Ikue Mori makes interesting music using electronic drums. I love how she collaborates with multiple other artists to combine each of their unique sounds. It allows her to produce different mixtures of music. I also like how immersive it is with headphones. It always amazes me how people can get the “360º surround sound” by having each earbud play different sounds. Integrating this into the music really adds to the ambience. Mori previously used physical drums, but made the switch to electronic music. The partially computer generated sound probably comes from noises the computer makes that are then manipulated by the artist. Although these sounds come from a computer and are not organic noises from a physical instrument, Mori’s unique sound can still be heard across her works.
Month: September 2021
String Art
var dx1;
var dy1;
var dx2;
var dy2;
function setup() {
createCanvas(400, 300);
background(200);
}
function draw() {
drawLines(0, 50, 400, 200, 150, 300, 100, 100, 50);
drawLines(100, 0, 300, 300, 150, 200, 350, 100, 50);
drawLines(0, 200, 300, 100, 300, 300, 400, 300, 50);
drawLines(350, 100, 300, 200, 150, 300, 350, 100, 50);
noLoop();
}
function drawLines(x1, y1, x2, y2, x3, y3, x4, y4, numLines) {
dx1 = (x3-x1)/numLines;
dy1 = (y3-y1)/numLines;
dx2 = (x4-x2)/numLines;
dy2 = (y4-y2)/numLines;
line(x1, y1, x3, y3);
line(x2, y2, x4, y4);
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
}
Although my art isn’t super cool, I’m glad that I was able to write a method to draw shapes. Now I can spam it as many times as I want and just put in the parameters for where the lines should start and end 🙂
Project 4
// Michelle Dang
//Section D
//mtdang
var dx1;
var dy1;
var dx2;
var dy2;
var dx3;
var dy3;
var dx4;
var dy4;
var numLines = 40; //number of lines
function setup() {
createCanvas(400, 400);
background(0);
dx1 = -600/numLines;
dy1 = 50/numLines;
dx2 = (200)/numLines; //dx4 dx2 is negative
dy2 = (-200)/numLines;
dx3 = 0;
dy3 = 200/numLines;
dx4 = -300/numLines;
dy4 = -200/numLines;
}
function draw() {
background(0);
//white interactive shape
stroke(255);
var x1 = constrain(mouseX, 200, 200);
var y1 = 0;
var x2 = 0;
var y2 = mouseY
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += -dx1;
y1 += dy1;
x2 += -dx2;
y2 += dy2;
}
var x1 = constrain(mouseX, 200, 200);
var y1 = 0;
var x2 = height;
var y2 = mouseY;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
//red shape 1
stroke(255,0,0);
var x1 = width/2;
var y1 = 0;
var x2 = width/2;
var y2 = height;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx3;
y1 += dy3;
x2 += dx4;
y2 += dy4;
}
//red shape 2
var x1 = width/2;
var y1 = 0;
var x2 = width/2;
var y2 = height;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx3;
y1 += dy3;
x2 += -dx4;
y2 += dy4;
}
//blue shape 1
stroke(0,0,255);
var x1 = 0;
var y1 = 0;
var x2 = height;
var y2 = height;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
// blue shape 2
var x1 = width;
var y1 = 0;
var x2 = 0;
var y2 = height;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += -dx1;
y1 += dy1;
x2 += -dx2;
y2 += dy2;
}
}
I struggled a lot at first trying to understand the starting example code, but after playing around with the numbers it wasn’t too bad. I changed some of the variables to be negative to create a symmetrical effect 🙂
LO 4
Michelle Dang (mtdang) Section D
Nigel John Stanford is an artist that created Cymatics, a music video that focuses on chladni plate art. He placed sand on a chladni plate (a sheet of metal) that was attached to a speaker. By playing different tones, the plate is divided into regions that vibrate in opposite directions and the sand shifts to locations where there are no vibrations. This creates symmetrical patterns based on the frequency of the audio. He experimented with different amounts of sand, shapes of chladni plates, and levels of volume as well as what frequencies created the most interesting repeatable patterns. I am interested in how formulaic the relationship is between frequency and the patterns. How does a higher frequency affect the pattern compared to a slightly lower frequency?
