For this week’s looking outwards, I am looking at Taisai Manheim’s Looking Outwards from week 2, in which he took a look at Taxi, Taxi! Taxi, Taxi is a generative project ny Robert Hodgin. It is a digital installation in the SEC, or Samsung Experience Center, in NYC. The project focuses on NY taxis, using Open Data to create real-time simulations of all the different routes different Cabbies can take. It uses a mix of coding, and also an Open Street Map that models NYC in 3d, to create this installation. I think this is super cool to see something iconic in NYC, the yellow taxis, and have the information these Taxies generate to see the flow of life and individuality in a completely different light. Thanks for the read Tai!
Category: Uncategorized
Paul Greenway – Project 09 – Portrait
// Paul Greenway
// pgreenwa
// pgreenwa@andrew.cmu.edu
// Project-09-Portrait
var originalPortrait;
function preload() {
//original portrait image from imgur
var portraitUrl = "https://i.imgur.com/mqXKE8q.jpg";
originalPortrait = loadImage(portraitUrl);
}
function setup() {
createCanvas(700, 1200);
background(255);
originalPortrait.loadPixels();
frameRate(1000);
}
function draw() {
var px = random(width);
var py = random(height);
var circSize = random(5,20);
var ix = constrain(floor(px), 0, width-1);
var iy = constrain(floor(py), 0, height-1);
var theColorAtLocationXY = originalPortrait.get(ix, iy);
//fill circles with color based on base image
noStroke();
fill(theColorAtLocationXY);
//draw circle with dimension based on mouse position
ellipse(px, py, circSize*(mouseX*0.01), circSize*(mouseY*0.01));
}
For this project I wanted to create portrait generator that would adjust the size / resolution of the dots based on user input. While I found it hard implement the exact control over the image that I wanted, the final result was a portrait made up of circles with dimensions based on the mouse position.
Fanjie Mike Jin- Project 09- Portraits
/* Fanjie Mike Jin
fjin@andrew.cmu.edu
Section C
Project-09*/
var baseimage
function preload() {
//load in the picture of myself
var myImageURL = "https://i.imgur.com/gIuXiAy.jpg";
baseimage = loadImage(myImageURL);
}
function setup() {
//drawing the image
createCanvas(500, 500);
background(0);
baseimage.loadPixels();
// makes the pixels load faster
frameRate(1000);
}
function draw() {
//Enable mouse interactions to gerate stroke elements
var mousecolor = baseimage.get(mouseX, mouseY);
var x = random(width);
var y = random(height);
var ix = constrain(floor(x), 0, width-1);
var iy = constrain(floor(y), 0, height-1);
var color = baseimage.get(ix, iy);
noStroke();
fill(mousecolor);
//paint the canvas with the mouse using smaller ellipses
ellipse(mouseX,mouseY,random(4,20),random(4, 20));
fill(color);
//Use polygons as pixels with the randomized number of sides and dimensions
polygon(x,y,random(4,20),random(4,9));
}
//draw the polygons
function polygon(x, y, r, n) {
var angle = TWO_PI / n;
beginShape();
for (var i = 0; i < TWO_PI; i += angle) {
var a1 = x + cos(i) * r;
var a2 = y + sin(i) * r;
vertex(a1, a2);
}
endShape(CLOSE);
}
// reset the canvas to blank once the mouse is clicked
function mousePressed() {
clear();
}
In this project, I am trying to make the portrait in a rigid pointillism style as I really like the impressionist paintings. By varying the size of the randomized polygons, I am managing to simulate the feelings of that the protrait is being painted by rigid brushstrokes. Also, at the same time, I am able to make some touches to the image as the mouse interaction is enabled to digitally draw part of the portrait.
