//Lauren Park
//ljpark@andrew.cmu.edu
//Section D
//Project-07-Curves
function setup() {
createCanvas(480, 480);
}
function draw() {
background(10);
drawHypotrochoid();
drawHypocycloid();
}
function drawHypotrochoid() {
var x;
var y;
//mouse controls color change for stroke
var r = constrain(mouseX, 0, 300);
var g = constrain(mouseY, 0, 300);
var b = 150;
push();
fill('#53E9FF');
stroke(r, g, b);
strokeWeight(3);
translate(width/2, height/2);
//control hypotrochoid
var ha1 = map(r, 0, width, 0, 100);
var ha2 = map(g, 0, height, 0, 100);
beginShape();
for (var i = 0; i < 480; i+=3) {
var angle1 = map(i, 0, 250, 0, 360);
//equation of hypotrochoid from MathWorld curves site
x = (ha1 - ha2)* cos(angle1) + b * cos((ha1 - ha2 * angle1));
y = (ha1 - ha2)* sin(angle1) - b * sin((ha1 - ha2 * angle1));
vertex(x, y);
}
endShape();
pop();
}
function drawHypocycloid() {
var x;
var y;
//mouse controls color change for stroke
var r = map(mouseX, 0, width, 50, 255);
var g = map(mouseY, 0, height, 50, 255);
push();
fill(254, 193, 255, 140);
stroke(r, g, 255);
strokeWeight(0.3);
translate(width/2, height/2);
//control hypocycloid
var hy1 = map(mouseX, 0, width, 0, 300);
var hy2 = map(mouseY, 0, height, 0, 300);
beginShape();
for (var a = 0; a < 200; a+=3) {
var angle2 = map(a, 0, 100, 0, 360);
//equation of hypocycloid from MathWorld curves site
x = (hy1 + hy2)* cos(angle2) + hy2* cos((hy1 + hy2)*angle2);
y = (hy1 + hy2)* sin(angle2) - hy2* sin((hy1 + hy2)*angle2);
vertex(x, y);
}
endShape();
pop();
}
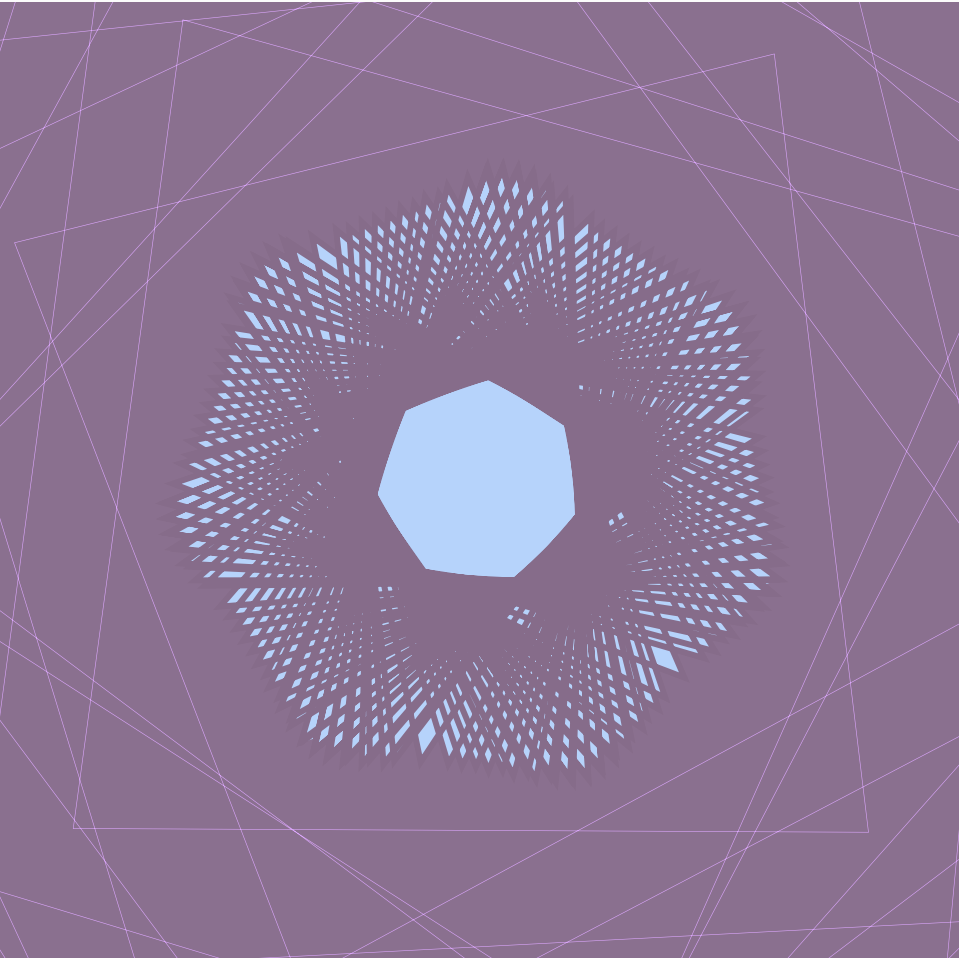
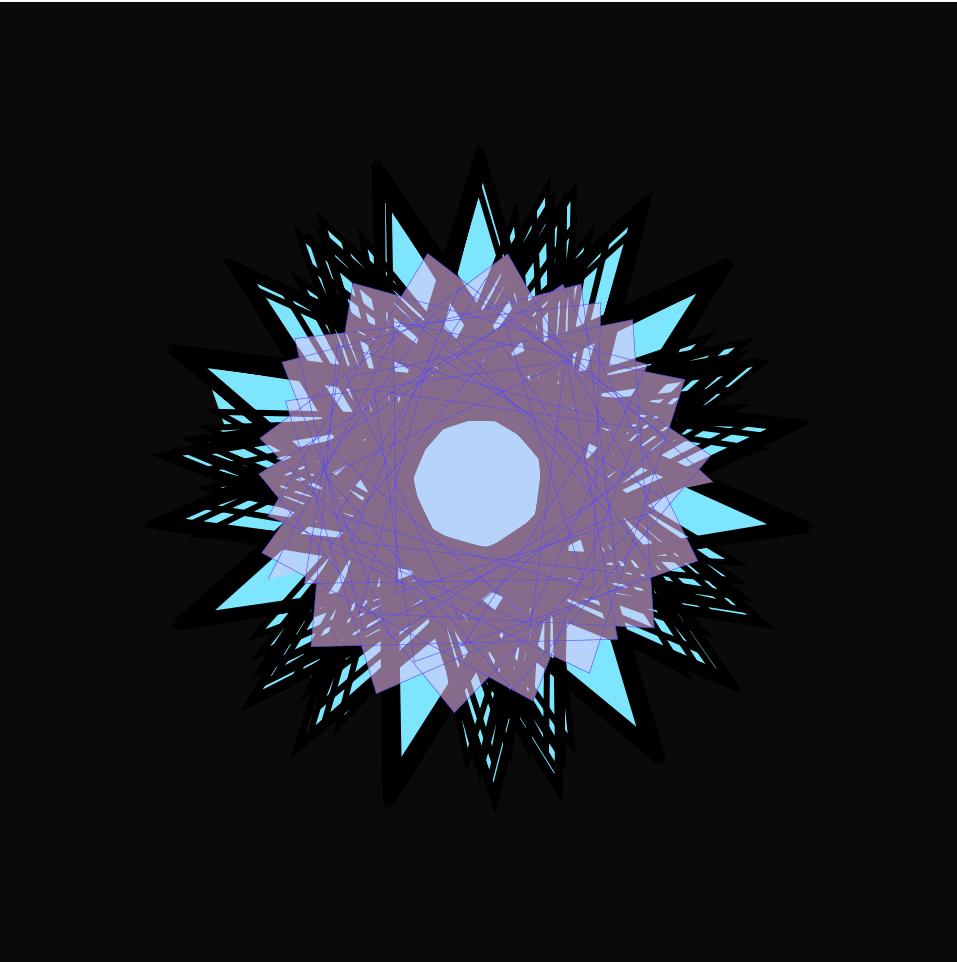
