KEY – NOTE NAME
A STRING:
1 – A
2 – B
3 – C#
4 – D
5 – E
D STRING:
Q – D
W – E
E – F#
R – G
T – A
G STRING:
A – G
S – A
D – B
F – C
G – D
C STRING:
Z – C
X – D
C – E
V – F
B – G
My final project is a playable viola that creates art in the background based on the notes played and length of the notes played. I drew the viola utilizing arcs, triangles, lines, and other 2D shapes. Then I added sounds to the notes played and ellipses that move with the music.
Each note is connected to a key, that when pressed, plays a note and a visual representation of the sound as a green dot showing the placement of the note on the fingerboard. In addition, there are 20 uniquely colored balls hiding behind the viola that are connected to 20 notes that can be played with the keyboard. Each ball will move at its individual vertical and horizontal velocities when the key is pressed. When the key is lifted, the sound will stop and ball will stop in place. When the performer is finished playing, it will leave a pattern of balls in the background. With the end of each unique composition, the background will look unique, linking each unique piece of music with a unique piece of art.
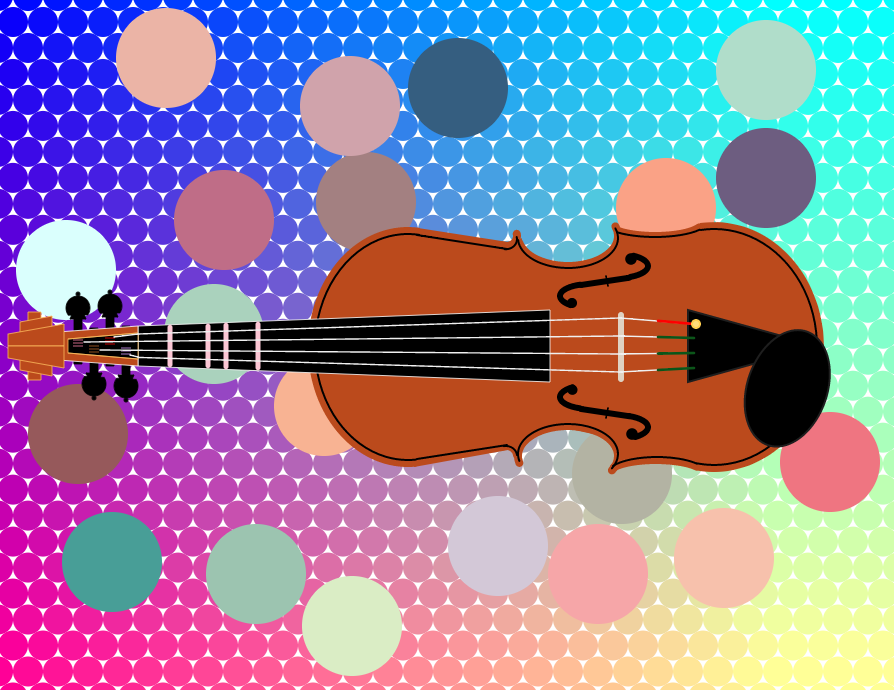
Note: WordPress does not display the audio correctly, so here is a zip file with the audio files and functioning code. In order to run this code, you access it in the terminal and type “python -m SimpleHTTPServer” and the code will run properly. Above is a screenshot of the completely functioning code.
Make sure to turn the volume up!