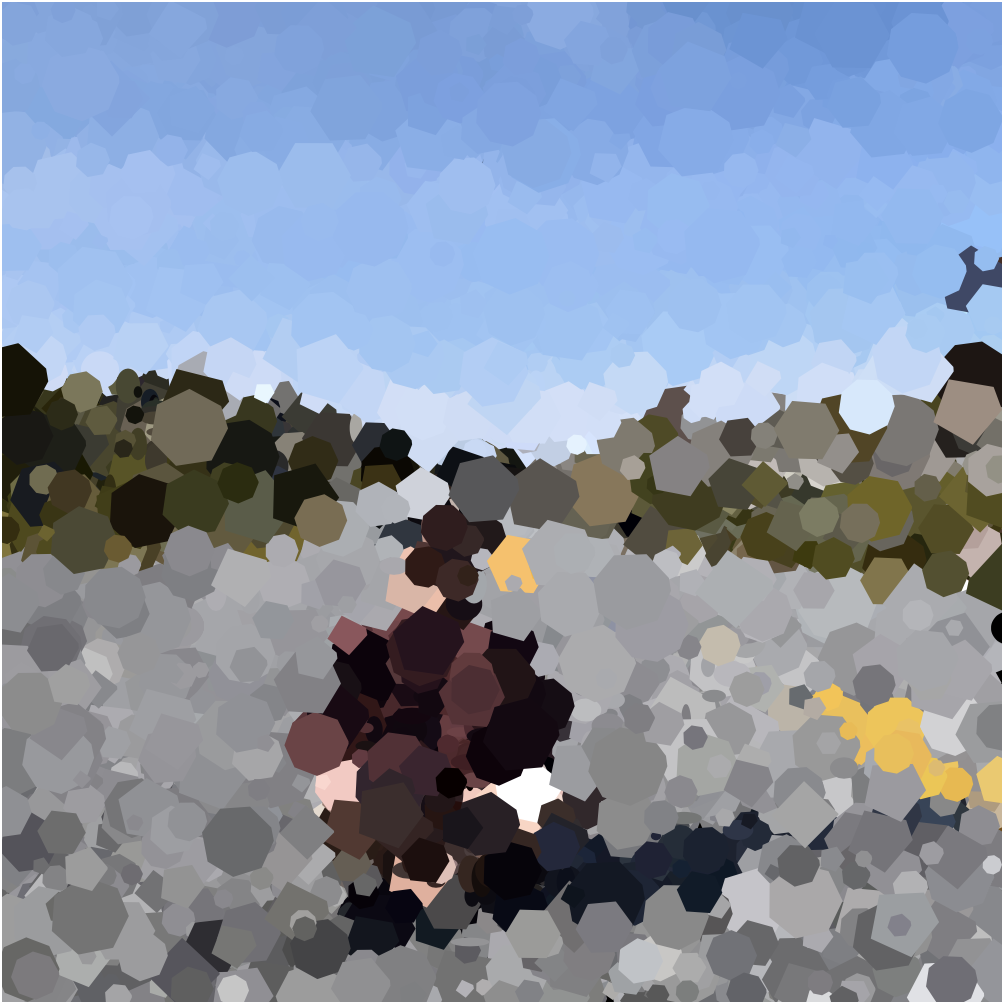
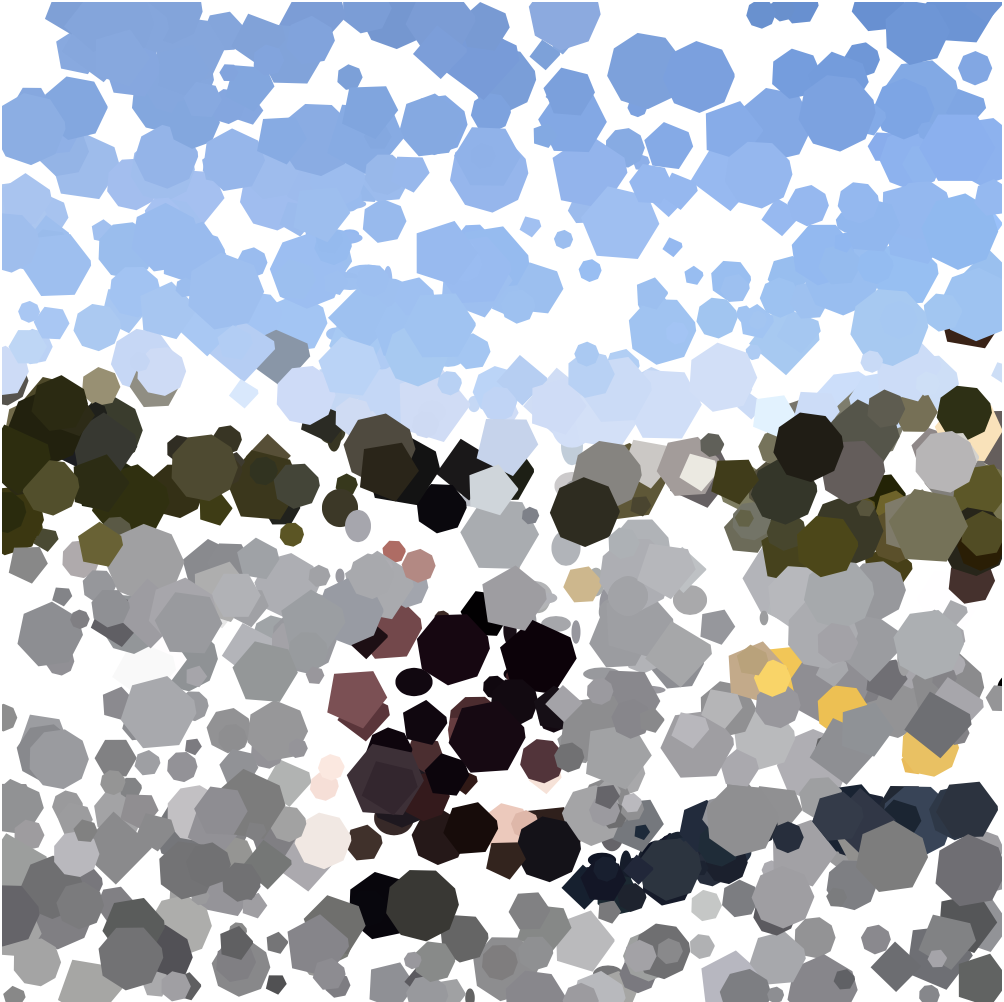
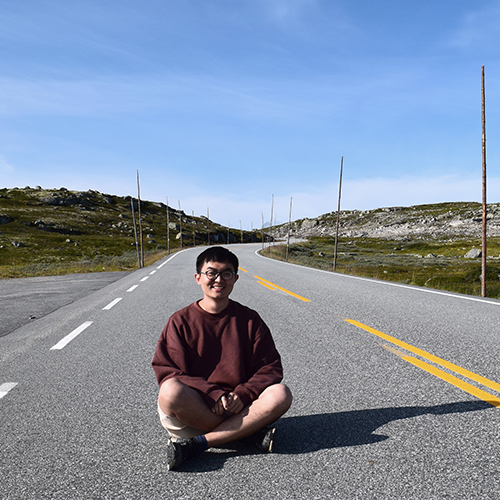
Monica Chang – Looking Outwards – 09
http://refikanadol.com/works-grid
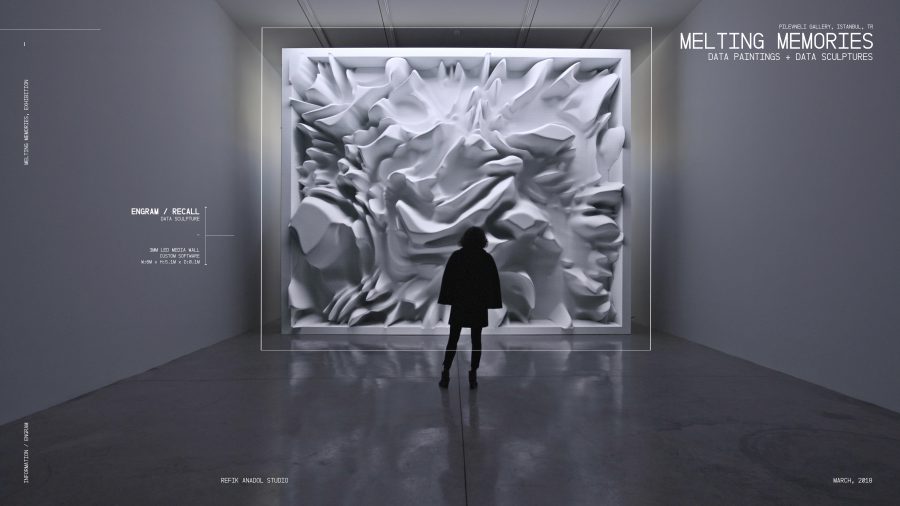
For this Looking Outwards post, I was intrigued by one of Kristine Kim’s Looking Outwards post where she looked into the artist, Refik Anadol. Something that really pulls me towards Anadol’s whole portfolio is his ability to create visuals that are able to take the viewers to an alternate universe. Kristine also articulates his ability to play around with the functionalities of that of architectural characteristics which makes sense when his pieces often take the space into account when being presented.