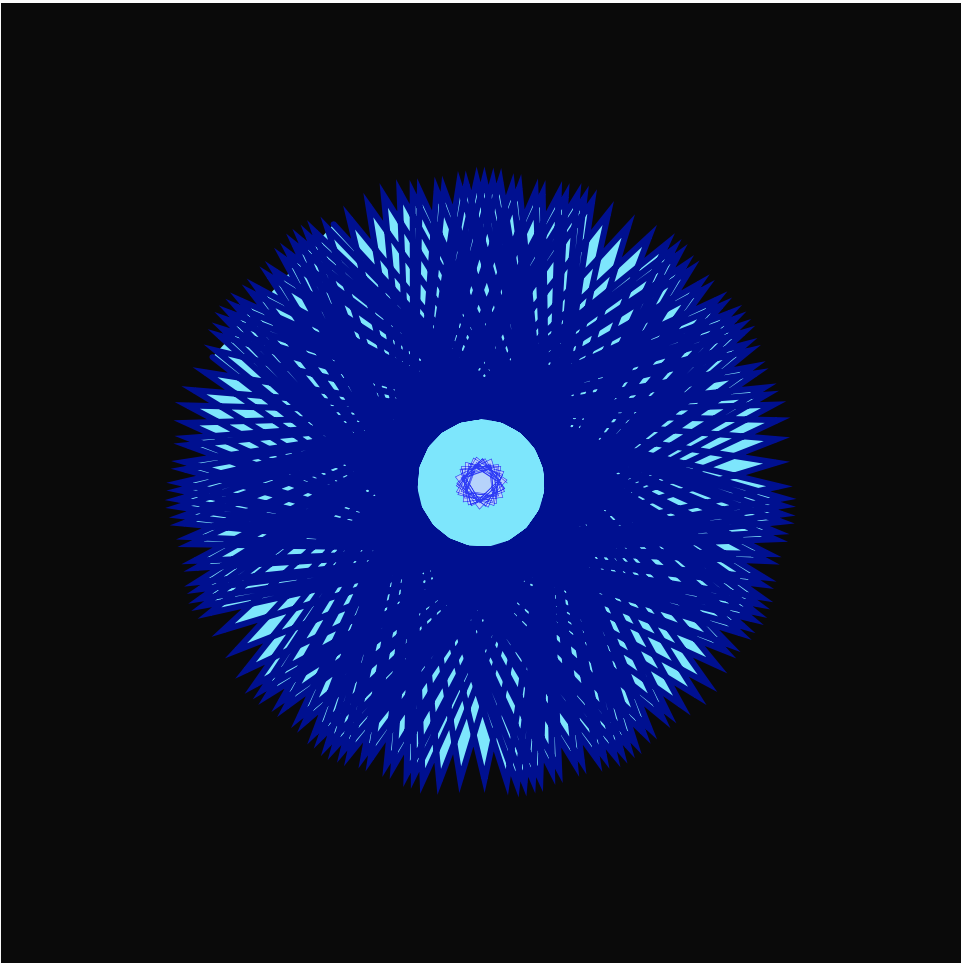
My idea for this project initially came from visuals of a rotating flower. However, throughout this process of figuring out this project, I became more interested in intricate patterns within this flower shape. And so, I was more focused on adding different color changes and perspectives when the mouse moves, to show off different designs of this form. When the mouse is somewhere near the top left edge of the canvas, I want to show the two different curves separately to represent seed-like forms, so that when the mouse moves in other directions, then the flower can gradually grow and spread to a wider view. Overall, it was very challenging but very satisfying to create patterns that remind of the kaleidoscope effect.