// coordinated for basic star shape:
//lineA:
var lineAx1 = 0
var lineAy1 = 0
var lineAx2 = 0
var lineAy2 = -125
//lineB:
var lineBx1 = 119
var lineBy1 = -39
var lineBx2 = 0
var lineBy2 = 0
//lineC:
var lineCx1 = 0
var lineCy1 = 0
var lineCx2 = 73
var lineCy2 = 101
//lineD:
var lineDx1 = -73
var lineDy1 = 101
var lineDx2 = 0
var lineDy2 = 0
//lineE:
var lineEx1 = 0
var lineEy1 = 0
var lineEx2 = -119
var lineEy2 = -39
//distance for string art end points variables initialized:
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 40; //number of string art lines in each 'shape'
var rot = 0 //rotation effect (DISABLED bc of noLoop() call; comment out noLoop() to see rotation)
// color variables for shading effect:
var r = 0;
var g = 50;
var b = 100;
function setup() {
createCanvas(400, 300);
background(200);
}
function draw() {
background(200); // called for rotation effect
translate(width/2, height/2); // center canvas
//rotation effect (DISABLED while noLoop() is activated):
rotate(radians(rot));
rot += 1;
// color variables for shade change effect re-defined after each rotation:
r = 0;
g = 50;
b = 100;
//lines to create basic star shape, coordinates set by variables:
line(lineAx1, lineAy1, lineAx2, lineAy2);
line(lineBx1, lineBy1, lineBx2, lineBy2);
line(lineCx1, lineCy1, lineCx2, lineCy2);
line(lineDx1, lineDy1, lineDx2, lineDy2);
line(lineEx1, lineEy1, lineEx2, lineEy2);
// drawLines() called to create the string art effect:
drawLines(lineAx1, lineAy1, lineAx2, lineAy2, lineBx1, lineBy1, lineBx2, lineBy2);
drawLines(lineBx1, lineBy1, lineBx2, lineBy2, lineCx1, lineCy1, lineCx2, lineCy2);
drawLines(lineCx1, lineCy1, lineCx2, lineCy2, lineDx1, lineDy1, lineDx2, lineDy2);
drawLines(lineDx1, lineDy1, lineDx2, lineDy2, lineEx1, lineEy1, lineEx2, lineEy2);
drawLines(lineEx2, lineEy2, lineEx1, lineEy1, lineAx1, lineAy1, lineAx2, lineAy2);
// comment noLoop() out to see roration:
noLoop();
}
//drawLines function made for string art effect, takes x and y coordinates of both connecting lines (8 parameters):
function drawLines(line1x1, line1y1, line1x2, line1y2, line2x1, line2y1, line2x2, line2y2) {
// set distance between end points for string lines:
dx1 = (line1x2-line1x1)/numLines;
dy1 = (line1y2-line1y1)/numLines;
dx2 = (line2x2-line2x1)/numLines;
dy2 = (line2y2-line2y1)/numLines;
//initialize variables for string line end points:
var x1 = line1x1;
var y1 = line1y1;
var x2 = line2x1;
var y2 = line2y1;
// string lines drawn until specified number of lines is hit:
for (var i = 0; i <= numLines; i += 1) {
stroke(r, g, b);
line(x1, y1, x2, y2);
// string line variables update to create string art effect:
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
//color effect:
if (i <= numLines/2) {
r += 255/numLines;
g -= 255/numLines;
b += 100/numLines;
} else {
r -= 255/numLines;
g += 255/numLines;
b -= 100/numLines;
}
}
}
I decided to do a star shape for this project, and I’ve attached an image of my initial sketches. It took me a while to figure out how I wanted to use the ‘string art’ style, but I like the outcome that this method created.
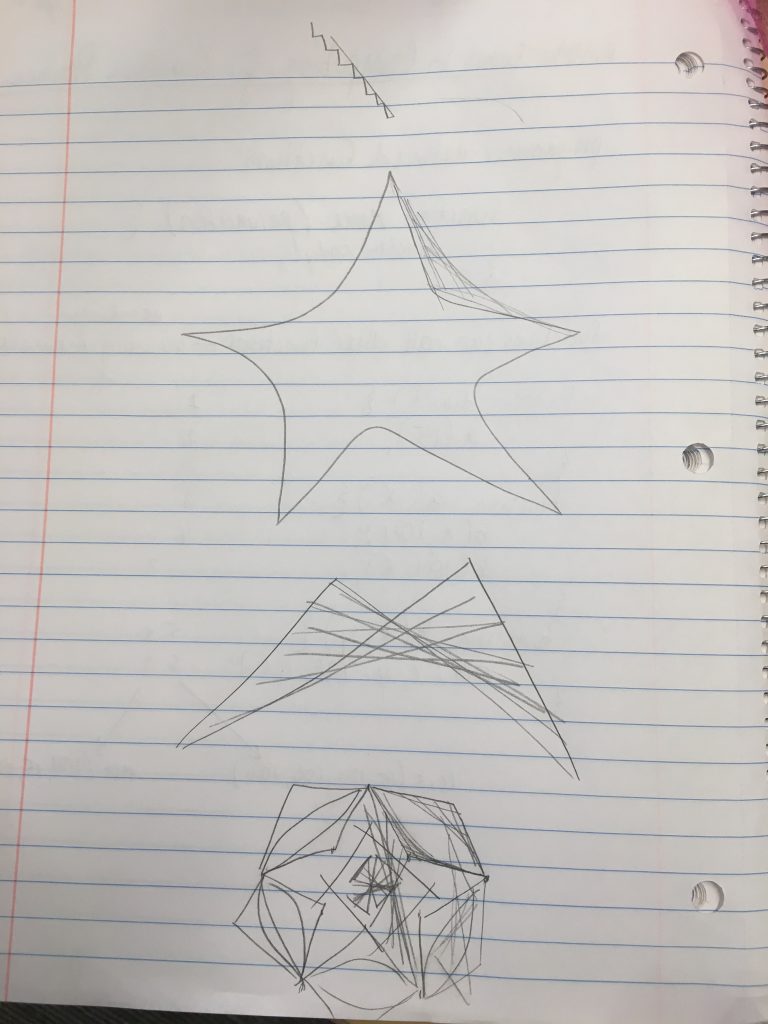