 - (headH / 5);
cheekLX = 300 - (headW / 4);
cheekRX = 300 + (headW / 4);
cheekY = 300 + (headW / 5);
r = random(40, 255);
g = random(40, 255);
b = random(40, 255);
}
function draw() {
background(255 - r, 255 - g, 255 - b);
fill(r, g, b);
noStroke(0);
ellipse(300, 300, headW, headH);
ellipse(cheekLX, cheekY, headW / 2, headH / 2);
ellipse(cheekRX, cheekY, headW / 2, headH / 2);
fill(240);
ellipse(eyeLX, eyeY, headW / 5, headH / 5);
ellipse(eyeRX, eyeY, headW / 5, headH / 5);
fill(230 - r, 230 - b, 230 - g);
ellipse(eyeLX + (headW / 40), eyeY + (headH / 40), 30, 30);
ellipse(eyeRX - (headW / 40), eyeY + (headH / 40), 30, 30);
fill(r - 100, g - 100, b - 100);
bezier(cheekLX, cheekY, cheekLX + headW / 4, cheekY + headH / 4, cheekRX - headW / 4, cheekY + headH / 4, cheekRX, cheekY);
fill(r - 30, g - 30, b - 30);
ellipse(300, 300, noseW, noseH);
ellipse(300 - (noseW / 2), 300 + (noseH / 6), noseH / 3, noseH / 3);
ellipse(300 + (noseW / 2), 300 + (noseH / 6), noseH / 3, noseH / 3);
}
function mousePressed() {
headW = random(250, 450);
headH = random(300, 450);
noseH = random(60, 150);
noseW = random(50, 110);
r = random(40, 255);
g = random(40, 255);
b = random(40, 255);
eyeLX = 300 - (headW / 6);
eyeRX = 300 + (headW / 6);
eyeY = 300 - (headH / 5);
cheekLX = 300 - (headW / 4);
cheekRX = 300 + (headW / 4);
cheekY = 300 + (headW / 5);
}
Looking Outwards – 02
One piece of generative art that has always amazed me is the game by Mossmouth studios titled Spelunky. Initially a browser game, it was defined by its dungeon structure program, which built a large 4 by 4 for room for each level, building a cohesive and constantly different game as you play through its 16+ levels. The game itself is an excellent work of programming and code design, but its most impressive feature is how the game knows how it creates a path within its trap filled, maze like dungeon algorithm to create a fun, non confusing experience for the player that allows them to explore and move forward in their own way. The code is variable enough to create a new experience each time you play, but not too confusing where the player feels lost. The algorithm plays such a huge role in the experience, but it is overshadowed by the unique visuals and immersive music.
https://store.steampowered.com/app/239350/Spelunky/
William Su – Project 02 – Variable Face
// William Su
// Section E
// wsu1@andrew.cmu.edu
// Project-02
//data-width="640" data-height="480"
var eyeSize = 20;
var faceWidth = 100;
var faceHeight = 150;
var diam = 170;
var smileDiam = 0.2;
var endAng = 0.5;
var startAng = 0.1;
var fillnum = 100;
var weightnum = 1;
function setup() {
createCanvas(640, 480);
}
function draw() {
stroke(0);
strokeWeight(weightnum);
background(180);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
arc(width / 2, height / 2, smileDiam * diam, smileDiam * diam, startAng * PI, endAng * PI);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'faceWidth' gets a random value between 75 and 150.
faceWidth = random(75, 150);
faceHeight = random(100, 200);
eyeSize = random(10, 30);
startAng = random(0.05, 0.3);
endAng = random(0.4, 0.8);
smileDiam = random(0.2, .4);
fillnum1 = random (50, 200);
fillnum2 = random (100, 250);
fillnum3 = random (10, 150);
fill(fillnum1, fillnum2, fillnum3);
weightnum = random(1,5);
}
In this project, I utilized the template given and added 3 extra areas of variability. The first one is different smiles, the second is the color of the face, the third is the stroke width.
