/*Kevin Riordan
Section C
kzr@andrew.cmu.edu
project_10*/
var trees = [];//make empty trees array
function setup() {
createCanvas(480, 480);
for (var i = 0; i < 6; i++){
var randX = random(-width/5,width + (width / 3));//putting in 6 trees at the start
trees[i] = makeTree(randX);
}
frameRate(10);//setting framerate
}
function draw() {
background('#D8BFD8'); //setting background color
makeBackestMountains();//furthest mountains
makeBackMountains();//next furthest mountains
updateAndDisplayTrees();//moving and displaying trees
removeTreesThatHavePassed();//taking out trees that are safely offscreen
addNewTreesProb();//putting in new trees sometimes
makeFrontMountains();//make foreground
}
//updates and displays trees as they move to the left
function updateAndDisplayTrees(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
//takes trees out of array once they are safely off screen
function removeTreesThatHavePassed(){
var treesKept = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x > -width / 3) {
treesKept.push(trees[i]);
}
}
trees = treesKept;
}
//adds new trees with set chance
function addNewTreesProb() {
var newTreeChance = 0.02;
if (random(0,1) < newTreeChance) {
trees.push(makeTree(width + (width / 3)));
}
}
//moves trees at 2 pixels per call
function treeMove() {
this.x += this.speed;
}
//makes trees with specified things
function makeTree(xCoord) {
var tree = {x: xCoord, speed: -2.0, move: treeMove, display: treeDisplay, size: random(0.5,1.3), grad: round(random(0,3))}
return tree;
}
//draws tree recursively with fractals
function drawTree(x, y, angle, depth, size){
if(depth != 0){
var x2 = x + (Math.cos(angle) * 10 * size * depth);
var y2 = y + (Math.sin(angle) * 15 * size * depth);
line(x, y, x2, y2);
drawTree(x2, y2, angle - (PI / 8), depth - 1, size);//left side of tree
drawTree(x2, y2, angle + (PI / 8), depth - 1, size);//right side of tree
}
}
//sets color based on the grad, 4 different colors possible
function treeDisplay(){
var treeColor;
if(this.grad == 0) {
stroke('#FFF8DC');
}
if(this.grad == 1) {
stroke('#FFEBCD');
}
if(this.grad == 2) {
stroke('#FFE4C4');
}
if(this.grad == 3) {
stroke('#FFDEAD');
}
strokeWeight(2);
drawTree(this.x, height-30, -PI / 2, 6, this.size);//draws actual tree with fifth parameter changing the overall scale of it
}
//makes foreground with given detail, moves at same speed as trees
function makeFrontMountains() {
var terrainSpeed1 = 0.00005;
var terrainDetail1 = 0.005;
stroke('#DAA520');
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail1) + (millis() * terrainSpeed1);
var y1 = map(noise(t), 0,1, 400, height-30);
line(x,y1,x,height);
}
endShape();
}
//makes mountains in background, moves slower than trees and foreground
function makeBackMountains() {
var terrainSpeed2 = 0.000025;
var terrainDetail2 = 0.005;
stroke('#A0522D');
beginShape();
for (var x2 = 0; x2 < width; x2++) {
var u = (x2 * terrainDetail2) + (millis() * terrainSpeed2);
var y2 = map(noise(u), 0,1, 150, height - 30);
line(x2,y2,x2,height);
}
endShape();
}
//makes furthest mountains, moves very slowly
function makeBackestMountains() {
var terrainSpeed3 = 0.0000125;
var terrainDetail3 = 0.01;
stroke('#BC8F8F');
beginShape();
for (var x3 = 0; x3 < width; x3++) {
var v = (x3 * terrainDetail3) + (millis() * terrainSpeed3);
var y3 = map(noise(v), 0,1, 110, height - 150);
line(x3,y3,x3,height);
}
endShape();
}
I found the template for this project very helpful, but the part of this project that took me the most time was figuring out how to make the trees look more realistic. I used recursion and fractals to make the trees look nice, and realizing that I could call the same function inside of itself took a while for me to figure out. I also played around with speeds to make different things move at different speeds. I also found a website that gives color names for different colors, so I like the way my colors fit together for this project. This project made me really comfortable with functions and how they work together. I made the project look pretty close to how I wanted it to look which I am proud of.
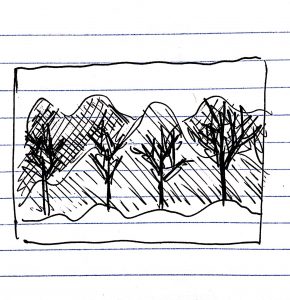