sketchDownload
/*Name:Camellia(Siyun) Wang;
Section: C;
Email Address: siyunw@andrew.cmu.edu;*/
//https://imgur.com/DBFXrXh coral2
//https://imgur.com/nyf5GKY coral1
//https://imgur.com/JXohvV2 group
//https://imgur.com/4hO2HW1 weed
//https://imgur.com/N4zWoA4
//https://imgur.com/J8R7qyD beach
var beaches = [];
var corals1 = [];
var corals1n = [];
var corals2 = [];
var corals2n = [];
var corals2b = [];
var groups = [];
var weeds = [];
var weeds2 = [];
var stars = [];
var fishes = [];
var bubbles = [];
var a;
var b;
var c;
var d;
var a1;
var b1;
var c1;
var d1;
function preload(){
backgroundImage = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/back.jpeg");
coral1 = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/coral1.png");
coral2 = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/coral-2.png");
group = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/group.png");
weed = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/weeds.png");
beach = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/beach.png");
star = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/starfish.png");
fish = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/fish.png");
fishschool = loadImage("https://courses.ideate.cmu.edu/15-104/f2021/wp-content/uploads/2021/11/fish-school.png");
}
function setup() {
a = random(-150,300);
b = random(-150,300);
c = random(-150,300);
d = random(-150,300);
a1 = random(1,-30);
b1 = random(1,-30);
c1 = random(1,-30);
d1 = random(1,-30);
createCanvas(480, 400);
//the beach
var bea = makeBeach(1,400);
beaches.push(bea);
//coral1
var cor1 = makeCoral1(150,200);
corals1.push(cor1);
//coral1n
var cor1n = makeCoral1n(1000,175);
corals1n.push(cor1n);
//coral2
var cor2 = makeCoral2(450,75);
corals2.push(cor2);
//coral2n
var cor2n = makeCoral2n(1300,230);
corals2n.push(cor2n);
//coral2b
var cor2b = makeCoral2b(1330,190);
corals2b.push(cor2b);
//group
var gro = makeGroup(550,140);
groups.push(gro);
//weed
var wee = makeWeed(220,55);
weeds.push(wee);
//weed2
var wee2 = makeWeed2(850,30);
weeds2.push(wee2);
var star = makeStar(1200,325);
stars.push(star);
var fish = makeFish(1220,25);
fishes.push(fish);
var bubble = makeBubble(185,480);
bubbles.push(bubble);
frameRate(30);
}
//the beach
function makeBeach(xb,yb) {
var B = {
x: xb,
y: yb,
move: beachMove,
speed: -1.5,
display: beachDisplay};
return B;
}
function beachMove() {
this.x += this.speed;
}
function beachDisplay(xb) {
image(beach, this.x, 0,1400,400);
}
function updateAndDisplayBeach(){
for(var i = 0; i < beaches.length; i++){
beaches[i].move();
beaches[i].display();
}
}
function removeBeachThatHaveSlippedOutOfView(){
for (var i = 0; i < beaches.length; i++){
if (beaches[i].x < -1440) {
beaches.shift(beaches[i]);
}
}
}
function addNewBeach(){
var lastBeaches = beaches[beaches.length - 1];
if(lastBeaches.x < -900){
var a = makeBeach(480,400);
beaches.push(a);
}
}
//coral1
function makeCoral1(xc1,yc1){
var C1 = {
x: xc1,
y: yc1,
