var r = 254;
var g = 247;
var b = 255;
var angle = 0;
function setup() {
createCanvas(600, 450);
background(220);
frameRate(20);
}
function draw() {
//bg color change
background(r, g - (mouseX / 15), b - (mouseY / 20));
//spinning rect
push();
fill(225, 250, 220, 50);
rectMode(CENTER);
translate(300, 225);
rotate(radians(angle));
rect(0, 0, 800, 20);
rect(0, 0, 20, 800);
pop();
angle += 5;
//rotating stars
push();
translate(width / 2, height / 2);
rotate(radians(mouseX / 7));
//blue stars
fill(227, 252, 250);
for (let i = 0; i < 6; i++){
push();
rotate(radians(60 * i));
translate(200 , 0);
star();
pop();
}
//pink stars
fill(255, 240, 240);
for (let i = 0; i < 6; i++){
push();
rotate(radians(60 * i + 30));
translate(200 , 0);
star();
pop();
}
pop();
//back circle
noStroke();
fill(255, 247, 204, 30);
circle(width / 2, height / 2, min(mouseX, 350));
fill(255, 247, 204, 70);
circle(width / 2, height / 2, min(mouseX, 300));
fill(240, 230, 122);
circle(width / 2, height / 2, 230);
//Drawing Grandma
//body
push();
var y = constrain(mouseY, 200, 400);
noStroke();
fill(247, 126, 142);
rect(width/2 - 120, y + 30, 240, y + 200, 80);
fill(245, 238, 164);
circle(width/2, y + 120, 10);
circle(width/2, y + 150, 10);
circle(width/2, y + 180, 10);
//head
noStroke();
fill(250, 231, 217);
ellipse(width/2, y, 200, 200);
//ears
ellipse((width/2)-90, y, 50, 50);
ellipse((width/2)+90, y, 50, 50);
//glasses
strokeWeight(1);
noFill();
stroke(30);
arc(width/2, y, 20, 20, PI, 0);
fill(235, 241, 255);
ellipse(width/2 - 20, y, 25, 25);
ellipse(width/2 + 20, y, 25, 25);
//nose
stroke(240, 208, 189);
strokeWeight(20);
strokeJoin(ROUND);
fill(240, 208, 189);
triangle(width/2, y + 5, width/2 - 10, y+20 ,width/2 + 10, y+20);
//hair
fill(214);
noStroke();
ellipse(width/2, y - 130, 60, 65);
push();
translate (width/2 + 50, y - 80);
rotate(radians(120));
ellipse (0, 0, 50, 130);
pop();
push();
translate (width/2 - 50, y - 80);
rotate(radians(-120));
ellipse (0, 0, 50, 130);
pop();
pop();
}
function star() {
//drawing the star
push();
rectMode(CENTER);
square(0, 0, 20);
rotate(radians(45));
square(0, 0, 20);
pop();
}
Month: September 2020
LO-03-Computational Art

This project explores how humankind can combine kinetic manufacturing with the nature’s biological construction. I’m most amazed by the fact that the creators actually directed the movement of the silkworms to monitor the thickness and silk layer produced. I admire this aspect so much because silkworms are living creatures and there are is no way that we can communicate with them to discipline or guide them, which makes the process of developing the kinetic manipulation very difficult since one tiny miscalculated factor could lead to a totally unexpected result structure.
I assume the team combined chemical, biological, physics, architectural and programming knowledge to put this project together. They should’ve programmed the various environmental factors of the room to be within a certain range for the silkworms to move the way they are expected to as well as calculating the position and size of the holes in the structure that release tensile stress.