Project 4: String Art
var dx1;
var dy1;
var dx2;
var dy2;
var dx3;
var dy3;
var numl = 40;//linenumber
var sw = 0.5;//strokeweight
function setup(){
createCanvas(300,400);
}
function draw(){
background(0);
strokeWeight(1);
//reference red
stroke(183,58,66);
line(10,10,50,250);
line(250,10,250,350);
line(50,250,250,350);
dx1 = (50-10)/numl;
dy1 = (250-10)/numl;
dx2 = (250-250)/numl;
dy2 = (350-10)/numl;
dx3 = (250-50)/numl;
dy3 = (350-250)/numl;
//defineshape
var x1 = 10;
var y1 = 10;
var x2 = 250;
var y2 = 10;
var x3 = 50;
var y3 = 350;
//drawlines
for (var c = 0; c <= numl; c = c + 1){
stroke(255,0,0);
strokeWeight(sw);
//squarelinepath
line(x1,y1,x2,y2);
//trianglelinepath
line(x2,y1,x1,x2);
//curvepath
line(x3,y1,x2,y2);
//rect
line(x1,y1,x2,y1);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
x3 += dx3;
y3 += dy3;
//interaction:changeslineform
if (mouseIsPressed){
x1 += 1;
y1 += 1;
x2 += 3;
y2 += 2;
x3 += 3;
y3 += 3;
}
}
}
I enjoyed coding this project because by changing a few variables, I get to create different interesting compositions. Compared to the previous projects, I feel like this one is more intuitive than planned. During the process, I faced difficulty understanding what each variable does. However, after looking at some examples and experimenting, I understood my variables better and was able to alter them to achieve the effects I wanted.
Looking Outwards-04
The project Forms-string quartet is created by Playmodes. It is a live performance by a string quartet that uses randomly generated graphics as a score. The algorithm uses chance and probability to generate specific graphics that are then played by the string quartet. It is an immersive experience with both electronic music and panoramic visuals. I think that the algorithm uses a set of possible points or graphics and then picks them in random orders for each instrument. The website then says that a synthesis algorithm is used to interpret the vertical fragments of the image and transform them into different aspects of the music. I admire this project mainly because the graphics are very beautiful and to see them and hear the noise interpreted from it is very satisfying. It makes music visually digestible and understandable for the audience which I really enjoy!
Project Name: “FORMS – string quartet”
Artist: Playmodes
Link: https://www.creativeapplications.net/maxmsp/forms-string-quartet/
Project 04: String Art
var dx1;
var dy1;
var dx2;
var dy2;
var dx3;
var dy3;
var dx4;
var dy4;
var dx5;
var dy5;
var dx6;
var dy6;
var dx7;
var dy7;
var dx8;
var dy8;
var numLines = 30;
var numLines2 = 40;
var numLines3 = 50;
var numLines4 = 60;
function setup() {
createCanvas(400, 300);
background(179,212,177);
line(100, 50, 150, 250);
dx1 = (150-75)/numLines;
dy1 = (70-50)/numLines;
dx2 = (250-150)/numLines;
dy2 = (300-250)/numLines;
line(400,0,400,300);
dx3 = (400-0)/numLines2;
dy3 = (0-300)/numLines2;
dx4 = (400-400)/numLines2;
dy4 = (300-0)/numLines2;
line(100, 50, 50, 125);
dx5 = (100-50)/numLines3;
dy5 = (125-50)/numLines3;
dx6 = (350-300)/numLines3;
dy6 = (200-25)/numLines3;
//line(50, 50, 50, 150);
line(400, 100, 400, 300);
dx7 = (400-50)/numLines4;
dy7 = 50/numLines4;
dx8 = (400-50)/numLines4;
dy8 = (300-150)/numLines4;
}
function draw() {
//yellow sunlight ray from upper right hand corner
var x3 = 375;
var y3 = 15;
var x4 = 0;
var y4 = 130;
for (var a = 0; a <= numLines2; a += 1) {
stroke(255,255,102);
line(x3, y3, x4, y4);
x3 += dx3;
y3 += dy3;
x4 += dx4;
y4 += dy4;
}
//green twisty shape that aligns with orange ray
var x7 = 150;
var y7 = 250;
var x8 = 250;
var y8 = 200;
for (var c = 0; c <= numLines4; c += 2) {
stroke(51,102,0);
line(x7, y7, x8, y8);
x7 -= dx7;
y7 -= dy7;
x8 += dx8;
y8 += dy8;
}
//blue "parallelogram" shaped solar panel
var x1 = 100;
var y1 = 50;
var x2 = 150;
var y2 = 250;
for (var i = 0; i <= numLines; i += 1) {
stroke(0, random(90, 105), random(195, 210));
line(x1, y1, x2, y2);
x1 += dx1;
y1 -= dy1;
x2 += dx2;
y2 -= dy2;
}
//orange ray from upper right left corner
var x5 = 0;
var y5 = 0;
var x6 = 500;
var y6 = 150;
for (var b = 0; b <= numLines3; b += 1) {
stroke(255,153,51);
line(x5, y5, x6, y6);
x5 -= dx5;
y5 += dy5;
x6 += dx6;
y6 += dy6;
}
noLoop();
}
I knew that the string “rays” reminded me of sunlight and spotlights, so from there I wanted to add in something (the panel) that would make sense. Given that a lot of solar panels are just above grass, I made the background light green and put a dark green string element just below it.
