My process for this project was to find a curve I wanted to start with, I started working with a lemniscate and realized I can make flowers out of the infinity symbol. I wanted to make something infinity symbol related but I realized flowers are pretty and work well with the shape. I then made the sizes of the flowers change and the colors change based on the mouseX and mouseY positions.
function setup() {
createCanvas(480, 480);
}
function draw() {
background(66,49,148);
var P=color(mouseX,0,mouseY); //small flowers
var R=color(0,mouseX,mouseY); //big flower
push();
fill(P);
push();
translate(width/(4/3),height/4);
scale(.5);
rotate(radians(45));
drawCurve(); //small flower top right
push();
rotate(radians(90));
drawCurve(); //small flower top right
pop();
pop();
push();
translate(width/(4),height/4);
scale(.5);
rotate(radians(45));
drawCurve(); //small flower top left
push();
rotate(radians(90));
drawCurve(); //small flower top left
pop();
pop();
push();
translate(width/(4/3),height/(4/3));
scale(.5);
rotate(radians(45));
drawCurve(); //small flower bottom right
push();
rotate(radians(90));
drawCurve(); //small flower bottom right
pop();
pop();
push();
translate(width/(4),height/(4/3));
scale(.5);
rotate(radians(45));
drawCurve(); //small flower bottom left
push();
rotate(radians(90));
drawCurve(); //small flower bottom left
pop();
pop();
pop();
push();
fill(R);
translate(width/2,height/2);
push();
drawCurve(); //big flower
pop();
push();