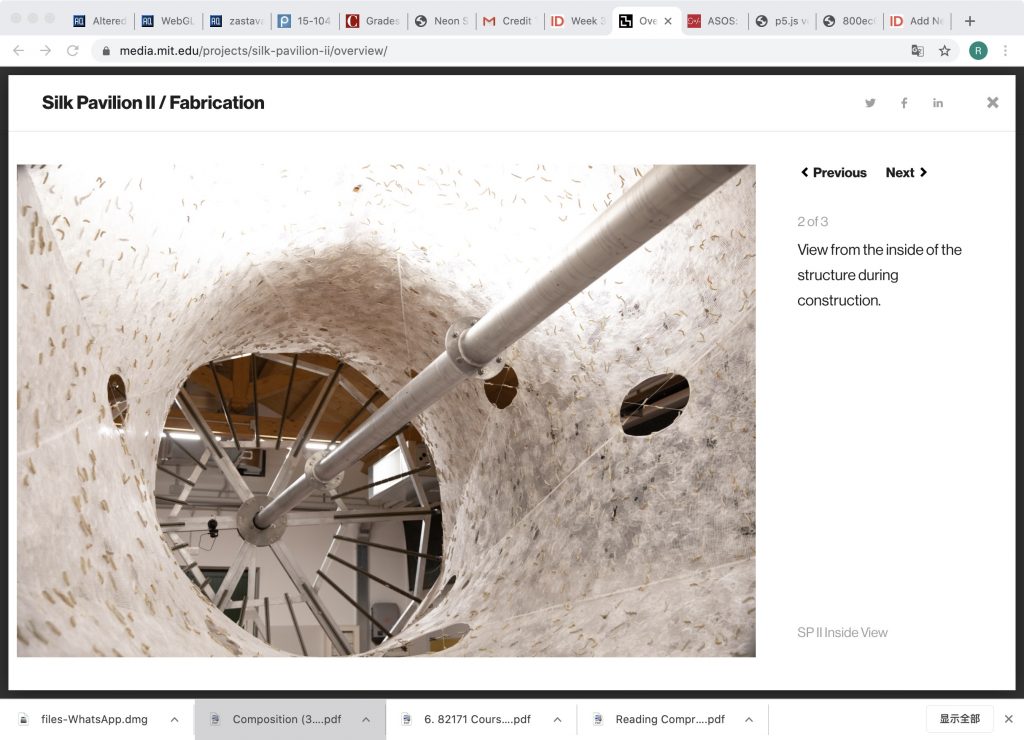
The team’s architectural background made this project a good combination of the natural curvy shape and scientific cleanness of the structure.
LO-03: Computational Fabrication
Designer Michael Schmidt and architect Francis Bitonti collaborated to create this 3D printed gown that is specifically designed for the model Dita Von Teese. They enforced the spiral formula to the computer rendering of the dress that would emphasize femininity qualities of her body. This was something that interested me as a design major, since I didn’t really connect fashion with coding. By learning about this project, I realized that computational art is a broad field that can be applied to anything related to design or art. The idea of bringing digital design into a physical form was fascinating. In addition, the complicated process of creating this dress highlights its beauty. The floor-length nylon gown was made using selective laser sintering (SLS), which builds up the material in layers from plastic powder fused together with a laser. The rigid plastic components are completely articulated to create a netted structure for fluidity and movement. Also they applied spirals based on the Golden Ratio to the computer rendered Von Teese’s body so that the garment would fit her perfectly. The dress has 4000 articulative joints and all were written into CAD code so that they can be printed.

Project 03: Pentachoron
Enjoy the lines and connections and gradient changes. I imagined this to be a screensaver.
