The project I choose for this week LO is Karlheinz Stockhauen’s KLAVIERSTÜCK XI (Piano Piece 11, 1956). The way he constructed his whole score utilizes randomness. Basically, he had 19 musical pieces spread on the floor, and he will choose one piece at a time from the floor. However, when he picked the same piece for the third time, he completed the score and stopped. By composing in this way, the song can start and end with any piece among the 19 of them, therefore creating random variations and changes. The thing interesting about this is the unpredictability of the score.
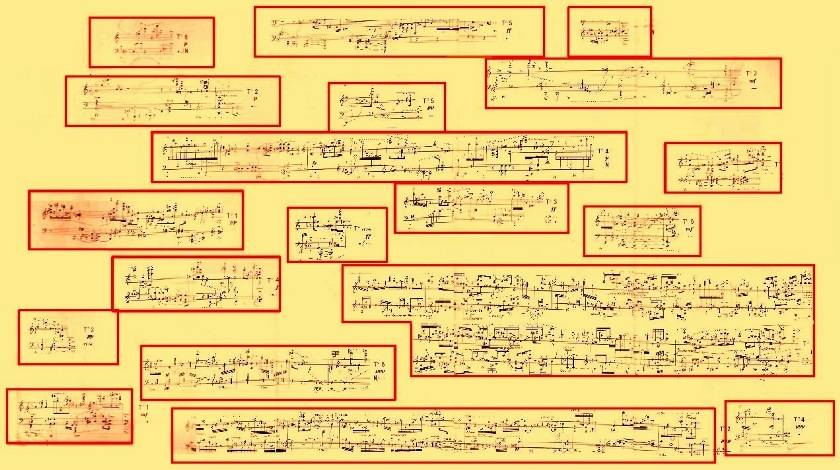