/* Anthony Ra
Section-A
ahra@andrew.cmu.edu
Project-09 */
var heaven;
var start = 100;
/* spelling of heaven as arrays to fill the image */
var name = ["H", "E", "A", "V", "E", "N"];
function preload() {
var heavenUrl = "http://i.imgur.com/ILpL0Fu.jpg" /* uploading picture */
heaven = loadImage(heavenUrl);
}
function setup() {
createCanvas(400, 400);
background(0);
imageMode(CENTER);
heaven.resize(400, 400);
heaven.loadPixels();
frameRate(5000);
}
function draw() {
/* series of sizes for the letters */
var size = random(20, 35);
/* starting point */
var px = randomGaussian(width/2, start);
var py = randomGaussian(height/2, start);
/* values constrained to the picture */
var heavenX = constrain(floor(px), 0, width);
var heavenY = constrain(floor(py), 0, height);
/* getting colors from the picture */
var col = heaven.get(heavenX, heavenY);
/* induces the speed of pixels array */
var i = floor(random(5));
push();
translate(px, py);
noStroke();
fill(col);
textSize(size);
text(name[i], 0, 0);
pop();
}
I don’t have any pictures of myself because .. I don’t. So, I used a personally funny picture of a dear friend who is always salty. Using a play on his name (Evan), I randomized the letters in the word “HEAVEN” and given each color based on the image. The origin of “Heaven” came from a typo from Au Bon Pain. I increased the rate of the letters appearing to an astronomical amount because I just want to see this face haha.
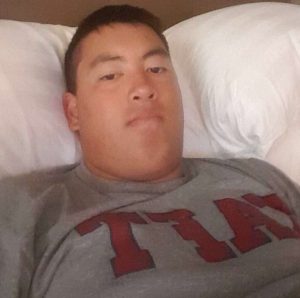
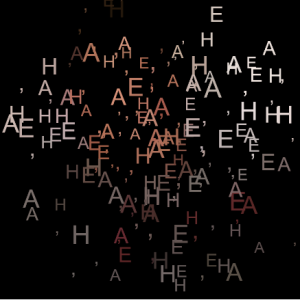
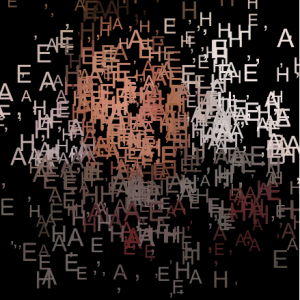
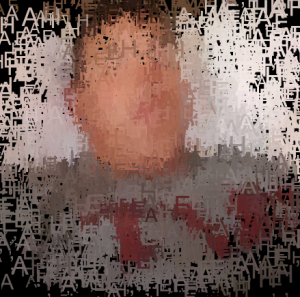