This project depicts a bicycle moving along a path through different scenery. Different sound effects play as the bicycle passes through grass, birds fly by, trees and fall foliage appear, and night starts to fall.
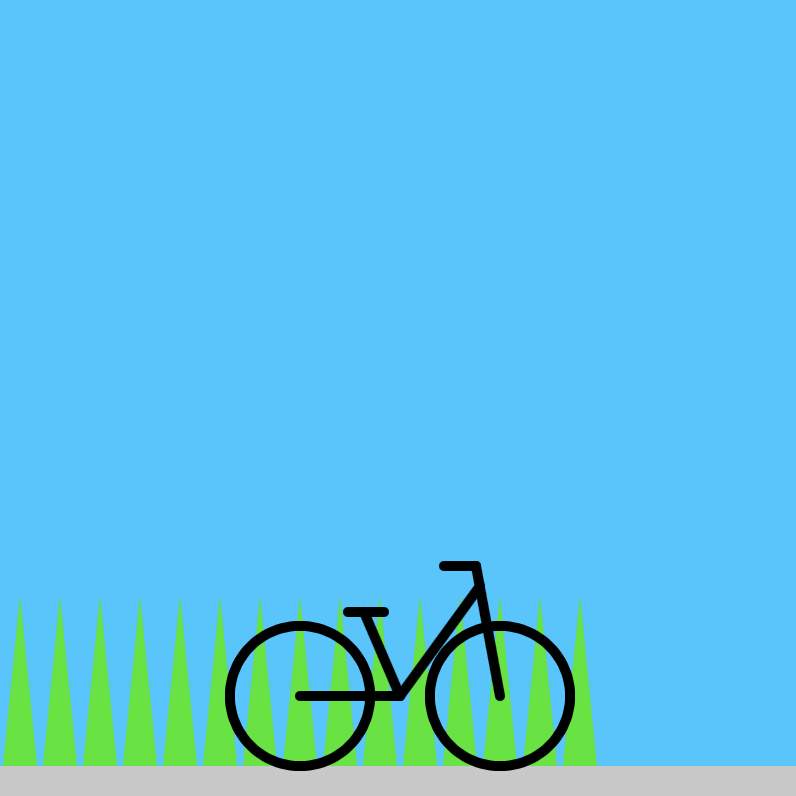
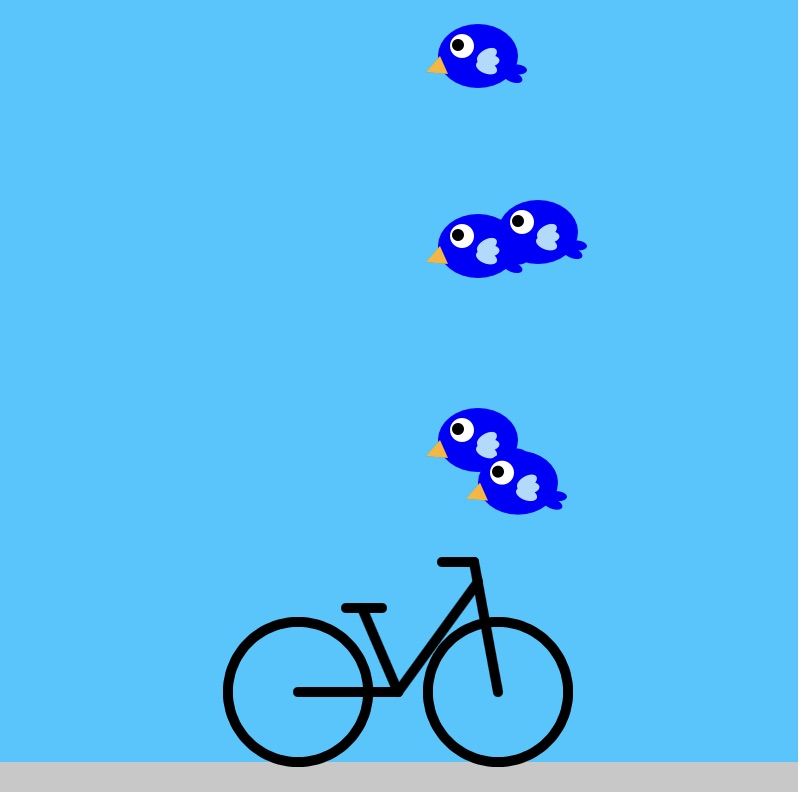
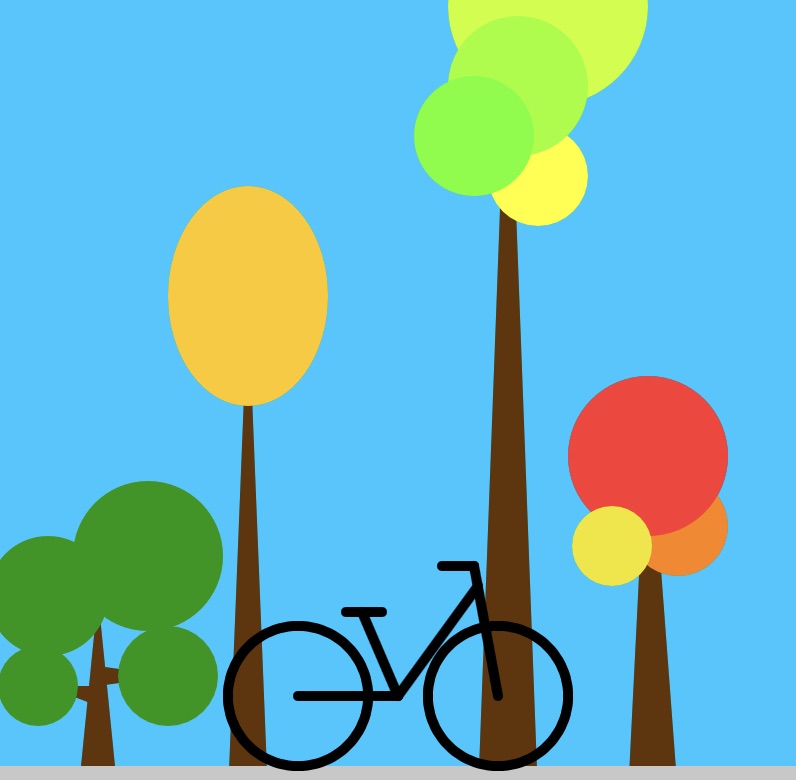
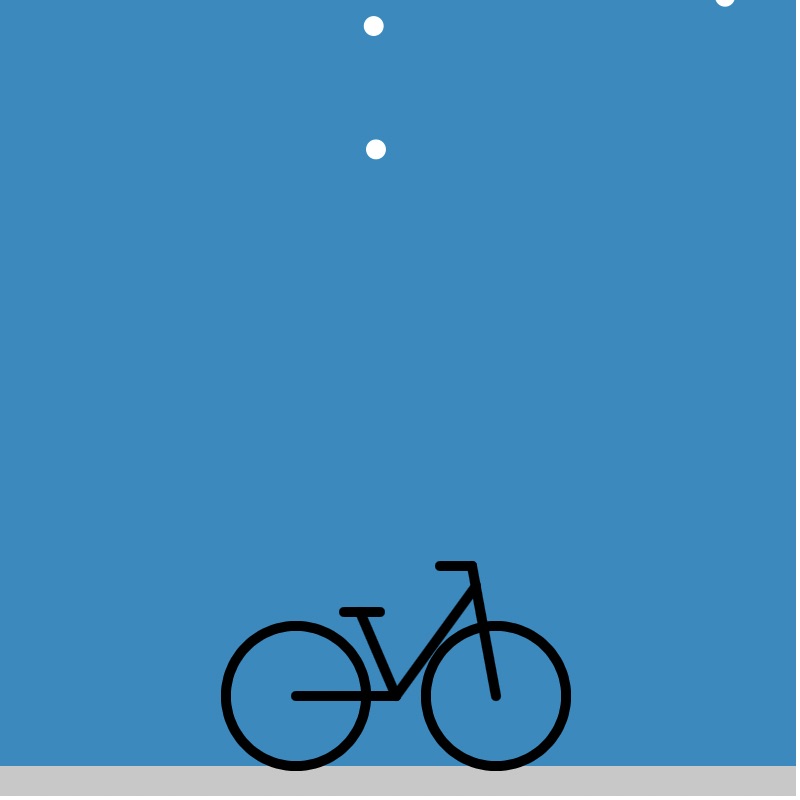
//Alana Wu
//ID: alanawu
//Project 10: Sonic Story
var birdSound;
var autumn;
var chimes;
function preload()
{
chimes = loadSound("http://localhost:8000/chimes3.wav");
birdSound = loadSound("http://localhost:8000/bird.wav");
autumn = loadSound("http://localhost:8000/autumn.wav");
chirping = loadSound("http://localhost:8000/chirping.wav");
}
function setup()
{
createCanvas(400, 400);
useSound();
frameRate(5);
}
function soundSetup()
{
birdSound.setVolume(5);
autumn.setVolume(3);
autumn.amp (.4);
chimes.setVolume(.5);
}
var x;
var y;
function draw()
{
background(0, 200, 255);
//biking through the grass, windchimes playing
if (frameCount < 40)
{
grass();
chimes.play();
}
//birds fly by and chirp
if (frameCount >= 40 & frameCount < 80)
{
bird(width + 400 - frameCount*10, frameCount*4);
bird(width + 430 - frameCount*10, height - frameCount*5);
bird(width + 400 - frameCount*10, height/2 - frameCount*3);
bird(width + 420 - frameCount*10, height/3 + frameCount*2);
bird(width + 400 - frameCount*10, 15 + frameCount*2);
if (frameCount % 8 == 0)
{
birdSound.play();
}
}
