// Lingfan Jiang
// Section B
// lingfanj@andrew.cmu.edu
// Project-10
var people = [];
function setup() {
createCanvas(480, 300);
// create an initial collection of people
for (var i = 0; i < 10; i++){
var rx = random(width);
people[i] = makePeople(rx);
}
}
function draw() {
background(240);
updateAndDisplaypeople();
removepeopleThatHaveSlippedOutOfView();
addNewpeopleWithSomeRandomProbability();
//belt
fill(180);
rect(-5, 190, 500, 110);
push();
translate(-100, 0);
for (var i = 0; i < 20; i++) {
stroke(100);
line((i + 3) * 50, 190, i * 50, 300);
};
pop();
stroke("pink");
line(0, 190, 480, 190);
line(0, 297, 480, 297);
//draw sushi bottom
noStroke();
fill(255);
beginShape();
curveVertex(180, 250);
curveVertex(250, 240);
curveVertex(300, 260);
curveVertex(250, 270);
endShape(CLOSE);
//draw sushi top
fill(255, 160, 160);
beginShape();
curveVertex(150, 240);
curveVertex(170, 235);
curveVertex(240, 220);
curveVertex(300, 230);
curveVertex(330, 260);
curveVertex(240, 250);
curveVertex(150, 260);
endShape(CLOSE);
//draw lines above sushi
stroke(255, 201, 201);
strokeWeight(7);
line(200, 230, 220, 246);
line(160, 240, 175, 252);
line(240, 222, 265, 245);
line(280, 225, 315, 256);
}
function updateAndDisplaypeople(){
// Update the people's positions, and display them.
for (var i = 0; i < people.length; i++){
people[i].move();
people[i].display();
}
}
function removepeopleThatHaveSlippedOutOfView(){
// If a people has dropped off the left edge,
// remove it from the array. This is quite tricky, but
// we've seen something like this before with particles.
// The easy part is scanning the array to find people
// to remove. The tricky part is if we remove them
// immediately, we'll alter the array, and our plan to
// step through each item in the array might not work.
// Our solution is to just copy all the people
// we want to keep into a new array.
var peopleToKeep = [];
for (var i = 0; i < people.length; i++){
if (people[i].x + people[i].breadth > 0) {
peopleToKeep.push(people[i]);
}
}
people = peopleToKeep; // remember the surviving people
}
function addNewpeopleWithSomeRandomProbability() {
// With a very tiny probability, add a new people to the end.
var newPeopleLikelihood = 0.007;
if (random(0,1) < newPeopleLikelihood) {
people.push(makePeople(width));
}
}
// method to update position of people every frame
function peopleMove() {
this.x += this.speed;
}
// draw the people and some windows
function peopleDisplay() {
fill(255);
stroke(0);
strokeWeight(5);
push();
translate(this.x, height - 100);
//people outline
fill(255, 235, 222);
ellipse(0, 0, this.breadth, this.breadth);
//eyes
fill(255);
strokeWeight(3);
ellipse(-30, -50, 30, 30);
ellipse(30, -50, 30, 30);
fill(0);
ellipse(-30, -40, 5, 5);
ellipse(30, -40, 5, 5);
pop();
}
function makePeople(birthLocationX) {
var ppl = {x: birthLocationX,
breadth: random(150, 300),
speed: -1.0,
move: peopleMove,
display: peopleDisplay}
return ppl;
}
When I first saw this assignment, it reminded me of the conveyor belt sushi right away. However, instead of letting the sushi move in front of a static human, I decided to let the sushi stay. For the generative background, I wanted to learn it since the abstract clock project. It took me some time to figure out how the generative code works, but it is definitely easier than I expected. As a result, it turns out pretty well. If I have more time to work on it, maybe I would do another layer of a background behind the “people”. Overall, it’s a super fun project to do.
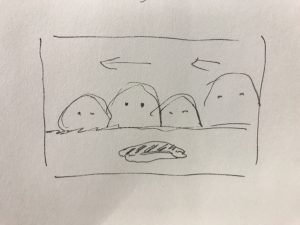