function setup() {
wd = 600
ht = 600
createCanvas(wd, ht);
background(60,80,140);
}
function draw() {
background(60,80,140)
s = second();
m = minute();
h = hour();
noStroke();
xPos = 10*s
yPos = 10*s
//the minute bar
fill(238,229,169)
rect(0, 29, wd, 79);
rect(0, ht-109, wd, 79);
fill(105,160,225)
rect(0, 49, wd*(m/60), 60)
rect(0, ht-100, wd*(m/60), 60)
//Hour Circle
fill(255);
circle(wd/2, ht/2, 199)
fill(255,81,13)
arc(wd/2, ht/2, 199, 199, -PI/2, -PI/2+h*(2*PI/12));
//Seconds cirlcles
fill(230,120,12)
if (s <= 30) {
circle(xPos, ht/2, 60);
} else {
circle(wd/2, yPos, 60);
}
if (s <= 30) {
circle(wd-xPos, ht/2, 60);
} else {
circle(wd/2, ht-yPos, 60);
}
}
Category: SectionB
LO #6
randomness
Zach Lieberman is a media artist who creates art with code. He uses Openframeworks for most of his creation, which is constantly updated on his instagram. In his recent works, he explored using a variety of random colors along with the wave effect and named the piece :color meditation. The slowly delayed motion of the colors moving towards the viewers’ perspective as it becomes thinner as it approaches the viewers’ eye, somehow gives the sense of relaxation. Another piece that he created recently is called “Grid push color”. I was intrigued by the combinations of random color he uses, and even though they are all unintentionally selected, they blend together very well. This piece consists of gradient squares which continuously changes color. This way, he successfully illustrates the movement of colors during the transitions of squares. This piece made me reach back my childhood memory of playing with a crystal prism.
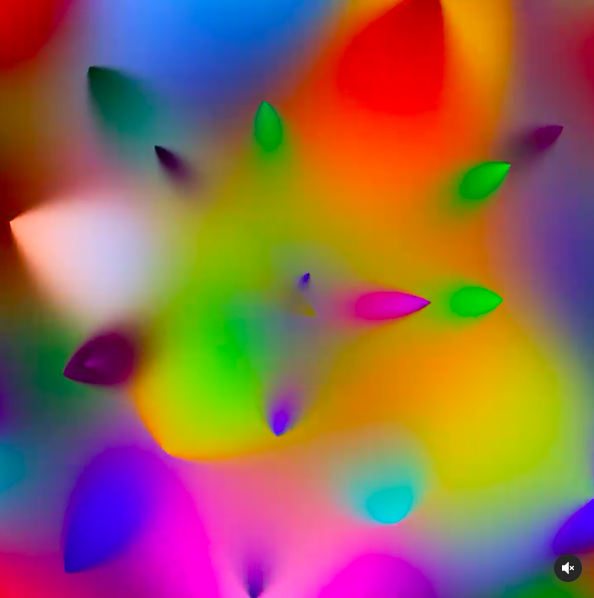
https://www.instagram.com/zach.lieberman/ (more works)
Project 06 – Abstract Clock
var x = [];
var y = [];
var sec; // second
var minutes; // minute
var hr; // hour
// every second, coffee fills up a little bit in a cup and coffee mug gets larger
// every minute, coffee completely fills up a cup
