sketch
var x1;//x for first circle
var y1;//y for first circle
var x2;//x for second circle
var y2;//y for second circle
var x3;//x for third circle
var y3;//y for third circle
var x4;//x for fourth circle
var y4;//y for fourth circle
var r=50;//radius
function setup(){
createCanvas(400,300);
background(200);
}
function draw(){
angleMode(DEGREES);
//d is degree of the angle
//To change color for each quarter of the circle
for(var d=90;d<=180; d+=2){
x1=200+r*cos(d);
y1=150+r*sin(d);
x2=200+(r+40)*cos(d+50);
y2=150+(r+40)*sin(d+50);
x3=200+(r+80)*cos(d+10);
y3=150+(r+80)*sin(d+10);
x4=200+(r+120)*cos(d+50);
y4=150+(r+120)*sin(d+50);
//line between 1st and 2nd circle
strokeWeight(0.03);
stroke(255);
line(x2,y2,x1,y1);
//line between 3rd and 1st, 2nd circle
line(x2,y2,x3,y3);
line(x1,y1,x3,y3);
//line between 4th circle and 1st, 2nd, 3rd circle
line(x3,y3,x4,y4);
line(x2,y2,x4,y4);
line(x1,y1,x4,y4);
}
for(var d=180;d<=270; d+=2){
x1=200+r*cos(d);
y1=150+r*sin(d);
x2=200+(r+40)*cos(d+50);
y2=150+(r+40)*sin(d+50);
x3=200+(r+80)*cos(d+10);
y3=150+(r+80)*sin(d+10);
x4=200+(r+120)*cos(d+50);
y4=150+(r+120)*sin(d+50);
//line between 1st and 2nd circle
strokeWeight(0.03);
stroke(0);
line(x2,y2,x1,y1);
//line between 3rd and 1st, 2nd circle
line(x2,y2,x3,y3);
line(x1,y1,x3,y3);
//line between 4th circle and 1st, 2nd, 3rd circle
line(x3,y3,x4,y4);
line(x2,y2,x4,y4);
line(x1,y1,x4,y4);
}
for(var d=270;d<=360; d+=2){
x1=200+r*cos(d);
y1=150+r*sin(d);
x2=200+(r+40)*cos(d+50);
y2=150+(r+40)*sin(d+50);
x3=200+(r+80)*cos(d+10);
y3=150+(r+80)*sin(d+10);
x4=200+(r+120)*cos(d+50);
y4=150+(r+120)*sin(d+50);
//line between 1st and 2nd circle
strokeWeight(0.03);
stroke(120);
line(x2,y2,x1,y1);
//line between 3rd and 1st, 2nd circle
line(x2,y2,x3,y3);
line(x1,y1,x3,y3);
//line between 4th circle and 1st, 2nd, 3rd circle
line(x3,y3,x4,y4);
line(x2,y2,x4,y4);
line(x1,y1,x4,y4);
}
for(var d=0;d<=90; d+=2){
x1=200+r*cos(d);
y1=150+r*sin(d);
x2=200+(r+40)*cos(d+50);
y2=150+(r+40)*sin(d+50);
x3=200+(r+80)*cos(d+10);
y3=150+(r+80)*sin(d+10);
x4=200+(r+120)*cos(d+50);
y4=150+(r+120)*sin(d+50);
//line between 1st and 2nd circle
strokeWeight(0.03);
stroke(230);
line(x2,y2,x1,y1);
//line between 3rd and 1st, 2nd circle
line(x2,y2,x3,y3);
line(x1,y1,x3,y3);
//line between 4th circle and 1st, 2nd, 3rd circle
line(x3,y3,x4,y4);
line(x2,y2,x4,y4);
line(x1,y1,x4,y4);
}
}
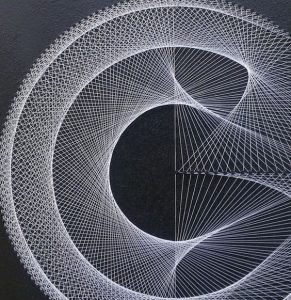
I first saw this image on pinterest. It inspired me to create this string art around a center point in circles. I found out how to find the points on circles and worked my way through.