The project I have chosen for this blog post is Pamela Z’s 2012 work “Fount”. “Fount” is a sound and visual art installation piece using projection and speakers (or a sound dome). The images displayed and sound projected are triggered by the presence of a viewer. With no viewer present, the image is blurred and no sound comes out of the speakers. Once a person is in the space, the image clears to reveal a beaker pouring water into a basin; the speakers play an audio of water pouring which accompanies the video. Although the piece itself is not generated by a computer algorithm, the intended performance of the piece involves a computer system sensing the presence of a viewer and setting the correct programs into motion. I think this piece of art is interesting because it requires interaction with a viewer, although the viewer only needs to be present. This sort of semi-interactive concept is intriguing from the perspective of an audience member like the piece is telling a secret to one viewer at a time.
Category: Uncategorized
Project-04: String Art
// coordinated for basic star shape:
//lineA:
var lineAx1 = 0
var lineAy1 = 0
var lineAx2 = 0
var lineAy2 = -125
//lineB:
var lineBx1 = 119
var lineBy1 = -39
var lineBx2 = 0
var lineBy2 = 0
//lineC:
var lineCx1 = 0
var lineCy1 = 0
var lineCx2 = 73
var lineCy2 = 101
//lineD:
var lineDx1 = -73
var lineDy1 = 101
var lineDx2 = 0
var lineDy2 = 0
//lineE:
var lineEx1 = 0
var lineEy1 = 0
var lineEx2 = -119
var lineEy2 = -39
//distance for string art end points variables initialized:
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 40; //number of string art lines in each 'shape'
var rot = 0 //rotation effect (DISABLED bc of noLoop() call; comment out noLoop() to see rotation)
// color variables for shading effect:
var r = 0;
var g = 50;
var b = 100;
function setup() {
createCanvas(400, 300);
background(200);
}
function draw() {
background(200); // called for rotation effect
translate(width/2, height/2); // center canvas
//rotation effect (DISABLED while noLoop() is activated):
rotate(radians(rot));
rot += 1;
// color variables for shade change effect re-defined after each rotation:
r = 0;
g = 50;
b = 100;
//lines to create basic star shape, coordinates set by variables:
line(lineAx1, lineAy1, lineAx2, lineAy2);
line(lineBx1, lineBy1, lineBx2, lineBy2);
line(lineCx1, lineCy1, lineCx2, lineCy2);
line(lineDx1, lineDy1, lineDx2, lineDy2);
line(lineEx1, lineEy1, lineEx2, lineEy2);
// drawLines() called to create the string art effect:
drawLines(lineAx1, lineAy1, lineAx2, lineAy2, lineBx1, lineBy1, lineBx2, lineBy2);
drawLines(lineBx1, lineBy1, lineBx2, lineBy2, lineCx1, lineCy1, lineCx2, lineCy2);
drawLines(lineCx1, lineCy1, lineCx2, lineCy2, lineDx1, lineDy1, lineDx2, lineDy2);
drawLines(lineDx1, lineDy1, lineDx2, lineDy2, lineEx1, lineEy1, lineEx2, lineEy2);
drawLines(lineEx2, lineEy2, lineEx1, lineEy1, lineAx1, lineAy1, lineAx2, lineAy2);
// comment noLoop() out to see roration:
noLoop();
}
//drawLines function made for string art effect, takes x and y coordinates of both connecting lines (8 parameters):
function drawLines(line1x1, line1y1, line1x2, line1y2, line2x1, line2y1, line2x2, line2y2) {
// set distance between end points for string lines:
dx1 = (line1x2-line1x1)/numLines;
dy1 = (line1y2-line1y1)/numLines;
dx2 = (line2x2-line2x1)/numLines;
dy2 = (line2y2-line2y1)/numLines;
//initialize variables for string line end points:
var x1 = line1x1;
var y1 = line1y1;
var x2 = line2x1;
var y2 = line2y1;
// string lines drawn until specified number of lines is hit:
for (var i = 0; i <= numLines; i += 1) {
stroke(r, g, b);
line(x1, y1, x2, y2);
// string line variables update to create string art effect:
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
//color effect:
if (i <= numLines/2) {
r += 255/numLines;
g -= 255/numLines;
b += 100/numLines;
} else {
r -= 255/numLines;
g += 255/numLines;
b -= 100/numLines;
}
}
}
I decided to do a star shape for this project, and I’ve attached an image of my initial sketches. It took me a while to figure out how I wanted to use the ‘string art’ style, but I like the outcome that this method created.