Project: String Art
//Jacky Lococo
//jlococo
//Section C
var dx1;
var dy1;
var dx2;
var dy2;
var dx3;
var dy3;
var dx4;
var dy4;
var dx5;
var dy5;
var dx6;
var dy6;
var dx7;
var dy7;
var dx8;
var dy8;
var dx9;
var dy9;
var dx10;
var dy10;
var dx11;
var dy11;
var dx12;
var dy12;
var numLines = 50;
var numLines2 = 25;
function setup() {
createCanvas(400, 400);
//peach upper shape
// line(150, 50, 50, 300); //guiding lines to help visualize
// line(250, 50, 350, 300);
dx1 = (50-150)/numLines;
dy1 = (300-50)/numLines;
dx2 = (350-250)/numLines;
dy2 = (300-50)/numLines;
//peach lower shape
// line(150, 350, 50, 100)//guiding lines
// line(250, 350, 350, 100)
dx3 = (50-150)/numLines
dy3 = (100-350)/numLines
dx4 = (350-250)/numLines
dy4 = (100-350)/numLines
//white spiral in the middle of canvas
// line(200, 70, 40, 200); //guiding lines
// line(40, 200, 200, 360);
dx5 = (40-200)/numLines;
dy5 = (200-70)/numLines;
dx6 = (200-40)/numLines;
dy6 = (360-200)/numLines
//burgundy bowtie shape in the back of comp
// line(200, 0, 400, 200) //guiding lines
// line(500, 300, 100, 400)
dx7 = (400-300)/numLines
dy7 = (100-0)/numLines
dx8 = (100-0)/numLines
dy8 = (400-300)/numLines
//top left corner lines
// line(150, 0, 0, 100) //guiding lines
dx9 = (0-150)/numLines
dy9 = (100-0)/numLines
dx10 = (0)/numLines
dy10 = (0)/numLines
//bottom right corner lines
// line(250, 400, 400, 300) //guiding lines
dx11 = (400-250)/numLines
dy11 = (300-400)/numLines
dx12 = (0)/numLines
dy12 = (0)/numLines
}
function draw() {
background(255, 63, 24);
//this is the bowtie shape in the back of the composition
var x7 = 300;
var y7 = 0;
var x8 = 100;
var y8 = 400;
for (var i = 0; i <= numLines; i += 1) {
stroke(80, 13,0)
line(x7, y7, x8, y8);
x7 += dx7;
y7 += dy7;
x8 -= dx8;
y8 -= dy8;
}
//upper peach colored upper curve
var x1 = 150;
var y1 = 50;
var x2 = 350;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1) {
stroke(255, 148, 108)
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 -= dx2;
y2 -= dy2;
}
//lower peach colored curve
var x3 = 150;
var y3 = 350;
var x4 = 350;
var y4 = 100;
for (var i = 0; i <= numLines; i += 1) {
stroke(255, 148, 108)
line(x3, y3, x4, y4);
x3 += dx3;
y3 += dy3;
x4 -= dx4;
y4 -= dy4;
}
//white spiral in the middle dividing the canvas
var x5 = 200;
var y5 = 70;
var x6 = 200;
var y6 = 360;
for (var i = 0; i <= numLines; i += 1) {
stroke(255, 235, 238)
line(x5, y5, x6, y6);
x5 += dx5;
y5 += dy5;
x6 += dx6;
y6 -= dy6;
}
//upper left corner lines
var x9 = 150;
var y9 = 0;
var x10 = 0;
var y10 = 0;
for (var i = 0; i <= numLines; i += 1) {
stroke(255)
line(x9, y9, x10, y10);
x9 += dx9;
y9 += dy9;
x10 += dx10;
y10 -= dy10;
}
//bottom right corner lines
var x11 = 250;
var y11 = 400;
var x12 = 400;
var y12 = 400;
for (var i = 0; i <= numLines; i += 1) {
stroke(255)
line(x11, y11, x12, y12);
x11 += dx11;
y11 += dy11;
x12 += dx12;
y12 += dy12;
}
//Inner circles, radius decreases by 5 for each