YouieCho-Project-02-Variable-Face
/*Youie Cho
Section E
minyounc@andrew.cmu.edu
Project-02-Variable-Face*/
var eyeSize = 15;
var faceWidth = 200;
var faceHeight = 150;
var cloudW = 40;
var cloudH = 60;
var cloudWs = 30;
var cloudHs = 40;
//eye color
var r = 100;
var g = 100;
var b = 100;
//background color
var rB = 73;
var gB = 129;
var bB = 242;
function setup() {
createCanvas(640, 480);
}
function draw() {
background(250, 140, 155);
fill(rB, gB, bB);
rect(30, 25, 570, 380);
//body
noStroke();
fill(224, 194, 121);
beginShape();
curveVertex(100, 600);
curveVertex(180, 600);
curveVertex(220, 360);
curveVertex(400, 330);
curveVertex(480, 600);
curveVertex(400, 600);
endShape();
//ears
fill(224, 194, 121);
ellipse(260, 200, faceWidth / 4 - 10, faceHeight / 2);
ellipse(380, 200, faceWidth / 4 + 10, faceHeight / 2 + 10);
//face
fill(242, 216, 155);
ellipse(width / 2, 270, faceWidth, faceHeight);
var eyeLX = width / 2 - faceWidth * 0.3;
var eyeRX = width / 2 + faceWidth * 0.3;
//eye
fill(r, g, b);
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
noFill();
//nose
fill(171, 129, 26);
triangle(285, 260, 315, 260, 300, 283);
//mouth
noFill();
strokeWeight(3);
stroke(84, 50, 9);
bezier(280, 283, 285, 287, 290, 287, 300, 283);
bezier(300, 283, 310, 287, 315, 287, 320, 283);
//cloud
fill(225)
noStroke();
ellipse(160, 100, cloudWs, cloudHs);
ellipse(180, 100, cloudW, cloudH);
ellipse(200, 100, cloudW, cloudH);
ellipse(220, 100, cloudW, cloudH);
ellipse(240, 100, cloudWs, cloudHs);
//cheeks
fill(255, 189, 158);
ellipse(235, 273, faceWidth / 5, faceHeight / 6);
ellipse(380, 273, faceWidth / 4, faceHeight / 6);
}
function mousePressed() {
faceWidth = random(180, 220);
faceHeight = random(150, 200);
eyeSize = random(10, 20);
cloudW = random(40, 50);
cloudH = random(50, 70);
cloudWs = random(20, 40);
cloudHs = random(30, 40);
r = random(26, 99);
g = random(34, 102);
b = random(4, 201);
rB = random(73, 209);
gB = random(129, 224);
bB = random(242, 255);
}
It was fun to try changing colors and shapes, and I think it was especially meaningful to learn to make these changes within various relationships. It also made me make a connection to the generative art I looked at Looking Outwards.
Ilona Altman – Looking Outwards – 02
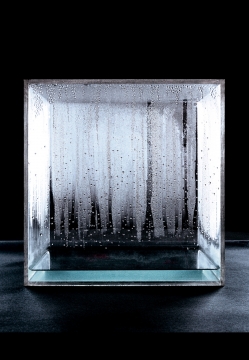
I was interested in the definition of a generative piece not necessarily having to be technological. Though technology and coding allows for systems to change depending on their environment, so can some physical structures. This piece is called Condensation Cube, and it was made by Hans Haacke in 1963. It is composed of sheets of plexiglass combined to make a square container, as well as some water and air. This piece is generative as within the system of this box, which can exist in a space with variable temperature, the pattern of the condensation changes. The artwork depends entirely on the place it exists in.
I find this piece interesting and beautiful, as its brings a natural process into focus. It makes me think about how all art in a way changes depending on the context in which it is placed.
