I worked too hard on this project. I ended up drawing all of the images in my code on procreate, so I do not have many sketches except for some resizing issues.
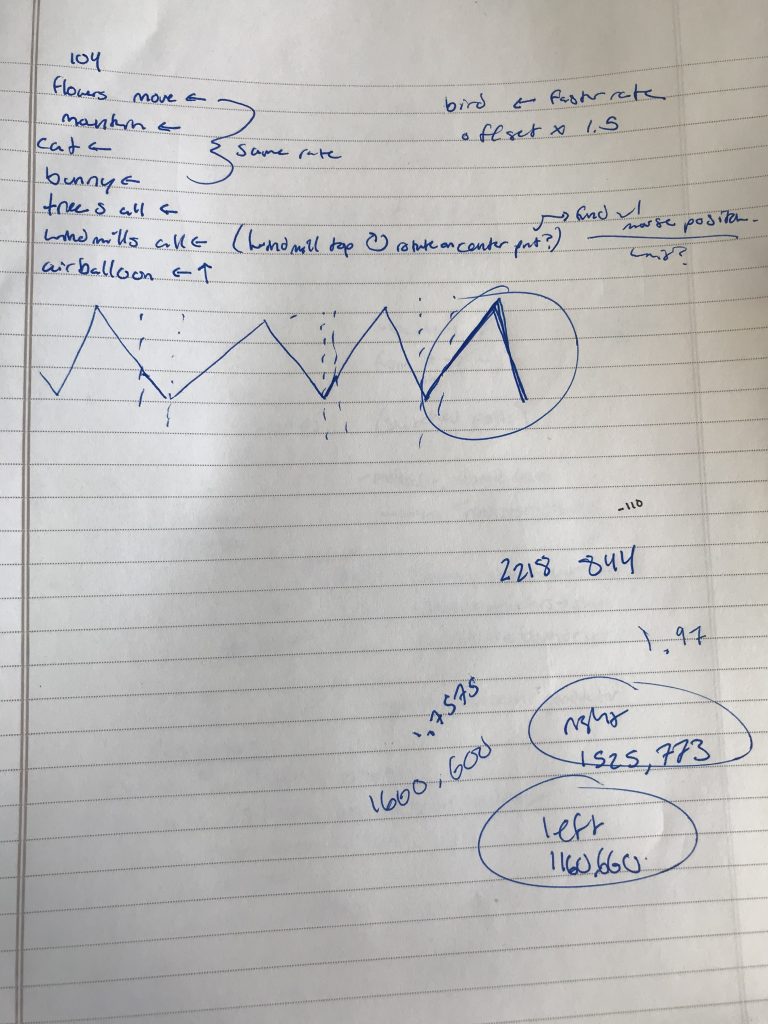
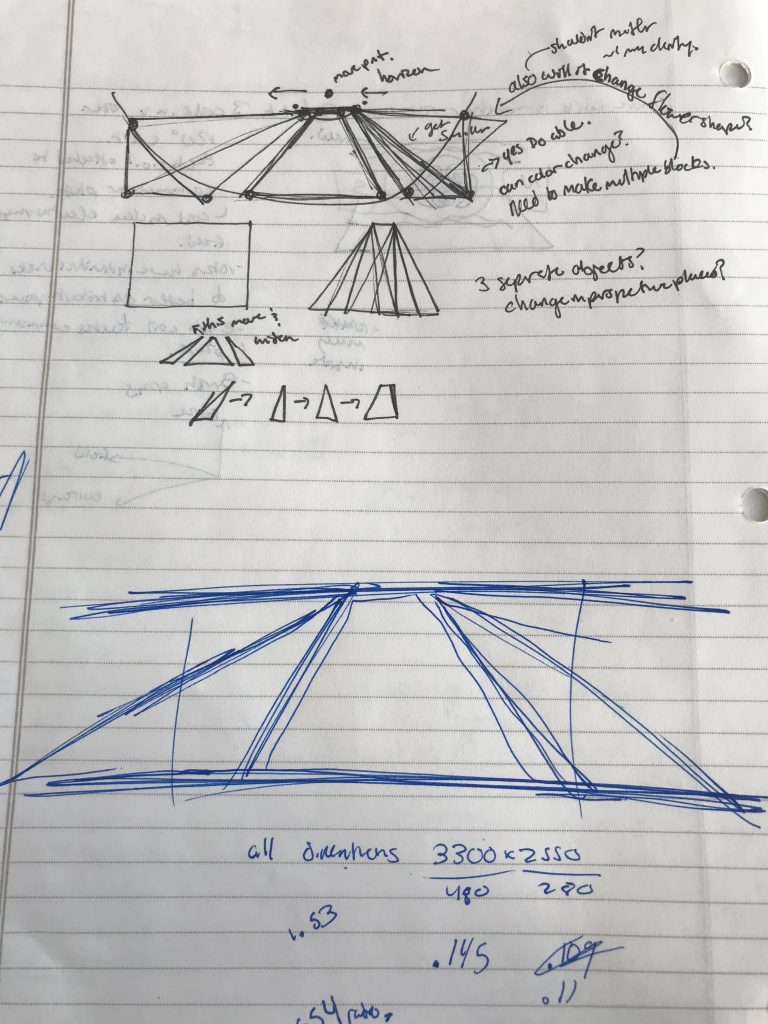
The code was easier to figure out than I thought, but because I decided to use so many elements it got repetitive fast, there are a few things I could have tried i think in ways of getting my stuff to show up more randomly, but because of the way I wrote my code I found that setting that up farther would have not been worth the time for the effect.