/*
Nicholas Wong
Section A
*/
var triSize = 100; //Triangle size (arbitrary number)
var rectSize = 400; //Rectangle size (also arbitrary)
var colorChange = false; //True means color value decreases, false means it increases
var shadeNum = 255; //Color value to be manipulated
function setup() {
createCanvas(450,600);
}
function draw() {
cursor(CROSS); // Changes Cursor to a cross
//Constrain mouse position to canvas
let mx = constrain(mouseX, 10, width - 10)
let my = constrain(mouseY, 10, height - 10)
//Background gets lighter as mouse moves further from center
background(dist(mouseX,mouseY,width/2,height/2) * 0.1);
//Stroke properties for lines
noFill();
stroke(255 - (dist(mouseX,mouseY,width/2,height/2) * 0.5)) //Lines get darker as mouse moves from center
strokeWeight(1.5)
//Square in background moves with mouse slightly
square(width/2 - rectSize/2 + 0.1*mx - 20, height/2 - rectSize/2 + my*0.1 - 20, rectSize)
//Large triangle moves with mouse slightly
triangle(width/2 - triSize*2 + my*0.25, height/2 - triSize*1.5 + mx*0.25 , width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25, width/2 - my*0.25, height/2 + triSize*2 - mx*0.25)
//Lines that connect 3 corners of the larger triangle to one corner of the smaller triangle
//Corner 1 (top left smaller triangle)
line(width/2 - triSize + mx*0.25, height/2 - triSize + my*0.25,width/2 - triSize*2+ my*0.25, height/2 - triSize*1.5 + mx*0.25)
line(width/2 + triSize - my*0.25, height/2 - triSize + mx*0.25,width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25)
line(width/2 - mx*0.25, height/2 + triSize - my*0.25, width/2 - my*0.25, height/2 + triSize*2 - mx*0.25)
//Corner 2 (top right smaller triangle)
line(width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25,width/2 - triSize + mx*0.25, height/2 - triSize + my*0.25)
line(width/2 - triSize*2 + my*0.25, height/2 - triSize*1.5 + mx*0.25,width/2 + triSize - my*0.25, height/2 - triSize + mx*0.25)
line(width/2 - triSize*2 + my*0.25, height/2 - triSize*1.5 + mx*0.25,width/2 - mx*0.25, height/2 + triSize - my*0.25)
//Corner 3 (bottom smaller triangle)
line(width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25,width/2 - mx*0.25, height/2 + triSize - my*0.25)
line(width/2 - my*0.25, height/2 + triSize*2 - mx*0.25,width/2 - triSize + mx*0.25, height/2 - triSize + my*0.25)
line(width/2 + triSize - my*0.25, height/2 - triSize + mx*0.25,width/2 - my*0.25, height/2 + triSize*2 - mx*0.25)
//Connecting 3 corners of the rectangle to one corner of the large triangle
//Corner 1 (top left large triangle)
line(width/2 - triSize*2 + my*0.25, height/2 - triSize*1.5 + mx*0.25, width/2 - rectSize/2 + 0.1*mx - 20, height/2 - rectSize/2 + my*0.1 - 20)
line(width/2 - triSize*2 + my*0.25, height/2 - triSize*1.5 + mx*0.25, width/2 + rectSize/2 + 0.1*mx - 20, height/2 - rectSize/2 + my*0.1 - 20)
line(width/2 - triSize*2 + my*0.25, height/2 - triSize*1.5 + mx*0.25, width/2 - rectSize/2 + 0.1*mx - 20, height/2 + rectSize/2 + my*0.1 - 20)
//Corner 2 (top right large triangle)
line(width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25,width/2 - rectSize/2 + 0.1*mx - 20, height/2 - rectSize/2 + my*0.1 - 20)
line(width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25,width/2 + rectSize/2 + 0.1*mx - 20, height/2 - rectSize/2 + my*0.1 - 20)
line(width/2 + triSize*2 - mx*0.25, height/2 - triSize*1.5 + my*0.25,width/2 + rectSize/2 + 0.1*mx - 20 , height/2 + rectSize/2 + my*0.1 - 20)
//Corner 3 (bottom large triangle)
line(width/2 - my*0.25, height/2 + triSize*2 - mx*0.25, width/2 - rectSize/2 + 0.1*mx - 20 , height/2 + rectSize/2 + my*0.1 - 20)
line(width/2 - my*0.25, height/2 + triSize*2 - mx*0.25, width/2 + rectSize/2 + 0.1*mx - 20 , height/2 + rectSize/2 + my*0.1 - 20)
//Pulsating gradient effect for triangle in middle