//bike through the autumn trees, wind is blowing
if (frameCount >= 80 & frameCount < 160)
{
if (frameCount % 10 == 0 && frameCount < 120)
{
autumn.play();
}
background(0, 200, 255);
trees (width + 1120 - frameCount*14, height);
}
if (frameCount >= 140)
{
night();
}
noStroke();
fill (200);
//road and bicycle
rect (0, 385, width, 20);
bicycle(150, 350);
//ends program after a certain amount of time
if (frameCount > 200)
{
noLoop();
}
}
function bicycle (x, y)
{
stroke(0);
strokeWeight(5);
noFill();
circle (x, y, 70, 70);
circle (x + 100, y, 70, 70);
line (x, y, x + 50, y);
line (x + 50, y, x + 32, y - 42);
line (x + 24, y - 42, x + 42, y - 42);
line (x + 50, y, x + 90, y - 55);
line (x + 88, y - 65, x + 100, y);
line (x + 88, y - 65, x + 72, y - 65);
}
function bird (x, y)
{
noStroke();
fill (0, 0, 255);
ellipse (x, y, 40, 32);
push();
translate(x, y);
rotate (radians(5));
ellipse(20, 5, 10, 5);
rotate (radians(15));
ellipse(20, 4, 10, 5);
//wing
fill (170, 220, 255);
ellipse(6, 4, 11, 6);
rotate(radians(-25));
ellipse(5, 3, 11, 6);
rotate(radians(-25));
ellipse(4, 2, 11, 6);
pop();
fill (255);
ellipse (x - 8, y - 5, 12, 12);
fill (0);
ellipse (x - 10, y - 5.5, 6, 6);
fill (255, 180, 30);
triangle (x - 26, y + 8, x - 19, y, x - 15, y + 9);
}
function grass()
{
fill (0, 230, 0);
noStroke();
push();
translate (400 - frameCount*20, 0);
for (var x = width; x >= 0; x -= 20)
{
triangle (x, height, x + 20, height, x + 10, height - 100);
}
pop();
}