Drawing took a few days, but I really like my images.
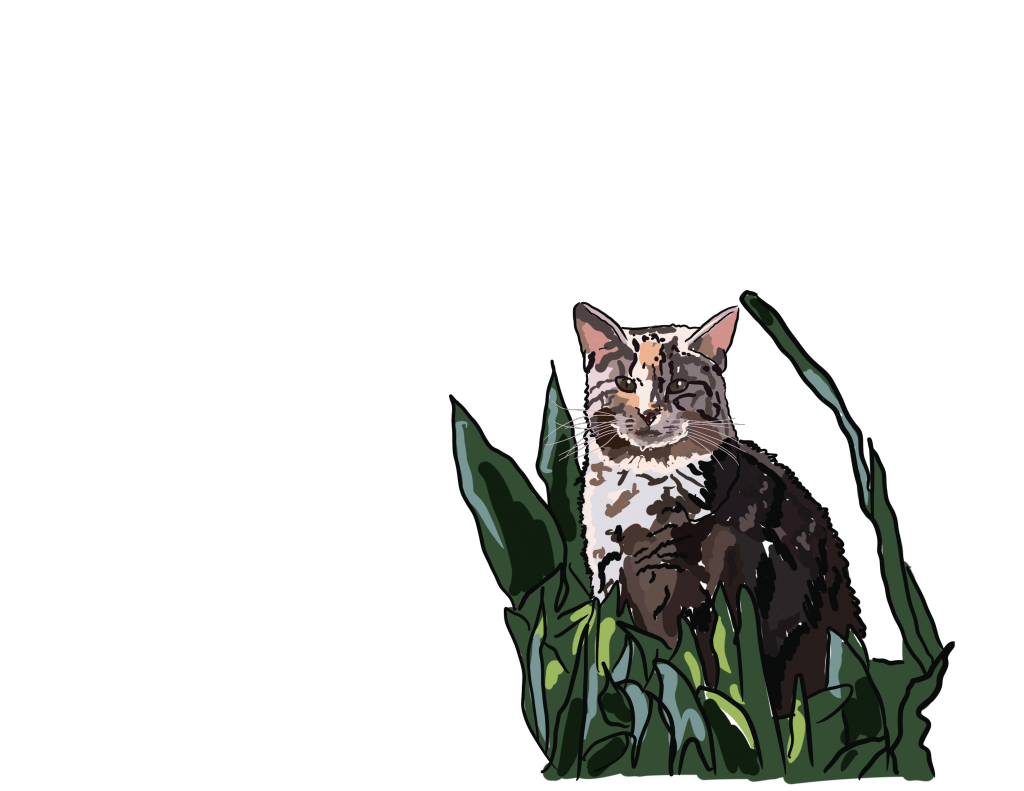
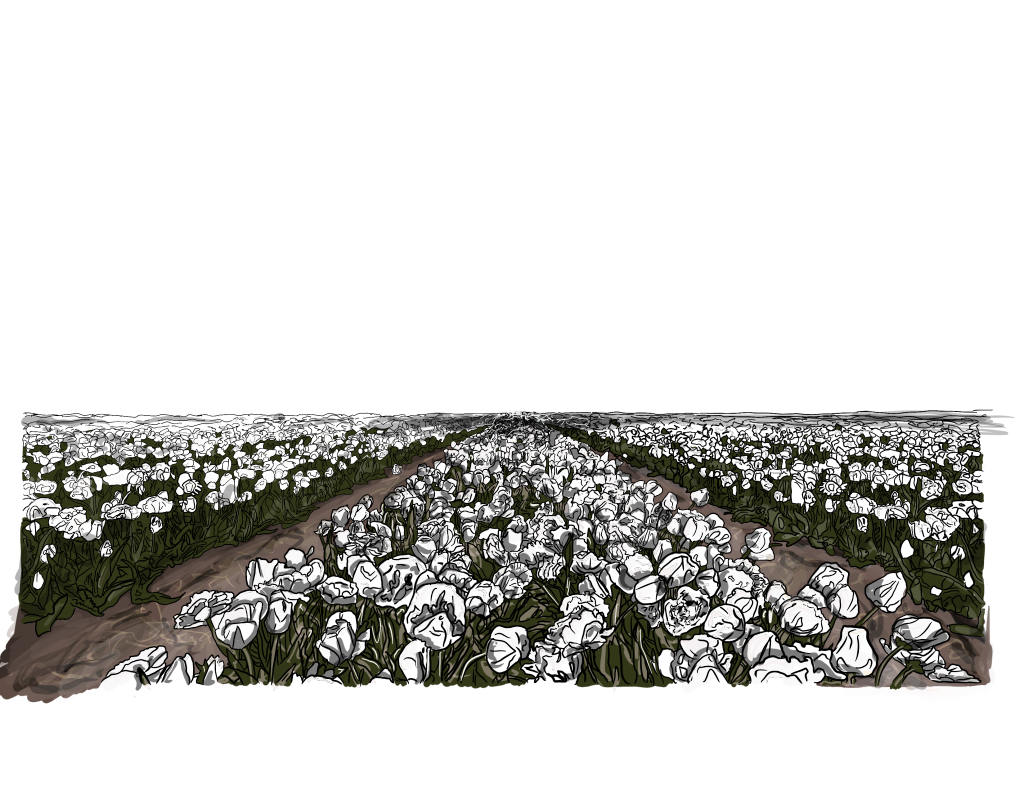
Some of the math I had to do really made me think and I can’t quite decide if using images made this take longer or shorter to code.
var szex = 550; // x size for some images
var szey = 365; //y size for some images
var szex2 = 367.2; //x size for smaller img
var szey2 = 240; //y size for smaller img
var tulip = [];
var offset = 0;
var skys = [];
var mountains = [];
var airballoons = [];
var trees = [];
var windmillBottoms = [];
var birds = [];
var electric = [];
var bunnys = [];
var fullWind = [];
var treeLines = [];
var cats = [];
function preload() {
flowerImg = loadImage("https://i.imgur.com/VSMWH0A.png");
tree1Img = loadImage("https://i.imgur.com/s66n2RJ.png");
mountainsImg = loadImage("https://i.imgur.com/FCSY9xA.png");
birdImg = loadImage("https://i.imgur.com/2zN4jIk.png");
windmillBottomImg = loadImage("https://i.imgur.com/xGOhbMn.png");
electricWindmillImg = loadImage("https://i.imgur.com/0R2RKsz.png");
airballoonImg = loadImage("https://i.imgur.com/HPmyDXQ.png");
bunnyImg = loadImage("https://i.imgur.com/tOtLTev.png");
fullWindmillImg = loadImage("https://i.imgur.com/EUCzk9P.png");
treeLineImg = loadImage("https://i.imgur.com/ipeIQSL.png");
catImg = loadImage("https://i.imgur.com/d9pAL7i.png");
skyImg = loadImage("https://i.imgur.com/AykCYLx.png");
}
function tulips(tx) {
var p = {x: tx,
right: tulipsRight,
display: tulipsDisplay};
return p;
}
function tulipsRight() {
return this.x + 900;
}
function tulipsDisplay(tx) {
flower2Img.resize(flower2Img.width - 100, flower2Img.height);
image(flower2Img, this.x, 190, 900, 105);//left
}
function bird(bx) {
var b = {x: bx,
right: birdRight,
display: birdDisplay};
return b;
}
function birdRight() {
return this.x + szex2 + width * 9;
}
function birdDisplay(tx) {
image(birdImg, this.x + 200, 0, 165.4, 100); //move faster across the screen
}
function airballoon(ax) {
var a = {x: ax,
right: airballoonRight,
display: airballoonDisplay};
return a;
}
function airballoonRight() {
return this.x + szex2 + width * 1.5;
}
function airballoonDisplay(tx) {
image(airballoonImg, this.x, 110, szex2, szey2);
}
function sky(sx) {
var s = {x: sx,
right: skyRight,
display: skyDisplay};
return s;
}
function skyRight() {
return this.x + 500;
}
function skyDisplay(tx) {
image(skyImg, this.x, 0, 500, 300);
}
function mountain(mx) {
var m = {x: mx,
right: mountainRight,
display: mountainDisplay};
return m;
}
function mountainRight() {
return this.x + 827 + width * 5;