There is one particular piece which Kristine included in her response which was Melting Memories by Anadol which held a concept of materializing memory. It was interesting to see something so fragile and non-tactile like memory was manifested into a visual and perceptive form. With methods like this, Anadol is able to create a new world with digital processed as Kristine also mentions in her response. Anadol’s body of work consists of these ideas of materializing areas/things in the world where one would not really be able to visual or imagine in physical form and he wants to create a path towards a future that connects the digital world with the one that surrounds us already.
Claire Lee – Project 09 – Computational Portrait
/*
Claire Lee
15-104 Section B
Project-09
*/
var portraitImage;
function preload() {
var portraitImageURL = "https://i.imgur.com/yxlQW1j.jpg"
portraitImage = loadImage(portraitImageURL);
}
function setup() {
createCanvas(600, 800);
background(0);
portraitImage.loadPixels();
frameRate(100);
}
function draw() {
var px1 = random(width);
var py1 = random(height);
var px2 = px1 + random(-20, 20);
var py2 = py1 + random(-20, 20);
var px3 = px1 + random(-20, 20);
var py3 = py1 + random(-20, 20);
var px4 = px1 + random(-20, 20);
var py4 = py1 + random(-20, 20);
var ix = constrain(floor(px1), 0, width - 1);
var iy = constrain(floor(py1), 0, height - 1);
var colorAtXY = portraitImage.get(ix, iy);
noStroke();
fill(colorAtXY);
quad(px1, py1, px2, py2, px3, py3, px4, py4);
}