if (shadeNum >= 255){
colorChange = true; //Change direction of gradient change
}
else if (shadeNum <= 0){
colorChange = false; //Change direction of gradient change
}
if (colorChange){ //Color becomes darker
shadeNum -= 2;
}
else{ //Color becomes lighter
shadeNum += 2;
}
//Triangle 3
fill(shadeNum) //Pulsating gradient shade
triangle(width/2 - triSize + mx*0.25, height/2 - triSize + my*0.25 , width/2 + triSize - my*0.25, height/2 - triSize + mx*0.25, width/2 - mx*0.25, height/2 + triSize - my*0.25)
//Stroke properties to lines connected to mouse
push();
strokeWeight(4);
stroke(dist(mouseX,mouseY,width/2,height/2) * 0.25) // Line gets brighter as it moves further from center
//Lines from smaller triangle that connect to mouse
line(width/2 - triSize + mx*0.25, height/2 - triSize + my*0.25, mx, my);
line(width/2 + triSize - my*0.25, height/2 - triSize + mx*0.25, mx, my);
line(width/2 - mx*0.25, height/2 + triSize - my*0.25, mx, my);
pop();
}
rdrice_sectionC_Project 2_FACE
//Robert Rice
//Section C
var gender = 0 //"gender", determines eyelashes
var eyeSize = 10 //sets eye diameter
var eyeSquint = 1 //how squinted the eyes are
var eyebrowLength = 70 //how long the eyebrows are
var mouthLength = 90 //how long the mouth is
var mouthPosition = 0 //adjust how high up the mouth is
var noseX1 = 250 //adjusts the base of the nose
var noseY1 = 230
var noseX2 = 270 //adjust the tip of the nose
var noseY2 = 220
function setup() {
createCanvas(400, 400);
text("p5.js vers 0.9.0 test.", 10, 15);
angleMode(DEGREES); //degrees bc i'm bad at math
}
function draw() {
background(0) //black background
noFill();
stroke(255); //white lines bc the background is black
strokeWeight(3); //draws the basic face shape
strokeJoin(ROUND);
line(140,270, 127,220);
arc(193,202, 136,136, 165, 73.38);
line(212,268, 232,288);
strokeWeight(2); //stroke weight for facial features
if (gender>0.5) { //Gives the face eyelashes if it's 'female'
line(186,180, 172,180); //does nothing if the face is 'male'
line(186,180, 174,173);
line(186,180, 180,168);
line(186+97,180, 172+97,180);
line(186+97,180, 174+97,173);
line(186+97,180, 180+97,168);
}
noStroke(); //Makes the pupils
fill(255);
ellipse(186,180, eyeSize, eyeSize / eyeSquint); //pupils squint based on
ellipse(186+97,180, eyeSize, eyeSize / eyeSquint); //eyeSquit variable
stroke(255); //makes the eyebrow arcs, which move with the eyes
strokeWeight(2);
noFill();
arc(201,6-((eyeSize/eyeSquint)/2), 351,351, eyebrowLength, 94.91);
//left eyebrow arc
arc(201+97,6-((eyeSize/eyeSquint)/2), 351,351, eyebrowLength, 94.91);
//right eyebrow arc
arc(246,78 - mouthPosition, 342,342, mouthLength,111.06); //mouth
noStroke();
fill(255);
triangle(255,188, noseX1,noseY1, noseX2,noseY2);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges.
gender = random(0,1); //variables defined at top of code
eyeSize = random(6,20);
eyeSquint = random(1,2);
eyebrowLength = random(69.42, 82.17);
mouthLength = random(79.13, 104.53);
mouthPosition = random(0,35);
noseX1 = random(247, 260);
noseY1 = random(200, 238);
noseX2 = random(270, 290);
noseY2 = random(199, 232);
}
Looking Outwards: Parametric Architecture
As an architecture student, parametric or generative design is a new field that is slowly gaining traction. This new method of designing allows for unexpected, novel ideas to emerge, and allows for rapid iteration with these completely original forms. The ability to use designs driven by algorithms allows one to bypass the technical constraints of designing forms with highly complex, repetitive elements. Furthermore, generative modeling allows for the creation of more organic and amorphous designs thanks to artificial intelligence being able to resolve complex issues like structural viability/stability.
