var compportraitImg;
function preload() {
ImageURL = "https://i.imgur.com/ZTxOcmt.jpg";
compportraitImg = loadImage(ImageURL);
}
function setup() {
// canvas proportional to image size
createCanvas(400, 480);
compportraitImg.loadPixels();
background(220);
frameRate(300);
}
function draw() {
//have the correct color show at right location
var kx = floor(random(compportraitImg.width));
var ky = floor(random(compportraitImg.height));
var colorLoc = compportraitImg.get(kx, ky);
noStroke();
fill(colorLoc);
//scale to size of canvas
x = map(kx, 0, compportraitImg.width, 0, width);
y = map(ky, 0, compportraitImg.height, 0, height);
var j = dist(width/2, height/2, x, y);
j = j % 20;
bubble(x, y, j, 4);
}
function bubble(x, y, radius, npoints) {
angle = PI / npoints;
beginShape();
for (let a = 0; a < PI; a += angle) {
sx = x + cos(a) * radius;
sy = y + sin(a) * radius;
vertex(x + cos(a) * radius, y + sin(a) * radius);
}
endShape();
}
I made my “custom pixel” in the shape of half of a hexagon and made it such that they populate the canvas in a circle pattern and leave some of the background peeking through.
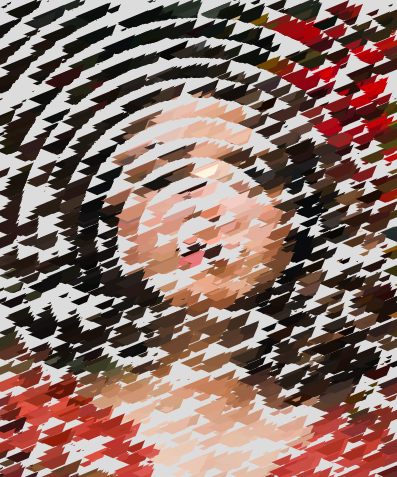
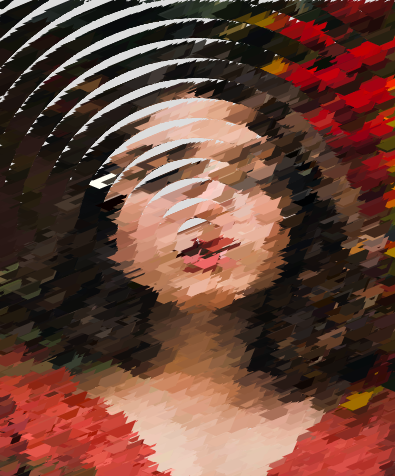