function setup() {
createCanvas(400, 400);
background(0);
noLoop();
}
function draw() {
stroke(255, 202, 141); //yellow
strokeWeight(0.5); //for diagonal lines
for (var x = 0; x <= 400; x += 50) { //draw diagonal lines
for (var y = 0; y <= 400; y += 50) {
line(x, y+height/8, x+width/8, y); // diagonal lines
line(x, y, x-width/8, y-height/8); // diagonal lines
}
}
strokeWeight(1.5); //for petals
hpetal (0, 50); //initial petal
for (var x = 0; x <= 400; x+=100) { //draw petals
for (var y = 50; y <= 450; y+=100) {
hpetal(x, y);
hpetal(x+50, y+50);
hpetal(x-50, y-50);
}
}
}
function hpetal (x, y) {
//left petal
fill(231, 193, 206); //pink
beginShape();
curveVertex(x, y);
curveVertex(x, y);
curveVertex(x+25, y-10);
curveVertex(x+50, y);
curveVertex(x+25, y+10);
curveVertex(x, y);
curveVertex(x, y);
endShape();
//right petal
fill(170, 201, 206); //pale green
beginShape();
curveVertex(x+50, y);
curveVertex(x+50, y);
curveVertex(x+75, y-10);
curveVertex(x+100, y);
curveVertex(x+75, y+10);
curveVertex(x+50, y);
curveVertex(x+50, y);
endShape();
//top petal
fill(244, 220, 208); //pale pink
beginShape();