}
function mountainDisplay(tx) {
image(mountainsImg, this.x - 20, -85, 827, 500);
}
function tree(tx) {
var t = {x: tx,
right: treeRight,
display: treeDisplay};
return t;
}
function treeRight() {
return this.x + szex + width * 1.5;
}
function treeDisplay(tx) {
image(tree1Img, this.x, 20, szex, szey);
}
function treeLine(tx) {
var t = {x: tx,
right: treeLineRight,
display: treeLineDisplay};
return t;
}
function treeLineRight() {
return this.x + szex + width * 1.7;
}
function treeLineDisplay(tx) {
image(treeLineImg, thisx, 25, szex, szey); //with mountains or without
}
function windmillBottom(wx) {
var w = {x: wx,
right: windmillBottomRight,
display: windmillBottomDisplay};
return w;
}
function windmillBottomRight() {
return this.x + szex2 + width * 2.2;
}
function windmillBottomDisplay(tx) {
image(windmillBottomImg, this.x + 150, 87, szex2, szey2);//dont show with mountain
}
function windmill(wx) {
var w = {x: wx,
right: windmillRight,
display: windmillDisplay};
return w;
}
function windmillRight() {
return this.x + 248 + width * 3.2;
}
function windmillDisplay(tx) {
image(fullwindmillImg, this.x + 20, 100, 248, 150);
}
function electricwindmill(wx) {
var w = {x: wx,
right: electricwindmillRight,
display: electricwindmillDisplay};
return w;
}
function electricwindmillRight() {
return this.x + szex + width * 10.2;
}
function electricwindmillDisplay(tx) {
image(electricWindmillImg, this.x , 5, szex, szey); //dont show with other windmills
}
function bunny(bx) {
var b = {x: bx,
right: bunnyRight,
display: bunnyDisplay};
return b;
}
function bunnyRight() {
return this.x + szex2 + width * 7.3;
}
function bunnyDisplay(tx) {
image(bunnyImg, this.x, 130, szex2, szey2);
}
function cat(cx) {
var c = {x: cx,
right: catRight,
display: catDisplay};
return c;
}
function catRight() {
return this.x + width * 5.5;
}
function catDisplay(tx) {
image(catImg, this.x + 200, 160, 206.75, 125);
}
function setup() {
createCanvas(480, 280);
var sk = sky(1);
skys.push(sk);
var tul = tulips(1);
tulip.push(tul);
var ar = airballoon(1);
airballoons.push(ar);
var mount = mountain(1);
mountains.push(mount);
var bunn = bunny(1);
bunnys.push(bunn);
var kitten = cat(1);
cats.push(kitten);
var bid = bird(1);
birds.push(bid);
var tr = tree(1);
trees.push(tr);
var tre = treeLine(1);
treeLines.push(tre);
var wmb = windmillBottom(1);
windmillBottoms.push(wmb);
var fw = windmill(1);
fullWind.push(fw);
var ew = electricwindmill(1);
electric.push(ew);
}
function draw() {
noStroke();
//SKY
for (var i = 0; i < skys.length; i++) {
var s = skys[i];