//Angela Rubin
//Section C
//aerubin@andrew.cmu.edu
//Final Project
//Initial Placement of Balls in Background
var x = 300;
var y = 175;
//Speed of Balls in both x and y directions
var dx = -1;
var dy = 2;
var x1 = 300;
var y1 = 175;
var dx1 = 2;
var dy1 = 1;
var x2 = 300;
var y2 = 175;
var dx2 = -1;
var dy2 = 1;
var x3 = 300;
var y3 = 175;
var dx3 = 2;
var dy3 = -2;
var x4 = 300;
var y4 = 175;
var dx4 = 2;
var dy4 = 3;
var x5 = 300;
var y5 = 175;
var dx5 = -3;
var dy5 = 2;
var x6 = 300;
var y6 = 175;
var dx6 = 3;
var dy6 = -3;
var x7 = 300;
var y7 = 175;
var dx7 = -3;
var dy7 = 4;
var x8 = 300;
var y8 = 175;
var dx8 = 4;
var dy8 = 3;
var x9 = 300;
var y9 = 175;
var dx9 = -4;
var dy9 = 4;
var x10 = 300;
var y10 = 175;
var dx10 = -1;
var dy10 = -2;
var x11 = 300;
var y11 = 175;
var dx11 = -2;
var dy11 = -1;
var x12 = 300;
var y12 = 175;
var dx12 = -2;
var dy12 = -2;
var x13 = 300;
var y13 = 175;
var dx13 = -2;
var dy13 = -3;
var x14 = 300;
var y14 = 175;
var dx14 = -3;
var dy14 = -2;
var x15 = 300;
var y15 = 175;
var dx15 = -3;
var dy15 = -4;
var x16 = 300;
var y16 = 175;
var dx16 = -4;
var dy16 = -3;
var x17 = 300;
var y17 = 175;
var dx17 = -1;
var dy17 = 2;
var x18 = 300;
var y18 = 175;
var dx18 = -2;
var dy18 = 3;
var x19 = 300;
var y19 = 175;
var dx19 = 3;
var dy19 = -2;
//Sound Names
var EonAString;
var DonAString;
var ConAString;
var BonAString;
var AonString;
var AonString2;
var GonDString;
var FonDString;
var EonDString;
var DonString;
var DonString2;
var ConGString;
var BonGString;
var AonGString;
var GonString;
var GonString2;
var FonCString;
var EonCString;
var DonCString;
var ConString;
//Background Pattern Variables
var diameter = 15; // diameter of the circles
var horizSpace = diameter; // horizontal spacing of the circles
var verSpace; // vertical spacing of the circles
function setup() {
createCanvas(450, 350);
verSpace = horizSpace * sqrt(0.75);
}
function preload() {
//Preloads each sound for each note
EonAString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/E-A_String.m4a");
DonAString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/D-A_String.m4a");
ConAString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/C-A_String.m4a");
BonAString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/B-A_String.m4a");
AonString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/A-String.m4a");
AonString2 = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/A-String2.m4a");
GonDString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/G-D_String.m4a");
FonDString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/F-D_String.m4a");
EonDString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/E-D_String.m4a");
DonString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/D-String.m4a");
DonString2 = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/D-String2.m4a");
ConGString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/C-G_String.m4a");
BonGString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/B-G_String.m4a");
AonGString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/A-G_String.m4a");
GonString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/G-String.m4a");
GonString2 = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/G-String2.m4a");
FonCString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/F-C_String.m4a");
