my-sketch.js
>sketch
/* Lan Wei
Section D
lanw@andrew.cmu.edu
Project 05
*/
function setup(){
createCanvas(600, 600);
background(0);
}
var gap = 20; //vertical distance between two waves
var dens = 20; //horizontal density of the waves
var waveRev = 4; // control how big the wave is
function draw(){
//draw ocean
var i = 0;
for (var y = -gap; y < height - gap; y += gap){
noFill();
stroke(255);
beginShape();
i ++; //will be used for color setting
for (var x = -50; x < width + 50; x += dens){
y += random(-gap/waveRev , gap/waveRev );//wave
curveVertex(x, y);
}
curveVertex(width, height);
curveVertex(0, height);
curveVertex(0, y - gap/waveRev);//just to find a point to close the shape
var blue = map(y, 0, height, 255, 30);
var green = map(y, 0, height, 220, 10);
fill(50, green, blue);
noStroke();
endShape();
}
noLoop();
drawFish();
}
//draw fish pattern
function drawFish(){
var dist = 100; //horizontal distance between fish
var level = 70; //vertical distance between fish
i = 0;
for (var x = dist; x < width; x += dist){
i ++;
if (i % 2 == 0){
for (var y = level; y < height; y += level){
fish(x, y);
}
}
else{
for (var y = level * 1/2; y < height; y += level){
fish(x, y);
}
}
}
}
//how the fish looks like
function fish(x, y){
var fishW = 30;
var fishH = 10;
var tailL = 10;
fill(240, 230, 140);
ellipse(x, y, fishW, fishH);//body
fill(0);
ellipse(x-10, y, 5, 5);//eye-black part
fill(255);
ellipse(x - 9, y, 1.5, 1.5);//eye-white part
fill(255, 215, 0);
triangle(x + fishW/2, y, x + fishW/2 + tailL, y + 5, x + fishW/2 + tailL, y - 5);
//tail
}
My initial idea was to create a scene of different depth of the ocean. I want to make the waves of some randomness and change the color to show depth difference. After I finished the waves, I added some fish to fulfill the project requirement, and surprisingly the effect is very good. What I feel the most helpful through the project is that I get familiar with ‘helper functions’ and practiced to create shape with irregular curves.
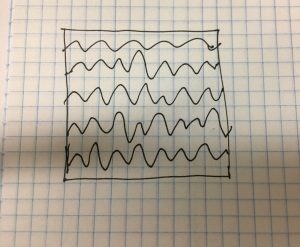
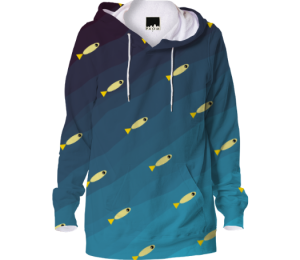