// every hour, the background color darkens
function setup() {
createCanvas(450, 300);
}
// each cup of coffee represents one minute
function draw() {
var hr = hour();
background(255-(hr*5), 255-(hr*5), 255-(hr*3)); // background color gets darker by the hour
minutes = minute();
sec = second();
for(var row = 0; row < 6; row +=1 ) {
for(var col = 0; col < 10; col += 1) {
fill(250, 247, 222); // cream color for mug
var current = row*10+col; // current mug
mug(40+(40*col), 43+(40*row), current); // draw the mug
}
}
}
function mug(x, y, cur) { // function to draw the mug
// body of the mug
var frac = 1;
if (cur < minutes) {
fill(117, 72, 50); // brown
} else if (cur == minutes) {
frac = sec/60;
fill(250+(117-250)*frac, 247+(72-247)*frac, 222+(50-222)*frac); // cream -> brown
} else {
return;
}
push();
translate(x, y);
scale(frac); // every second, the mug gets bigger
ellipse(0, 0, 30, 7); // top of mug
beginShape();
curveVertex(-15, 0);
curveVertex(-15, 0);
curveVertex(-14, 10);
curveVertex(-10, 25);
curveVertex(-5, 28);
curveVertex(0, 28);
curveVertex(5, 28);
curveVertex(10, 25);
curveVertex(14, 10);
curveVertex(15, 0);
curveVertex(15, 0);
endShape();
// mug handle
noFill();
strokeWeight(1.5);
beginShape();
curveVertex(12, 20);
curveVertex(12, 20);
curveVertex(18, 13);
curveVertex(15, 5);
curveVertex(14, 8);
curveVertex(14, 8);
endShape();
strokeWeight(1);
pop();
}
The idea behind this project stemmed from my love for coffee. My time is basically measured by cups of coffee, which inspired me to create a canvas full of coffee mugs. The hours of each day are represented by the darkening of the background. The minutes of each hour are represented by the sixty mugs. The seconds of each minute are represented by the size and color of the mugs (filling up the mugs).
My initial sketch:
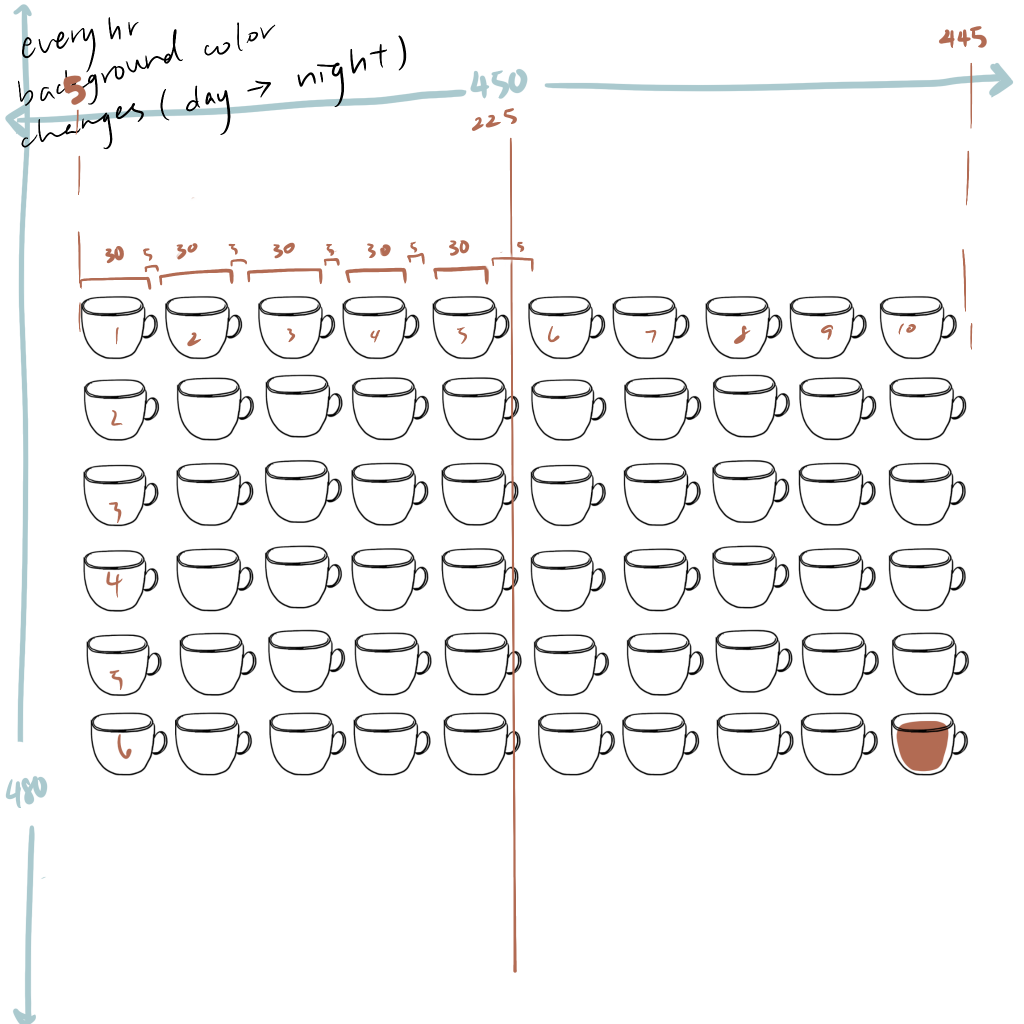
I found this project quite challenging. I realized that executing a concept in code can be a lot more difficult than expected.