EonCString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/E-C_String.m4a");
DonCString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/D-C_String.m4a");
ConString = loadSound("https://courses.ideate.cmu.edu/15-104/f2017/wp-content/uploads/2017/12/C-String.m4a");
}
function draw() {
background(255);
noStroke();
for(var i = 0; i < 30; i++) {
if (i%2==1) {var c = -1;}
if (i%2==0) {c = 0;}
for(var n = 0; n < 35+c; n++) {
fill(i*10, n*10, 255-(i*4));
ellipse(horizSpace*n+(-7.5*c), verSpace*i, diameter, diameter);
}
}
//Drawing the Balls in Background
fill(248, 179, 147);
ellipse(x, y, 50, 50);
fill(239, 117, 129);
ellipse(x1, y1, 50, 50);
fill(191, 109, 135);
ellipse(x2, y2, 50, 50);
fill(109, 93, 128);
ellipse(x3, y3, 50, 50);
fill(53, 94, 128);
ellipse(x4, y4, 50, 50);
fill(156, 196, 176);
ellipse(x5, y5, 50, 50);
fill(176, 221, 202);
ellipse(x6, y6, 50, 50);
fill(218, 237, 197);
ellipse(x7, y7, 50, 50);
fill(246, 166, 168);
ellipse(x8, y8, 50, 50);
fill(150, 89, 91);
ellipse(x9, y9, 50, 50);
fill(163, 128, 129);
ellipse(x10, y10, 50, 50);
fill(170, 210, 189);
ellipse(x11, y11, 50, 50);
fill(218, 255, 253);
ellipse(x12, y12, 50, 50);
fill(208, 163, 171);
ellipse(x13, y13, 50, 50);
fill(247, 193, 172);
ellipse(x14, y14, 50, 50);
fill(72, 158, 151);
ellipse(x15, y15, 50, 50);
fill(235, 180, 166);
ellipse(x16, y16, 50, 50);
fill(211, 200, 215);
ellipse(x17, y17, 50, 50);
fill(250, 162, 134);
ellipse(x18, y18, 50, 50);
fill(179, 179, 163);
ellipse(x19, y19, 50, 50);
//Viola Function to Draw Viola
makeViola();
//Color of Dots on Fingerboard
fill(0, 255, 0);
noStroke();
//B Natural, A String, KEY = 2
if (keyIsDown(50)) {
BonAString.play();
ellipse(86, (height/2)-6.7, 4, 4);
y+=dy;
x+=dx;
if(y>height-25 || y<25) {
dy=-dy;
}
if(x>width-25 || x<25) {
dx=-dx;
}
}
else {BonAString.stop();}
//C#, A String, KEY = 3
if (keyIsDown(51)) {
ConAString.play();
ellipse(105, (height/2)-7, 4, 4);
y1+=dy1;
x1+=dx1;
if(y1>height-25 || y1<25) {
dy1=-dy1;
}
if(x1>width-25 || x1<25) {
dx1=-dx1;
}
}
else {ConAString.stop();}
//D Natural, A String, KEY = 4
if (keyIsDown(52)) {
DonAString.play();
ellipse(114, (height/2)-7.3, 4, 4);
y2+=dy2;
x2+=dx2;
if(y2>height-25 || y2<25) {
dy2=-dy2;
}
if(x2>width-25 || x2<25) {
dx2=-dx2;
}
}
else {DonAString.stop();}
//E Natural, A String, KEY = 5
if (keyIsDown(53)) {
EonAString.play();
ellipse(130, (height/2)-7.8, 4, 4);
y3+=dy3;
x3+=dx3;
if(y3>height-25 || y3<25) {
dy3=-dy3;
}
if(x3>width-25 || x3<25) {
dx3=-dx3;
}
}
else {EonAString.stop();}
//E Natural, D String, KEY = W
if (keyIsDown(87)) {
EonDString.play();
ellipse(86, (height/2)-2.2, 4, 4);
y4+=dy4;
x4+=dx4;
if(y4>height-25 || y4<25) {
dy4=-dy4;
}
if(x4>width-25 || x4<25) {
dx4=-dx4;
}
}
else {EonDString.stop();}
//F#, D String, KEY = E
if (keyIsDown(69)) {
FonDString.play();
ellipse(105, (height/2)-2.3, 4, 4);
y5+=dy5;
x5+=dx5;
if(y5>height-25 || y5<25) {
dy5=-dy5;
}
if(x5>width-25 || x5<25) {
dx5=-dx5;
}
}
else {FonDString.stop();}
//G Natural, D String, KEY = R
if (keyIsDown(82)) {
GonDString.play();
ellipse(114, (height/2)-2.5, 4, 4);
y6+=dy6;
x6+=dx6;
if(y6>height-25 || y6<25) {
dy6=-dy6;
}
if(x6>width-25 || x6<25) {
dx6=-dx6;
}
}
else {GonDString.stop();}
//A Natural, D String, KEY = T
if (keyIsDown(84)) {
AonString.play();
ellipse(130, (height/2)-2.9, 4, 4);
