For this project, I wanted to create Van Gogh’s self portrait with the text “Van Gogh.” By using custom pixels, I was able to make words appear on the canvas in relation to the pixel color of the original image. Wait a little bit to view the full portrait!
let img;
function preload() {
//preloading the van gogh image
img = loadImage("https://i.imgur.com/wUgkJwF.jpg");
}
function setup() {
createCanvas(300, 400);
imageMode(CENTER);
background(0);
img.loadPixels();
}
function draw() {
//coverage of the words on the canvas
let randomWords = random(0, 400);
let randomWordstwo = random(0, 300);
//placement of words in relation to the image width and height
let x = floor(random(img.width));
let y = floor(random(img.height));
//pixel and text relationship
let pixel = img.get(x, y);
fill(pixel, 800);
textSize(5);
text('van gogh', x, y, randomWords, randomWordstwo);
}
Image Source: https://i.imgur.com/wUgkJwF.jpg
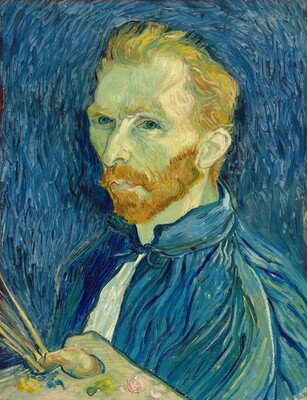
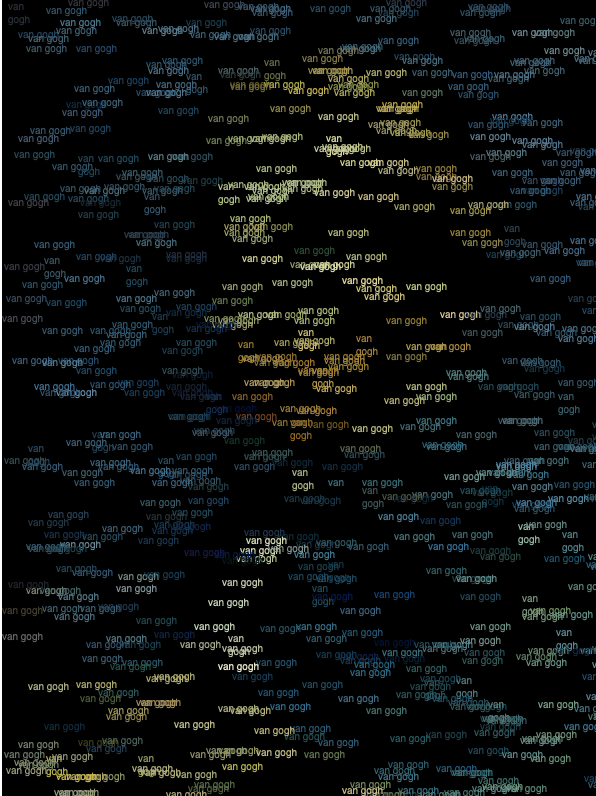
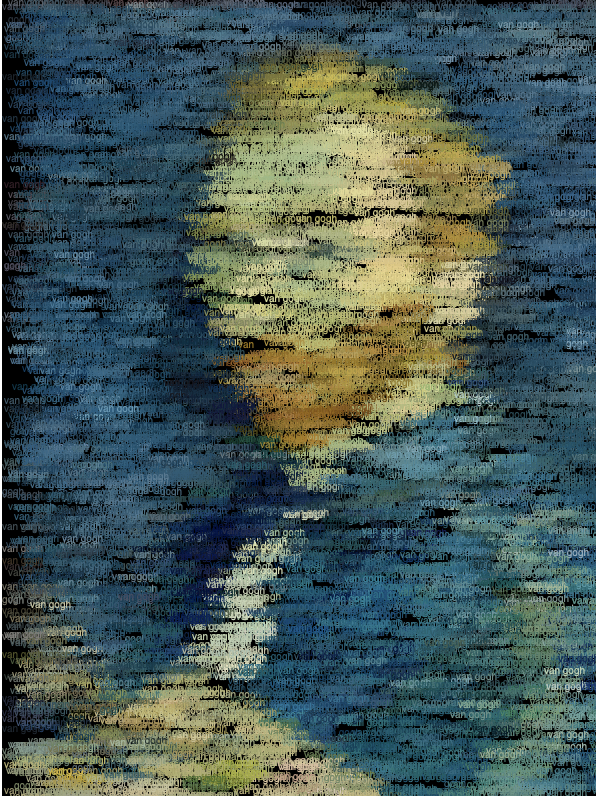