function trees (x)
{
//green tree
noStroke();
fill (100, 50, 0);
triangle (x - 10, height, x + 10, height, x, height - 100);
triangle (x - 26, height - 55, x, height - 55, x, height - 45);
triangle (x + 35, height - 60, x, height - 65, x, height - 55);
fill (0, 150, 0);
circle (x - 25, height - 100, 60);
circle (x + 25, height - 120, 75);
circle (x + 35, height - 60, 50);
circle (x - 30, height - 55, 40);
//yellow tree
x += 75;
fill (100, 50, 0);
triangle (x - 10, height, x + 10, height, x, height - 250);
fill (255, 200, 0);
ellipse (x, height - 250, 80, 110);
//red orange yellow tree
x += 200;
fill (100, 50, 0);
triangle (x - 10, height, x + 15, height, x, height - 200);
fill (255, 130, 0);
circle (x + 15, height - 135, 50);
fill (255, 50, 50);
circle (x, height - 170, 80);
fill (240, 230, 0);
circle (x - 18, height - 125, 40);
//tallest tree
x -= 75;
fill (100, 50, 0);
triangle (x + 20, height, x-10, height, x + 5, 0);
fill (255, 255, 0);
circle (x + 20, 90, 50);
fill (200, 255, 0);
circle (x + 25, 5, 100);
fill (150, 255, 0);
circle (x + 10, 45, 70);
fill (100, 255, 0);
circle (x-12, 70, 60);
}
//sky becomes night time, stars come out
function night()
{
if (frameCount % 15 == 0)
{
chirping.play();
}
background (0, 200 - (frameCount - 140), 255 - (frameCount-140));
fill (255);
noStroke();
if (frameCount > 160)
{
circle (random(width),random(height), 10);
}
circle (random(width),random(height), 10);
circle (random(width),random(height), 10);
}