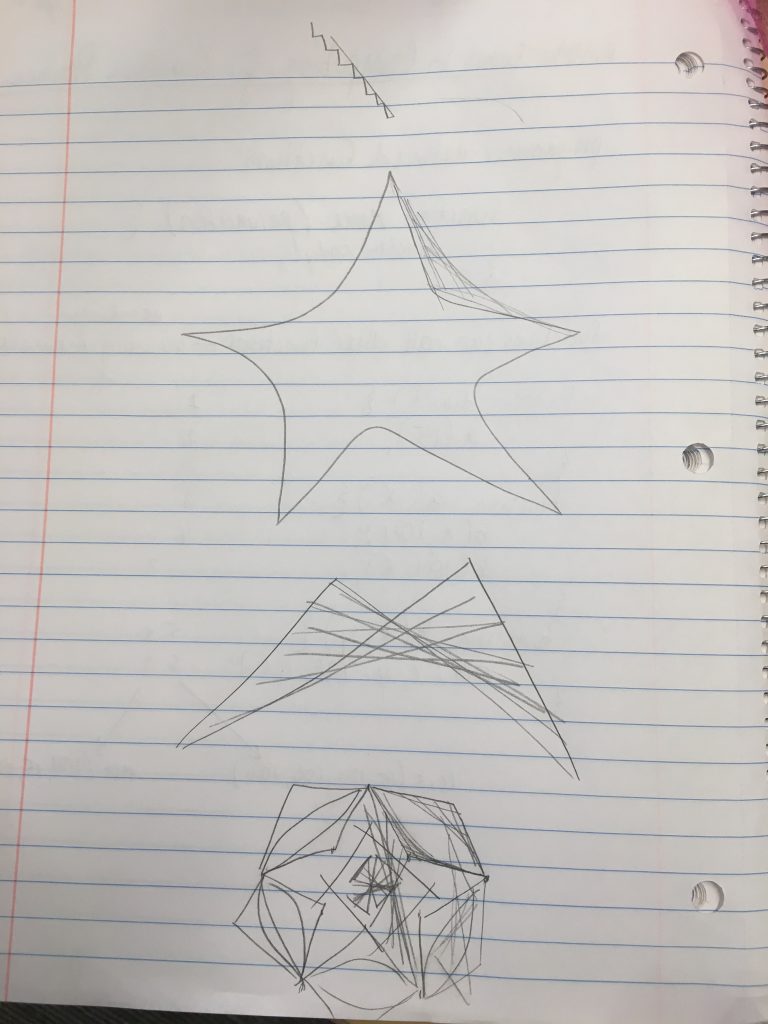
Project 4- String Art
//Eva Oney
//eoney
//section C
var c1 = 0;
var c2 = 255;
function setup() {
createCanvas(400, 300);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(0);
//loop for lines coming off the x axis
for (var i = 1; i <= 500; i += 5) {
stroke(255,0,0);
//I found my x and y values by originally setting x1 and x2 to mouseX
//and mouseY, then when I found a position I liked, I printed the value
//of mouseX and mouseY and got (15,400)
line(400 + i, 0, 15 +i, 150);
line(15 + i, 150, 400 +i, 400);
}
//loop for lines coming off the y axis
for(var i = 0; i <=500; i +=5) {
stroke(0,0,255);
line(0, 400 + i, 200, 15 + i );
line(400, 400 + i, 200, 15 +i);
}
noLoop();
}
I kept my code pretty simple so I could focus on understanding how the loops and parameters actually affect the image. I am not a math wiz by nature, so it is a little difficult for me to understand but I am slowly getting the hang of it.