Looking Outwards-06
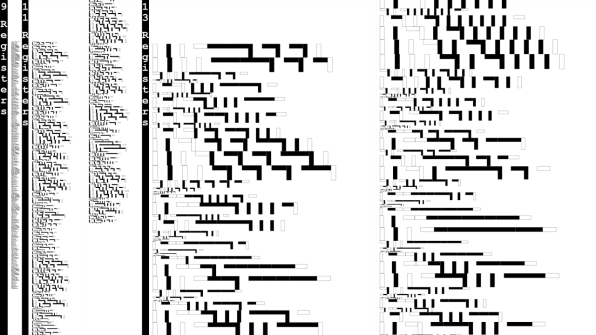
In looking at the application of randomness in generative art this week, I looked at Rami Hammour’s work on A Text of Random Meaning. It’s a visualization mapping out 18 columns of a “Register and Taps” random number generator in action. I found it interesting the way the final visualization simulates the look of long columns of text, but once viewers zoom in they find that the randomly generated lines are not actually representative of anything. I found the irony in this interaction very interesting. However, one of the interesting points I came across in my research was that technically in it’s simulated nature, there is no such thing as true randomness. In descriptions of Rami Hammour’s project, it is described as a “systematic cycle for producing randomness”.
Looking Outwards 06: Randomness
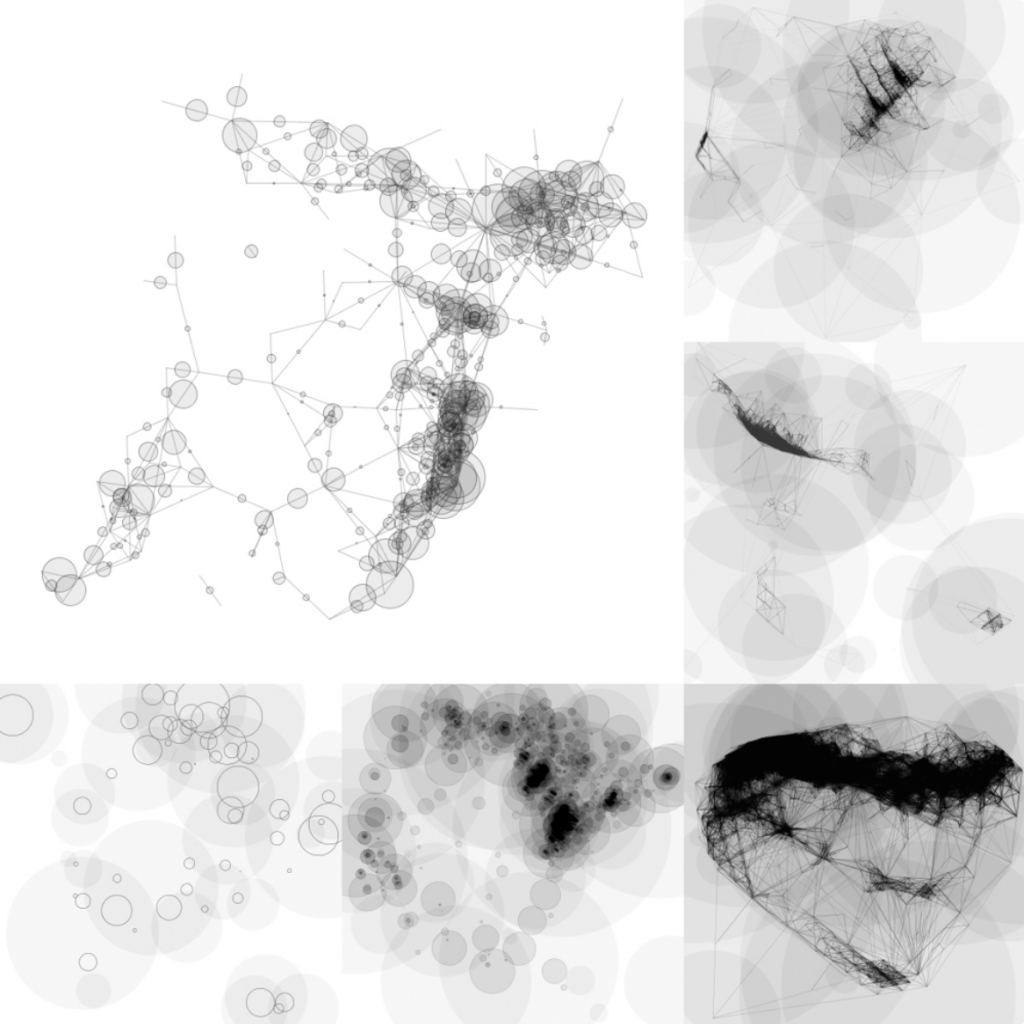
by Jesal Mehta
The project that I looked at for this week’s LO is “Emergence Algorithms in Processing” by Jesal Mehta in 2018. Even though this is considered more of Mehta’s exploration and design documentation, I still really enjoy seeing how he showed the algorithm slowly building up the piece and the contrast between the start and the end result. The circles in the piece are generated at random points of the canvas with random diameters. New circles are then drawn wherever two circles overlap and with new diameters that allow them to touch the two parent circles. The generation continues on, ultimately creating a full piece and pattern. I find it fascinating to see how a few simple circles can evolve into such a complicated piece. This also relates a lot to what we’ve already learned in class, and it makes me wonder how I could also explore randomness as Mehta did. The only controlled variable is the initial diameters, and the rest simply relies on the code and just pure randomness.
