At first I really did not know how to start off with my portrait. I looked and reread the timeline for a while until I decided I wanted to do some sort of recreation of Chuck Close’s portraits. Instead of using colors to create skin tone, I ended up sticking with the pixel colors that I got using .get for my images. It took me the longest to set up my photo than to actually get my random walk to work.
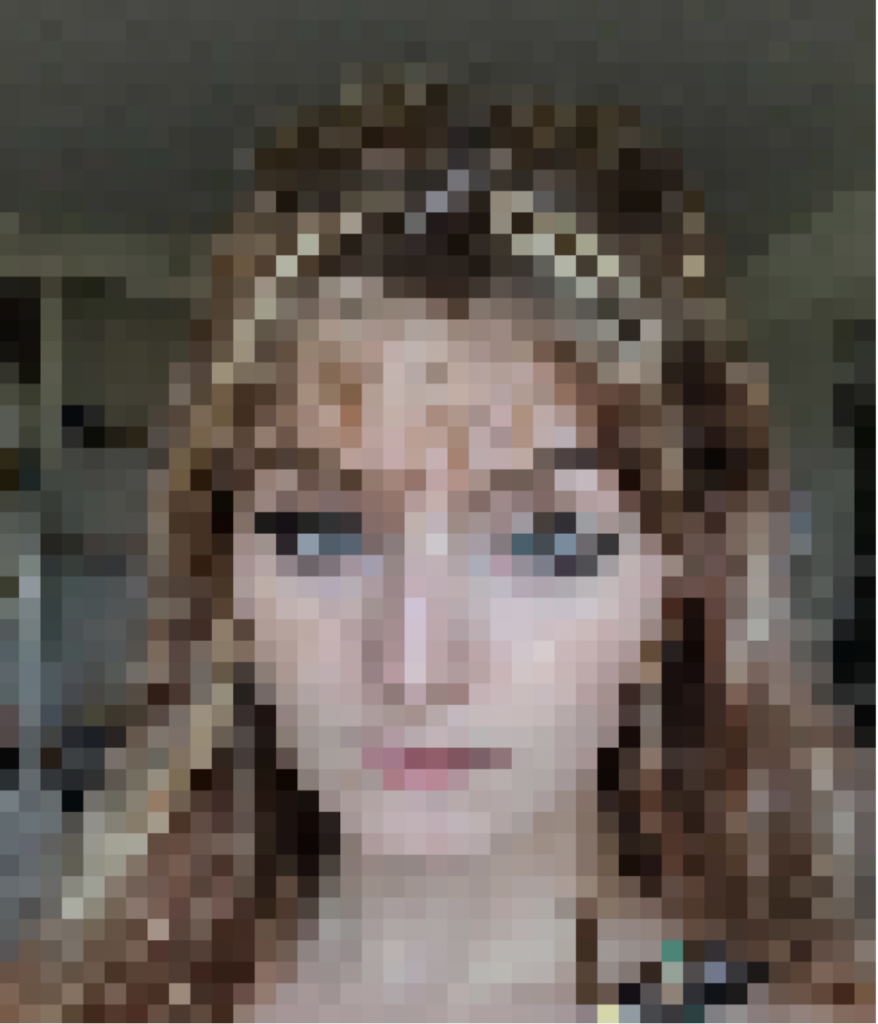
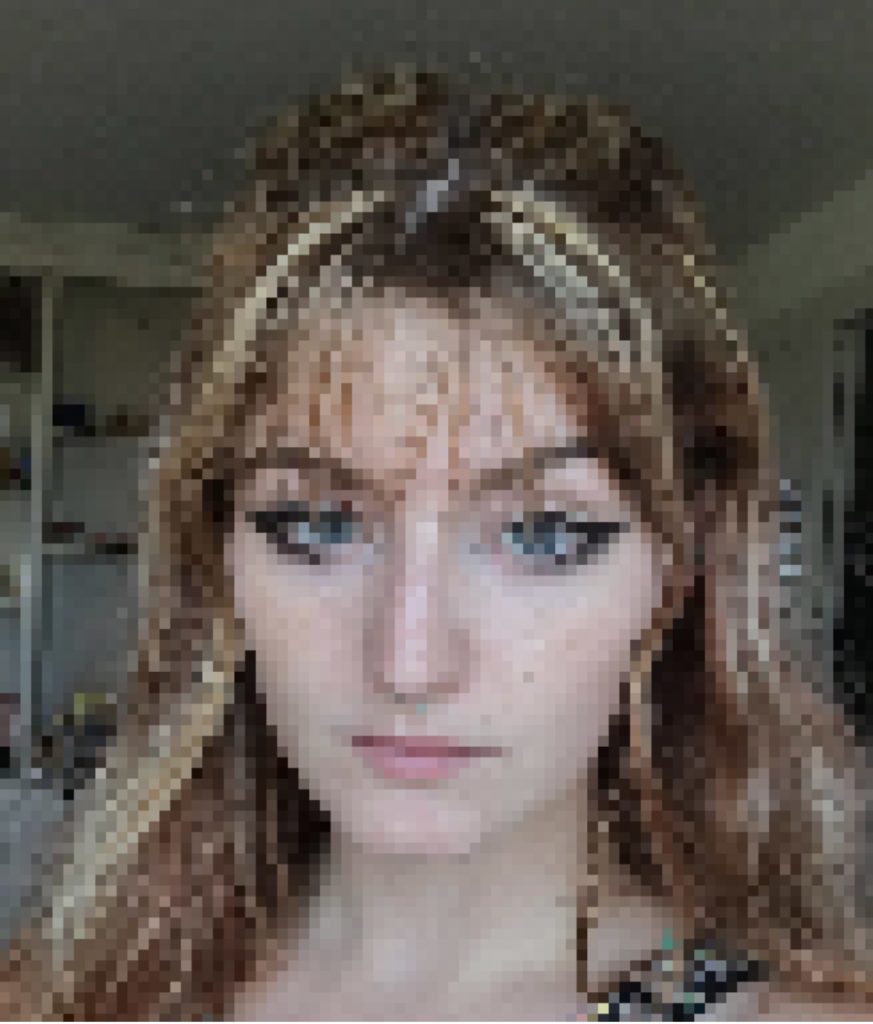
So to get my work to be more like Close’s I made an array of two different size circles inside my pixels. I thought this looked really cool but I was hoping to get more definition in my features by doing this.
