For this project, I first think about the way I want to represent the current time. Then, I choose to use two circles, one controlled by current second, the other controlled by current minute, to represent two planets rotating around the red sun in the center. The lines in gradually varied colors are the hour representation, 1 am/pm to 12am/pm starting from the line in lightest color to the brightest color to the lightest color. Since this is a 12 hours clock, the background color changes based on pm/am. When it is pm, the background is in dark blue, and when it is am, the background will be in light blue.
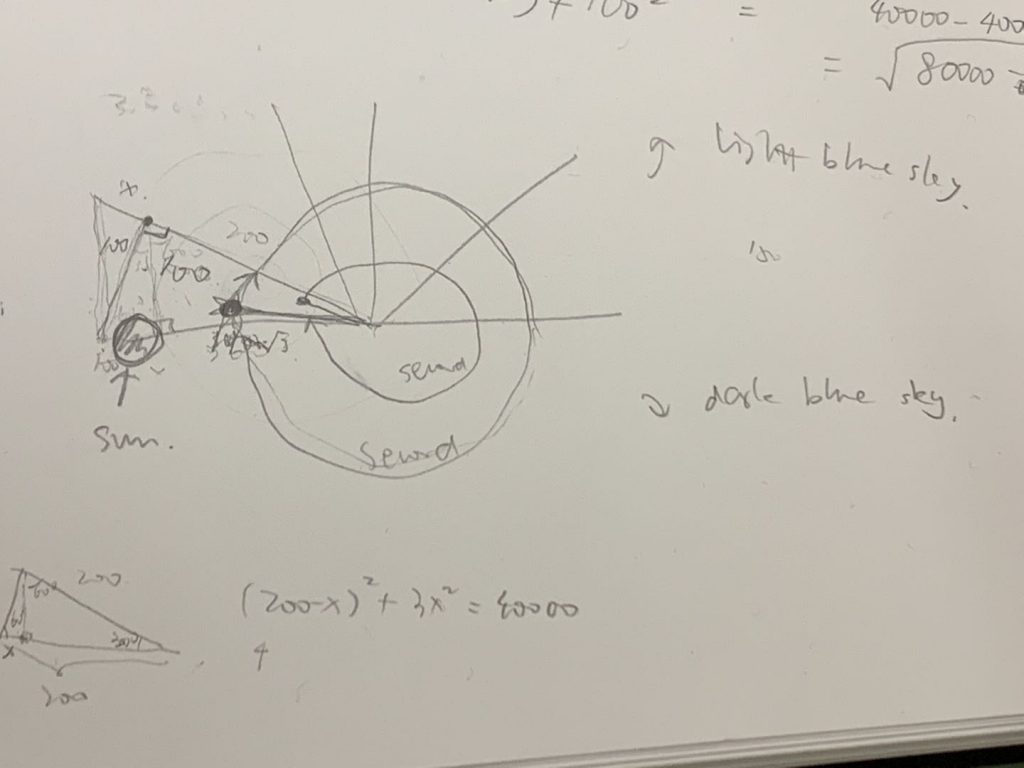
/*Name:Camellia(Siyun) Wang;
Section: C;
Email Address: siyunw@andrew.cmu.edu;*/
var angle= -180;
var radius = 0;
var s;
var h;
var m;
function setup() {
createCanvas(480, 480);
}
function hourLine(h){
//draw the time lines of 1-12 am/pm
translate(240,240);
stroke(238,232,170);
line(-200,0,0,0);//1pm/am
stroke(240,230,140);
line(0,0,200*cos(radians(-150)),200*sin(radians(-150)));//2pm/am
stroke(255,255,0);
line(0,0,200*cos(radians(-120)),200*sin(radians(-120)));//3pm/am
stroke(255,215,0);
line(0,0,200*cos(radians(-90)),200*sin(radians(-90)));//4pm/am
stroke(218,165,32);
line(0,0,200*cos(radians(-60)),200*sin(radians(-60)));//5pm/am
stroke(255,165,0);
line(0,0,200*cos(radians(-30)),200*sin(radians(-30)));//6pm/am
stroke(255,69,0);
line(200,0,0,0);//7pm/am
stroke(240,230,140);
line(0,0,200*cos(radians(150)),200*sin(radians(150)));//8pm/am
stroke(255,255,0);
line(0,0,200*cos(radians(120)),200*sin(radians(120)));//9pm/am
stroke(255,215,0);
line(0,0,200*cos(radians(90)),200*sin(radians(90)));//10pm/am
stroke(218,165,32);
line(0,0,200*cos(radians(60)),200*sin(radians(60)));//11pm/am
stroke(255,165,0);
line(0,0,200*cos(radians(30)),200*sin(radians(30)));//12pm/am
noStroke();
}
//the rotating circle(planet) on the outside representing minute
function minuteSun(m){
stroke(255,165,0);
fill(255,69,0);
circle(150*cos(radians(angle+m*6)),150*sin(radians(angle+m*6)),10);
}
//the rotating circle(planet) on the inside representing second
function secondSun(s){
stroke(255,215,0);
fill(255,99,71);
circle(60*cos(radians(angle+s*6)),60*sin(radians(angle+s*6)),10);
}
//the quuivery line representing current hour
function Hour(h){
strokeWeight(3);
stroke(255,240,245);
line(200*cos(radians(-210+30*h+random(-2,2))),200*sin(radians(-210+30*h+random(-2,2))),0,0);
strokeWeight(1);
}
function draw() {
if(h<=12){
background(173,216,230);//when it is am
}
else{
background(0,0,139);//when it is pm
}
noFill();
strokeWeight(4);
stroke(255,160,122);
circle(240,240,120);//orbit of second planet
circle(240,240,300);//orbit of minute planet
strokeWeight(2);
noStroke();
s = second();
m = minute();
h = hour();
hourLine();
minuteSun(m);
secondSun(s);
Hour(h);
//the center sun
strokeWeight(2);
stroke(255,0,0);
fill(255,0,0);
circle(0,0,20);
noFill();
noStroke();
}