LO – 5
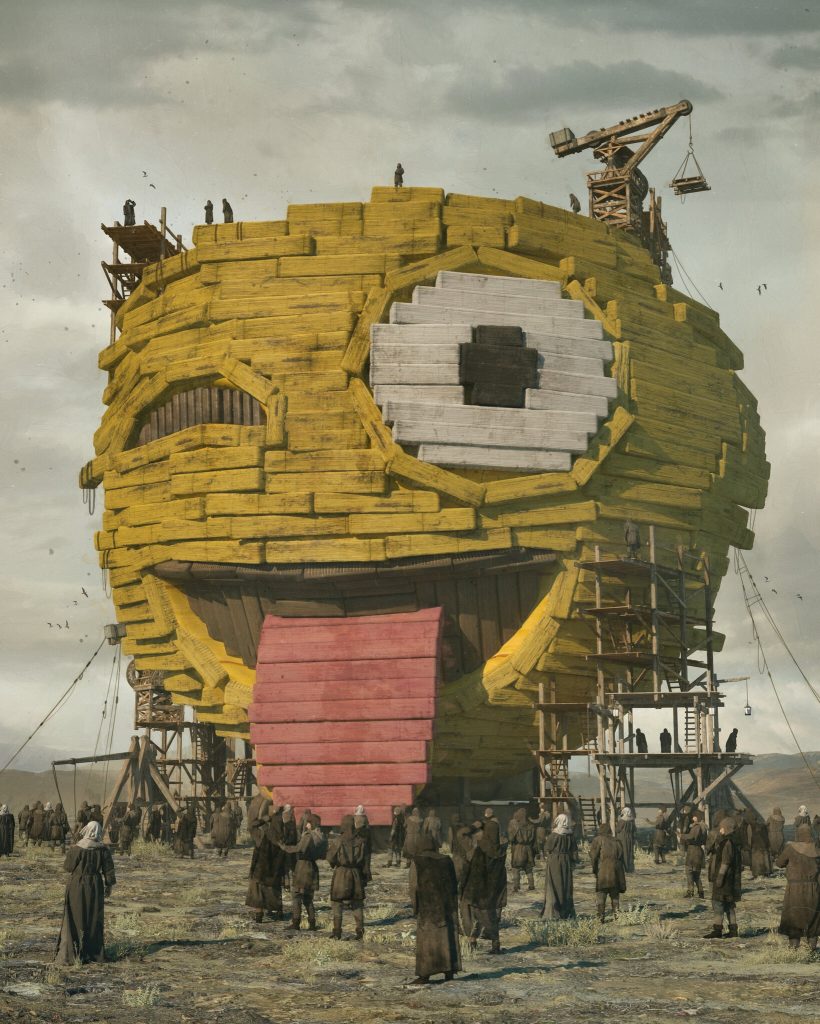
Within 3D computer graphics there are many different applications and possible uses for the various modeling programs that are used to create them. One person who works in 3D computer graphics is Mike Winkelmann, also known as “beeple”. Beeple is a digital artist who works with many different programs to create 3D images as well as drawing 3D images himself. He has been a digital artist for over 20 years and has done projects like “Everydays” since. Specifically, these “everydays” are digital art pieces in which he forces himself to create a new one everyday. I admire these projects because they’re all so different and oftentimes very bizarre. The algorithms that he uses are in programs like ‘Blender’ or ‘Toon Boom’. These programs are so powerful and allow him to model very precise things and produce high quality renderings at the end. He’s a very unique and outgoing artist and these sensibilities really manifest themselves in the crazy ideas that he creates. This specific image is one of those everydays that I like most.
Link: https://www.nytimes.com/2021/02/24/arts/design/christies-beeple-nft.html
Project 5 – Wallpaper
This week’s project asked us to make a wallpaper, so I decided to make my work based on african textiles. Attached is a sketch of my initial idea and my final product. Enjoy!