Currently, parametric design is being used practically mainly for aesthetic design features such as building facades, interior light fixtures, fenestration designs, and so on. However, generative designs will eventually become integral parts of overall structural form-finding for buildings and homes.
LMN Architects’ Voxman Music Building’s ceiling is a prominent example of generative architecture being utilized. The ceiling was parametrically designed, from its organic structure to the small, triangular apertures arranged on its surface. “The design integrates acoustic reflection, stage and house lighting, audiovisual elements,, and fire suppression into a single eye-popping ceiling system” – Architect Magazine.


Project 03 – Dynamic Flower
Originally, I had no design in mind for my project. After creating some elements, I realized I had the base for a flower. I used differing opacities to create an aesthetic design.
var x = -225;
var y = -300;
/*d was used as a variable for translation as the artwork was originally
created with a grid*/
var d = 50;
var r = 50;
var angle = 45;
var colorChange = 255;
function setup() {
createCanvas(450, 600);
background(255);
}
function draw() {
var colorReduce = mouseX / 50
background(255);
var extend = 3 * d; //stretches dimensions on varying shapes
translate(width / 2, height / 2); //makes the center the origin
extend -= .3 * mouseX; //modifies the stretching variable
//determines whether an RGB value should be increased or decreased
if (mouseX <= width / 2){
colorChange -= colorReduce
} else {
colorChange = 255
colorChange += colorReduce;
}
/*creates the triangle-circle combination and rotates according to
mousePressed*/
push();
fill(0, 0, colorChange, 60);
rotate(radians(45 - 2 * angle)); //rotates the shapes around the center
translate(-4.5 * d, -6 * d); //simplifies the location of each combo
circle(7 * d + extend, 6 * d, 50);
triangle(5 * d, 6.5 * d, 5 * d, 5.5 * d, 7 * d + extend, 6 * d);
circle(4.5 * d, 9 * d + extend, 50);
triangle(5 * d, 6.5 * d, 4.5 * d, 9 * d + extend, 4 * d, 6.5 * d);
circle(2 * d - extend, 6 * d, 50);
triangle(4 * d, 6.5 * d, 2 * d - extend, 6 * d, 4 * d, 5.5 * d);
circle(4.5 * d, 3 * d - extend, 50);
triangle(4 * d, 5.5 * d, 4.5 * d, 3 * d - extend, 5 * d, 5.5 * d);
pop();
/*creates the tilted petals of the flower and rotates them with the center
when the mouse is pressed*/
push();
