// Han Yu
// hyu1@andrew.cmu.edu
// Section D
// Project 06
var bubbley = 480;
var noiseParam = 0;
var otherbubbley = 510;
function setup() {
createCanvas(480, 480);
noStroke();
frameRate(10);
}
function draw() {
background(41,221,245);
// fectch time
var H=hour();
var M=minute();
var S=second();
// compute location of fish
var hourfish=map(H, 0, 23, 35, width-51);
var minutefish=map(M, 0, 59, 50, width-71);
var secondfish=map(S, 0, 59, 40, width-41);
//bubbles
//sets sidemove to noise value
var sideMove = noise(noiseParam);
//map time sidemove between -20 and 20
sideMove = map(sideMove, 0, 1, -20, 20);
//color of bubble
fill(51,231,255);
//draws bubbles in diff sizes y and x
ellipse(width/2+sideMove,bubbley,50,50);
ellipse(width/2+sideMove+50,bubbley-30,30,30);
ellipse(width/3+sideMove/2, otherbubbley, 40,40);
//make it go up
bubbley -= 10;
otherbubbley -= 5;
//resets to bottome when at top
if (bubbley < -25) {
bubbley = 480
}
if (otherbubbley < -20) {
otherbubbley = 510
}
//increase parameter by 0.3
noiseParam += 0.3
// hour fish
fill(252,237,60);
ellipse(hourfish, 100, 70, 35);
triangle(hourfish+30, 100, hourfish+50,
80, hourfish+50, 120);
// minute fish
fill(246,214,48);
ellipse(minutefish, 200, 100, 50);
triangle(minutefish+45, 200, minutefish+65,
180, minutefish+65, 220);
// second fish
fill(245,184,44);
ellipse(secondfish, 340, 80, 50);
triangle(secondfish-35, 340, secondfish-55,
320, secondfish-55, 360);
// sand
fill(255,249,207);
rect(0, 410, width, height);
}
I started out this project hoping to represent time more interactively, something more than just a clock. I have always been fond of the underwater so I chose it as the theme of my abstract clock. My initial design is attached below. I changed around a bit because I later found out the constant shit of colors is not a good representation of time. Overall I enjoyed doing this project, it helped it lot with learning time application and randomness.
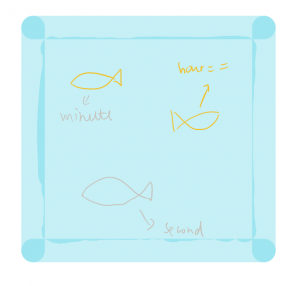