y7+=dy7;
x7+=dx7;
if(y7>height-25 || y7<25) {
dy7=-dy7;
}
if(x7>width-25 || x7<25) {
dx7=-dx7;
}
}
else {AonString.stop();}
//A Natural, A String, KEY = 1
if (keyIsDown(49)) {
AonString2.play();
y16+=dy16;
x16+=dx16;
if(y16>height-25 || y16<25) {
dy16=-dy16;
}
if(x16>width-25 || x16<25) {
dx16=-dx16;
}
}
else {AonString2.stop();}
//A Natural, G String, KEY = S
if (keyIsDown(83)) {
AonGString.play();
ellipse(86, (height/2)+2.2, 4, 4);
y8+=dy8;
x8+=dx8;
if(y8>height-25 || y8<25) {
dy8=-dy8;
}
if(x8>width-25 || x8<25) {
dx8=-dx8;
}
}
else {AonGString.stop();}
//B Natural, G String, KEY = D
if (keyIsDown(68)) {
BonGString.play();
ellipse(105, (height/2)+2.3, 4, 4);
y9+=dy9;
x9+=dx9;
if(y9>height-25 || y9<25) {
dy9=-dy9;
}
if(x9>width-25 || x9<25) {
dx9=-dx9;
}
}
else {BonGString.stop();}
//C Natural, G String, KEY = F
if (keyIsDown(70)) {
ConGString.play();
ellipse(114, (height/2)+2.5, 4, 4);
y10+=dy10;
x10+=dx10;
if(y10>height-25 || y10<25) {
dy10=-dy10;
}
if(x10>width-25 || x10<25) {
dx10=-dx10;
}
}
else {ConGString.stop();}
//D Natural, G String, KEY = G
if (keyIsDown(71)) {
DonString.play();
ellipse(130, (height/2)+2.9, 4, 4);
y11+=dy11;
x11+=dx11;
if(y11>height-25 || y11<25) {
dy11=-dy11;
}
if(x11>width-25 || x11<25) {
dx11=-dx11;
}
}
else {DonString.stop();}
//D Natural, D String, KEY = Q
if (keyIsDown(81)) {
DonString2.play();
y17+=dy17;
x17+=dx17;
if(y17>height-25 || y17<25) {
dy17=-dy17;
}
if(x17>width-25 || x17<25) {
dx17=-dx17;
}
}
else {DonString2.stop();}
//D Natural, C String, KEY = X
if (keyIsDown(88)) {
DonCString.play();
ellipse(86, (height/2)+6.3, 4, 4);
y12+=dy12;
x12+=dx12;
if(y12>height-25 || y12<25) {
dy12=-dy12;
}
if(x12>width-25 || x12<25) {
dx12=-dx12;
}
}
else {DonCString.stop();}
//E Natural, C String, KEY = C
if (keyIsDown(67)) {
EonCString.play();
ellipse(105, (height/2)+6.7, 4, 4);
y13+=dy13;
x13+=dx13;
if(y13>height-25 || y13<25) {
dy13=-dy13;
}
if(x13>width-25 || x13<25) {
dx13=-dx13;
}
}
else {EonCString.stop();}
//F Natural, C String, KEY = V
if (keyIsDown(86)) {
FonCString.play();
ellipse(114, (height/2)+7, 4, 4);
y14+=dy14;
x14+=dx14;
if(y14>height-25 || y14<25) {
dy14=-dy14;
}
if(x14>width-25 || x14<25) {
dx14=-dx14;
}
}
else {FonCString.stop();}
//G Natural, C String, KEY = B
if (keyIsDown(66)) {
GonString.play();
ellipse(130, (height/2)+7.5, 4, 4);
y15+=dy15;
x15+=dx15;
if(y15>height-25 || y15<25) {
dy15=-dy15;
}
if(x15>width-25 || x15<25) {
dx15=-dx15;
}
}
else {GonString.stop();}
//G Natural, G String, KEY = A
if (keyIsDown(65)) {
GonString2.play();
y18+=dy18;
x18+=dx18;
if(y18>height-25 || y18<25) {
dy18=-dy18;
}
if(x18>width-25 || x18<25) {
dx18=-dx18;
}
}
else {GonString2.stop();}
//C Natural, C String, KEY = Z
if (keyIsDown(90)) {
ConString.play();
y19+=dy19;
x19+=dx19;
if(y19>height-25 || y19<25) {
dy19=-dy19;
}
if(x19>width-25 || x19<25) {
dx19=-dx19;
}
}
else {ConString.stop();}
}
function makeViola() {
stroke(255);
strokeWeight(.4);
push();
noStroke();
strokeWeight(1);
fill(187, 74, 28);
//Upper Bout
arc(205, (height/2)+.5, 100, 120, 1.5, 4.81, CHORD);
//Lower Bout
arc(358, (height/2)+.5, 110, 125, 4.6, 8, CHORD);
quad(210, (height/2)-59, 208, (height/2)+60.5, 255, (height/2)+52, 255, (height/2)-52);
noFill();
strokeWeight(4);
stroke(187, 74, 28);
//little curve
arc(254, (height/2)-55, 11, 10, -.2, 2);