This portrait project was really interesting because we got to work with inserting and manipulating images from external sources. It took me a while to understand what each piece of code was meant to do, but it turned out to be fairly straightforward. I tried experimenting with different degrees of randomness for the shapes. Orignally, I was trying to go for a clean-cut geometric look, but I ended up liking the look of multi-layered small shapes better because the smaller shapes kind of resemble rough brushstrokes.
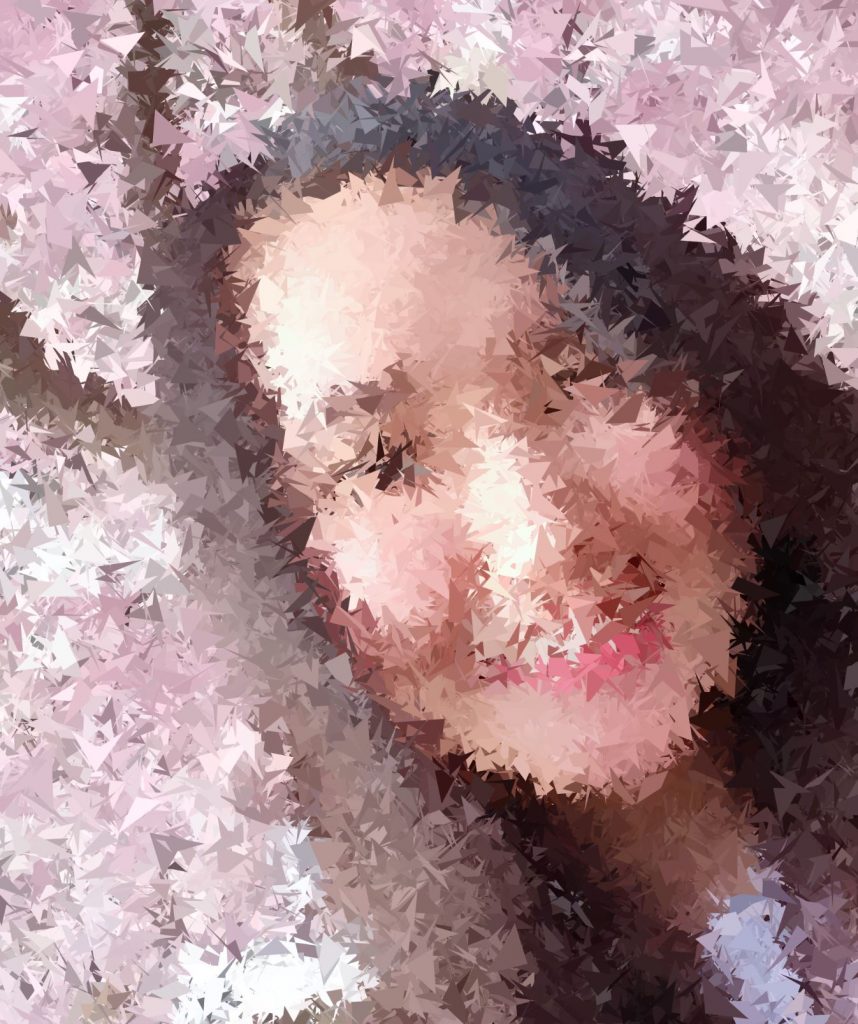
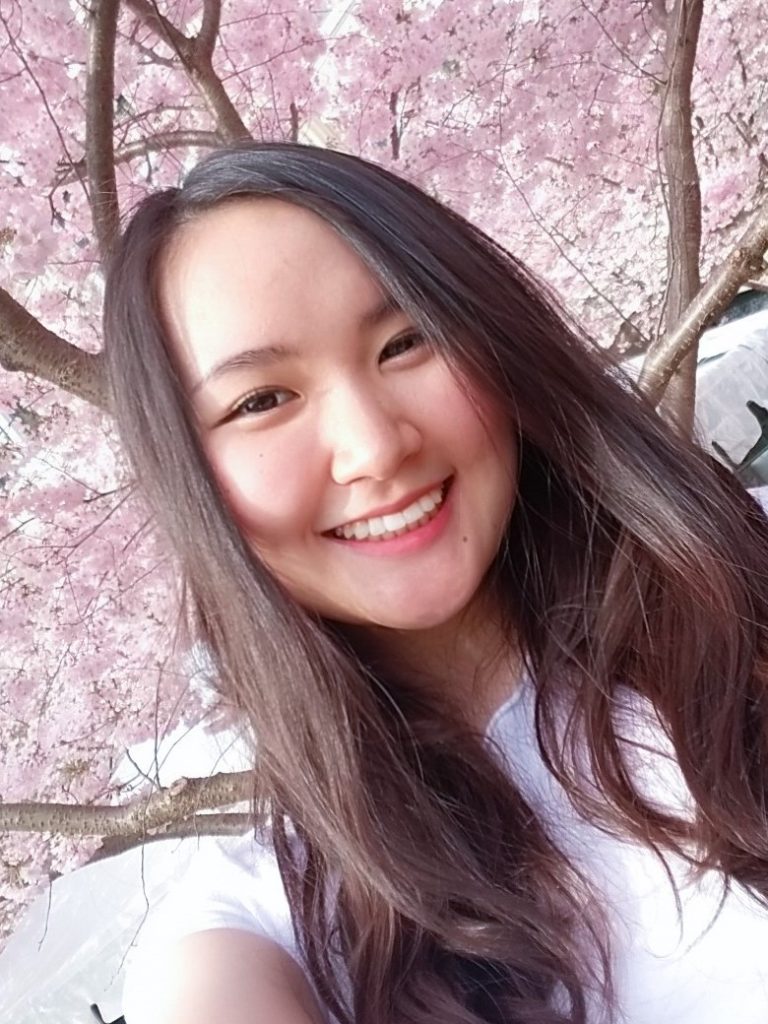
Katrina Hu – Project 09 – Computational Portrait
/*Katrina Hu
15104-C
kfh@andrew.cmu.edu
Project-09*/
var originalImage;
function preload() {
var myImageURL = "https://i.imgur.com/g3iK76T.jpg";
originalImage = loadImage(myImageURL);
}
function setup() {
createCanvas(480, 480);
background(0);
originalImage.loadPixels();
frameRate(500);
}
function draw() {
//defining the variables
var px = random(width);
var py = random(height);
var ix = constrain(floor(px), 0, width-1);
var iy = constrain(floor(py), 0, height-1);
var theColorAtLocationXY = originalImage.get(ix, iy);
//drawing the random dashes
strokeWeight(4);
stroke(theColorAtLocationXY);
line(px + random(-10, 10), py + random(-10, 10), px + random(-10, 10), py + random(-10, 10));
//drawing the random ellipses
fill(theColorAtLocationXY);
ellipse(px, py, random(1, 8), random(1, 8));
}
I chose to make a portrait of my friend Chelsea. It was fun to experiment with the random sizes and positions of my ellipses and lines.