image(skyImg, s.x - offset, 0, 500, 400);//left
}
// clean up statement
if (skys.length > 0 & skys[0].right() < offset) {
skys.shift();
}
// make a new sky
var lastSky = skys[skys.length-1];
if (lastSky.right() - offset < width) {
var s = sky(lastSky.right());
skys.push(s);
}
//MOUNTAIN
for (var i = 0; i < mountains.length; i++) {
var m = mountains[i];
image(mountainsImg, m.x - offset, -78, 827, 500);
}
// make a new mountain
var lastMountain = mountains[mountains.length-1];
if (lastMountain.right() - offset < width) {
var m = mountain(lastMountain.right());
mountains.push(m);
}
//TREE
for (var i = 0; i < trees.length; i++) {
var tr = trees[i];
image(tree1Img, tr.x - offset, 23, szex, szey);
}
// clean up statement
if (trees.length > 0 & trees[0].right() < offset) {
trees.shift();
}
// make a new tree
var lastTree = trees[trees.length-1];
if (lastTree.right() - offset < width) {
var tr = tree(lastTree.right());
trees.push(tr);
}
//TREELINE
for (var i = 0; i < treeLines.length; i++) {
var tre = treeLines[i];
image(treeLineImg, tre.x - offset, 25, szex, szey); //with mountains or without
}
// clean up statement
if (treeLines.length > 0 & treeLines[0].right() < offset) {
treeLines.shift();
}
// make a new treeline
var lastTreeLine = treeLines[treeLines.length-1];
if (lastTreeLine.right() - offset < width) {
var tre = treeLine(lastTreeLine.right());
treeLines.push(tre);
}
//WINDMILLBOTTOM
for (var i = 0; i < windmillBottoms.length; i++) {
var wnd = windmillBottoms[i];
image(windmillBottomImg, wnd.x - offset, 87, szex2, szey2);
}
// clean up statement
if (windmillBottoms.length > 0 & windmillBottoms[0].right() < offset) {
windmillBottoms.shift();
}
// make a new windmill
var lastwindmillBottom = windmillBottoms[windmillBottoms.length-1];
if (lastwindmillBottom.right() - offset < width) {
var wnd = windmillBottom(lastwindmillBottom.right());
windmillBottoms.push(wnd);
}
//ELECTRIC WINDMILL
for (var i = 0; i < electric.length; i++) {
var ew = electric[i];
image(electricWindmillImg, ew.x - offset, 5, szex, szey); //dont show with other windmills
}
// clean up statement
if (electric.length > 0 & electric[0].right() < offset) {
electric.shift();
}
// make a new windmill
var lastElectricWindmill = electric[electric.length-1];
if (lastElectricWindmill.right() - offset < width) {
var ew = electricwindmill(lastElectricWindmill.right());
electric.push(ew);
}
//WINDMILL
for (var i = 0; i < fullWind.length; i++) {
var full = fullWind[i];
image(fullWindmillImg, full.x - offset, 100, 248, 150); //can be anytime show up
}
// clean up statement