//largest circle
stroke(255)
strokeWeight(1)
fill(80, 13, 0)
ellipse(200, 200, 50, 50)
stroke(255)
strokeWeight(1)
fill(80, 13, 0)
ellipse(200, 200, 45, 45)
stroke(255)
strokeWeight(1)
fill(80, 13, 0)
ellipse(200, 200, 40, 40)
//smallest circle
stroke(255)
strokeWeight(1)
fill(80, 13, 0)
ellipse(200, 200, 35, 35)
noLoop()
}
This project was a little bit difficult, I think I mainly approached it by trying to find patterns within the code given and use that to make any desired shapes. Once I got the hang of the process and why certain numbers were used, it became a lot easier.
Looking Outwards 04: Sound Art
Project Title: Music For Robots
Year of Creation: 2014
Artists: Squarepusher x Z-Machines
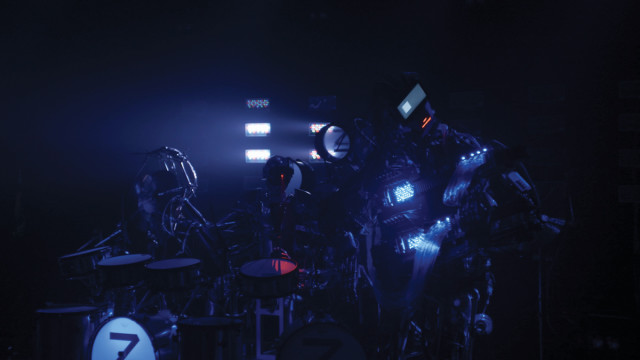
I admire how the robots in this project can together create such exciting and layered music because it’s wildly entertaining despite sounding admittedly quite choppy. I admire the rigid precision of the performances because although such precision is impossible for humans to obtain, it reminds me of what robots in this artwork can’t really obtain, portray or evoke: emotional response. I’m inspired by the innovation and attention to detail, but I must say that the music didn’t leave me feeling a certain way, which in my opinion, music should do. Of course, I don’t think the primary purpose of this endeavor was to make music specifically for people to enjoy. I know that the creators used “custom build mechanics” and Max MSP patches, which lets you connect software and objects with virtual patch cords. Squarepusher’s music interests include electronic, jazz and drum and bass. These artistic sensibilities manifested in the ASHURA (the robot drummer that plays over 20 drums). I could hear how the creator included some free jazz elements into the overall technical electronic experience.
Looking Outwards 04: Sound Art
The project I have chosen for this blog post is Pamela Z’s 2012 work “Fount”. “Fount” is a sound and visual art installation piece using projection and speakers (or a sound dome). The images displayed and sound projected are triggered by the presence of a viewer. With no viewer present, the image is blurred and no sound comes out of the speakers. Once a person is in the space, the image clears to reveal a beaker pouring water into a basin; the speakers play an audio of water pouring which accompanies the video. Although the piece itself is not generated by a computer algorithm, the intended performance of the piece involves a computer system sensing the presence of a viewer and setting the correct programs into motion. I think this piece of art is interesting because it requires interaction with a viewer, although the viewer only needs to be present. This sort of semi-interactive concept is intriguing from the perspective of an audience member like the piece is telling a secret to one viewer at a time.