Gretchen Kupferschmid – Project 02 – Variable Face
var eyeSize = 7.588;
var mouthWidth = 20.166;
var mouthHeight = 17.656;
var flowerSize = 226.69;
var flowerColor = 208;
var blushHeight = 5.565;
var cactusHeight = 217;
var cactusArm = 75.357;
var cactusColor = 119;
function setup() {
createCanvas(480, 640);
}
function draw (){
background(199, 241, 242);
//ground
fill(193, 173, 139);
noStroke();
rect(0, 359.79, 480, 280.209);
//ground shadow
fill(142, 123, 94);
noStroke();
ellipse(142.96, 426.9, 217.735, 29.888);
//cactus shadow
fill(93, 135, 56);
noStroke();
ellipse (209.98, 426.9, 80.461, 37.36);
beginShape();
curveVertex (209.16, 437.58);
curveVertex (170, 430.95);
curveVertex (167.32, 397.88);
curveVertex (164.59, 363.31);
curveVertex (156, 248.85);
curveVertex (173, 148.38);
curveVertex (224, 131.63);
curveVertex (262, 204.89);
curveVertex (262, 303.27);
curveVertex (251, 392.23);
curveVertex (248, 430.95);
curveVertex (209.16, 437.58);
endShape();
beginShape();
curveVertex (189, 359.09);
curveVertex (171, 358.09);
curveVertex (145, 352.09);
curveVertex (113, 337.09);
curveVertex (88, 272.09);
curveVertex (112, 247.09);
curveVertex (137, 268.09);
curveVertex (189, 317.09);
curveVertex (189, 359.09);
endShape();
beginShape();
curveVertex (233, 294.73);
curveVertex (231, 260.73);
curveVertex (255.96, 226.97);
curveVertex (278, 204.73);
curveVertex (308, 192.73);
curveVertex (334, 219.73);
curveVertex (312, 272.73);
curveVertex (275, 297.73);
curveVertex (252.77, 301.71);
curveVertex (233, 294.73);
endShape();
//cactus main body
fill(cactusColor, 173, 78);
noStroke();
beginShape();
curveVertex (217.38, 430.71);
curveVertex (184.74, 422.24);
curveVertex (179.02, 340.31);
curveVertex (172.99, 248.24);
curveVertex (187.26, 152.24);
curveVertex (230.06, 136.24);
curveVertex (261.96, 206.24);
curveVertex (261.96, 300.24);
curveVertex (252.72, 385.24);
curveVertex (250.21, 423.24);
curveVertex (217.38, 430.71);
endShape();
beginShape();
curveVertex (189, 350);
curveVertex (171, 349);
curveVertex (152.15, 345.15);
curveVertex (145, 343);
curveVertex (113, 328);
curveVertex (93.55, 304.65);
curveVertex (88, 263);
curveVertex (112, 238);
curveVertex (137, 259);
curveVertex (182.98, 298.1);
curveVertex (189, 308);
curveVertex (197.95, 333.5);
curveVertex (189, 350);
endShape()
beginShape();
curveVertex (239, 274);
curveVertex (237, 240);
curveVertex (261.96, 206.24);
curveVertex (284, 184);
curveVertex (314, 172);
curveVertex (340, 199);
curveVertex (318, 252);
curveVertex (281, 277);
curveVertex (260.25, 280.94);
curveVertex (239, 274);
endShape();
ellipse (114.46, 276.75, 57, 79.492);
