//Kade Stewart
//Section B
//kades
//Project-09
var pic;
var bar = 0;
var barspeed = 5;
function preload() {
pic = loadImage("https://i.imgur.com/TQDoVD9.png");
}
function setup() {
createCanvas(480, 480);
}
function draw() {
//resets the image every time draw is called
image(pic, 0, 0);
loadPixels(pic);
// loop thru each row
for (var y = 0; y < height; y++) {
//every row, shift the pixels over some random amount
//the limit is dictated by how recently an invisible bar has passed over it
var limit = floor( ( ( (height + bar) - y) % height ) / 10 );
var shift = floor(random(0, limit));
// if (limit > 10) {
// continue;
// }
// inner loop that goes thru each pixel in the row
for (var x = 0; x < width; x++) {
//this is the way to target each pixel in the pixels array
var i = ( ( (x + shift) % width ) + y * width ) * 4;
//setting the color of a pixel to the one a certain number away
//pixels[i] is the red, [i+1] is the green, [i+2] is the blue
set(x,y, color(pixels[i], pixels[i + 1], pixels[i + 2]))
}
}
//actually draws the pixels as dictated above
updatePixels();
//moves the invisible bar down, wrapping when it hits the bottom
bar = (bar + barspeed) % height;
}
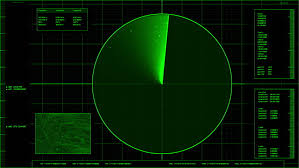
I wanted to make a portrait that emulated the updating of a radar screen. While I didn’t do it exactly, I ended up using a downward moving bar to update the portrait. At first, it was wiping the screen to black. My final has the invisible bar resolving a horizontal shift in the bars of pixels.