arc(254, (height/2)+55, 11, 10, 4.3, 6.2);
//Waist of Viola
arc(285, (height/2)-55, 50, 30, -.2, 3);
arc(285, (height/2)+55, 50, 30, 3, 6.6);
//lower bout
arc(328.5, (height/2)-62, 48, 14, .12, 2.9);
arc(329, (height/2)+64, 48, 14, 3.3, 6.1);
fill(187, 74, 28);
//filling in sparce areas
quad(252, (height/2)-48, 252, (height/2)+48, 260, (height/2)+51, 260, (height/2)-51);
quad(260, (height/2)-45, 260, (height/2)+47, 280, (height/2)+38, 280, (height/2)-36);
quad(279, (height/2)-38, 279, (height/2)+38, 307, (height/2)+44, 306, (height/2)-43);
quad(305, (height/2)-44, 306, (height/2)+46, 313, (height/2)+52, 313, (height/2)-52);
quad(312, (height/2)-53, 312, (height/2)+55, 333, (height/2)+53, 333, (height/2)-52);
quad(332, (height/2)-53, 332, (height/2)+53, 352, (height/2)+58, 352, (height/2)-58);
//Black Outline of Body
strokeWeight(1);
stroke(0);
noFill();
arc(205, (height/2)+.5, 100-7, 120-7, 1.5, 4.81);
arc(358, (height/2)+.5, 110-7, 125-7, 4.6, 8);
line(209, (height/2)-59+3, 254, (height/2)-52+3);
line(209, (height/2)+59-3, 254, (height/2)+52-3);
arc(254, (height/2)-55+1.6, 11, 10, -.2, 1.7);
arc(254, (height/2)+55, 11, 10, 4.5, 6.2);
arc(285, (height/2)-54, 50, 30, -.2, 3.1);
arc(285, (height/2)+54, 50, 30, 3, 6.6);
arc(328.5, (height/2)-62, 52, 15, .17, 2.88);
arc(329.5, (height/2)+64-.5, 49, 16, 3.3, 6.05);
pop();
//finger board
fill(0);
quad(80-10, (height/2)-10, 80-10, (height/2)+10, 250+26, (height/2)+18, 250+26, (height/2)-18);
//Pegs
stroke(0);
quad(38, (height/2)+9, 38+5-1, (height/2)+9, 38+5-1, (height/2)-17, 38, (height/2)-17);
quad(38+8, (height/2)+9+8, 38+5+8-1, (height/2)+9+8, 38+5+8-1, (height/2)-17+8, 38+8, (height/2)-17+8);
quad(38+16, (height/2)+9+1, 38+5+16-1, (height/2)+9+1, 38+5+16-1, (height/2)-17+1, 38+16, (height/2)-17+1);
quad(38+24, (height/2)+9+7, 38+5+24-1, (height/2)+9+7, 38+5+24-1, (height/2)-17+7, 38+24, (height/2)-17+7);
//Peg Ends
fill(0);
arc(40, (height/2)-20+1, 12, 12, 2.5, 6.9, CHORD);
arc(40+16, (height/2)-20, 12, 12, 2.5, 6.9, CHORD);
arc(40+8, (height/2)+20-1, 12, 12, 4+3-1.3, 3+.85, CHORD);
arc(40+24, (height/2)+20, 12, 12, 4+3-1.3, 3+.85, CHORD);
//Peg Dots
ellipse(40, (height/2)-26, 2, 2);
ellipse(40+16, (height/2)-26-1, 2, 2);
ellipse(40+8, (height/2)+26, 2, 2);
ellipse(40+24, (height/2)+26+1, 2, 2);
//Peg Triangles
triangle(36, (height/2)-13.5, 44, (height/2)-13.5, 40, (height/2)-23);
triangle(36+16, (height/2)-13.5-1, 44+16, (height/2)-13.5-1, 40+16, (height/2)-23-1);
triangle(36+8, (height/2)+13.5, 44+8, (height/2)+13.5, 40+8, (height/2)+23);
triangle(36+24, (height/2)+13.5+1, 44+24, (height/2)+13.5+1, 40+24, (height/2)+23+1);
stroke(255);
//peg box
fill(187, 74, 28);
quad(70, (height/2)-10, 70, (height/2)+10, 30, (height/2)+7, 30, (height/2)-7);
stroke(252, 185, 91);
//Black Section of Peg Box
fill(0);
quad(35, (height/2)-3.5, 35, (height/2)+3.5, 70, (height/2)+6.5, 70, (height/2)-6.5);
line(35, (height/2)-3.5, 70, (height/2)-6.5);
line(35, (height/2)+3.5, 70, (height/2)+6.5);
push();
//String colors in peg box
stroke(250, 127, 164); //pink
line(37.5, (height/2)-3, 42.5, (height/2)-3);
line(37.5, (height/2)-1.5, 42.5, (height/2)-1.5);
line(37.5, (height/2), 42.5, (height/2));
stroke(180, 101, 40); //brown
line(37.5+8, (height/2)-3+3, 42.5+8, (height/2)-3+3);
line(37.5+8, (height/2)-1.5+3, 42.5+8, (height/2)-1.5+3);