ellipse (307.36, 209.41, 67.402, cactusArm);
ellipse (220.44, cactusHeight, 98.039, 190);
//flower
fill(214, 171, flowerColor);
noStroke();
beginShape();
curveVertex (106.31, 246.7);
curveVertex (96.93, 241.91);
curveVertex (94.92, 230.34);
curveVertex (98.63, flowerSize);
curveVertex (100.55, 230.61);
curveVertex (106.1, 236.04);
curveVertex (106.1, 233.22);
curveVertex (107.38, flowerSize);
curveVertex (113.13, flowerSize);
curveVertex (117.82, 234.52);
curveVertex (120.38, 229.52);
curveVertex (126.78, flowerSize);
curveVertex (127.84, 233.65);
curveVertex (121.66, 243.87);
curveVertex (115.37, 246.79);
curveVertex (106.31, 246.7);
endShape();
//eyes black part
fill(0);
noStroke();
ellipse (206.25, 209.41, eyeSize, eyeSize);
ellipse (229.96, 209.41, eyeSize, eyeSize);
//eyes white part
fill(255);
noStroke();
ellipse (207.02, 208.28, 3.026, 3.026);
ellipse (230.96, 208.28, 3.026, 3.026);
//blush cheeks
fill(193, 118, 148);
noStroke();
ellipse (198.2, 222.36, 7.791, blushHeight);
ellipse (236.59, 222.36, 7.791, blushHeight);
//mouth
fill(0);
noStroke();
arc (219.36, 227.43, mouthWidth, mouthHeight, 0, PI );
}
function mousePressed () {
eyeSize = random(6.5, 12);
mouthWidth = random(11, 44);
mouthHeight = random(10, 25);
flowerSize = random(220, 230);
flowerColor = random(80, 255);
blushHeight = random(4, 9);
cactusHeight = random(190, 225);
cactusArm = random(65, 120);
cactusColor = random(0, 150);
}
Gretchen Kupferschmid – Looking Outward – 02
https://obvious-art.com/edmond-de-belamy.html
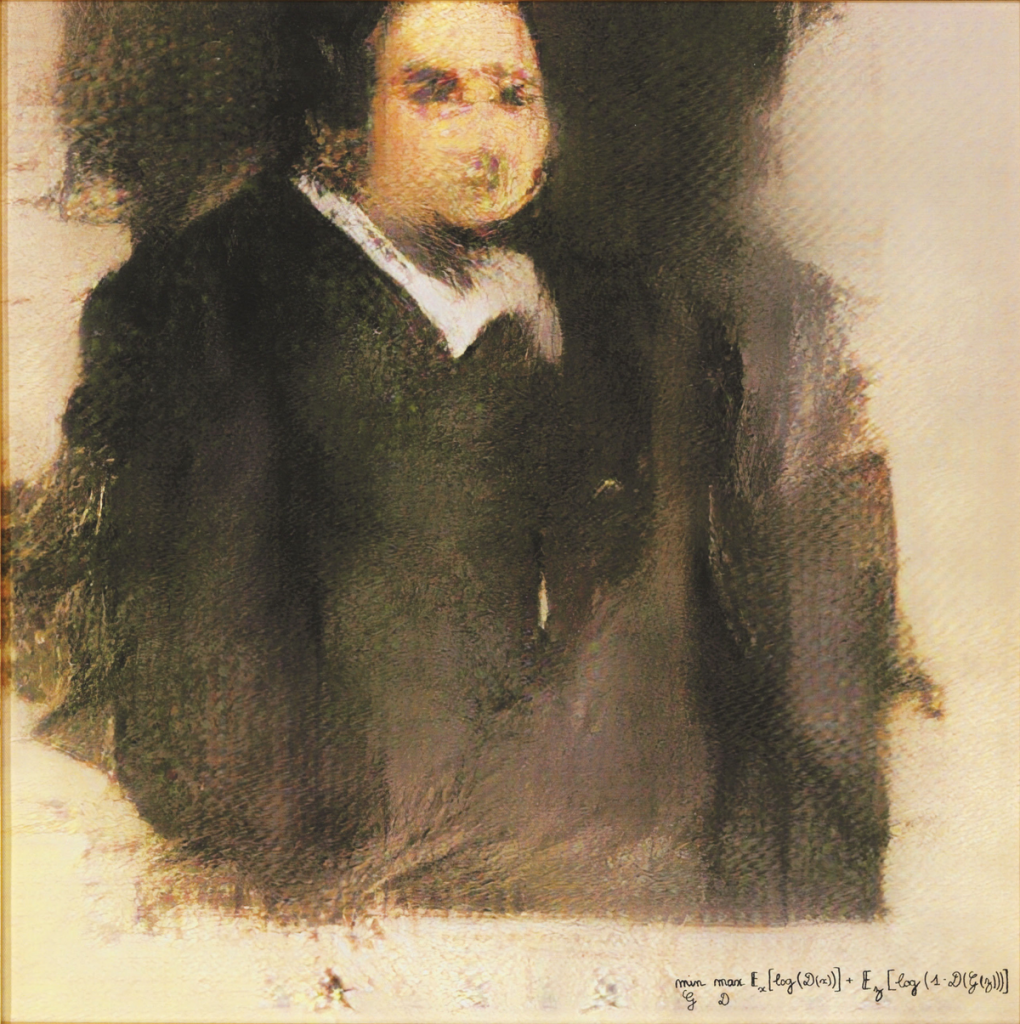
Created by the art collective studio based in Paris called Obvious, the painting titled Portait of Edmond de Belamy was generated by an AI algorithm. I find this project to be so intriguing because it replicates a style of art that I don’t typically associate with technology as well as combines historical art styles with new technological developments. It also begins to question the role of creativity in machines and how we can distinguish the human brain and algorithm’s creative processes. The algorithm works using the Generative Adversarial Network algorithm and takes information from 15,000 portraits. The generator is fed the data from 14th and 15th century portraits to produce art and the discriminator reviews these outputs. The artistic sensibilities of this piece are reflected through the 14th and 15th century art styles and also through what the algorithm begins to learn about these styles and techniques.
A video showing the creative process of the painting
Charmaine Qiu – Project 02 – Variable Face
MousePressed was really fun to work with!