rotate(radians(45 + angle));
fill(120, 30, 220, 35);
circle(140, 0, 280);
circle(0, 140, 280);
circle(-140, 0, 280);
circle(0, -140, 280);
pop();
//creates the non-tilted petals and rotates around the center with mouse
push();
rotate(radians(angle));
fill(255, 176, 221, 120);
circle(140, 0, 280);
circle(0, 140, 280);
circle(-140, 0, 280);
circle(0, -140, 280);
pop();
/*creates the ellipses in the center of the petals and rotates them around
the center*/
push();
fill(colorChange, 192, 176, 200);
rotate(radians(2 * angle));
ellipse(0, 100 + r, r, 2 * r);
ellipse(0, -100 - r, r, 2 * r);
ellipse(100 + r, 0, 2 * r, r);
ellipse(-100 - r, 0, 2 * r, r);
pop();
//changes the size of the ellipses based on mouse position
if (mouseY >= height / 2) {
r = 45 + .05 * mouseY;
} else {
r = 50
}
//creates the green center square and rotation for mousePressed
push();
rotate(radians(angle - 45));
rectMode(CENTER);
fill(0, 255, 0, 80);
rect(0, 0, 2 * d - 0.6 * extend, 2 * d - 0.6 * extend);
pop();
//creates the red center square and rotation for mousePressed
push();
rotate(radians(angle));
rectMode(CENTER);
fill(255, 0, 0, 80);
rect(0, 0, 2 * d - .6 * extend, 2 * d - .6 * extend);
pop();
//creates the blue gradient when the y-mouse position changes
let i=3;
while (i<15) {
noStroke(); //produces a graident effect based on ellipses
fill(168, 235, 255, 10);
ellipse(width / 3, height + i, mouseY + 50 * i, mouseY + 50 * i);
ellipse(-width / 3, -height, mouseY + 50 * i, mouseY + 50 * i);
ellipse(width / 3, -height, mouseY + 50 * i, mouseY + 50 * i);
ellipse(-width / 3, height + i, mouseY + 50 * i, mouseY + 50 * i);
i += 1; //increases the modifier as long as i<15
}
}
function mousePressed() {
//for when mouseX is less than the width, the angle of rotations increase
if (mouseX < width) {
angle += 45
}
}
LO-03-Computational Fabrication
Neri Oxman’s Silk Pavilion. 2013.
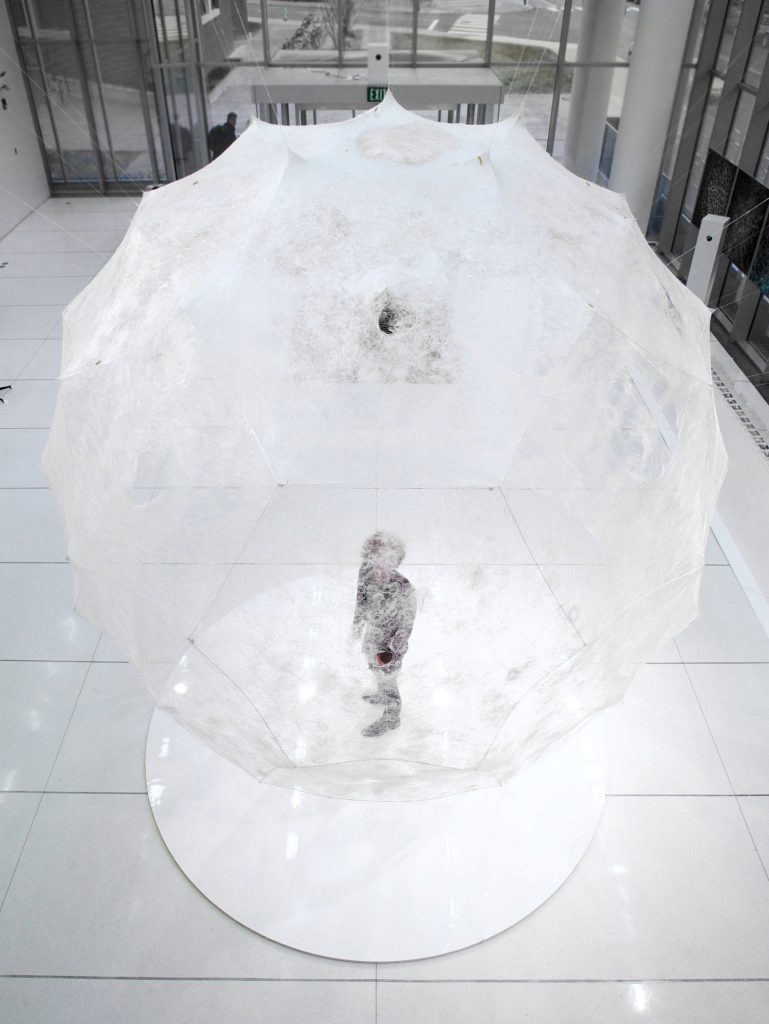
The Silk Pavilion uses a combination of computational and biological fabrication to create a pavilion that articulates the beauty and structure of silk while using a sustainable method of gathering silk. The project addresses that about 1,000 silk cocoons are boiled per tshirt, which kill the larva in the process. The silk pavilion makes the silkworms spin in sheets rather than cocoons due to the human made structure and grid, thus allowing a more sustainable method of harvesting silk.