Project-04: String Art
//i made mine a smile lol
var dx1;
var dy1;
var dx2;
var dy2;
var dy3;
var dy4;
var numLines = 50;
var x = 0;
var y = 0;
function setup() {
createCanvas(400, 300);
background(244, 195, 195); //baby pink color
line(50, 50, 150, 300); //start line
line(300, 300, 350, 100); //end line
dx1 = (150-50)/numLines;
dy1 = (300-50)/numLines;
dx2 = (350-300)/numLines;
dy2 = (100-300)/numLines;
line(50, 50, 150, 300); //start line
line(300, 300, 350, 100); //end line
dx3 = (150-50)/numLines;
dy3 = (300-50)/numLines;
dx4 = (350-300)/numLines;
dy4 = (100-300)/numLines;
}
function draw() {
fill(0,0,0);
ellipse(300, 100, 10,10);
ellipse(220, 100, 10,10);
//lines going across canvas-parallel look
for (var i = 0; i <=400; i +=20) {
stroke(53,81,95); // grey blue color
line(width - i, y, x, y + i*4);
line(i, height, width, height - i*4);
line(width - i, height, x, height - i*4);
line(i, y, width, y + i*4);
}
//circle on outside
for (var i = 0; i <=400; i +=20) {
stroke(255,255,255); //white
line(width - i, y, width, height - i);
line(i, height, x, y + i);
line(width - i, height, width, y + i);
line(i, y, x, height - i);
}
// function given from instructions
var x1 = 0;
var y1 = 0;
var x2 = 350;
var y2 = 350;
for (var i = 0; i <= numLines; i += 1) {
stroke(255,0,0); //red
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
noLoop();
var x3 = 350;
var y3 = 350;
var x4 = 0;
var y4 = 0;
for (var i = 0; i <= numLines; i += 1) {
fill (255,0,0); //red
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
}
Looking Outwards: 04
Sound Art
The project that caught my attention was The Product – Soundmachines, Creative Sound Production Device 2011. This video shows three units, which resembles a standard record player, translate concentric visual patterns into control signals for further processing in any music software. Each rotation of the record discs holds three tracks, and they can be synced into a sequencer. This project was created by a producer/sound designer Yannick Labbé. I was fascinated by the sounds produced by the record discs, it had a techno feel to it, and it was interesting watching the visual/sound elements come together to create this ensemble.
Project-04
//Nami Numoto
//mnumoto
//Section A
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 50;
var r = 0; //red
var g = 0; //green
var b = 0; //blue
function setup() {
createCanvas(400, 400);
background(200);
line(50, 50, 150, 300);
line(300, 300, 350, 100);
var r = random(0,255);
var g = random(0,255);
var b = random(0,255);
dx1 = (150-50)/numLines;
dy1 = (300-50)/numLines;
dx2 = (350-300)/numLines;
dy2 = (100-300)/numLines; //shape 1 (given)
ex1 = 2 * (150-50)/numLines;
ey1 = 2 * (300-50)/numLines;
ex2 = 2 * (350-300)/numLines;
ey2 = 2 * (100-300)/numLines; //shape 2
fx1 = 4 * (150-50)/numLines;
fy1 = 4 * (300-50)/numLines;
fx2 = 4 * (350-300)/numLines;
fy2 = 4 * (100-300)/numLines; //shape 3
}
function draw() {
var x1 = 50;
var y1 = 50;
var x2 = 300;
var y2 = 300;
stroke(r, 0, 0); //set the stroke colour to some shade of red
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
stroke(0, g, 0); //set the stroke colour to some shade of green
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += ex1;
y1 += ey1;
x2 += ex2;
y2 += ey2;
}
stroke(0, 0, b); //set the stroke colour to some shade of blue
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += fx1;
y1 += fy1;
x2 += fx2;
y2 += fy2;
}
noLoop();
}
I’m not sure why it’s showing up the way it is – baseline, I struggled a lot with this project (mostly technically).