/* Charmaine Qiu
Section E
charmaiq@andrew.cmu.edu
Project-02-Variable_Face */
var faceWidth = 200;
var faceHeight = 200;
var eyeSize = 20;
var eyebrow1 = 300;
var eyebrow2 = 300;
var mouthLeft = 230;
var mouthRight = 260;
var star = 5
var star2 = 7
function setup() {
createCanvas(480, 640);
}
function draw() {
background(28, 42, 87);
//stars
fill(255);
ellipse(100, 100, star, star);
ellipse(400, 400, star, star);
ellipse(300, 200, star2, star2);
ellipse(60, 500, star2, star2);
ellipse(120, 450, 6, 6);
ellipse(200, 550, 3, 3);
ellipse(350, 160, 3, 3);
ellipse(80, 260, 3, 3);
ellipse(420, 70, 5, 5);
ellipse(300, 40, 4, 4);
ellipse(420, 580, star, star);
//body
noStroke();
fill(141, 230, 73);
beginShape();
vertex(230, 370);
vertex(225, 450);
vertex(235, 440);
vertex(250, 500);
vertex(255, 490);
vertex(320, 480);
vertex(330, 485);
vertex(320, 430);
vertex(335, 435);
vertex(300, 370);
endShape(CLOSE);
//head
ellipse(width / 2, height / 2, faceWidth, faceHeight);
triangle(220, 230, 230, 200, 240, 230);
//eyes
noStroke();
fill(0);
var eyeLX = width / 2 - faceWidth * 0.25;
var eyeRX = width / 2 + faceWidth * 0.25;
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
fill(222, 45, 13);
ellipse(eyeLX, height / 2, eyeSize / 2, eyeSize / 2);
ellipse(eyeRX, height / 2, eyeSize / 2, eyeSize / 2);
//eyebrows
triangle(150, 295, 160, 290, 190, eyebrow1);
triangle(290, eyebrow1, 320, 290, 330, 295);
//nose
ellipse(width / 2, height/ 2, 7, 10);
//mouth
triangle(mouthLeft, 400, 240, 390, mouthRight, 400);
// strokeWeight()
// line(200, 320, width / 2 - faceWidth * 0.25, 320);
// line(width / 2 - faceWidth * 0.25, 320, 260, 320);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'faceWidth' gets a random value between 75 and 150.
faceWidth = random(150, 300);
eyeSize = random(20, 50);
eyebrow1 = random(290, 302);
eyebrow2 = random(290, 302);
mouthLeft = random(220,240);
mouthRight = random(240,260);
star = random(2, 15);
star2 = random(2, 15);
}
Alice Cai Project 02
var eyeSize = 50;
var faceWidth = 250;
var faceHeight = 300;
var mouthWidth = 100
var mouthHeight = 50
var flufF = 50
function setup() {
createCanvas(640,480);
}
function draw() {
background(252, 136, 3);
//body
fill(234, 255, 0);
strokeWeight(5);
ellipse(width / 2, height, faceWidth, faceHeight);
//face
fill(234, 255, 0);
strokeWeight(5);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
//eyes
fill(255);
stroke(0);
strokeWeight(0);
var eyeLX = width / 2 - faceWidth * 0.2;
var eyeRX = width / 2 + faceWidth * 0.2;
ellipse(eyeLX + 10, height / 2, eyeSize + 10, eyeSize);
ellipse(eyeRX + 10 , height / 2, eyeSize + 10, eyeSize);
fill(0);
stroke(0);
strokeWeight(0);
var eyeLX = width / 2 - faceWidth * 0.2;
var eyeRX = width / 2 + faceWidth * 0.2;
ellipse(eyeLX, height / 2, eyeSize, eyeSize);
ellipse(eyeRX, height / 2, eyeSize, eyeSize);
//mouth
fill(194, 66, 66);
strokeWeight(0);
ellipse(width / 2, height / 1.5, mouthWidth, mouthHeight);
//fluff
stroke(234, 255, 0);
strokeWeight(30);
line(width / 2.05, 80, width /2.1 , 120);
stroke(234, 255, 0);
strokeWeight(20);
line(width / 2.5, 80, width /2.1 , 120);
stroke(234, 255, 0);
strokeWeight(10);
line(width / 2.3, 50, width /2.1 , 120);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'faceWidth' gets a random value between 75 and 150.
faceWidth = random(200, 250);
faceHeight = random(250, 350);
eyeSize = random(30, 60);
mouthHeight = random(40, 60);
mouthWidth = random(75, 100);
}
This is my chick! I first made variables for the values that I wanted to change. Then made a canvas and shapes. I used canvas width/height variable to control a lot of shape positioning. Finally, I set some values to random when click, and also set restraints so shapes don’t come out of the face.