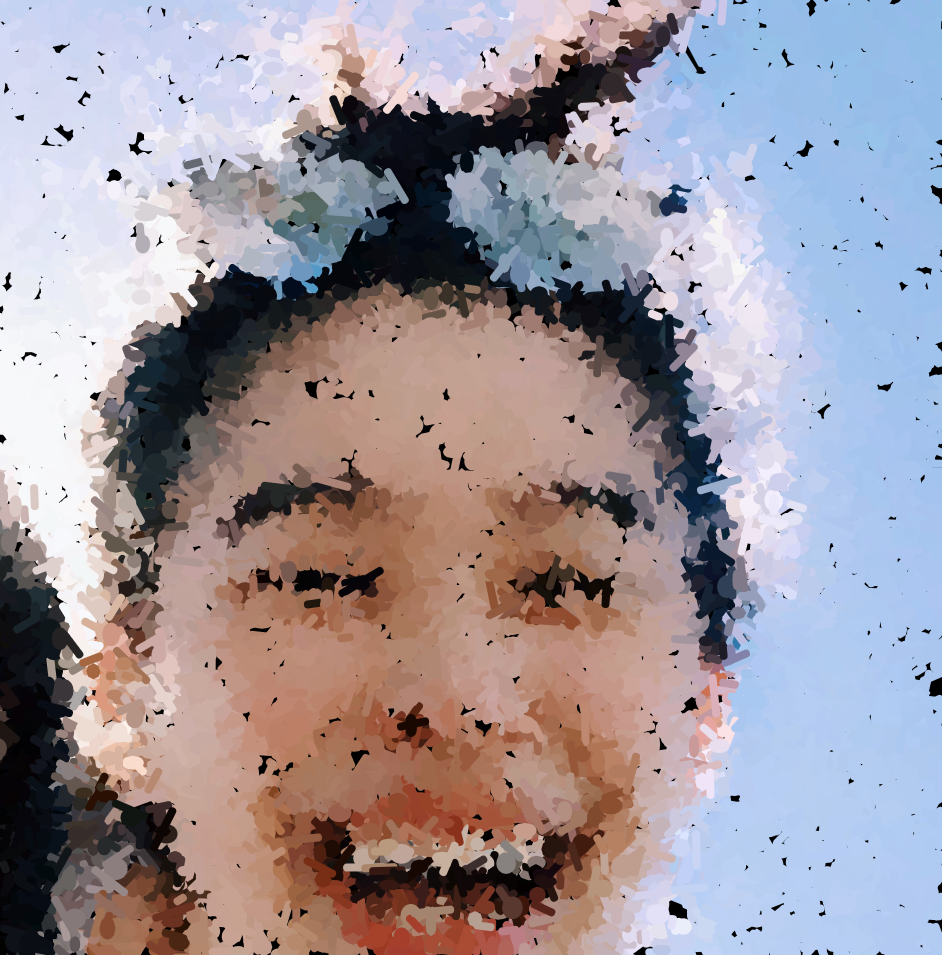
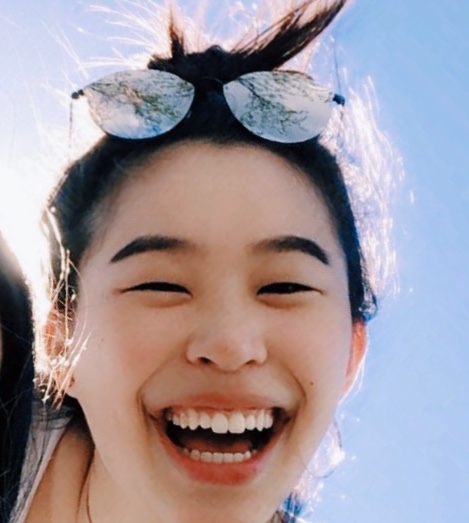
Sammie Kim – Project 09 – Portrait
//Sammie Kim
//Section D
//sammiek@andrew.cmu.edu
//Project 09 - Computational Portrait
var img;
var smallPoint;
var largePoint;
var pointSize;
//preloading image from imgur
function preload() {
img = loadImage('https://i.imgur.com/yXi77UB.jpg');
}
//setting the speed and size of loading image pixels
function setup() {
createCanvas(300, 300);
background(0);
imageMode(CENTER);
img.loadPixels();
frameRate(100);
noStroke();
smallPoint = 1;
largePoint = 10;
}
function draw() {
var x = floor(random(img.width)); //random x location for ellipse
var y = floor(random(img.height)); //random y location for ellipse
var imageColor = img.get(x, y); //picking the x, y coordinates from the image
fill(imageColor); //fill the ellipses with the color from image
//let the points get smaller toward the bottom of canvas that has more details
var pointSize = map(y, 0, height, largePoint, smallPoint);
ellipse(x, y, pointSize, pointSize);
}
This project was entertaining as I was able to recreate my own portrait by using points. Observing how the points would gradually generate the full photo, I purposely made the bottom half points tiny to better reflect the detailed areas.
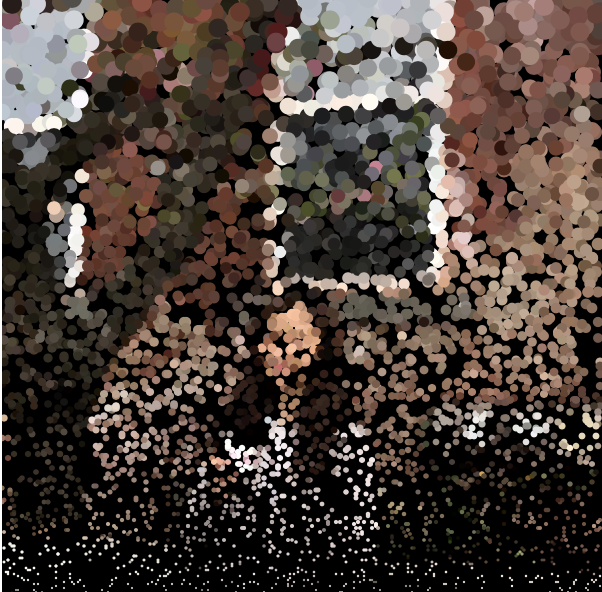
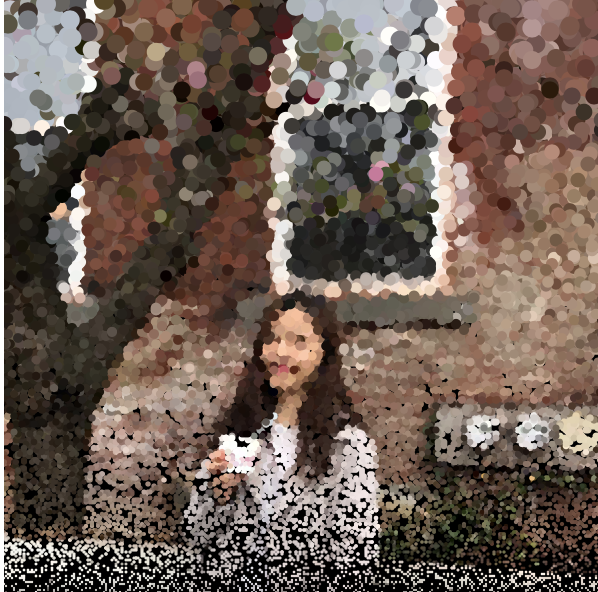
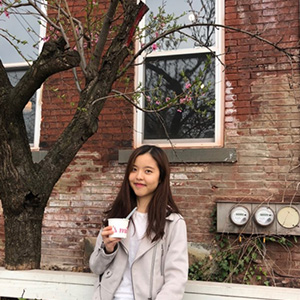
Charmaine Qiu – Looking Outwards – 09
In Jamie Park’s Looking Outwards 05, she introduced me to a 3d artist from creative cloud named Roger Kilimanjaro. He is a 3d artist from Paris, France, and he uses Maxon Cinema 4d to render his projects. He mainly creates animated videos of motions that loops over and over in a satisfying manner. On his social media pages, you can find endless short videos and images of realistic and yet unusual 3d renderings that come to life. The videos