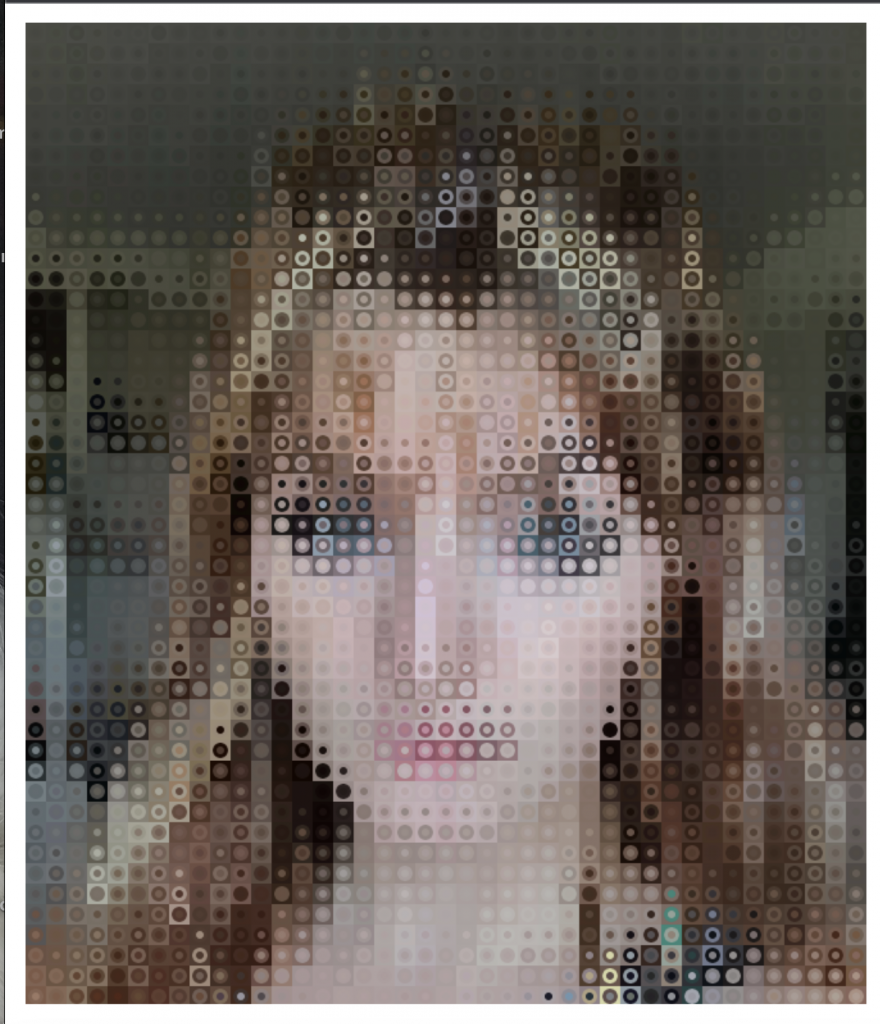
So I ended up using my Ipad to make a grid of areas in the face that I wanted to be more or less pixelated. I ended up counting and working from this top image to make sure I was using the right constrains in my for loops. I probably could have shortened my code for this project, but I was happy with how it was so I did not bother.