move: coral1Move,
speed: -1.5,
display: coral1Display};
return C1;
}
function coral1Move(){
this.x +=this.speed;
}
function coral1Display(xc1,yc1){
image(coral1, this.x, this.y,200,200);
}
function updateAndDisplayCoral1(){
for(var i = 0; i < corals1.length; i++){
corals1[i].move();
corals1[i].display();
}
}
function removeCoral1ThatHaveSlippedOutOfView(){
for (var i = 0; i < corals1.length; i++){
if (corals1[i].x < -1440) {
corals1.shift(corals1[i]);
}
}
}
function addNewCoral1(){
var lastCoral1 = corals1[corals1.length - 1];
if(lastCoral1.x < -900){
var a = makeCoral1(480,200);
corals1.push(a);
}
}
//coral1n
function makeCoral1n(xc1n,yc1n){
var C1n = {
x: xc1n,
y: yc1n,
move: coral1nMove,
speed: -1.5,
display: coral1nDisplay};
return C1n;
}
function coral1nMove(){
this.x +=this.speed;
}
function coral1nDisplay(xc1,yc1){
image(coral1, this.x, this.y,200,200);
}
function updateAndDisplayCoral1n(){
for(var i = 0; i < corals1n.length; i++){
corals1n[i].move();
corals1n[i].display();
}
}
function removeCoral1nThatHaveSlippedOutOfView(){
for (var i = 0; i < corals1n.length; i++){
if (corals1n[i].x < -1440) {
corals1n.shift(corals1n[i]);
}
}
}
function addNewCoral1n(){
var lastCoral1n = corals1n[corals1n.length - 1];
if(lastCoral1n.x < -900){
var a = makeCoral1n(480,175);
corals1n.push(a);
}
}
//coral2
function makeCoral2(xc2,yc2){
var C2 = {
x: xc2,
y: yc2,
move: coral2Move,
speed: -1.5,
display: coral2Display};
return C2;
}
function coral2Move(){
this.x +=this.speed;
}
function coral2Display(xc2,yc2){
image(coral2, this.x, this.y,200,200);
}
function updateAndDisplayCoral2(){
for(var i = 0; i < corals2.length; i++){
corals2[i].move();
corals2[i].display();
}
}
function removeCoral2ThatHaveSlippedOutOfView(){
for (var i = 0; i < corals2.length; i++){
if (corals2[i].x < -1440) {
corals2.shift(corals2[i]);
}
}
}
function addNewCoral2(){
var lastCoral2 = corals2[corals2.length - 1];
if(lastCoral2.x < -900){
var a = makeCoral2(480,75);
corals2.push(a);
}
}
//coral2n
function makeCoral2n(xc2n,yc2n){
var C2n = {
x: xc2n,
y: yc2n,
move: coral2nMove,
speed: -1.5,
display: coral2nDisplay};
return C2n;
}
function coral2nMove(){
this.x +=this.speed;
}
function coral2nDisplay(xc2n,yc2n){
image(coral2, this.x, this.y,200,200);
}
function updateAndDisplayCoral2n(){
for(var i = 0; i < corals2n.length; i++){
corals2n[i].move();
corals2n[i].display();
}
}
function removeCoral2nThatHaveSlippedOutOfView(){
for (var i = 0; i < corals2n.length; i++){
if (corals2n[i].x < -1440) {
corals2n.shift(corals2n[i]);
}
}
}
function addNewCoral2n(){
var lastCoral2n = corals2n[corals2n.length - 1];
if(lastCoral2n.x < -900){
var a = makeCoral2n(480,230);
corals2n.push(a);
}
}
//coral2b
function makeCoral2b(xc2b,yc2b){
var C2b = {
x: xc2b,
y: yc2b,
move: coral2bMove,
speed: -1.5,
display: coral2bDisplay};
return C2b;
}
function coral2bMove(){
this.x +=this.speed;
}
function coral2bDisplay(xc2b,yc2b){
image(coral2, this.x, this.y,220,220);
}