are very pleasing to look at with the attentive incorporation of popping colors and logical audios as support. At the same time, Roger utilizes his skills to create advertisements for food companies such as Krispy Kream. I agree with Jamie that it is fascinating to see how software like Maxon Cinema 4d allows a vast range of potentials for designers around the world, and it must be life changing for its developers to realize what they contributed to elevate the designers’ experience of their project making process.
Jina Lee – Looking Outwards 08
This video is of Mario Klingemann’s speech at the 2015 Eyeo Festival.
Mario Klingemann is a German artist who is known for being one of the first people to utilize computer programming with arts. His works and studies involve researching the connections between creativity, culture and perception through the perspectives of artificial intelligence and mechanical learning. He told his speech the 2015 Eyeo Festival. He discusses about his approaches towards the concept of “order” from the perspectives of a programmer and an artist. I was interested in his work with the image collections of the British Library Labs. In this work, Klingemann classified 1 million images from the British Library Lab. He did this by using machines learning techniques to define different index for each image. He created an artwork with the information he obtained. Through the assignment we just did that involves locating the brightest pixel of the image, I admire this project so much for his skills of being able to sort through such a gigantic array of information. In his presentation, Klingemann uses various images and diagrams to help people better understand and visualize the information that he is trying to communicate. As a student who is interested in communication design, I am studying how to ease the process of transfer of information between different types of people. His method of coordinately visual graphical information through data maps with his presentation really made the data-grasping task of mine as an audience a lot smoother and this is what I am trying to learn from in design too.