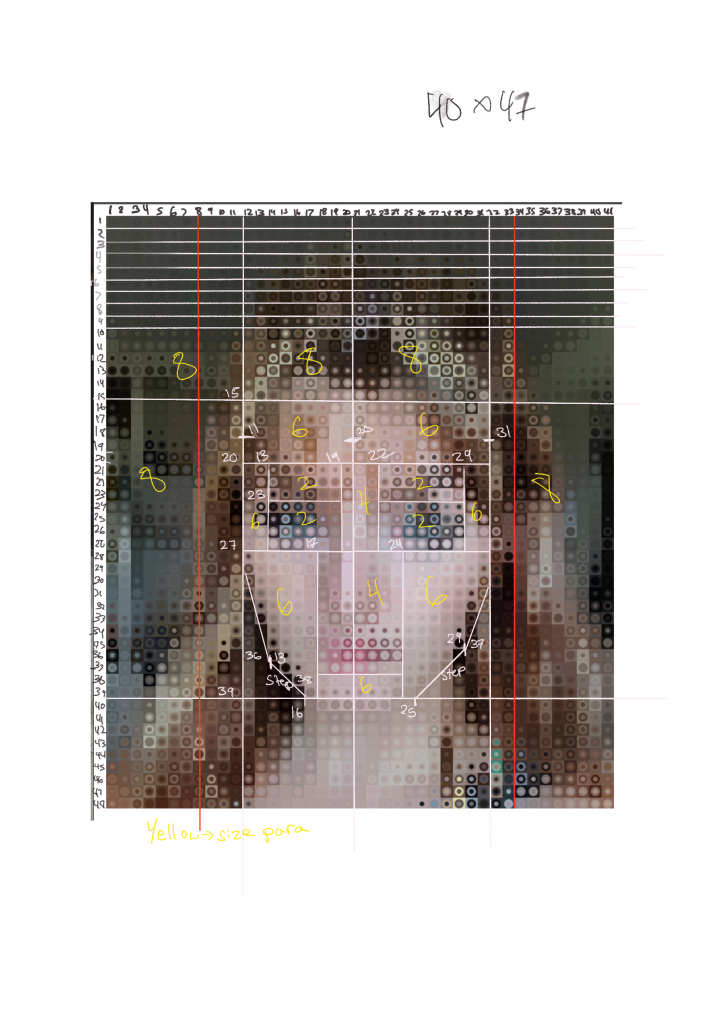
So, for every new and smaller square I was building I was also making my two circles on the top, unless the pixels got too small. I played around with the numbers a lot here. I felt that my image was very stagnant and decided to use noise to get my circles to vibrate in certain parameters. I thought doing this was quite funny because it reminded my a bit of the saying that the Mona Lisas eyes follow you across the room and, I felt that my entire face was doing the same.
var noiseParam = 0;
var noiseStep = 0.1;
var img;
function preload(){
img = loadImage("https://i.imgur.com/5n4h1dT.png");
}
function setup() {
img.resize(img.width/3, img.height/3);//pixel image info
createCanvas(img.width, img.height * .9);
}
function main(dx,dy){
for(var col = 0; col < img.width; col += 8){ //main
for(var row = 0; row < img.height; row += 8){
var c = img.get(col,row);
fill(color(c));
noStroke();
rect(col,row,8,8);
ellipse((col + 4) + dx, (row - 4) + dy, 6, 6);
ellipse((col + 4) + dx, (row - 12) + dy, 3, 3);
}
}
}
function face(){
for(var col = 88; col < 248; col += 6){ //whole face
for(var row = 120; row < 320 ; row += 6){
var c = img.get(col, row);
fill(color(c));
noStroke();
rect((col + 1.5), (row + 1.5), 5, 5);
ellipse((col + 5.5) + dx, (row - 4) + dy, 5, 5);
ellipse((col + 5.5) + dx, (row - 10) + dy, 2.5, 2.5);
}
}
}
function mainFeature(){
for(var col = 104; col < 230; col += 4){ //clarity for face features == more pixels
for(var row = 160; row < 216 ; row += 4){
var c = img.get(col,row);
fill(color(c));
noStroke();
rect((col + 4.5), (row + 4.5), 3, 3);
ellipse((col + 8.5) + dx , (row - 4) + dy, 2, 2);
ellipse((col + 8.5) + dx , (row - 6) + dy, 1.5, 1.5);
}
}
for(var col = 128; col < 186; col += 4){ //cont.
for(var row = 216; row < 296 ; row += 4){
var c = img.get(col,row);
fill(color(c));
noStroke();
rect((col + 4.5), (row + 4.5), 3, 3);
ellipse((col + 8.5) + dx ,(row - 4) + dy, 2, 2);
ellipse((col + 8.5) + dx , (row - 6) + dy, 1.5, 1.5);
}
}
}
function eyes(){
for(var col = 104; col < 136; col += 2){ //eye area 1: no circle, too small
for(var row = 184; row < 216 ; row += 2){
var c = img.get(col,row);
fill(color(c));
noStroke();
rect((col + 6.5), (row + 6.5), 1, 1);
}
}
for(var col = 176; col < 232; col += 2){ //eye area 2: no circle, too small
for(var row = 184; row < 216 ; row += 2){
var c = img.get(col, row);
fill(color(c));
noStroke();
rect((col + 6.5), (row + 6.5), 1, 1);
}
}
}
function draw() {
background(0);
var offset = noise(noiseParam);
offset = map(offset, 0, 1, -8, 8);
dx = offset;
dy = offset;
main(dx, dy);
face(dx, dy);
mainFeature(dx, dy);
eyes(dx, dy);
noiseParam += noiseStep;
}