function updateAndDisplayCoral2b(){
for(var i = 0; i < corals2b.length; i++){
corals2b[i].move();
corals2b[i].display();
}
}
function removeCoral2bThatHaveSlippedOutOfView(){
for (var i = 0; i < corals2b.length; i++){
if (corals2b[i].x < -1440) {
corals2b.shift(corals2b[i]);
}
}
}
function addNewCoral2b(){
var lastCoral2b = corals2b[corals2b.length - 1];
if(lastCoral2b.x < -900){
var a = makeCoral2b(480,190);
corals2b.push(a);
}
}
//group
function makeGroup(xg,yg){
var G = {
x: xg,
y: yg,
move: groupMove,
speed: -1.5,
display: groupDisplay};
return G;
}
function groupMove(){
this.x +=this.speed;
}
function groupDisplay(xg,yg){
image(group, this.x, this.y,350,350);
}
function updateAndDisplayGroup(){
for(var i = 0; i < groups.length; i++){
groups[i].move();
groups[i].display();
}
}
function removeGroupThatHaveSlippedOutOfView(){
for (var i = 0; i < groups.length; i++){
if (groups[i].x < -1440) {
groups.shift(groups[i]);
}
}
}
function addNewGroup(){
var lastGroup = groups[groups.length - 1];
if(lastGroup.x < -900){
var a = makeGroup(480,140);
groups.push(a);
}
}
//weed
function makeWeed(xw,yw){
var W = {
x: xw,
y: yw,
move: weedMove,
speed: -1.5,
display: weedDisplay};
return W;
}
function weedMove(){
this.x +=this.speed;
}
function weedDisplay(xw,yw){
image(weed, this.x, this.y,300,300);
}
function updateAndDisplayWeed(){
for(var i = 0; i < weeds.length; i++){
weeds[i].move();
weeds[i].display();
}
}
function removeWeedThatHaveSlippedOutOfView(){
for (var i = 0; i < weeds.length; i++){
if (weeds[i].x < -1440) {
weeds.shift(weeds[i]);
}
}
}
function addNewWeed(){
var lastWeed = weeds[weeds.length - 1];
if(lastWeed.x < -900){
var a = makeWeed(480,55);
weeds.push(a);
}
}
//weed2
function makeWeed2(xw2,yw2){
var W2= {
x: xw2,
y: yw2,
move: weed2Move,
speed: -1.5,
display: weed2Display};
return W2;
}
function weed2Move(){
this.x +=this.speed;
}
function weed2Display(xw2,yw2){
image(weed, this.x, this.y,300,300);
}
function updateAndDisplayWeed2(){
for(var i = 0; i < weeds2.length; i++){
weeds2[i].move();
weeds2[i].display();
}
}
function removeWeed2ThatHaveSlippedOutOfView(){
for (var i = 0; i < weeds2.length; i++){
if (weeds2[i].x < -1440) {
weeds2.shift(weeds2[i]);
}
}
}
function addNewWeed2(){
var lastWeed2 = weeds2[weeds2.length - 1];
if(lastWeed2.x < -900){
var a = makeWeed2(480,30);
weeds2.push(a);
}
}
//stars
function makeStar(xs,ys){
var S = {
x: xs,
y: ys,
move: starMove,
speed: -1.5,
display: starDisplay};
return S;
}
function starMove(){
this.x +=this.speed;
}
function starDisplay(xs,ys){
image(star, this.x, this.y,75,75);
}
function updateAndDisplayStar(){
for(var i = 0; i < stars.length; i++){
stars[i].move();
stars[i].display();
}
}
function removeStarThatHaveSlippedOutOfView(){
for (var i = 0; i < stars.length; i++){
if (stars[i].x < -1440) {
stars.shift(stars[i]);
}
}
}
function addNewStar(){
var lastStar = stars[stars.length - 1];
if(lastStar.x < -900){
var a = makeStar(480,325);
stars.push(a);
}
}
//fish school
