//Catherine Liu
//Section D
//jianingl@andrew.cmu.edu
//Assignment-06-Project
//an abstract clock where the objects move according to seconds, minutes, and hours
var xPos = []; //array for x position of clouds
var yPos = []; //array for y position of clouds
function setup() {
createCanvas(480, 400);
//creates random positions for 60 clouds
for (i = 0; i < 60; i++) {
xPos[i] = random(0,480);
yPos[i] = random(0,150)
}
}
function draw() {
background(155,212,255);
fill(0,0,102);
rect(0,height/2, width, height/2);
//draws a number of clouds according to the current second
for (i = 0; i <= second(); i++) {
clouds(xPos[i], yPos[i]);
}
push();
sunMoon(-150, 0); //calls function that draws the rotating sun and moon
pop();
//aliens move closer to each other according to minutes
print(minute().toString())
rightAlien(480 - 8 * minute(), height/2); //calls function that draws right alien
leftAlien(0 + 8 * minute(), height/2); //calls function that draws left alien
}
function rightAlien (x, y) { //draws right alien
stroke(0,102,51);
strokeWeight(5);
//arm waves up and down according to seconds using mod
if (second() % 2 == 0) {
line(x-40, y-60, x-13,y-30); //left arm
} else if (second() % 2 != 0) {
line(x-45, y-50, x-13,y-30); //left arm
}
line(x+13, y-30, x+30, y-15); //right arm
line(x, y-50, x, y-30); //neck
stroke(204, 255, 153);
line(x-10, y, x-10, height); //left leg
line(x+10, y, x+10, height); // right leg
stroke(0,102,51);
line(x-17, y-70, x-7, y-50); //antennae
line(x+17, y-70, x+7, y-50); //antennae
noStroke();
fill(204, 255, 153);
ellipse(x, y-50, 30,20); //head
rect(x-15, y-35, 30,120,10); //body
fill(0,102,51)
//eyes look back when the aliens pass each other halfway
if (minute() < 31) {
circle(x-10, y-51, 5); // left eye
circle(x-3, y-51, 5); // right eye
} else if (minute() >= 31) {
circle(x+10, y-51, 5); // left eye
circle(x+3, y-51, 5); // right eye
}
}
function leftAlien(x, y) { //draws left alien
stroke(0,102,51);
strokeWeight(5);
//arm waves up and down according to seconds using mod
if (second() % 2 != 0) {
line(x+40, y-60, x+13,y-30); //left arm
} else if (second() % 2 == 0) {
line(x+45, y-50, x+13,y-30); //left arm
}
line(x-13, y-30, x-30, y-15); //left arm
line(x, y-50, x, y-30); //neck
stroke(204, 255, 153);
line(x-10, y, x-10, height); //left leg
line(x+10, y, x+10, height); //right leg
stroke(0,102,51);
line(x-17, y-70, x-7, y-50); //left antennae
line(x+17, y-70, x+7, y-50); //right antennae
noStroke();
fill(204, 255, 153);
ellipse(x, y-50, 30,20); //left man's head
rect(x-15, y-35, 30,120,10); //left man's body
fill(0,102,51)
//eyes look back when the aliens pass each other halfway
if (minute() < 31) {
circle(x+10, y-51, 5); // left eye
circle(x+3, y-51, 5); // right eye
} else if (minute() >= 31) {
circle(x-10, y-51, 5); // left eye
circle(x-3, y-51, 5); // right eye
}
}
function sunMoon (x, y) { //sun and moon rotates according to hour, from 0-12 it is sun, from 12-23 it is moon
translate(width/2, height/2);
var dx = 30 * hour();
rotate (radians(dx));
if (hour() > 12 & hour() <=23) {
fill(255, 243, 176);
arc(x, y, 100, 100, 0, PI + QUARTER_PI, PIE); //moon
ellipse(x + 30, y - 30, 5, 10);
ellipse(x - 30, y + 70, 5, 10);
} else if (hour() >= 0 & hour() <= 12) {
fill(255, 128, 0);
ellipse(x, y, 100, 100); //sun
}
}
function clouds(x, y) { //draws a cloud at position x, y
fill(233, 254, 255);
rect(x, y, 20, 10,30); //cloud
}
I started off this project by sketching a rough idea of what I wanted to make, which was two people playing badminton, with the shuttlecock moving from one end to the next in minutes. The sun and moon would then rotate according to the hour.
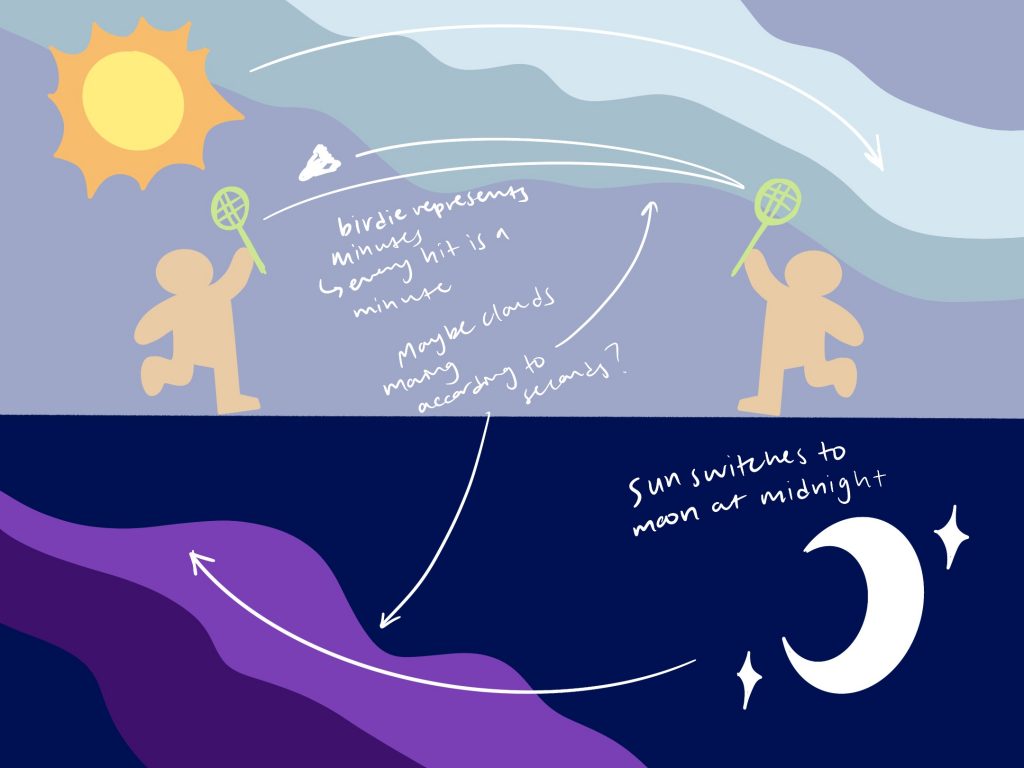
However, I came across some coding problems so I changed my idea slightly. Now, they are aliens that can only move with every minute. As they move closer to each other, they are waving and their eyes follow each other. While I kept the sun and moon concept the same, I changed the background clouds to increase numbers according to seconds.