The idea was to make a fractal of sorts by squaring (hence the doubling and quadrupling) the original shape. I also intended to make each segment a different shade of red, blue, and green, infinitely.
Any suggestions? I was going to do a loop, but I was running low on time so I simply did it manually three times.
Project-04-String-Art
Project 04: String Art
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 30;
var ex1;
var ey1;
var ex2;
var ey2;
var enumLines = 30;
var fx1;
var fy1;
var fx2;
var fy2;
var fnumLines = 30;
function setup() {
createCanvas(400, 300);
background(220);
line(10, 10, 10, 100); //top left shape
line(10, 55, 100, 55);
dx1 = (10 - 10)/numLines;
dy1 = (100 - 10)/numLines;
dx2 = (100 - 10)/numLines;
dy2 = (55 - 55)/numLines;
line(130, 150, 200, 200); //bottom shape
ex1 = (200 - 130)/enumLines;
ey1 = (200 - 150)/enumLines;
ex2 = (130 - 200)/enumLines;
ey2 = (200 - 150)/enumLines;
line(250, 50, 250, 150); //top right shape
fx1 = (250 - 250)/fnumLines;
fy1 = (150 - 50)/fnumLines;
fx2 = (300 - 200)/fnumLines;
fy2 = (100 - 100)/fnumLines;
}
function draw() {
var x1 = 10 //top left shape
var y1 = 10
var x2 = 100
var y2 = 55
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
var eex1 = 130 //bottom shape
var eey1 = 150
var eex2 = 200
var eey2 = 200
for (var i = 0; i <= enumLines; i += 1) {
line(eex1, eey1, eex2, eey2);
eex1 += ex1;
eey1 += ey1;
eex2 += ex2;
eey2 += ey2;
}
var ffx1 = 250
var ffy1 = 50
var ffx2 = 250
var ffy2 = 150
for (var i = 0; i <= fnumLines; i += 1) {
line(ffx1, ffy1, ffx2, ffy2);
ffx1 += fx1;
ffy1 += fy1;
ffx2 += fx2;
ffy2 += fy2;
}
noLoop();
}
I thought this project was very difficult, but I am happy with my outcomes.
LO 4: Sound Art
The Cotodama lyric speaker was produced to act as a canvas that would digitally display the lyrics to any song playing through its speakers. Although this doesn’t exactly fall under the classification of computationally generated music, I feel like this is a product that works under very similar mechanics.
I like the product itself due to its elegant look, though it looks as if it comes as two separate pieces, it is actually one solid unit designed to look like vinyl record jackets leaned up against the wall. I also think it’s a great example of sound data being used in coordination with databases to create visuals. When connected to an audio source, its display panel sources lyrics from databases
and displays the lyrics in sync with the song. It also is programmed to analyze a song’s mood to pick corresponding fonts and animations. In cases where no lyrics can be found for a song, the speaker will display AI-generated animations that move in sync with the music instead.
https://lyric-speaker.com/en/history/
Musical and Computational Inspiration
The mix of computer science and music is a combination that I love to no end. As both a musician and an avid computer science nerd, the idea that there are ways to combine these two concepts is amazing to me. There is one musical instrument that has always fascinated me. That would have to be the Theremin. Built by physicist Lev Sergeyevich Termen, the theremin is an instrument that does not need to be touched in order to create sound. The Theremin works by generating electromagnetic waves between two antennae. Kind of like a graph, the horizontal antenna controls volume while the vertical antenna controls pitch. I admire this instrument because of its originality and uniqueness. The sounds produced are heavily saturated computer-generated sounds that are like no other. When a musician is playing the Theremin, their hands move through space like they are dancing.