The primary structure uses a CNC (computer numerical control) machine to lay down 25 panels of silk thread. 6,500 silkworms were then used to finish the rest of the structure.
The algorithms that programmed the robotic arm to create the primary structure must have first analyzed the natural biological process and pattern that silkworms take when forming cocoons as the project wanted to replicate that process. Neri Oxman’s team attached small magnets on the heads of the silkworms to track their movements, and using that data they then created a path for the arm to move in ways like the worms.
I found this project fascinating for a multitude of reasons, the first being the combination of biological processes and digital processes. Additionally, the Silk Pavilion is able to achieve an architectural scale of a process that is done on a much smaller scale (silkworm cocoons). In this case, studying biological formations allows for us to vary scales of production of natural systems. By analyzing nature we can create complex parametric designs that allow us to reflect organic structures and designs with computer based methods of learning. Oxman’s ambition to combine the organic with computers reflects in the pavilion in its large form that displays the natural path of silkworms shown in varying patches of density.
Pinwheel drawing
My process for this project at first was to just mess around and see what shapes i can get to move, and then I realized I can make a moving pinwheel thats speed and direction is determined by mouse clicks and what side of the canvas the clicks occur. Everything after the pinwheel was design to make the appearance look better, the pinwheel is the main part of this piece.
//Anthony Prestigiacomo aprestig section:C
var angle=0;
var degree=5;
var x=-300;
var y=0;
var hvel=5;
var cloudx=0;
var cloudxx=0;
cloudyy=0;
var cloudy=-10;
var r=50;
var vcloud=2;
var vcloud2=2.75;
var suncolor=0;
var bcolor=0;
var mooncolor=0;
function setup() {
createCanvas(600, 450);
background(203,234,246);
}
function draw() {
background(203, 234, 246);
noStroke();
fill(bcolor);
rect(0,0,600,450); //night sky
translate(width / 2, height / 3);
push();
if(mouseX>300){ //twinkling stars
scale(2);
};
fill(203, 234, 246);
circle(50,100,1); //stars
circle(-50,100,1);
circle(57,250,1);
circle(82,-58,1);
circle(-200,133,1);
circle(-5,-124,1);
circle(-72,-230,1);
circle(100,10,1);
circle(150,45,1);
circle(-150,-67,1);
circle(50,50,1);
circle(-50,-50,1);
circle(-50,50,1);
circle(-150,125,1);
circle(-75,-30,1);
circle(-45,-75,1);
circle(-75,-75,1);
circle(-200,-125,1);
pop();
push();
noStroke();
fill(suncolor); //sun
circle(-150,-150,250);
fill(203, 234, 246); //moon
circle(150,150,250);
fill(mooncolor);
circle(105,105,25); //moon craters
circle(100,220,20);
circle(175,175,75);
circle(190,90,40);
push();
translate(-300,-height/3);
fill(212,124,47); //bird on the mouse
circle(mouseX,mouseY,50);
fill(48,46,59);
circle(mouseX+25,mouseY-13,25);
fill(105,107,102);
