For my project I mainly based my project on whack a mole game combined with the pokemon that everyone loves, Diglet. The game lasts for 60 sec and during time the player would want to hit as many diglets as possible. Once the game ends the user can press r to restart the game. Hope you enjoy the game!
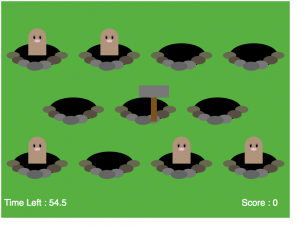
//Alvin Luk
//Section A
//akluk@andrew.cmu.edu
//Final Project
//initialize constants and variables
//number of rows of diglets in the canvas
var rows = 3;
//body height of the diglet
var diglet_h = 30;
//number of diglets in the game
var num_diglets = 11;
//array to contain the diglet objects
var diglets = [];
//temporary dummie variable to help push all the diglets
var temp_diglet;
//number of diglets the player has hit in a single run of game/score
var num_hit;
//number of 0.5 sec passed elapsed
var time_elapsed = 0;
//duration of game 60sec
var game_time = 120;
//whether the game has ended
var ended = 0;
//hammer mode to determine if hammer is down or up
var mode;
//start position for even rows
var startx1 = 60;
//start position for odd row
var startx2 = 120;
//spacing with each diglet
var spacing = 120;
//height spacing
var dy = 90;
function setup() {
createCanvas(480, 360);
//creates the diglets in the right positions
// and pushes it into the array of diglets
for (var i = 0; i < rows; i++) {
//even rows
if ((i % 2) == 0){
for (var j = startx1; j < width; j += spacing){
temp_diglet = new diglet(j,dy*(i+1));
diglets.push(temp_diglet);
}
}
//odd rows
else {
for (var j = startx2; j < width; j += spacing){
temp_diglet = new diglet(j,dy*(i+1));
diglets.push(temp_diglet);
}
}
}
//initialize score for player
num_hit = 0;
//update the diglets ever half second to determine if they pop up
//and update timer every 0.5 sec
setInterval(function(){
for (var index = 0; index < num_diglets; index ++){
diglets[index].update();
}
time_elapsed += 1;
}, 500);
}
function draw() {
background(40,180,40);
noStroke();
//change the display of the hammer
if (mouseIsPressed){
mode = 1;
}
else {
mode = 0;
}
//end the game when the timer has ended
if (time_elapsed >= game_time){
ended = 1;
}
//if game has not ended, draw the holes for the diglets in the correct location
if (ended == 0){
for (var i = 0; i < rows; i++) {
//odd rows
if ((i % 2) == 0){
for (var j = 60; j < width; j += 120){
drawHole(j,dy*(i+1));
}
}
//even rows
else {
for (var j = 120; j < width; j += 120){
drawHole(j,dy*(i+1));
}
}
}
//draw every diglet in the array of diglets
for (var index = 0; index < num_diglets; index ++){
diglets[index].draw();
}
//draw hammer in passive position
if (mode == 0) {
fill(130,82,1);
rect(mouseX-5,mouseY-25,10,50);
fill(120);
rect(mouseX-25,mouseY-35, 50,20);
}
//draw hammer in active position
else {
fill(130,82,1);
rect(mouseX,mouseY-5,50,10);
fill(120);
rect(mouseX-10,mouseY-25, 20,50);
}
//display score and time left for the game
fill(255);
textSize(15);
text("Score : " + num_hit, 400,340);
text("Time Left : " + (game_time-time_elapsed)/2,5,340);
}
//game over screen
else {
fill(0);
rect(0,0,width,height);
fill(255);
text("Game Over!", width/2 - width/10, height/2);
text("Final Score : " + num_hit, width/2 - width/10,height/2 + height/15);
text("Press R to restart", width/2 - width/10, height/2 + 2*height/15);
}
}
//draw the hole sorry for much random numbers to make it look natural
function drawHole(x,y){
fill(0);
ellipse(x,y,80,40);
fill(103,104,76);
ellipse(x-40,y,22,12);
ellipse(x+40,y,22,12);
fill(90,77,65);
ellipse(x-32,y+8,20,12);
ellipse(x+28,y+8,24,14);
fill(119,120,119);
ellipse(x-22,y+13,20,12);
ellipse(x+12,y+16,24,15);
fill(100,100,100);
ellipse(x-4,y+18,28,15);
}
//object of diglet
function diglet(x,y,duration){
//base variables
this.x = x;
this.y = y;
this.duration = duration;
//draw the diglet, with eyes and body
this.draw = function() {
if (this.duration > 0) {
//brown of diglet
fill(181,147,119);
rect(this.x-diglet_h/2,this.y-diglet_h,diglet_h,diglet_h);
ellipse(this.x,this.y-diglet_h,diglet_h,diglet_h);
fill(0);
ellipse(this.x-diglet_h/6,this.y-diglet_h,3,6);
ellipse(this.x+diglet_h/6,this.y-diglet_h,3,6);
fill(255);
ellipse(this.x-diglet_h/6,this.y-diglet_h-2,1,1);
ellipse(this.x+diglet_h/6,this.y-diglet_h-2,1,1);
//nose color
fill(239,187,210);
ellipse(this.x,this.y-diglet_h+6,diglet_h/3,5);
}
}
//update whether the diglet appears
this.update = function(){
if (this.duration > 0){
this.duration = this.duration - 0.5;
}
else {
var temp_ratio = random(0,1);
if (temp_ratio < 0.15){
this.duration = random([1,2,3,4]);
}
}
}
//check if user clicked the diglet
this.inDiglet = function(click_x,click_y){
if (((click_x > this.x-diglet_h/2) & (click_x < this.x-diglet_h/2) && (click_y > this.y - diglet_h) && (click_y < this.y)) || (dist(click_x,click_y,this.x,this.y-diglet_h) < diglet_h)){
if (this.duration > 0){
this.duration = 0;
num_hit += 1;
}
}
}
}
//check if when the mouse is pressed if it hits any diglet
function mousePressed() {
for (var i = 0; i < num_diglets; i++){
diglets[i].inDiglet(mouseX,mouseY);
}
}
//when the game is over and you press r reset the game
function keyTyped(){
if ((key === 'r') & (ended == 1)){
ended = 0;
time_elapsed = 0;
ended = 0;
num_hit = 0;
}
}