//Mihika Bansal
//mbansal@andrew.cmu.edu
//Section E
//Project 5
function setup() {
createCanvas(500, 500);
}
function draw() {
push();
background(250, 141, 98); // making my background canvas
translate(width / 2, height / 2);
pop();
var s = second();
var m = minute();
var h = hour();
let sAngle = map(s, 0, 60, 0, 360);
let mAngle = map(m, 0, 60, 0, 360);
let hAngle = map(h, 0, 24, 0, 360);
translate(width / 2, height / 2);
//drawing the second hand circle
fill(255);
noStroke();
push();
for (var i = 0; i < s; i ++){
rotate(radians(sAngle)); //creates a pattern based on the second that it is currently
ellipse(0, -210, 10, 10);
}
pop();
//drawing the minute hand circle
push();
fill(178, 219, 213);
for (var j = 0; j < m; j ++){
rotate(radians(mAngle)); // creates a pattern based on what minute it is
ellipse(0, -135, 18, 18);
}
pop();
//the hour hand
push();
fill(43, 97, 109);
for (var k = 0; k < h; k ++){
rotate(radians(hAngle)); //creates a pattern based on what hour it currently is, displays that number
ellipse(0, -50, 25, 25);
}
pop();
}
This project was very fun to create. Playing with animations based on time was very interesting. The patterns that formed through the integration of for loops and based on the number for minutes and seconds was very pleasing.
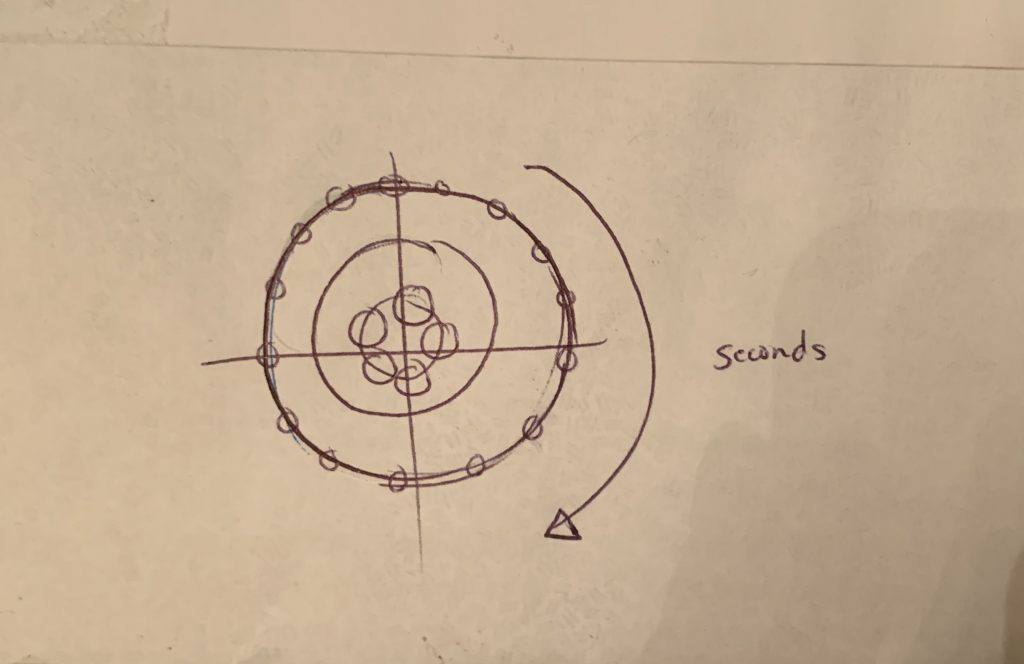