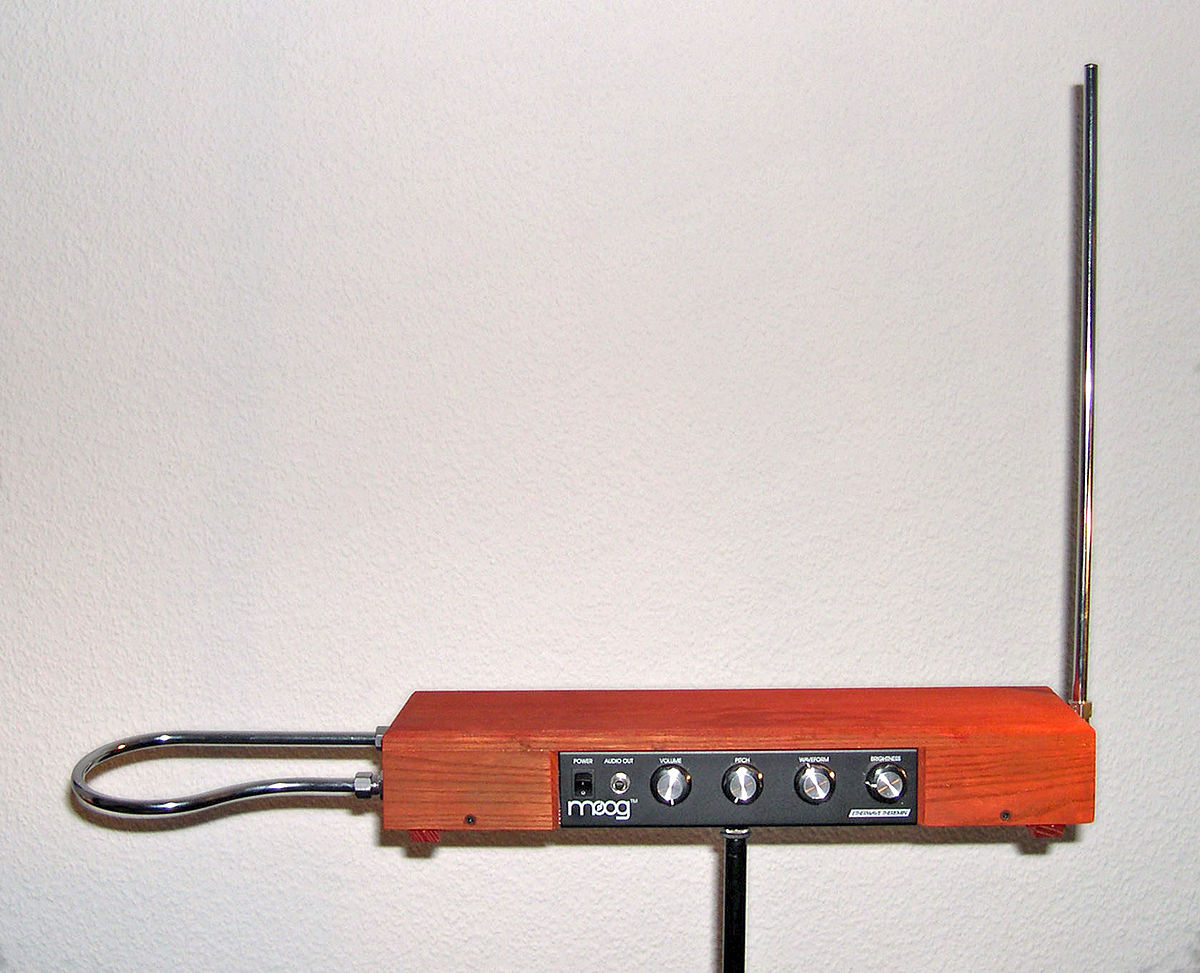