curveVertex(x+50, y-50);
curveVertex(x+50, y-50);
curveVertex(x+60, y-25);
curveVertex(x+50, y);
curveVertex(x+40, y-25);
curveVertex(x+50, y-50);
curveVertex(x+50, y-50);
endShape();
//bottom petal
fill(170, 201, 229); //pale blue
beginShape();
curveVertex(x+50, y);
curveVertex(x+50, y);
curveVertex(x+60, y+25);
curveVertex(x+50, y+50);
curveVertex(x+40, y+25);
curveVertex(x+50, y);
curveVertex(x+50, y);
endShape();
}
Through this project, I became more comfortable with using nested loops and understood better how they work. This is my initial sketch:
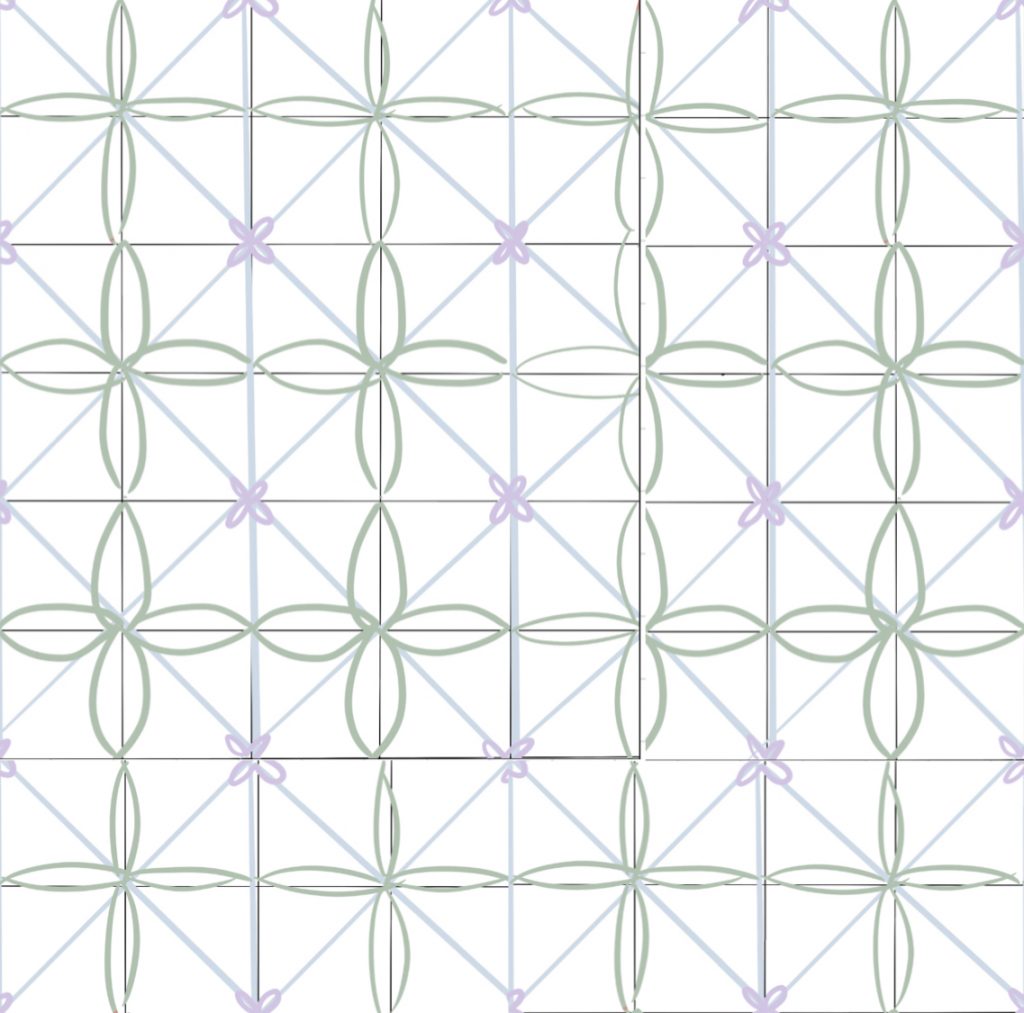
I wanted to create a wallpaper that involved these symmetrical petals, and so I started by drawing them. Then I explored a little by shifting and overlapping the petals for a more interesting effect. This is the version without the overlaps, which I quite enjoy as well:
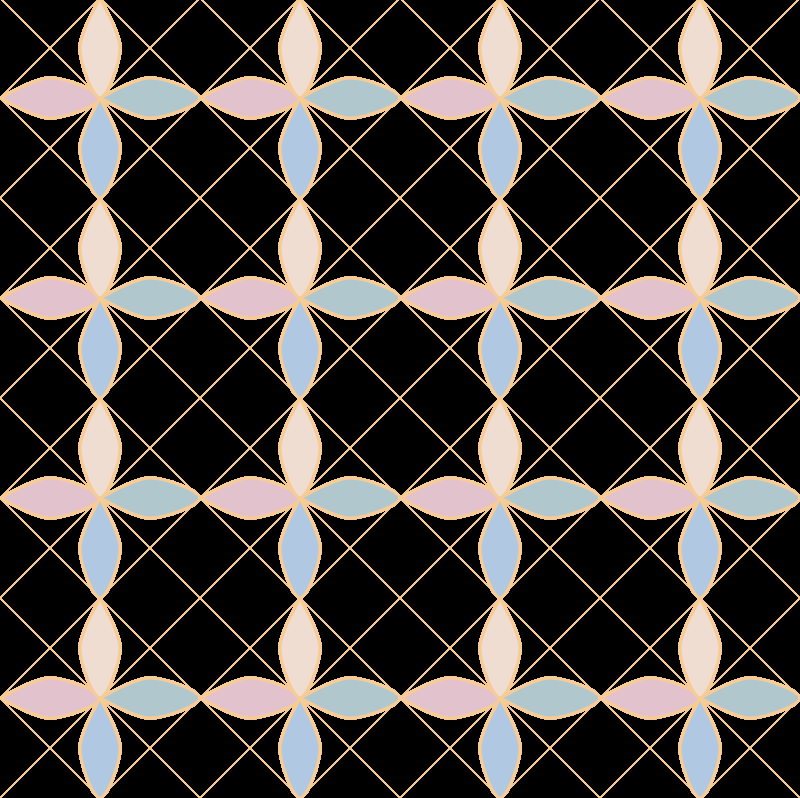
My design doesn’t quite remind me of wallpaper, instead, I see it more as a pattern for a silk square scarf. I really enjoyed testing and adding different elements to generate a pattern for this project!