/* Youie Cho
Section E
minyounc@andrew.cmu.edu
Project-05-Wallpaper*/
function setup() {
createCanvas(600, 600);
noStroke();
}
function draw() {
background(0);
drawPattern();
}
function drawPattern() {
var diam = 5; // dots
var r = 0;
var b = 0;
var ratioC = 0; //increase for circle
var ratioR = 0; //increase for rectangle
var increment = 25;
for (var y = increment; y < height; y += increment) {
for (var x = increment; x < width; x+= increment) {
//color gradient
r = map(y, 0, height, 255, 0);
b = map(x, 0, height, 0, 255);
fill(r, 100, b);
//dot
ellipse(x, y, diam, diam);
//outer circle increases from bottom to top
noFill();
stroke(r, 100, b);
strokeWeight(0.5);
ratioC = map(y, 0, height, 110, 0);
ellipse(x, y, diam + ratioC, diam + ratioC);
//inner circle increases from bottom to middle
if(y < width / 2) {
strokeWeight(0.3);
ellipse(x, y, diam + ratioC / 2, diam + ratioC / 2);
//squares decrease from bottom to middle
} else {
rectMode(CENTER);
ratioR = map(y, height, 0, 60, 0);
rect(x, y, diam + ratioR, diam + ratioR);
}
}
}
noLoop();
}
During this project, it was fun to experiment with mapping color, scale, and shapes along vertical and horizontal axes. The pattern shapes are composed of: 1. dot + inner circle + outer circle, and 2. dot + inner circle + square.
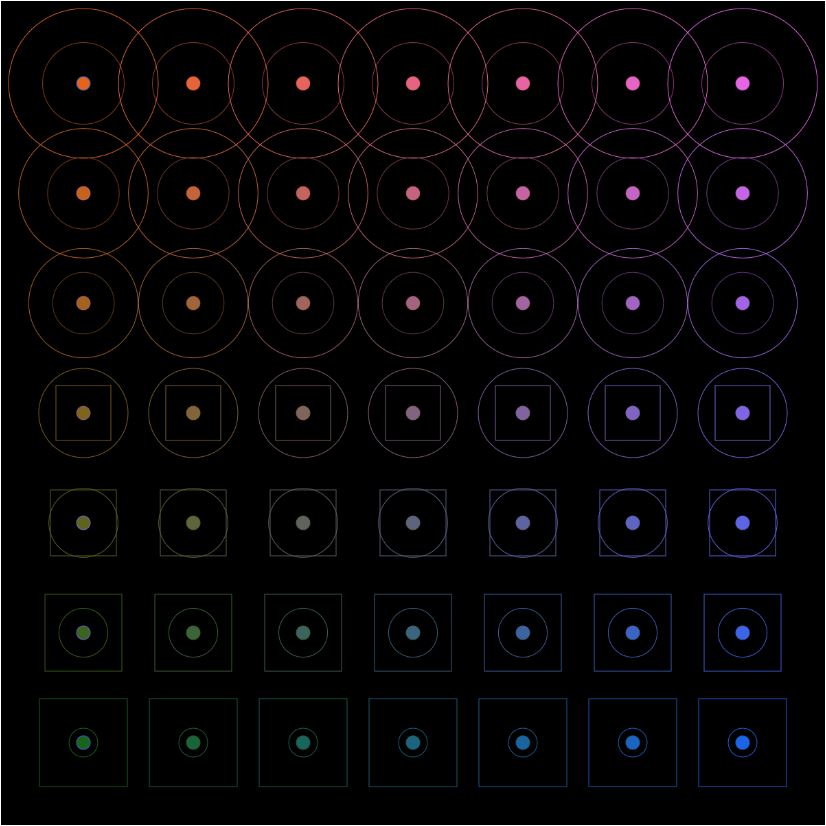