sketch
function setup() {
createCanvas(480, 480);
frameRate(15);
}
function draw() {
background(255, 200, 200);
fill(255, 255, 255, 64);
var nPoints = 30;
var radius = 30;
//fire body
//red fire
push();
translate(width / 2 - 30, height / 2);
beginShape();
noStroke();
fill(201, 75, 56);
beginShape();
for (var i = 0; i < nPoints ; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = 5.5 * radius * cos(theta);
var py = 5.5 * radius * sin(theta);
if (i > 15 & i < 24) {
vertex(px + random(-2, 2), py + random(-2, 2) - 50);
} else {
vertex(px + random(-2, 2), py + random(-2, 2));
}
}
endShape();
pop();
//red-orange fire
push();
translate(width / 2 - 30, height / 2);
beginShape();
noStroke();
fill(230, 112, 84);
beginShape();
for (var i = 0; i < nPoints ; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = 5 * radius * cos(theta);
var py = 5 * radius * sin(theta);
if (i > 15 & i < 24) {
vertex(px + random(-2, 2), py + random(-2, 2) - 50);
} else {
vertex(px + random(-2, 2), py + random(-2, 2));
}
}
endShape();
pop();
//orange fire
push();
translate(width / 2 - 30, height / 2);
beginShape();
noStroke();
fill(235, 173, 78);
beginShape();
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = 3.5 * radius * cos(theta);
var py = 3.5 * radius * sin(theta);
if (i > 13 & i < 22) {
vertex(px + random(-4, 4), py + random(-4, 4) - 40);
} else {
vertex(px + random(-4, 4), py + random(-4, 4));
}
}
endShape();
pop();
//yellow fire
push();
translate(width / 2 - 30, height / 2);
beginShape();
noStroke();
fill(240, 209, 90);
beginShape();
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = 1.5 * radius * cos(theta);
var py = 1.5 * radius * sin(theta);
if (i > 14 & i < 24) {
vertex(px + random(-5, 5), py + random(-5, 5) - 30);
} else {
vertex(px + random(-5, 5), py + random(-5, 5));
}
}
endShape();
pop();
//left cheek
beginShape();
smooth();
noFill();
curveVertex(width / 4 + 5 + random(-2, 2), height / 2 + 60 + random(-2, 2));
curveVertex(width / 4 + 5 + random(-2, 2), height / 2 + 50 + random(-2, 2));
curveVertex(width / 4 + 10 + 10 + random(-2, 2), height / 2 + 50 - 10 + random(-2, 2));
curveVertex(width / 4 + 10 + 20 + 5 + random(-2, 2), height / 2 + 50 - 5 + random(-2, 2));
curveVertex(width / 4 + 10 + 20 + 8 + random(-2, 2), height / 2 + 50 + 14 + random(-2, 2))
curveVertex(width / 4 + 10 + 20 + random(-2, 2), height / 2 + 50 + 20 + random(-2, 2))
endShape();
//right cheek
beginShape();
smooth();
noFill();
curveVertex(width / 2 + 60 + random(-2, 2), height / 3 + 40 + random(-2, 2));
curveVertex(width / 2 + 40 + random(-2, 2), height / 3 + 20 + random(-2, 2));
curveVertex(width / 2 + 40 - 12 + random(-2, 2), height / 3 + 20 + 15 + random(-2, 2));
curveVertex(width / 2 + 40 + random(-2, 2), height / 3 + 20 + 30 + random(-2, 2));
curveVertex(width / 2 + 40 + 10 + random(-2, 2), height / 3 + 20 + 30 + random(-2, 2))
curveVertex(width / 2 + 80 + random(-2, 2), height / 3 + 30 + random(-2, 2))
endShape();
//mouth
beginShape();
smooth();
noFill();
curveVertex(width / 4 + 10 + 20 + 5 + random(-2, 2) - 20, height / 2 + 50 - 5 + random(-2, 2) + 50);
curveVertex(width / 4 + 10 + 20 + 5 + random(-2, 2), height / 2 + 50 - 5 + random(-2, 2));
curveVertex(width / 4 + 10 + 20 + 30 + random(-2, 2), height / 2 - 25 + random(-2, 2));
curveVertex(width / 2 + random(-2, 2) - 20, height / 2 - 30 + random(-2, 2));
curveVertex(width / 2 + 40 - 12 + random(-2, 2), height / 3 + 20 + 15 + random(-2, 2));
curveVertex(width / 2 + 40 - 12 + random(-2, 2) + 30, height / 3 + 20 + 15 + random(-2, 2));
endShape();
//left eye
push();
fill(255);
translate(width / 4, height / 2);
beginShape();
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-1, 1), py + random(-1, 1));
}
endShape(CLOSE);
//left pupil
push();
fill(0);
var mouseXeyes = map(mouseX, 0, 1000, -10, 10);
var mouseYeyes = map(mouseY, 0, 800, -10, 10);
ellipse(mouseXeyes, mouseYeyes, 20, 20);
pop();
//right eye
translate(width / 4, -height / 6);
beginShape();
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-1, 1), py + random(-1, 1));
}
endShape(CLOSE);
//right pupil
push();
fill(0);
var mouseXeyes = map(mouseX, -500, 1000, -10, 10);
var mouseYeyes = map(mouseY, -500, 1000, -10, 10);
ellipse(mouseXeyes, mouseYeyes, 20, 20);
pop();
pop();
//bottom text
stroke(0);
fill(0);
textFont("Georgia");
textSize(22);
text("calcifer.", width / 2 - 30, height / 10 * 9 + 10);
//egg
push();
noStroke();
fill(255);
beginShape();
smooth();
ellipse(mouseX, mouseY, 50, 60)
endShape(CLOSE);
stroke(0);
beginShape();
vertex(mouseX - 23, mouseY - 10)
vertex(mouseX - 15, mouseY - 2)
vertex(mouseX - 5, mouseY - 10)
vertex(mouseX + 5, mouseY - 2)
vertex(mouseX + 15, mouseY - 10)
vertex(mouseX + 25, mouseY - 2)
endShape();
pop();
}
For this project, I was inspired by the moving circle in the example given. I used this to attempt to recreate one of my favorite characters from Studio Ghibli, Calcifer. The moving points give Calcifer the feel of a live fire.
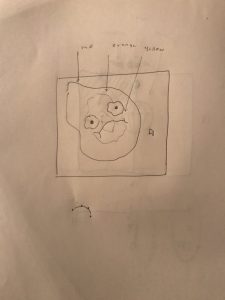