triangle(mouseX-35,mouseY-5,mouseX+5,mouseY-5,mouseX-25,mouseY+25);
fill(255,255,0);
triangle(mouseX+25, mouseY-13, mouseX+30,mouseY-13, mouseX+25, mouseY-8);
pop();
fill(255); //cloud white
circle(cloudx,cloudy,r); //top cloud
circle(cloudx+25,cloudy,r);
circle(cloudx+12.5,cloudy-25,r);
circle(cloudxx-100, cloudy+150,r); //bottom cloud
circle(cloudxx-75,cloudy+150,r);
circle(cloudxx-87.5,cloudy+125,r);
cloudxx+=vcloud2;
cloudx+= vcloud;
if(cloudxx>400){ //makes clouds reappear on left side of screen
cloudxx=-300;
};
if(cloudx>325){
cloudx=-300;
}
pop();
push();
strokeWeight(10);
stroke(0);
line(0,0,0,height); //pinwheel handle
noStroke();
rotate(radians(angle)); //rotate pinwheel
push();
push();
if(mouseX>width/2){ //scale white triangles
scale(.5)
};
fill(255);
triangle(0,0,100,100,60,10);
triangle(0,0,100,-100,10,-60);
triangle(0,0,-100,100,-10,60);
triangle(0,0,-100,-100,-60,-10);
pop();
if(mouseY>height/3){ //scale colored triangles
scale(1.25)
}
fill(255,0,0);
triangle(0,0,0,145,25,50); //red triangle
fill(0,255,0);
triangle(0,0,0,-145,-25,-50); //green triangle
fill(0,0,255);
triangle(0,0,145,0,50,-25); //blue triangle
fill(255,255,0);
triangle(0,0,-145,0,-50,25); //yellow triangle
pop();
pop();
fill(0);
circle(0,0,25); //black middle circle
fill(255);
circle(0,0,12.5); //small white triangle
rect(x,y-105, 100,1); //wind lines
rect(x+50,y-100, 100,1);
rect(x+25,y-95, 100,1);
x +=hvel;
if(x>=300){ //wind starts at left when passing x=300
x=-300
};
angle+=degree;
fill(0,100,0);
rect(-300, 250,600,75); //grass
}
function mousePressed() {
if(mouseX < width / 2) {
degree -= 5
} else {
degree += 5
};
if (mouseX<width/2 & mouseY>height/3){
suncolor=0;
bcolor=0;
mooncolor=color(211,211,211);
} else {
suncolor=color(255,255,0);
bcolor=color(203, 234, 246);
mooncolor=color(203, 234, 246);
}
}
LO 03: Computational Fabrication
Artwork: Thallus
Creator: Zaha Hadid Architects
Thallus is a complex 3D-printed sculpture. The form is mesmerizing as an open, expending, and curved piece. The “walls” of the sculpture are created by intricate webbings of curves resembling roots. Not only does this create an aesthetic design, the complexity of it truly impressed me. The generation of the art was done through extensive computational geometry. Additionally, more algorithms had to be created for the printing robots to create the spiraling effect and create the artwork in a single piece. A large amount of research was done by the Zaha Hadid to not only create the design with computations, but also to enable the actual printing. The artistry of the designers is apparent with the expansive, yet open spiral that creates a flow to the work. Every part of the piece perfectly coincides with each other to create a balance of elegance and abstractness. The art itself is impressive, however, the most substantial part to me is the complex mathematics and robotic algorithms that actually allowed for the design to come to life in the 3D format.
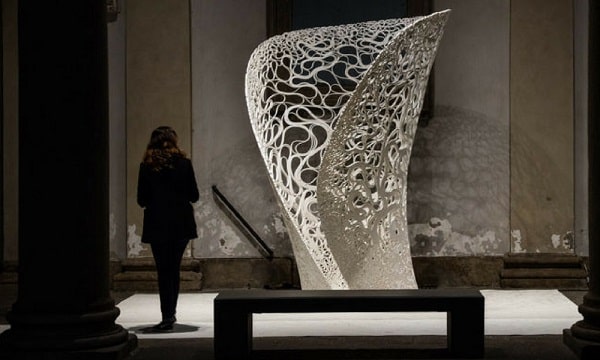