rotate(radians(90));
drawCurve(); //big flower
pop();
}
function drawCurve() {
var a=constrain(mouseX/2,0,width);
strokeWeight(3.5);
beginShape();
var nPoints=360;
for(var i=0; i<nPoints; i++){
var t=map(i,0,nPoints,0,PI); //lemniscate formulas
x=(a*cos(t))/(1+sin(t)^2);
y=(a*sin(t)*cos(t))/(1+sin(t)^2);
vertex(x,y);
}
endShape(CLOSE);
push();
beginShape();
scale(.67)
var nPoints=360;
for(var i=0;i<nPoints;i++){
var r=map(i,0,nPoints,0,-PI); ///other half of lemniscate
x=(a*cos(r))/(1+sin(r)^2);
y=(a*sin(r)*cos(r))/(1+sin(r)^2);
vertex(x,y);
}
endShape(CLOSE);
pop();
}
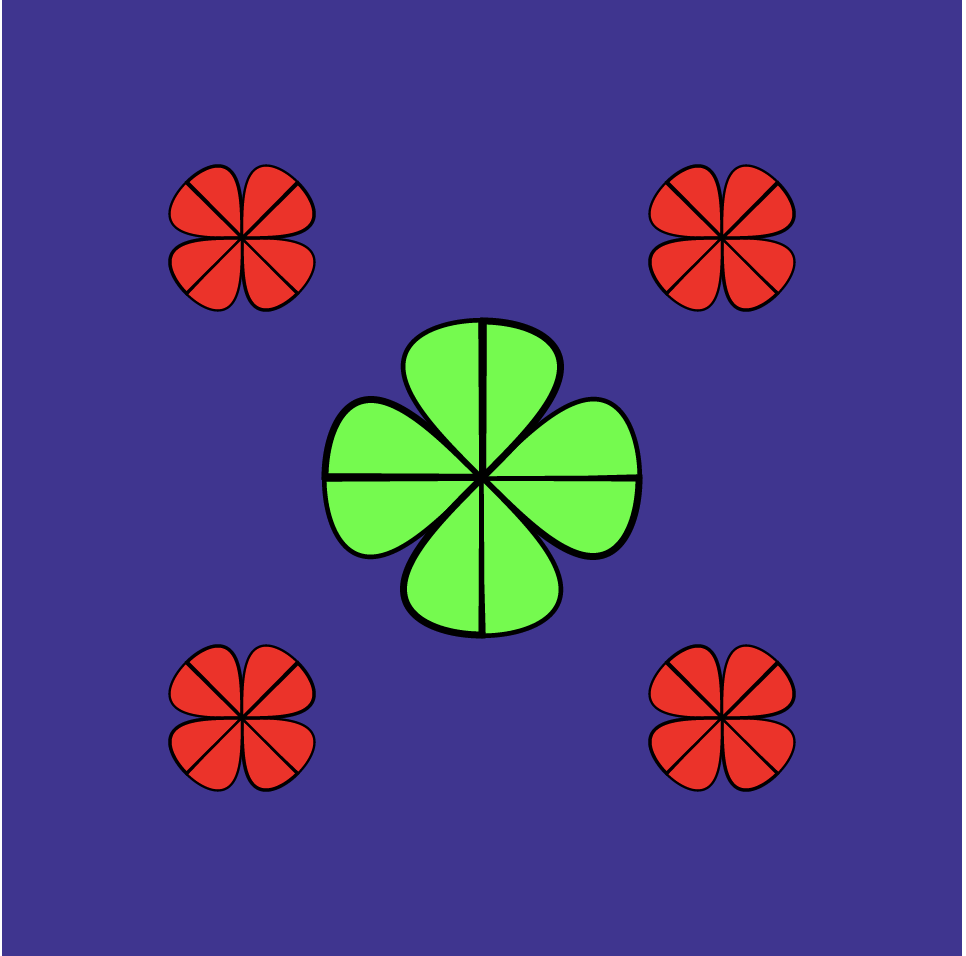
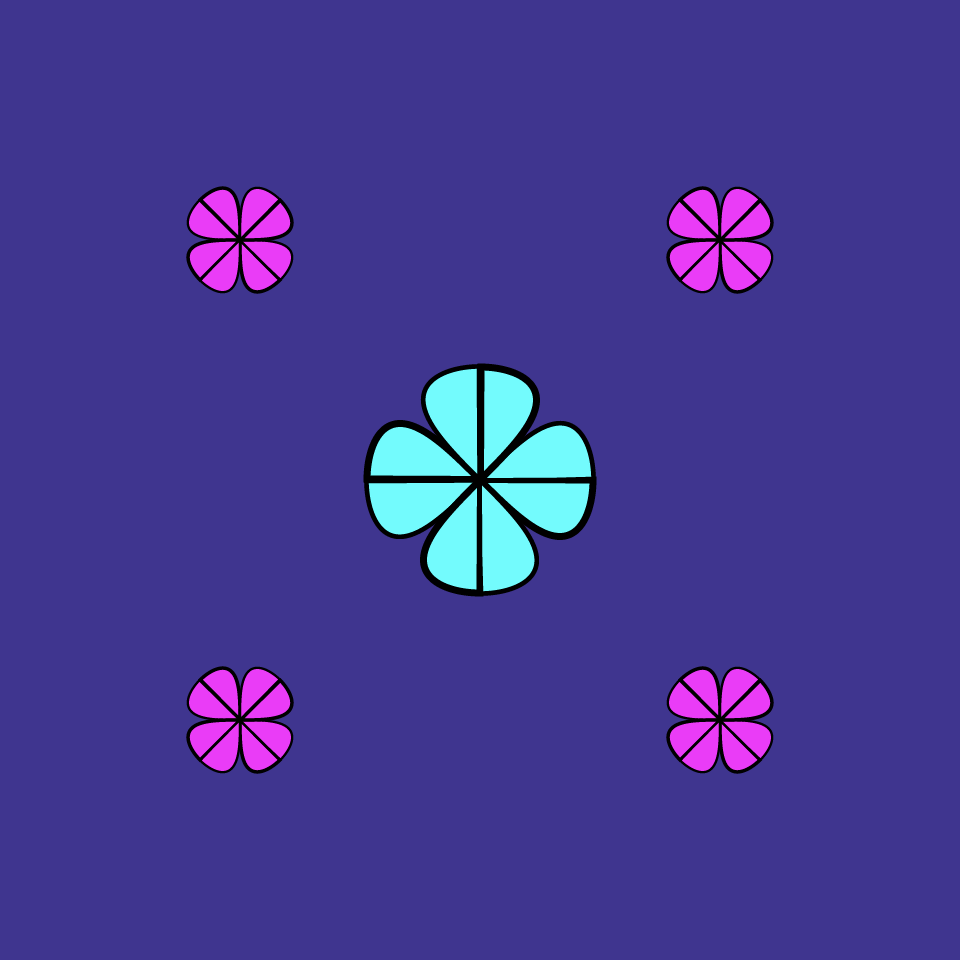
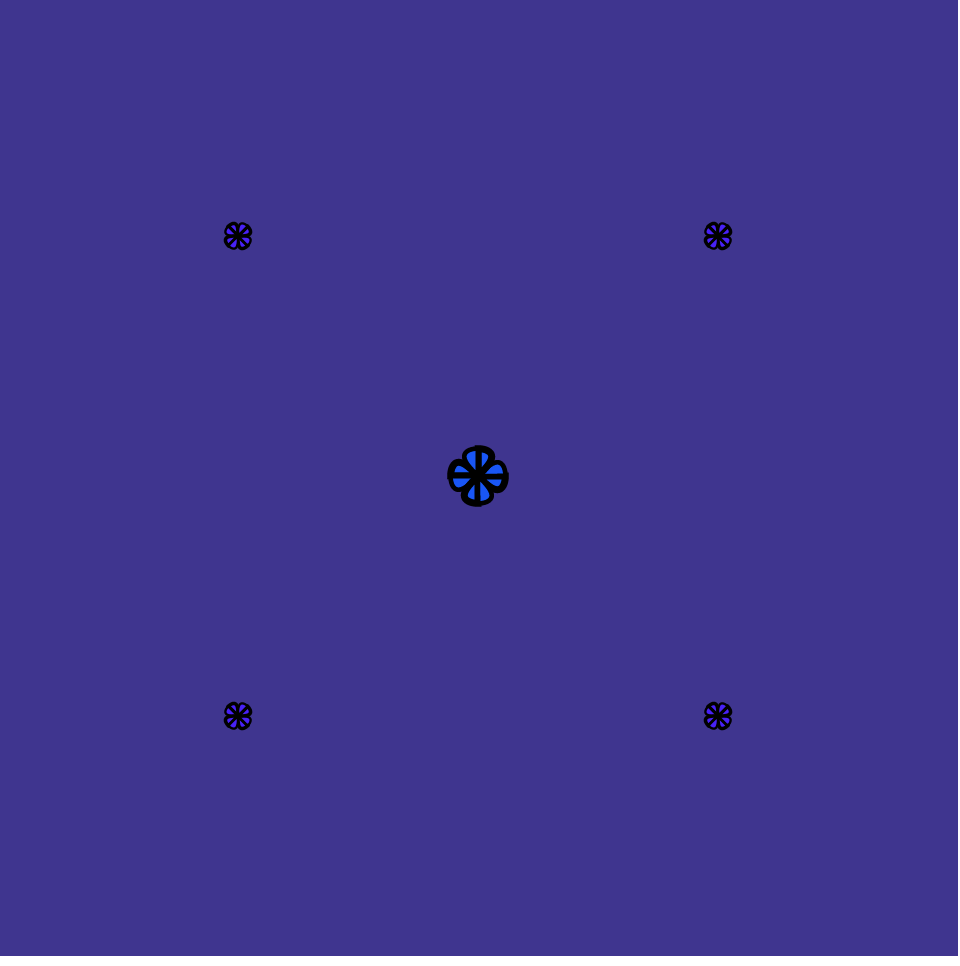
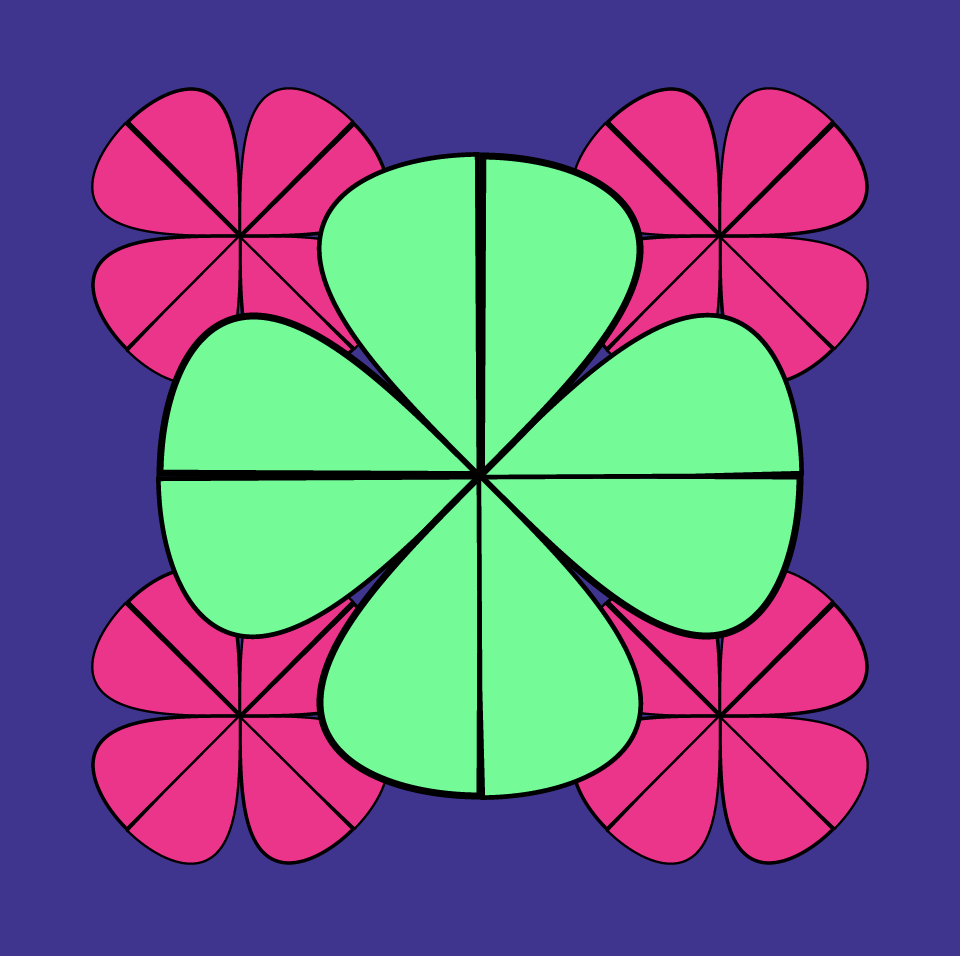