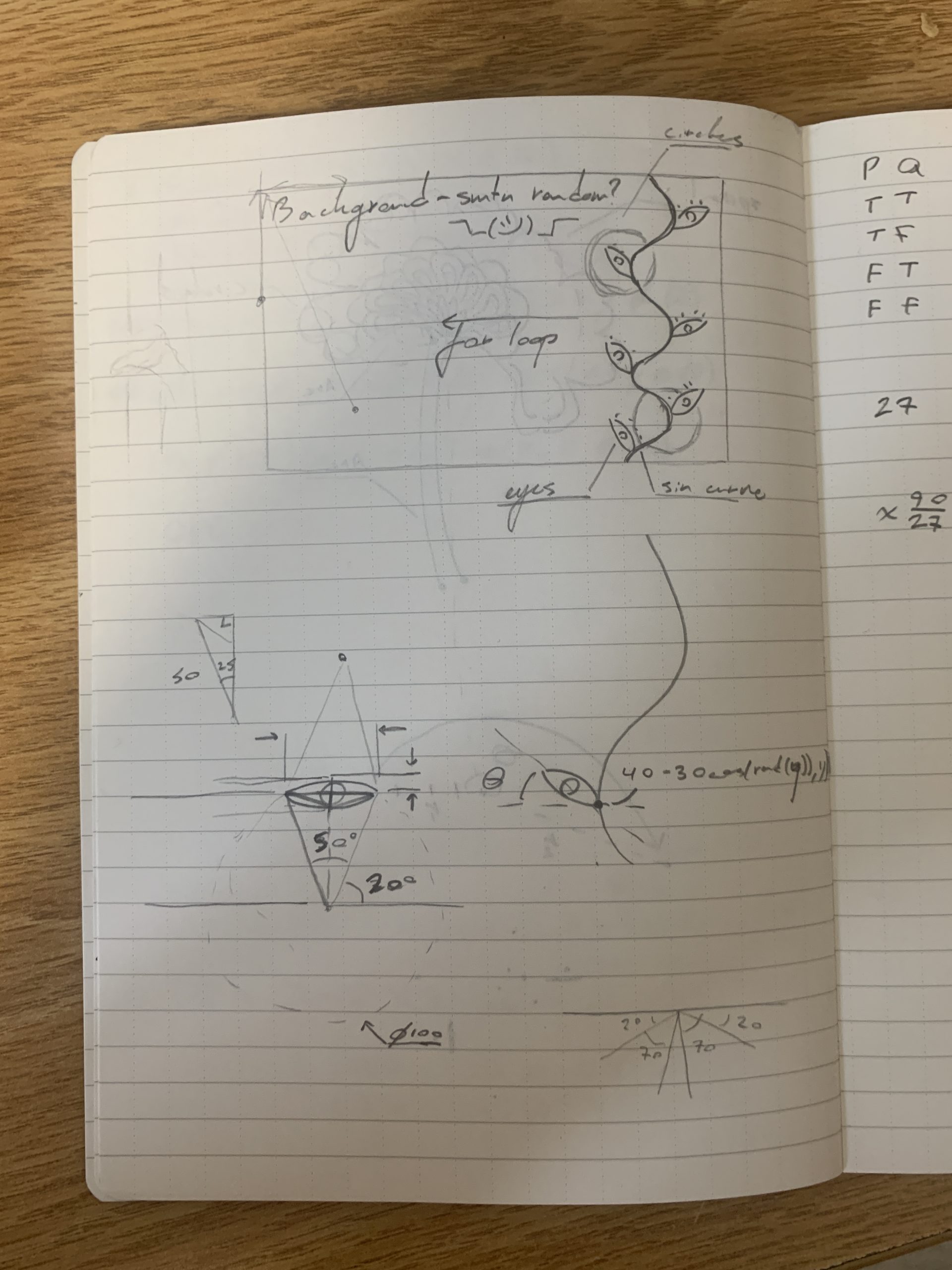
var num = 6; //number of itterations of everything
function setup() {
createCanvas(600, 400);
background(250, 249, 246);
}
/*let eyeBrown = color(165,42,42);
let bgBrown = color(255, 200, 168);
let branchGreen = color(63, 146, 65);
let turq = color(64,224,208);*/
function drawBackground() {
//random lines from ex 4 but in for loop
stroke(255, 200, 168);
strokeWeight(1);
for (var y = 0; y < height; y+=20) {
for (var x = 0; x < width; x+=20) {
if (random(1) > 0.5) {
line(x, y, x+20, y+20);
}
else {
line(x, y+20, x+20, y);
}
}
}
//ornaments
for (var j = 0; j <= width; j+=width/num) {
var colors = [color(145,205,211), color(234,204,84)]
noStroke();
fill(random(colors));
circle(random(j, j+(width/num)), random(height/2), random(40,60));
circle(random(j, j+(width/num)), random(height/2,height), random(40,60));
push();
fill(200,74,55);
translate(random(j,j+(width/num)), random(height));
for (var r = 10; r < 60; r+=10) {
for (var i = 0; i < 360; i+=30) {
push();
scale(0.3);
circle(r*cos(radians(i)), r*sin(radians(i)), r/3);
pop();
}
}
pop();
}
}
function drawEye(a, b, side) {
push();
translate(a, b);
//alternates side of branch
if (side == 1) {
rotate(degrees(-.009));
} else if (side == -1) {
rotate(degrees(.009));
}
//eye arcs
//blue filling
push();
scale(0.7);
noFill();
strokeWeight(10);
stroke(52,173,193);
arc(0, -45, 100, 100, radians(65), radians(180-65));
push();
rotate(radians(180));
arc(0, -45, 100, 100, radians(65), radians(180-65));
pop();
pop();
//green arcs
strokeWeight(3);
noFill();
stroke(50, 118, 112);
arc(0, -45, 100, 100, radians(65), radians(180-65));
push();
rotate(radians(180));
arc(0, -45, 100, 100, radians(65), radians(180-65));
pop();
//gold arcs
push();
scale(0.9);
noFill();
strokeWeight(2);
stroke(234,178,57);
arc(0, -45, 100, 100, radians(65), radians(180-65));
push();
rotate(radians(180));
arc(0, -45, 100, 100, radians(65), radians(180-65));
pop();
pop();
fill(165,42,42);
noStroke();
circle(0, 0, 10); //eyeball
//lashes
strokeWeight(1);
push();
translate(0, 45);
rotate(radians(-35));
stroke(0);
for (var i = 0; i <= 50; i+=10) {
rotate(radians(10));
line(0, -52, 0, -54);
}
pop();
pop();
}
function drawBranches() {
stroke(63, 146, 65);
