Pandora
This project was inspired by the landscape of the planet Pandora created by director James Cameron in the blockbuster Avatar.
//Sihan Dong
//sihand@andrew.cmu.edu
//Section B
//Project Week 10: generative landscape
var buds = [];
var terrainSpeed = 0.0001;
var terrainDetail = 0.007;
function setup() {
createCanvas(600, 300);
// create the initial buds
for (var i = 0; i < 10; i++){
var budx = random(width);
buds[i] = makeBuds(budx);
}
frameRate(50);
}
function draw() {
background(0);
updateAndDisplayBuds();
removeBudsThatHaveSlippedOutOfView();
addNewBudsWithSomeRandomProbability();
updateHalo();
}
function updateHalo() {
//update the halo's position
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0,1, 0, height/2);
fill(90, 240, 248, 40);//upper halo
ellipse(x, y, 2, 80);
fill(37, 142, 242, 40);//lower halo
ellipse(x, y+50, 2, 90);
}
}
function updateAndDisplayBuds(){
// Update the bud's positions, and display them.
for (var i = 0; i < buds.length; i++){
buds[i].move();
buds[i].display();
}
}
function removeBudsThatHaveSlippedOutOfView(){
var budsToKeep = [];
for (var i = 0; i < buds.length; i++){
if (buds[i].x + buds[i].budWidth/2 > 0) {
budsToKeep.push(buds[i]);
}
}
buds = budsToKeep;
}
function addNewBudsWithSomeRandomProbability() {
var newBudLikelihood = 0.03;
if (random(0,1) < newBudLikelihood) {
buds.push(makeBuds(width));
}
}
function budsMove() {
this.x += this.speed;
}
function budsDisplay(birthLocationX) {
fill(this.color);
noStroke();
//draw the flower
push();
translate(this.x, height);
//draw the stem
rect(0, -this.stemHeight-5, this.stemWidth, this.stemHeight+5);
ellipseMode(CENTER);
//draw the petals
ellipse(0, -this.stemHeight-this.budHeight/2, this.budWidth, this.budHeight);
ellipse(10, -this.stemHeight-this.budHeight/2, this.budWidth, this.budHeight);
ellipse(-5, -this.stemHeight-this.budHeight/2-5, this.budWidth, this.budHeight-5);
ellipse(15, -this.stemHeight-this.budHeight/2-5, this.budWidth, this.budHeight-5);
ellipse(this.stemWidth/2, -this.stemHeight-this.budHeight/2-5, this.budWidth+5, this.budHeight+5);
pop();
}
function makeBuds(birthLocationX) {
var bds = {x:birthLocationX,
speed: -1.5,
color: color(random(150, 200), random(180), 255,
random(100, 155)), //produce a blue-purple-ish color
stemHeight: random(10, height/2),
stemWidth: random(8, 10),
budHeight: random(40, 60),
budWidth: random(15, 25),
move: budsMove,
display: budsDisplay}
return bds;
}
Here’s a preliminary sketch of the color choices. I did not end up doing the green leafy plants.
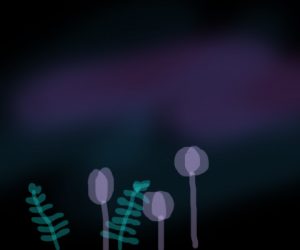
Here’s an image from the movie of which my project is a doodle-y representation.
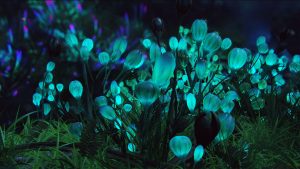