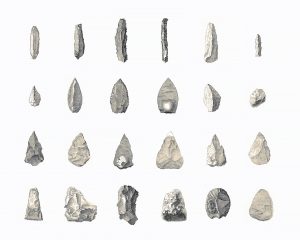
Sarah Kang- Looking Outwards-08
Darius Kazemi is a computer programmer and artist specializing in “weird internet art”. After graduating from Worcester Polytechnic Institute, he was temporarily a video game designer before co-founding a technology collective called Feel Train with Courtney Stanton. In this collective, Kazemi collaborates with other artists to create programmatic bots, such as the “StayWokeBot” and a twitter bot project called “Relive 44” which started reposting every tweet that former President Barack Obama has posted on the platform, in May of 2017.
In his festival lecture, Darius refers to his most known project, which is his Amazon shopper bot. Every month, he gives his bot a $50 amazon gift card and waits for his random packages to arrive at his doorstep. His program uses customized API values with the collaboration of Amazon and the US Postal Shipping system to create a personal shopper that buys him random books and CD’s. Essentially, his program has an output in the form of his random monthly packages. He goes on to explain how he explores the parameters of his API guidelines using Google StreetView and the resulting images.
What I admire about Darius Kazemi’s work is that he explores rudimentary and everyday elements that go unnoticed, and turns it into an opportunity for a new perspective or interest. His Amazon shopper bot would provide the opportunity to the receiver to read or listen to something they never even knew existed or something they would never purchase themselves.
Eyeo 2014 – Darius Kazemi from Eyeo Festival on Vimeo.