if (fullWind.length > 0 & fullWind[0].right() < offset) {
fullWind.shift();
}
// make a new windmill
var lastWindmill = fullWind[fullWind.length-1];
if (lastWindmill.right() - offset < width) {
var full = windmill(lastWindmill.right());
fullWind.push(full);
}
//AIRBALLOONS
for (var i = 0; i < airballoons.length; i++) {
var air = airballoons[i];
image(airballoonImg, air.x - offset, 110 - offset/10, szex2, szey2);
}
// clean up statement
if (airballoons.length > 0 & airballoons[0].right() < offset) {
airballoons.shift();
}
// make a new balloom
var lastAirballoon = airballoons[airballoons.length-1];
if (lastAirballoon.right() - offset < width) {
var air = airballoon(lastAirballoon.right());
airballoons.push(air);
}
//background for tulips
for(var i = 0 ; i < width; i ++){
fill(253, 218 - offset / 75, 42);
rect(0, 195, 480, 100);
}
//slight change every time offset increases so slight won't notice till big change
//TULIPS
for (var i = 0; i < tulip.length; i++) {
var t = tulip[i];
image(flowerImg, t.x - offset, 190, 900, 105);//left
}
// clean up statement
if (tulip.length > 0 & tulip[0].right() < offset) {
tulip.shift();
}
// make a new tulip
var lastTulip = tulip[tulip.length-1];
if (lastTulip.right() - offset < width) {
var t = tulips(lastTulip.right());
tulip.push(t);
}
//BIRD ??NEEDS FIX
for (var i = 0; i < birds.length; i++) {
var bids = birds[i];//need to change spawn pnt
image(birdImg, bids.x - (offset + offset), 0, 165.4, 100); //move faster across the screen
}
// clean up statement
if (birds.length > 0 & birds[0].right() < offset) {
birds.shift();
}
// make a new bird //make less often
var lastBird = birds[birds.length-1];
if (lastBird.right() - (offset + offset) < width) {
var bids = bird(lastBird.right());
birds.push(bids);
}
//BUNNY
for (var i = 0; i < bunnys.length; i++) {
var buns = bunnys[i];
image(bunnyImg, buns.x - offset, 130, szex2, szey2);
}
// clean up statement
if (bunnys.length > 0 & bunnys[0].right() < offset) {
bunnys.shift();
}
// make a new rabbit //make less often
var lastBunny = bunnys[bunnys.length-1];
if (lastBunny.right() - offset < width) {
var buns = bunny(lastBunny.right());
bunnys.push(buns);
}
//CAT
for (var i = 0; i < cats.length; i++) {
var kittens = cats[i];
image(catImg, kittens.x - offset , 160, 206.75, 125);
}
// clean up statement
if (cats.length > 0 & cats[0].right() < offset) {
cats.shift();
}
// make a new cat //make less often
var lastCat = cats[cats.length-1];
if (lastCat.right() - offset < width) {
var kittens = cat(lastCat.right());
cats.push(kittens);
}
offset ++;
}