for (var x = 0; x <= width; x+= width/num) {
push();
translate(x, random(-50,50));
var side = 1;
for (var y = -100; y < height+100; y += 1) {
//draw alternating eyes
if ((y%50) == 0 & side == 1) {
drawEye(cosy+19,y-10,side);
side = -1*side;
} else if ((y%50) == 0 & side == -1){
drawEye(cosy-19,y-10,side);
side = -1*side;
}
//draw branches (vertical cosine curves)
var cosy = 40-30 * cos(radians(y));
stroke(50, 118, 112);
strokeWeight(5);
point(cosy, y);
}
pop();
}
}
var count = 0;
function draw() {
drawBackground();
drawBranches();
noLoop();
}
Looking Outwards 5
For this week’s looking outwards blog, I looked at Andy Lomas’ Morphogenetic Creations. Presented at the Watermans Art Centre, is meant to illustrate and explore how “intricate complex structures, such as those found in nature, can be created emergently through computational simulation of growth processes.” The installation includes prints, projections and videos, and stereoscopic work. Lomas uses randomization in his algorithms in order to make his creations more complex than anything he could hard code. This also helps illustrate how small changes in the algorithm can lead to massively different outcomes.
Project 5: Wallpaper
During my process of making this wallpaper, I got inspiration from the Studio Ghibli film Spirited Away. The ducklings taking a bath with a towel on top. I wanted to represent the rolled-up towel on the duck’s head on some, and the rolled-up towel loosened on the head on others which created a pattern.
Looking Outwards 05: 3D Computer Graphics
I admire the project named Supermassive Blackhole by Nika Maisuradze because of the way they created the feeling of 3D even though it is just a 2D image. The details in this piece to create the illusion of a black hole actually being there are really well done. The color schemes incorporated into this piece are also put together nicely to make it seem surreal and 3D. The highlights in some parts creating emphasis on things help create movement within the piece as well. I know the Nika used the software called Blender and Blackmagic Design Fusion. The feeling of a black hole that Nika wanted is really shown with the way the atmosphere and the background are transparent in some places and just opaquely black in others.