function makeFish(xf,yf){
var F = {
x: xf,
y: yf,
move: fishMove,
speed: -3.0,
display: fishDisplay};
return F;
}
function fishMove(){
this.x +=this.speed;
}
function fishDisplay(xf,yf){
image(fishschool, this.x, this.y,200,200);
}
function updateAndDisplayFish(){
for(var i = 0; i < fishes.length; i++){
fishes[i].move();
fishes[i].display();
}
}
function removeFishThatHaveSlippedOutOfView(){
for (var i = 0; i < fishes.length; i++){
if (fishes[i].x < -1440) {
fishes.shift(fishes[i]);
}
}
}
function addNewFishRandomly(){
var newFishLikelihood = 0.002;
if (random(0,2) < newFishLikelihood) {
fishes.push(makeFish(480,25));
}
}
//bubbles
function makeBubble(xb,yb){
var B = {
x: xb,
y: yb,
yspeed: -3,
move: bubbleMove,
speed: -1.5,
display: bubbleDisplay};
return B;
}
function bubbleMove(){
this.x +=this.speed;
this.y +=this.yspeed;
}
function bubbleDisplay(xb,yb){
noStroke();
fill(255);
ellipse(this.x,this.y,10,10);
ellipse(this.x+a,this.y+a1,20,20);
ellipse(this.x+b,this.y+b1,17,17);
ellipse(this.x+c,this.y+c1,23,23);
ellipse(this.x+d,this.y+d1,15,15);
}
function updateAndDisplayBubble(){
for(var i = 0; i < bubbles.length; i++){
bubbles[i].move();
bubbles[i].display();
}
}
function removeBubbleThatHaveSlippedOutOfView(){
for (var i = 0; i < bubbles.length; i++){
if (bubbles[i].x < -1440) {
bubbles.shift(bubbles[i]);
}
}
}
function addNewBubbleRandomly(){
var newBubbleLikelihood = 0.02;
if (random(0,2) < newBubbleLikelihood) {
bubbles.push(makeBubble(185,480));
}
}
function draw() {
background(backgroundImage);
updateAndDisplayBubble();
addNewBubbleRandomly();
removeBubbleThatHaveSlippedOutOfView()
updateAndDisplayWeed2();
addNewWeed2();
removeWeed2ThatHaveSlippedOutOfView();
updateAndDisplayBeach();
addNewBeach();
removeBeachThatHaveSlippedOutOfView();
updateAndDisplayWeed();
addNewWeed();
removeWeedThatHaveSlippedOutOfView();
updateAndDisplayCoral2();
addNewCoral2();
removeCoral2ThatHaveSlippedOutOfView();
updateAndDisplayFish();
addNewFishRandomly();
removeFishThatHaveSlippedOutOfView();
updateAndDisplayCoral2b();
addNewCoral2b();
removeCoral2bThatHaveSlippedOutOfView();
updateAndDisplayCoral2n();
addNewCoral2n();
removeCoral2nThatHaveSlippedOutOfView();
updateAndDisplayGroup();
addNewGroup();
removeGroupThatHaveSlippedOutOfView();
image(fish,140,100,200,230);
updateAndDisplayCoral1();
addNewCoral1();
removeCoral1ThatHaveSlippedOutOfView();
updateAndDisplayCoral1n();
addNewCoral1n();
removeCoral1nThatHaveSlippedOutOfView();
updateAndDisplayStar();
addNewStar();
removeStarThatHaveSlippedOutOfView();
}
I find the code of this project not too complicated but rather a bit complex, because I need to create many objects to draw on the canvas. Also, the most difficult part is the first object. After successfully make the first object work, the others are much the same with subtle differences. The landscape I created is an under ocean landscape, narrating a fish’s journey wandering around.
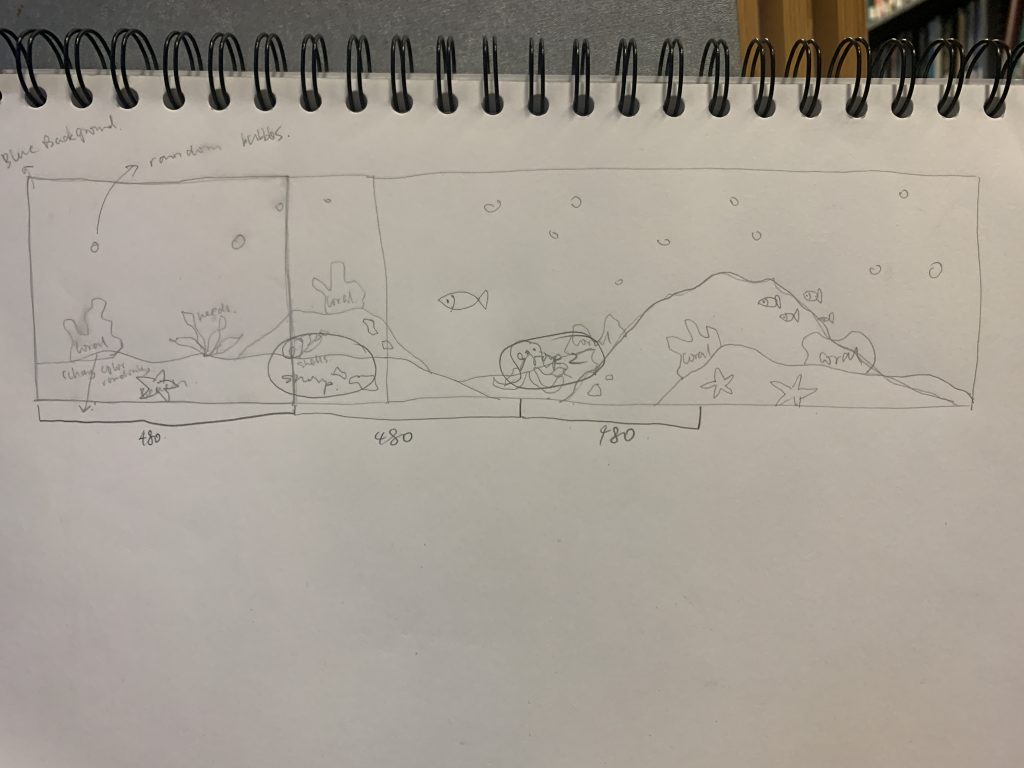