/* Charmaine Qiu
charmaiq@andrew.cmu.edu
section E
project 06, Abstract Clock */
function setup() {
createCanvas(300, 300);
}
function draw() {
background(255,200,200);
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
//variables that fits the time into certain coundaries
var poolW = map(M, 0, 59, 70, width);
var eyesH = map(H, 0, 23, 30, 1);
noStroke();
//creating the elements of the girl that does not change over time
//body
fill(252, 73, 3);
ellipse(width / 2, height, 130, 200);
//face
fill(255, 238, 227);
ellipse(width / 2 - 40, height / 2 + 20, 30, 30);
ellipse(width / 2 + 40, height / 2 + 20, 30, 30);
ellipse(width / 2, height / 2, 100, 120);
angleMode(DEGREES);
//hair
fill(245, 108, 66);
arc(width / 2, height / 2, 110, 140, -180, 360);
ellipse(100, 90, 50, 50);
ellipse(200, 90, 50, 50);
//mouth
arc(width / 2, height / 2 + 50, 40, 50, -180, 360);
//table
fill(201, 150, 113);
rect(0, 250, width, 70);
//HANGRY
fill(255, 238, 227);
//("HANGRY", width / 2 - 23, 140);
text("When is my FOOD coming?", width / 2 - 70, 40);
//when it is midnight, "HANGRY" appears on the head
if (H == 59){
text("HANGRY", width / 2 - 23, 140);
}
//drooling drops that falls down every second
push();
translate(160, 200);
m = floor(millis()) % 1000;
m = map(m, 0, 1000, 0, 200);
fill(147, 206, 237);
ellipse(0, m, 7, 7 + m * 7 / 200);
pop();
//the pool gets gets larger as a minute goes by
fill(147, 206, 237);
ellipse(width / 2, height, poolW, 50);
//the eyes becomes smaller as hours in a day pass by
fill(80);
ellipse(width / 2 - 20, height / 2 + 15, 10, eyesH);
ellipse(width / 2 + 20, height / 2 + 15, 10, eyesH);
}
This project is very interesting since I was able to design and compute a graphic that evolves around time. I took the idea of creating a literal clock further by creating a narrative to my work.
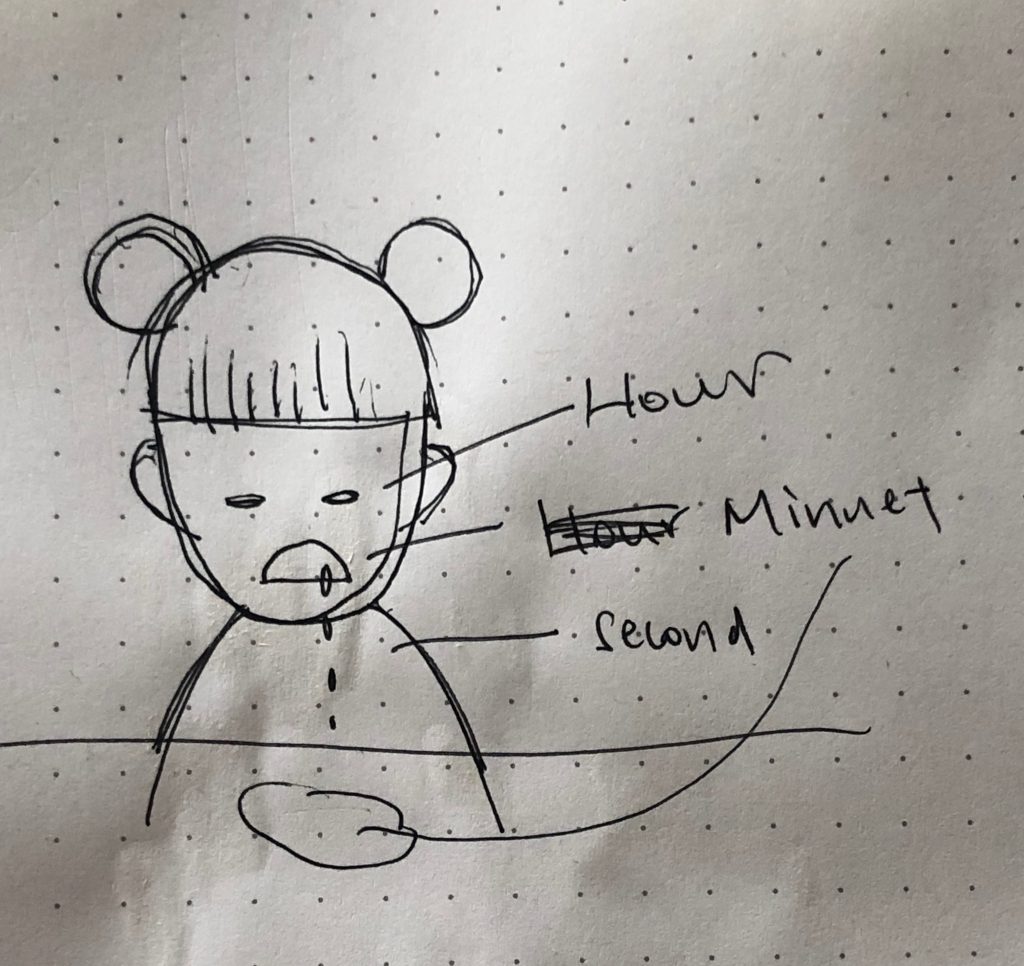