line(37.5+8, (height/2)+3, 42.5+8, (height/2)+3);
stroke(255, 0, 0); //red
line(37.5+16, (height/2)-3-1, 42.5+16, (height/2)-3-1);
line(37.5+16, (height/2)-1.5-1, 42.5+16, (height/2)-1.5-1);
line(37.5+16, (height/2)-1, 42.5+16, (height/2)-1);
stroke(216, 154, 253); //purple
line(37.5+8+16, (height/2)-3+3+1, 42.5+8+16, (height/2)-3+3+1);
line(37.5+8+16, (height/2)-1.5+3+1, 42.5+8+16, (height/2)-1.5+3+1);
line(37.5+8+16, (height/2)+3+1, 42.5+8+16, (height/2)+3+1);
pop();
fill(187, 74, 28);
//Inner Most Scroll Piece
quad(15, (height/2)-18+1, 15, (height/2), 43-18-3.5, (height/2), 43-18-3.5, (height/2)-18+1);
quad(15, (height/2)+18-1, 15, (height/2), 43-18-3.5, (height/2), 43-18-3.5, (height/2)+18-1);
//Middle Scroll Piece
quad(13-2, (height/2)-13+1, 13-2, (height/2), 43-18+2, (height/2), 43-18+2, (height/2)-16+1);
quad(13-2, (height/2)+13-1, 13-2, (height/2), 43-18+2, (height/2), 43-18+2, (height/2)+16-1);
//Outer Most Scroll Piece
quad(14-9, (height/2)-6, 14-9, height/2, 43-10, height/2, 43-10, (height/2)-11);
quad(14-9, (height/2)+6, 14-9, height/2, 43-10, height/2, 43-10, (height/2)+11);
//Bridge
stroke(223, 210, 194);
strokeWeight(3);
line(311, (height/2)-16, 311, (height/2)+16);
stroke(30);
strokeWeight(1);
//Tail piece
fill(0);
triangle(345, (height/2)-18, 345, (height/2)+18, 410, (height/2));
//Chin rest
push();
rotate(radians(19));
ellipse(437, (height/2)-118, 40, 60);
pop();
push();
stroke(251, 198, 212);
strokeWeight(2.5);
line(86, (height/2)-9.5, 86, (height/2)+9.5);
line(105, (height/2)-10, 105, (height/2)+10);
line(114, (height/2)-10.5, 114, (height/2)+10.5);
line(130, (height/2)-11, 130, (height/2)+11);
pop();
//Strings
stroke(240);
strokeWeight(.7);
line(58, (height/2)-4.5, 70, (height/2)-6); //Upper A String
line(70, (height/2)-6, 311, (height/2)-14); //A String
line(43, (height/2)-2, 311, (height/2)-5); //D String
line(51, (height/2)+2, 311, (height/2)+4); //G String
line(70, (height/2)+5.7, 311, (height/2)+13); //C String
line(66, (height/2)+4.5, 70, (height/2)+5.5); //Upper C String
line(311, (height/2)-14, 348, (height/2)-11); //Lower A String
line(311, (height/2)-5, 348, (height/2)-4); //Lower D String
line(311, (height/2)+4, 348, (height/2)+3.5); //Lower G String
line(311, (height/2)+13, 348, (height/2)+11); //Lower C String
stroke(255, 0, 0); //Red String Ends
strokeWeight(1.3);
line(330, (height/2)-12.6, 348, (height/2)-11);
stroke(11, 85, 25); //Green String Ends
line(330, (height/2)-4.5, 348, (height/2)-4);
line(330, (height/2)+3.8, 348, (height/2)+3.5);
line(330, (height/2)+12, 348, (height/2)+11);
//Fine Tuner
strokeWeight(1);
stroke(255, 206, 79);
fill(255, 220, 123);
ellipse(349, (height/2)-11, 4, 4);
stroke(0);
strokeWeight(3);
noFill();
//Upper F Hole
arc(296, (height/2)-25, 30, 12, 2.7, 4);
line(291, (height/2)-31, 315, (height/2)-34.6);
arc(310, (height/2)-40, 30, 12, 5.7, 7);
//Lower F Hole
arc(296, (height/2)+25.5, 30, 12, 2.3, 3.65);
line(291, (height/2)+31, 315, (height/2)+34.6);
arc(310, (height/2)+40.6, 30, 12, 5.5, 6.8);
//little lines on F Holes
strokeWeight(.8);
line(305, (height/2)-30, 304, (height/2)-35);
line(305, (height/2)+31, 304, (height/2)+36);
//Dots on F Holes
fill(0);
ellipse(287, (height/2)-21.5, 4.3, 4.3);
ellipse(287.2, (height/2)+21.9, 4.3, 4.3);
ellipse(316.5, (height/2)-43.5, 5, 5);
ellipse(317, (height/2)+44, 5, 5);
}